Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / clr / src / BCL / System / Collections / EmptyReadOnlyDictionaryInternal.cs / 1305376 / EmptyReadOnlyDictionaryInternal.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== /*============================================================ ** ** Class: EmptyReadOnlyDictionaryInternal ** **[....] ** ** ** Purpose: List for exceptions. ** ** ===========================================================*/ using System.Diagnostics.Contracts; namespace System.Collections { /// This is a simple implementation of IDictionary that is empty and readonly. [Serializable] internal sealed class EmptyReadOnlyDictionaryInternal: IDictionary { // Note that this class must be agile with respect to AppDomains. See its usage in // System.Exception to understand why this is the case. public EmptyReadOnlyDictionaryInternal() { } // IEnumerable members IEnumerator IEnumerable.GetEnumerator() { return new NodeEnumerator(); } // ICollection members public void CopyTo(Array array, int index) { if (array==null) throw new ArgumentNullException("array"); if (array.Rank != 1) throw new ArgumentException(Environment.GetResourceString("Arg_RankMultiDimNotSupported")); if (index < 0) throw new ArgumentOutOfRangeException("index", Environment.GetResourceString("ArgumentOutOfRange_NeedNonNegNum")); if ( array.Length - index < this.Count ) throw new ArgumentException( Environment.GetResourceString("ArgumentOutOfRange_Index"), "index"); Contract.EndContractBlock(); // the actual copy is a NOP } public int Count { get { return 0; } } public Object SyncRoot { get { return this; } } public bool IsSynchronized { get { return false; } } // IDictionary members public Object this[Object key] { get { if (key == null) { throw new ArgumentNullException("key", Environment.GetResourceString("ArgumentNull_Key")); } Contract.EndContractBlock(); return null; } set { if (key == null) { throw new ArgumentNullException("key", Environment.GetResourceString("ArgumentNull_Key")); } if (!key.GetType().IsSerializable) throw new ArgumentException(Environment.GetResourceString("Argument_NotSerializable"), "key"); if( (value != null) && (!value.GetType().IsSerializable ) ) throw new ArgumentException(Environment.GetResourceString("Argument_NotSerializable"), "value"); Contract.EndContractBlock(); throw new InvalidOperationException(Environment.GetResourceString("InvalidOperation_ReadOnly")); } } public ICollection Keys { get { return new Object[0]; } } public ICollection Values { get { return new Object[0]; } } public bool Contains(Object key) { return false; } public void Add(Object key, Object value) { if (key == null) { throw new ArgumentNullException("key", Environment.GetResourceString("ArgumentNull_Key")); } if (!key.GetType().IsSerializable) throw new ArgumentException(Environment.GetResourceString("Argument_NotSerializable"), "key" ); if( (value != null) && (!value.GetType().IsSerializable) ) throw new ArgumentException(Environment.GetResourceString("Argument_NotSerializable"), "value"); Contract.EndContractBlock(); throw new InvalidOperationException(Environment.GetResourceString("InvalidOperation_ReadOnly")); } public void Clear() { throw new InvalidOperationException(Environment.GetResourceString("InvalidOperation_ReadOnly")); } public bool IsReadOnly { get { return true; } } public bool IsFixedSize { get { return true; } } public IDictionaryEnumerator GetEnumerator() { return new NodeEnumerator(); } public void Remove(Object key) { throw new InvalidOperationException(Environment.GetResourceString("InvalidOperation_ReadOnly")); } private sealed class NodeEnumerator : IDictionaryEnumerator { public NodeEnumerator() { } // IEnumerator members public bool MoveNext() { return false; } public Object Current { get { throw new InvalidOperationException(Environment.GetResourceString("InvalidOperation_EnumOpCantHappen")); } } public void Reset() { } // IDictionaryEnumerator members public Object Key { get { throw new InvalidOperationException(Environment.GetResourceString("InvalidOperation_EnumOpCantHappen")); } } public Object Value { get { throw new InvalidOperationException(Environment.GetResourceString("InvalidOperation_EnumOpCantHappen")); } } public DictionaryEntry Entry { get { throw new InvalidOperationException(Environment.GetResourceString("InvalidOperation_EnumOpCantHappen")); } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
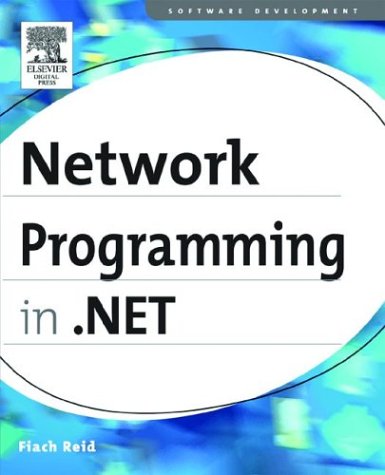
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- HttpModulesSection.cs
- DbConnectionFactory.cs
- XPathAncestorQuery.cs
- MissingMemberException.cs
- LogLogRecordHeader.cs
- CatalogPart.cs
- UnsafeNetInfoNativeMethods.cs
- RelativeSource.cs
- HotSpotCollection.cs
- HtmlTable.cs
- XmlSchema.cs
- DependencyPropertyKey.cs
- RangeBase.cs
- SrgsSemanticInterpretationTag.cs
- TypeToTreeConverter.cs
- SiteMapNode.cs
- LabelDesigner.cs
- CallbackValidatorAttribute.cs
- String.cs
- TableAutomationPeer.cs
- CLRBindingWorker.cs
- Blend.cs
- XmlSerializerNamespaces.cs
- DirectoryLocalQuery.cs
- ComplusTypeValidator.cs
- FieldDescriptor.cs
- MergeFailedEvent.cs
- BinaryObjectInfo.cs
- ModelMemberCollection.cs
- ComponentGuaranteesAttribute.cs
- LinearGradientBrush.cs
- QuotedPrintableStream.cs
- CodeActivityContext.cs
- InstanceData.cs
- XPathScanner.cs
- DebugTracing.cs
- WrappedKeySecurityToken.cs
- AsyncSerializedWorker.cs
- StagingAreaInputItem.cs
- Command.cs
- CodeObject.cs
- ComboBoxRenderer.cs
- SafeNativeMethods.cs
- DataBinder.cs
- GCHandleCookieTable.cs
- FormattedTextSymbols.cs
- EntitySqlQueryBuilder.cs
- DateTimeFormat.cs
- TextEditorDragDrop.cs
- XslTransform.cs
- IdentityModelStringsVersion1.cs
- HostVisual.cs
- Classification.cs
- WebPartConnectVerb.cs
- DecoderReplacementFallback.cs
- DrawingAttributesDefaultValueFactory.cs
- XhtmlBasicLinkAdapter.cs
- DomainConstraint.cs
- SqlCacheDependencyDatabaseCollection.cs
- ZoneMembershipCondition.cs
- _IPv6Address.cs
- MouseActionValueSerializer.cs
- ReadOnlyHierarchicalDataSourceView.cs
- OdbcParameter.cs
- Color.cs
- PenContext.cs
- VariableAction.cs
- GridLengthConverter.cs
- MemoryRecordBuffer.cs
- InkCanvasFeedbackAdorner.cs
- DataGrid.cs
- TextSimpleMarkerProperties.cs
- BitmapEffectInput.cs
- RouteUrlExpressionBuilder.cs
- MarshalDirectiveException.cs
- CSharpCodeProvider.cs
- GeometryDrawing.cs
- httpstaticobjectscollection.cs
- FormViewInsertedEventArgs.cs
- SiteMapProvider.cs
- ValidatingPropertiesEventArgs.cs
- SQLInt32Storage.cs
- InputScope.cs
- OutputWindow.cs
- WebPartConnectionsCancelEventArgs.cs
- WebResourceAttribute.cs
- SiteMap.cs
- DbDataSourceEnumerator.cs
- MarginCollapsingState.cs
- RequestQueue.cs
- BulletedListEventArgs.cs
- Pens.cs
- DelayedRegex.cs
- OracleConnection.cs
- RangeValuePattern.cs
- StylusPointPropertyInfoDefaults.cs
- SchemaDeclBase.cs
- NamedPipeConnectionPool.cs
- FilterableAttribute.cs
- XmlUtil.cs