Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / Designer / System / data / design / DataSourceCollectionBase.cs / 1 / DataSourceCollectionBase.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All Rights Reserved. // Information Contained Herein is Proprietary and Confidential. // //----------------------------------------------------------------------------- namespace System.Data.Design{ using System; using System.Collections; using System.ComponentModel; using System.Diagnostics; using System.Globalization; ////// internal abstract class DataSourceCollectionBase : CollectionBase, INamedObjectCollection, IObjectWithParent { private DataSourceComponent collectionHost; internal DataSourceCollectionBase(DataSourceComponent collectionHost) { this.collectionHost = collectionHost; } internal virtual DataSourceComponent CollectionHost { get { return this.collectionHost; } set { this.collectionHost = value; } } protected virtual Type ItemType { get { return typeof(IDataSourceNamedObject); } } protected abstract INameService NameService { get; } [Browsable(false)] object IObjectWithParent.Parent { get { return this.collectionHost; } } protected virtual string CreateUniqueName(IDataSourceNamedObject value) { string suggestedName = StringUtil.NotEmpty(value.Name) ? value.Name : value.PublicTypeName; return NameService.CreateUniqueName(this, suggestedName, 1); } ////// This function will check to see namedObject have a name conflict with the names /// used in the collection and fix the name for namedObject if there is a conflict /// /// internal protected virtual void EnsureUniqueName(IDataSourceNamedObject namedObject) { if (namedObject.Name == null || namedObject.Name.Length == 0 || this.FindObject(namedObject.Name) != null) { namedObject.Name = CreateUniqueName(namedObject); } } internal virtual protected IDataSourceNamedObject FindObject(string name) { IEnumerator e = this.InnerList.GetEnumerator(); while( e.MoveNext() ) { IDataSourceNamedObject existing = (IDataSourceNamedObject) e.Current; if (StringUtil.EqualValue(existing.Name, name)) { return existing; } } return null; } public void InsertBefore(object value, object refObject) { int index = List.IndexOf(refObject); if (index >= 0) { List.Insert(index, value); } else { List.Add(value); } } protected override void OnValidate( object value ) { base.OnValidate( value ); ValidateType( value ); } public void Remove(string name) { INamedObject obj = NamedObjectUtil.Find( this, name ); if( obj != null ) { this.List.Remove( obj ); } } ////// This function only check the name to be valid, typically used by Insert function, which already ensure the name is unique /// /// internal protected virtual void ValidateName(IDataSourceNamedObject obj) { this.NameService.ValidateName(obj.Name); } ////// This function checks the name to be unique and valid, typically used by rename /// /// /// internal protected virtual void ValidateUniqueName(IDataSourceNamedObject obj, string proposedName) { this.NameService.ValidateUniqueName(this, obj, proposedName); } protected void ValidateType( object value ) { if (!ItemType.IsInstanceOfType(value)) { throw new InternalException(VSDExceptions.DataSource.INVALID_COLLECTIONTYPE_MSG, VSDExceptions.DataSource.INVALID_COLLECTIONTYPE_CODE, true); } } public INameService GetNameService() { return NameService; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
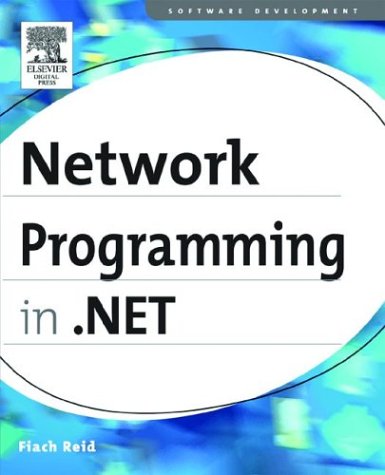
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SqlCacheDependencyDatabaseCollection.cs
- ColumnBinding.cs
- wgx_exports.cs
- UndirectedGraph.cs
- CodeNamespaceCollection.cs
- TimeZone.cs
- ServiceDebugBehavior.cs
- CacheSection.cs
- Peer.cs
- Exceptions.cs
- WebPartEditorApplyVerb.cs
- ListBox.cs
- DBConnectionString.cs
- NewExpression.cs
- HttpRawResponse.cs
- ElementNotEnabledException.cs
- CodeLinePragma.cs
- CommentAction.cs
- MetadataConversionError.cs
- IdentitySection.cs
- VoiceInfo.cs
- AuthorizationRuleCollection.cs
- SqlClientMetaDataCollectionNames.cs
- ExpandCollapsePattern.cs
- XmlCharCheckingWriter.cs
- PixelShader.cs
- ISFTagAndGuidCache.cs
- SystemIcmpV4Statistics.cs
- XmlSchema.cs
- InfoCardAsymmetricCrypto.cs
- ServiceBehaviorElementCollection.cs
- StateWorkerRequest.cs
- WsdlParser.cs
- FontCollection.cs
- ToolStripLabel.cs
- ImageSourceValueSerializer.cs
- ControlCachePolicy.cs
- WebSysDisplayNameAttribute.cs
- BamlLocalizationDictionary.cs
- RayHitTestParameters.cs
- ExtendedProperty.cs
- AnyReturnReader.cs
- X509ChainPolicy.cs
- DifferencingCollection.cs
- DocumentSchemaValidator.cs
- ActivationArguments.cs
- MonitorWrapper.cs
- SmiEventSink_DeferedProcessing.cs
- RtfFormatStack.cs
- PrintPageEvent.cs
- AuthenticateEventArgs.cs
- MarkedHighlightComponent.cs
- WebBrowserPermission.cs
- EdgeProfileValidation.cs
- SoapHeaderException.cs
- WindowsListBox.cs
- StorageEntitySetMapping.cs
- ErrorWebPart.cs
- KeyboardDevice.cs
- InvokeMemberBinder.cs
- DataGridViewCellParsingEventArgs.cs
- DrawListViewColumnHeaderEventArgs.cs
- Transform.cs
- DiagnosticsConfigurationHandler.cs
- HttpRequestWrapper.cs
- DataGridViewColumn.cs
- InputProcessorProfiles.cs
- LinqDataSourceUpdateEventArgs.cs
- EntityDataSourceContainerNameItem.cs
- UnknownWrapper.cs
- StylusPointDescription.cs
- HwndHost.cs
- Identity.cs
- BitmapCacheBrush.cs
- ZipIOCentralDirectoryBlock.cs
- ExplicitDiscriminatorMap.cs
- WindowsTooltip.cs
- Triangle.cs
- DataContract.cs
- KerberosReceiverSecurityToken.cs
- DynamicILGenerator.cs
- HttpCachePolicy.cs
- StickyNoteContentControl.cs
- HttpConfigurationSystem.cs
- XamlFigureLengthSerializer.cs
- HtmlValidationSummaryAdapter.cs
- ExpressionList.cs
- DirectoryObjectSecurity.cs
- _LocalDataStoreMgr.cs
- DataShape.cs
- CodeDelegateCreateExpression.cs
- basecomparevalidator.cs
- PermissionRequestEvidence.cs
- HttpClientProtocol.cs
- MinimizableAttributeTypeConverter.cs
- MetabaseSettingsIis7.cs
- ObjectCloneHelper.cs
- TreeViewEvent.cs
- RightsManagementEncryptedStream.cs
- DefaultSerializationProviderAttribute.cs