Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / MS / Internal / IListConverters.cs / 1305600 / IListConverters.cs
//---------------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // Description: Converters for IList, IList , IList // IList and IList . // // History: // 7/20/2005 garyyang - created // //--------------------------------------------------------------------------- using System; using System.Collections.Generic; using System.ComponentModel; using System.Globalization; using System.Text; using System.Windows; using MS.Internal; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; using MS.Internal.PresentationCore; namespace System.Windows.Media.Converters { // // The following are IList converters for IList , IList , IList , IList , IList . // More code sharing might be achieved by creating a generic TypeConverter. But XAML parser doesn't // support generic TypeConverter now. // /// /// The base converter for IList of T to string conversion in XAML serialization /// [FriendAccessAllowed] // all implementations are used by Framework at serialization public abstract class BaseIListConverter : TypeConverter { ////// Indicates if a type can be converted from (returns true for string). /// public override bool CanConvertFrom(ITypeDescriptorContext td, Type t) { return t == typeof(string); // can only convert from string } ////// Indicates if a type can be converted to (returns true for string). /// public override bool CanConvertTo(ITypeDescriptorContext context, Type destinationType) { return destinationType == typeof(string); // can only convert to string } ////// Converts from a string. /// public override object ConvertFrom(ITypeDescriptorContext td, CultureInfo ci, object value) { if (null == value) { throw GetConvertFromException(value); } string s = value as string; if (null == s) { throw new ArgumentException(SR.Get(SRID.General_BadType, "ConvertFrom"), "value"); } return ConvertFromCore(td, ci, s); } ////// Converts to a string /// public override object ConvertTo(ITypeDescriptorContext context, CultureInfo culture, object value, Type destinationType) { if (destinationType != null) return ConvertToCore(context, culture, value, destinationType); // Pass unhandled cases to base class (which will throw exceptions for null value or destinationType.) return base.ConvertTo(context, culture, value, destinationType); } ////// To be implemented by subclasses to convert to various types from string. /// internal abstract object ConvertFromCore(ITypeDescriptorContext td, CultureInfo ci, string value); ////// To be implemented by subclasses to convert string to various types. /// internal abstract object ConvertToCore(ITypeDescriptorContext context, CultureInfo culture, object value, Type destinationType); internal TokenizerHelper _tokenizer; internal const char DelimiterChar = ' '; } ////// TypeConverter to convert IList of double to and from string. /// The converted string is a sequence of delimited double number. /// public sealed class DoubleIListConverter : BaseIListConverter { internal sealed override object ConvertFromCore(ITypeDescriptorContext td, CultureInfo ci, string value) { _tokenizer = new TokenizerHelper(value, '\0' /* quote char */, DelimiterChar); // Estimate the output list's capacity from length of the input string. Listlist = new List (Math.Min(256, value.Length / EstimatedCharCountPerItem + 1)); while (_tokenizer.NextToken()) { list.Add(Convert.ToDouble(_tokenizer.GetCurrentToken(), ci)); } return list; } internal override object ConvertToCore(ITypeDescriptorContext context, CultureInfo culture, object value, Type destinationType) { IList list = value as IList ; if (list == null) { throw GetConvertToException(value, destinationType); } // Estimate the output string's length from the element count of the List. StringBuilder builder = new StringBuilder(EstimatedCharCountPerItem * list.Count); for (int i = 0; i < list.Count; i++) { if (i > 0) builder.Append(DelimiterChar); builder.Append(list[i].ToString(culture)); } return builder.ToString(); } // A double is estimated to take 5 characters on average, plus 1 delimiter, e.g. "5.328 ". private const int EstimatedCharCountPerItem = 6; } /// /// TypeConverter to convert IList of ushort to and from string. /// The converted string is a sequence of delimited ushort number. /// public sealed class UShortIListConverter : BaseIListConverter { internal override object ConvertFromCore(ITypeDescriptorContext td, CultureInfo ci, string value) { _tokenizer = new TokenizerHelper(value, '\0' /* quote char */, DelimiterChar); Listlist = new List (Math.Min(256, value.Length / EstimatedCharCountPerItem + 1)); while (_tokenizer.NextToken()) { list.Add(Convert.ToUInt16(_tokenizer.GetCurrentToken(), ci)); } return list; } internal override object ConvertToCore(ITypeDescriptorContext context, CultureInfo culture, object value, Type destinationType) { IList list = value as IList ; if (list == null) { throw GetConvertToException(value, destinationType); } StringBuilder builder = new StringBuilder(EstimatedCharCountPerItem * list.Count); for (int i = 0; i < list.Count; i++) { if (i > 0) builder.Append(DelimiterChar); builder.Append(list[i].ToString(culture)); } return builder.ToString(); } // A ushort is estimated to take 2 characters on average, plus 1 delimiter. private const int EstimatedCharCountPerItem = 3; } /// /// TypeConverter to convert IList of bool to and from string. /// The converted string is a sequence of delimited 0s and 1s. "0" for false and "1" for true. /// public sealed class BoolIListConverter : BaseIListConverter { internal override object ConvertFromCore(ITypeDescriptorContext td, CultureInfo ci, string value) { _tokenizer = new TokenizerHelper(value, '\0' /* quote char */, DelimiterChar); Listlist = new List (Math.Min(256, value.Length / EstimatedCharCountPerItem + 1)); while (_tokenizer.NextToken()) { list.Add(Convert.ToInt32(_tokenizer.GetCurrentToken(), ci) != 0); } return list; } internal override object ConvertToCore(ITypeDescriptorContext context, CultureInfo culture, object value, Type destinationType) { IList list = value as IList ; if (list == null) { throw GetConvertToException(value, destinationType); } StringBuilder builder = new StringBuilder(EstimatedCharCountPerItem * list.Count); for (int i = 0; i < list.Count; i++) { if (i > 0) builder.Append(DelimiterChar); builder.Append((list[i] ? 1 : 0)); } return builder.ToString(); } // A bool takes 1 character plus 1 delimiter. private const int EstimatedCharCountPerItem = 2; } /// /// TypeConverter to convert IList of Point to and from string. /// The converted string is a sequence of delimited Point values. Point values are converted by PointConverter. /// public sealed class PointIListConverter : BaseIListConverter { internal override object ConvertFromCore(ITypeDescriptorContext td, CultureInfo ci, string value) { _tokenizer = new TokenizerHelper(value, '\0' /* quote char */, DelimiterChar); Listlist = new List (Math.Min(256, value.Length / EstimatedCharCountPerItem + 1)); while (_tokenizer.NextToken()) { list.Add((Point) converter.ConvertFrom(td, ci, _tokenizer.GetCurrentToken())); } return list; } internal override object ConvertToCore(ITypeDescriptorContext context, CultureInfo culture, object value, Type destinationType) { IList list = value as IList ; if (list == null) { throw GetConvertToException(value, destinationType); } StringBuilder builder = new StringBuilder(EstimatedCharCountPerItem * list.Count); for (int i = 0; i < list.Count; i++) { if (i > 0) builder.Append(DelimiterChar); builder.Append((string) converter.ConvertTo(context, culture, list[i], typeof(string))); } return builder.ToString(); } private PointConverter converter = new PointConverter(); // A point takes 2 double and 2 delimiters. Estimated to be 12 characters. private const int EstimatedCharCountPerItem = 12; } /// /// TypeConverter to convert IList of Char to and from string. /// The converted string is a concatenation of all the chars in the IList. There is no delimiter. /// public sealed class CharIListConverter : BaseIListConverter { internal override object ConvertFromCore(ITypeDescriptorContext td, CultureInfo ci, string value) { return value.ToCharArray(); } internal override object ConvertToCore(ITypeDescriptorContext context, CultureInfo culture, object value, Type destinationType) { IListlist = value as IList ; if (list == null) { throw GetConvertToException(value, destinationType); } // An intermediate allocation is needed to construct the string as string doesn't take IList // in its constructor. char[] chars = new char[list.Count]; list.CopyTo(chars, 0); return new string(chars); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
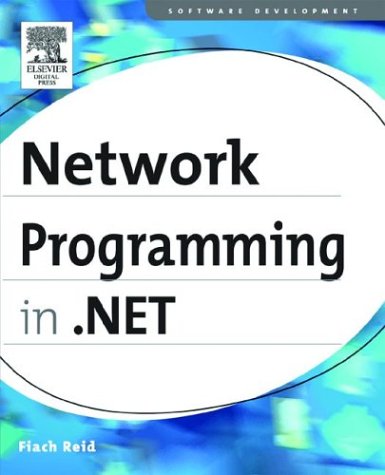
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Delegate.cs
- DynamicQueryableWrapper.cs
- DataViewListener.cs
- Random.cs
- MediaPlayer.cs
- DataProtection.cs
- SqlSelectStatement.cs
- UnsafeNativeMethodsPenimc.cs
- ClientUrlResolverWrapper.cs
- TransportationConfigurationTypeInstallComponent.cs
- GridToolTip.cs
- SqlConnectionHelper.cs
- PriorityRange.cs
- XmlDocumentFieldSchema.cs
- _Semaphore.cs
- HttpStreams.cs
- CacheDependency.cs
- WindowsEditBoxRange.cs
- HttpStaticObjectsCollectionBase.cs
- CodeDelegateCreateExpression.cs
- Missing.cs
- clipboard.cs
- PathFigureCollectionConverter.cs
- Rotation3DAnimation.cs
- PreservationFileWriter.cs
- DataGridItemEventArgs.cs
- OdbcFactory.cs
- EntityTransaction.cs
- ExtenderControl.cs
- XmlComplianceUtil.cs
- ACL.cs
- TypeNameConverter.cs
- SmuggledIUnknown.cs
- RequestQueue.cs
- WasHttpModulesInstallComponent.cs
- TextParagraphView.cs
- MembershipUser.cs
- NotifyInputEventArgs.cs
- FixedFindEngine.cs
- PagesChangedEventArgs.cs
- XmlNamespaceManager.cs
- D3DImage.cs
- documentation.cs
- StandardBindingOptionalReliableSessionElement.cs
- TextDecoration.cs
- ProfileSettings.cs
- ConfigXmlAttribute.cs
- DriveInfo.cs
- ExternalFile.cs
- InkCanvasFeedbackAdorner.cs
- DependencyObject.cs
- ZipIOCentralDirectoryBlock.cs
- CodePrimitiveExpression.cs
- ProcessHostMapPath.cs
- ValidatingReaderNodeData.cs
- SpellCheck.cs
- ContainerUIElement3D.cs
- ApplicationException.cs
- SpeechRecognizer.cs
- _NegotiateClient.cs
- TextRange.cs
- DataObjectCopyingEventArgs.cs
- SimpleFileLog.cs
- CodeTypeMemberCollection.cs
- CompositeFontParser.cs
- TypeHelpers.cs
- XmlChoiceIdentifierAttribute.cs
- nulltextcontainer.cs
- UpdatePanelControlTrigger.cs
- Win32Native.cs
- TextureBrush.cs
- AsyncStreamReader.cs
- EmptyElement.cs
- PermissionAttributes.cs
- StorageInfo.cs
- Misc.cs
- ServiceAuthorizationElement.cs
- EntityDesignerDataSourceView.cs
- EntityCommand.cs
- SqlPersonalizationProvider.cs
- XmlConvert.cs
- ConfigurationLocation.cs
- DocumentReferenceCollection.cs
- ErrorView.xaml.cs
- GridItem.cs
- SqlUnionizer.cs
- ChameleonKey.cs
- SystemResources.cs
- Renderer.cs
- SafeViewOfFileHandle.cs
- ButtonColumn.cs
- DbDataReader.cs
- SignatureConfirmationElement.cs
- DelegatingConfigHost.cs
- UnsupportedPolicyOptionsException.cs
- CopyCodeAction.cs
- SafeRightsManagementSessionHandle.cs
- IndexerNameAttribute.cs
- NullableBoolConverter.cs
- ToolTip.cs