Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx35 / System.WorkflowServices / System / ServiceModel / Dispatcher / WorkflowRequestContext.cs / 1305376 / WorkflowRequestContext.cs
//------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace System.ServiceModel.Dispatcher { using System.Collections.Generic; using System.Collections.ObjectModel; using System.Diagnostics.CodeAnalysis; using System.IdentityModel.Policy; using System.Runtime; using System.Runtime.Diagnostics; using System.Runtime.Serialization; using System.ServiceModel.Diagnostics; using System.ServiceModel.Security; using System.Diagnostics; [Serializable] class WorkflowRequestContext { [NonSerialized] WorkflowOperationAsyncResult asyncResult; [NonSerialized] AuthorizationContext authorizationContext; IDictionarycontextProperties; ReadOnlyCollection
Link Menu
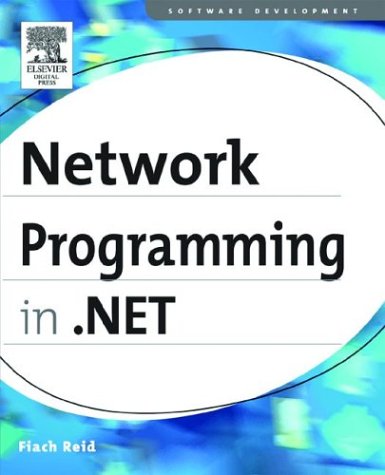
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SelectionRangeConverter.cs
- FrameworkContentElement.cs
- RichTextBoxConstants.cs
- CookieProtection.cs
- MapPathBasedVirtualPathProvider.cs
- FlowDocument.cs
- TemplateBindingExpression.cs
- XmlSchemaAnyAttribute.cs
- SiteMapNodeItem.cs
- clipboard.cs
- DelegateArgumentReference.cs
- TypeDelegator.cs
- InvalidDataContractException.cs
- DesigntimeLicenseContext.cs
- ConsoleKeyInfo.cs
- FollowerQueueCreator.cs
- RadioButtonPopupAdapter.cs
- MembershipUser.cs
- Dump.cs
- PropagatorResult.cs
- ObjectDataSourceDesigner.cs
- DeleteIndexBinder.cs
- ListItemCollection.cs
- VoiceChangeEventArgs.cs
- CodeAttributeArgumentCollection.cs
- LogEntry.cs
- PeerCustomResolverSettings.cs
- CollectionBuilder.cs
- TextSchema.cs
- ContainsSearchOperator.cs
- CannotUnloadAppDomainException.cs
- GlyphRunDrawing.cs
- Table.cs
- MessagePropertyVariants.cs
- InkPresenterAutomationPeer.cs
- ManagementOperationWatcher.cs
- Duration.cs
- ProfileEventArgs.cs
- DataGridViewIntLinkedList.cs
- MsmqIntegrationAppDomainProtocolHandler.cs
- DbLambda.cs
- ViewGenResults.cs
- ListCollectionView.cs
- Grid.cs
- GeometryGroup.cs
- BitArray.cs
- ActiveDocumentEvent.cs
- Executor.cs
- MsmqIntegrationSecurity.cs
- SqlUserDefinedAggregateAttribute.cs
- LassoSelectionBehavior.cs
- RegexGroupCollection.cs
- WhitespaceRule.cs
- ServiceAuthorizationManager.cs
- FrameSecurityDescriptor.cs
- DataSourceProvider.cs
- XmlSerializerSection.cs
- TextWriter.cs
- FixedSOMTable.cs
- TimeSpanSecondsOrInfiniteConverter.cs
- OTFRasterizer.cs
- TreeNodeStyleCollection.cs
- DetailsViewAutoFormat.cs
- GregorianCalendar.cs
- SettingsContext.cs
- TcpActivation.cs
- BindableTemplateBuilder.cs
- DataControlImageButton.cs
- XmlAnyElementAttribute.cs
- StringKeyFrameCollection.cs
- ListViewTableRow.cs
- ObjectTag.cs
- ParameterToken.cs
- Walker.cs
- Line.cs
- RealizationContext.cs
- EntityDataSourceColumn.cs
- EmptyElement.cs
- InstancePersistenceEvent.cs
- XmlIlGenerator.cs
- PairComparer.cs
- Util.cs
- ClosableStream.cs
- ContextMarshalException.cs
- SpecularMaterial.cs
- QualifiedCellIdBoolean.cs
- XmlDownloadManager.cs
- ComponentDispatcherThread.cs
- FamilyMap.cs
- HttpCachePolicy.cs
- EntityRecordInfo.cs
- SystemDropShadowChrome.cs
- SqlCacheDependency.cs
- TextReader.cs
- BaseCollection.cs
- SharedPersonalizationStateInfo.cs
- ComponentEditorForm.cs
- QuestionEventArgs.cs
- PrinterUnitConvert.cs
- Identifier.cs