Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / ndp / fx / src / DataWeb / Client / System / Data / Services / Client / ALinq / ResourceExpression.cs / 1 / ResourceExpression.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// Base class for expressions representing resources // // // @owner [....] //--------------------------------------------------------------------- namespace System.Data.Services.Client { using System; using System.Diagnostics; using System.Collections.Generic; using System.Linq; using System.Linq.Expressions; ////// Abstract base class for expressions that support Query Options /// internal abstract class ResourceExpression : Expression { ///Singleton InputReferenceExpression that should be used to indicate a reference to this element of the resource path internal InputReferenceExpression inputRef; ///expand paths private ListexpandPaths; /// custom query options private DictionarycustomQueryOptions; /// /// Creates a Resource expression /// /// the return type of the expression internal ResourceExpression(ExpressionType nodeType, Type type, ListexpandPaths, Dictionary customQueryOptions) : base(nodeType, type) { this.expandPaths = expandPaths ?? new List (); this.customQueryOptions = customQueryOptions ?? new Dictionary (); } abstract internal ResourceExpression Cast(Type type); abstract internal bool HasQueryOptions { get; } abstract internal Type ResourceType { get; } /// /// Does this expression produce at most 1 resource? /// abstract internal bool IsSingleton { get; } ////// Expand query option for ResourceSet /// internal virtual ListExpandPaths { get { return this.expandPaths; } set { this.expandPaths = value; } } /// /// custom query options for ResourceSet /// internal virtual DictionaryCustomQueryOptions { get { return this.customQueryOptions; } set { this.customQueryOptions = value; } } /// /// Creates an ///that refers to this component of the resource path. /// The returned expression is guaranteed to be reference-equal (object.ReferenceEquals) /// to any other InputReferenceExpression that also refers to this resource path component. /// The InputReferenceExpression that refers to this resource path component internal InputReferenceExpression CreateReference() { if (this.inputRef == null) { this.inputRef = new InputReferenceExpression(this.ResourceType, this); } return this.inputRef; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
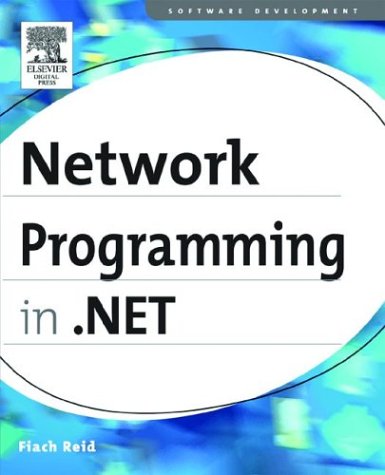
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CombinedTcpChannel.cs
- Pen.cs
- ReferentialConstraint.cs
- BitmapFrameDecode.cs
- DataSourceCollectionBase.cs
- KoreanLunisolarCalendar.cs
- MaterializeFromAtom.cs
- Models.cs
- streamingZipPartStream.cs
- WebPartCloseVerb.cs
- querybuilder.cs
- OptimizedTemplateContentHelper.cs
- RequestResizeEvent.cs
- BuildResultCache.cs
- ZipIOExtraFieldElement.cs
- SvcMapFile.cs
- HotSpot.cs
- ChangePasswordAutoFormat.cs
- EntityContainerAssociationSet.cs
- SettingsContext.cs
- BindingEntityInfo.cs
- EventSinkActivityDesigner.cs
- SessionEndingCancelEventArgs.cs
- ErrorWebPart.cs
- BitSet.cs
- EnvelopedSignatureTransform.cs
- EdmItemError.cs
- DesigntimeLicenseContext.cs
- QuestionEventArgs.cs
- NameTable.cs
- ExpressionConverter.cs
- WindowsToolbarAsMenu.cs
- XmlSecureResolver.cs
- HostedNamedPipeTransportManager.cs
- UnsafeNativeMethods.cs
- MultiByteCodec.cs
- Decimal.cs
- BmpBitmapDecoder.cs
- HttpInputStream.cs
- TlsnegoTokenAuthenticator.cs
- SqlDataSourceDesigner.cs
- TextEffect.cs
- BindingMemberInfo.cs
- XPathNodeHelper.cs
- ProtocolsConfigurationHandler.cs
- BaseTemplateCodeDomTreeGenerator.cs
- OneOfElement.cs
- PackWebResponse.cs
- ErrorHandler.cs
- ParenthesizePropertyNameAttribute.cs
- EmptyControlCollection.cs
- ContentTextAutomationPeer.cs
- StsCommunicationException.cs
- QilXmlReader.cs
- ControlCollection.cs
- SafeCoTaskMem.cs
- TemplateParser.cs
- NodeCounter.cs
- UiaCoreTypesApi.cs
- TdsRecordBufferSetter.cs
- Point4DValueSerializer.cs
- Matrix.cs
- HwndMouseInputProvider.cs
- PenContexts.cs
- InputLanguageSource.cs
- XmlSerializerSection.cs
- HtmlProps.cs
- RotationValidation.cs
- Attribute.cs
- GridViewHeaderRowPresenterAutomationPeer.cs
- DataServiceEntityAttribute.cs
- TrackingProfileManager.cs
- InputDevice.cs
- ApplicationTrust.cs
- BitmapSizeOptions.cs
- Italic.cs
- HtmlSelect.cs
- ReflectionTypeLoadException.cs
- KnownBoxes.cs
- GradientBrush.cs
- LostFocusEventManager.cs
- SmtpMail.cs
- ImportedNamespaceContextItem.cs
- Vector3D.cs
- GuidelineCollection.cs
- ClientBuildManager.cs
- Directory.cs
- GeneralTransform3DGroup.cs
- PeerCollaborationPermission.cs
- OuterGlowBitmapEffect.cs
- SwitchAttribute.cs
- RootAction.cs
- SoapAttributeAttribute.cs
- ServiceOperationDetailViewControl.cs
- AsymmetricAlgorithm.cs
- MsmqElementBase.cs
- XsltCompileContext.cs
- DBConnectionString.cs
- ChtmlTextWriter.cs
- OleDbError.cs