Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Framework / MS / Internal / Annotations / Anchoring / SubtreeProcessor.cs / 1 / SubtreeProcessor.cs
//------------------------------------------------------------------------------ // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // SubTreeProcessor is an abstract class defining the API for // processing an element tree (walking the tree and loading // annotations), generating locators, and generating query // fragments for locator parts. // Spec: http://team/sites/ag/Specifications/Anchoring%20Namespace%20Spec.doc // // History: // 1/2003 rruiz: created - based on architectural discussions and design // by axelk, rruiz, magedz // 07/21/2003: rruiz: Ported to WCP tree. // 08/18/2003: rruiz: Updated to Anchoring Namespace Spec. // 05/07/2004: ssimova: Removed XPath expressions // //----------------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.Diagnostics; using System.Windows; using System.Windows.Annotations; using System.Windows.Annotations.Storage; using System.Windows.Media; using MS.Utility; using System.Xml; namespace MS.Internal.Annotations.Anchoring { ////// SubTreeProcessor is an abstract class defining the API for /// processing an element tree (walking the tree and loading /// annotations), generating locators, and generating query /// fragments for locator parts. /// internal abstract class SubTreeProcessor { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors ////// Creates an instance of SubTreeProcessor. Subclasses should /// pass the manager that created and owns them. /// /// the manager that owns this processor ///manager is null protected SubTreeProcessor(LocatorManager manager) { if (manager == null) throw new ArgumentNullException("manager"); _manager = manager; } #endregion Constructors //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- #region Public Methods ////// Gives the processor a chance to process annotations for this node before its /// children are processed. If calledProcessAnnotations is set to true then the /// children will not be individually processed. /// /// node to process /// indicates the callback was called by /// this processor ////// a list of AttachedAnnotations loaded during the processing of /// this node; can be null if no annotations were loaded /// ///node is null public abstract IListPreProcessNode(DependencyObject node, out bool calledProcessAnnotations); /// /// This method is always called after PreProcessSubTree and after the node's children have /// been processed (unless that step was skipped because PreProcessSubtree returned true for /// calledProcessAnnotations). /// /// the node that is being processed /// the combined value (||'d) of calledProcessAnnotations /// on this node's subtree; in the case when the children were not individually processed, false is /// passed in /// indicates the callback was called by this processor ///list of AttachedAnnotations loaded during the post processing of this node; can /// be null if no annotations were loaded ///node is null public virtual IListPostProcessNode(DependencyObject node, bool childrenCalledProcessAnnotations, out bool calledProcessAnnotations) { if (node == null) throw new ArgumentNullException("node"); calledProcessAnnotations = false; // do nothing here return null; } /// /// Generates a locator part list identifying node. /// ////// Most subclasses will simply return a ContentLocator with one locator /// part in it. In some cases, more than one locator part may be /// required (e.g., a node which represents path to some data may /// need a separate locator part for each portion of the path). /// /// the node to generate a locator for /// specifies whether or not generating should /// continue for the rest of the path; a SubTreeProcessor could return false if /// it processed the rest of the path itself ////// a locator identifying 'node'; in most cases this locator will /// only contain one locator part; can return null if no /// locator part can be generated for the given node /// ///node is null public abstract ContentLocator GenerateLocator(PathNode node, out bool continueGenerating); ////// Searches the logical tree for a node matching the values of /// locatorPart. The search begins with startNode. /// ////// Subclasses can choose to only examine startNode or traverse /// the logical tree looking for a match. The algorithms for some /// locator part types may require a search of the tree instead of /// a simple examination of one node. /// /// locator part to be matched, must be of the type /// handled by this processor /// logical tree node to start search at /// return flag indicating whether the search /// should continue (presumably because the search was not exhaustive) ///returns a node that matches the locator part; null if no such /// node is found ///locatorPart or startNode are /// null ///locatorPart is of the incorrect /// type public abstract DependencyObject ResolveLocatorPart(ContentLocatorPart locatorPart, DependencyObject startNode, out bool continueResolving); ////// Returns a list of XmlQualifiedNames representing the /// the locator parts this processor can resolve/generate. /// public abstract XmlQualifiedName[] GetLocatorPartTypes(); #endregion Public Methods //------------------------------------------------------ // // Public Operators // //------------------------------------------------------ //----------------------------------------------------- // // Public Events // //------------------------------------------------------ //----------------------------------------------------- // // Public Properties // //----------------------------------------------------- //----------------------------------------------------- // // Protected Methods // //------------------------------------------------------ //----------------------------------------------------- // // Protected Properties // //------------------------------------------------------ #region Protected Properties ////// The manager that created and owns this processor. /// protected LocatorManager Manager { get { return _manager; } } #endregion Protected Properties //------------------------------------------------------ // // Private Fields // //----------------------------------------------------- #region Private Fields // LocatorProcessingManager that created this subtree processor private LocatorManager _manager; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // SubTreeProcessor is an abstract class defining the API for // processing an element tree (walking the tree and loading // annotations), generating locators, and generating query // fragments for locator parts. // Spec: http://team/sites/ag/Specifications/Anchoring%20Namespace%20Spec.doc // // History: // 1/2003 rruiz: created - based on architectural discussions and design // by axelk, rruiz, magedz // 07/21/2003: rruiz: Ported to WCP tree. // 08/18/2003: rruiz: Updated to Anchoring Namespace Spec. // 05/07/2004: ssimova: Removed XPath expressions // //----------------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.Diagnostics; using System.Windows; using System.Windows.Annotations; using System.Windows.Annotations.Storage; using System.Windows.Media; using MS.Utility; using System.Xml; namespace MS.Internal.Annotations.Anchoring { ////// SubTreeProcessor is an abstract class defining the API for /// processing an element tree (walking the tree and loading /// annotations), generating locators, and generating query /// fragments for locator parts. /// internal abstract class SubTreeProcessor { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors ////// Creates an instance of SubTreeProcessor. Subclasses should /// pass the manager that created and owns them. /// /// the manager that owns this processor ///manager is null protected SubTreeProcessor(LocatorManager manager) { if (manager == null) throw new ArgumentNullException("manager"); _manager = manager; } #endregion Constructors //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- #region Public Methods ////// Gives the processor a chance to process annotations for this node before its /// children are processed. If calledProcessAnnotations is set to true then the /// children will not be individually processed. /// /// node to process /// indicates the callback was called by /// this processor ////// a list of AttachedAnnotations loaded during the processing of /// this node; can be null if no annotations were loaded /// ///node is null public abstract IListPreProcessNode(DependencyObject node, out bool calledProcessAnnotations); /// /// This method is always called after PreProcessSubTree and after the node's children have /// been processed (unless that step was skipped because PreProcessSubtree returned true for /// calledProcessAnnotations). /// /// the node that is being processed /// the combined value (||'d) of calledProcessAnnotations /// on this node's subtree; in the case when the children were not individually processed, false is /// passed in /// indicates the callback was called by this processor ///list of AttachedAnnotations loaded during the post processing of this node; can /// be null if no annotations were loaded ///node is null public virtual IListPostProcessNode(DependencyObject node, bool childrenCalledProcessAnnotations, out bool calledProcessAnnotations) { if (node == null) throw new ArgumentNullException("node"); calledProcessAnnotations = false; // do nothing here return null; } /// /// Generates a locator part list identifying node. /// ////// Most subclasses will simply return a ContentLocator with one locator /// part in it. In some cases, more than one locator part may be /// required (e.g., a node which represents path to some data may /// need a separate locator part for each portion of the path). /// /// the node to generate a locator for /// specifies whether or not generating should /// continue for the rest of the path; a SubTreeProcessor could return false if /// it processed the rest of the path itself ////// a locator identifying 'node'; in most cases this locator will /// only contain one locator part; can return null if no /// locator part can be generated for the given node /// ///node is null public abstract ContentLocator GenerateLocator(PathNode node, out bool continueGenerating); ////// Searches the logical tree for a node matching the values of /// locatorPart. The search begins with startNode. /// ////// Subclasses can choose to only examine startNode or traverse /// the logical tree looking for a match. The algorithms for some /// locator part types may require a search of the tree instead of /// a simple examination of one node. /// /// locator part to be matched, must be of the type /// handled by this processor /// logical tree node to start search at /// return flag indicating whether the search /// should continue (presumably because the search was not exhaustive) ///returns a node that matches the locator part; null if no such /// node is found ///locatorPart or startNode are /// null ///locatorPart is of the incorrect /// type public abstract DependencyObject ResolveLocatorPart(ContentLocatorPart locatorPart, DependencyObject startNode, out bool continueResolving); ////// Returns a list of XmlQualifiedNames representing the /// the locator parts this processor can resolve/generate. /// public abstract XmlQualifiedName[] GetLocatorPartTypes(); #endregion Public Methods //------------------------------------------------------ // // Public Operators // //------------------------------------------------------ //----------------------------------------------------- // // Public Events // //------------------------------------------------------ //----------------------------------------------------- // // Public Properties // //----------------------------------------------------- //----------------------------------------------------- // // Protected Methods // //------------------------------------------------------ //----------------------------------------------------- // // Protected Properties // //------------------------------------------------------ #region Protected Properties ////// The manager that created and owns this processor. /// protected LocatorManager Manager { get { return _manager; } } #endregion Protected Properties //------------------------------------------------------ // // Private Fields // //----------------------------------------------------- #region Private Fields // LocatorProcessingManager that created this subtree processor private LocatorManager _manager; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
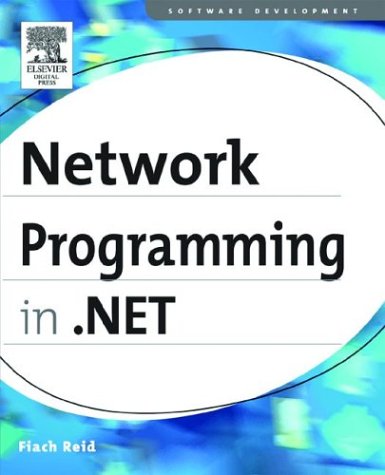
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XAMLParseException.cs
- RuleSettings.cs
- PreviewPageInfo.cs
- MailHeaderInfo.cs
- ApplicationInfo.cs
- Input.cs
- DrawListViewColumnHeaderEventArgs.cs
- ISFClipboardData.cs
- MbpInfo.cs
- CodeTypeDeclaration.cs
- ConfigurationValues.cs
- FileSecurity.cs
- ScriptingScriptResourceHandlerSection.cs
- ObjectConverter.cs
- LocalizableResourceBuilder.cs
- StreamSecurityUpgradeProvider.cs
- DesignerGeometryHelper.cs
- HttpRuntimeSection.cs
- ConfigXmlWhitespace.cs
- WorkflowViewElement.cs
- SQLInt64.cs
- HttpDictionary.cs
- ControlTemplate.cs
- FormViewUpdateEventArgs.cs
- SerializerWriterEventHandlers.cs
- WebPartUtil.cs
- BinaryReader.cs
- ComNativeDescriptor.cs
- __Filters.cs
- Stack.cs
- GridViewAutomationPeer.cs
- externdll.cs
- SiteMapNodeItemEventArgs.cs
- ToolStripDropDownClosedEventArgs.cs
- NonPrimarySelectionGlyph.cs
- KerberosRequestorSecurityToken.cs
- ConnectionsZone.cs
- RoutedUICommand.cs
- ConfigurationSection.cs
- AnalyzedTree.cs
- GridViewDeletedEventArgs.cs
- DesignBindingPropertyDescriptor.cs
- HtmlShim.cs
- UniformGrid.cs
- PointHitTestResult.cs
- WmpBitmapEncoder.cs
- StatusStrip.cs
- XmlUnspecifiedAttribute.cs
- AjaxFrameworkAssemblyAttribute.cs
- WSHttpBindingElement.cs
- ProviderConnectionPointCollection.cs
- ContextMenuAutomationPeer.cs
- Compiler.cs
- ConfigurationSchemaErrors.cs
- ProgressiveCrcCalculatingStream.cs
- AppearanceEditorPart.cs
- GZipDecoder.cs
- SafeTimerHandle.cs
- AssemblyUtil.cs
- BitmapEffectDrawingContent.cs
- AdapterDictionary.cs
- smtppermission.cs
- InvalidContentTypeException.cs
- IPPacketInformation.cs
- Int16Storage.cs
- TimeSpanOrInfiniteConverter.cs
- Thread.cs
- ElementsClipboardData.cs
- DataListComponentEditor.cs
- PartitionedDataSource.cs
- MarshalDirectiveException.cs
- InvokeMethodActivityDesigner.cs
- CalendarBlackoutDatesCollection.cs
- MediaContextNotificationWindow.cs
- TypeInitializationException.cs
- BackgroundFormatInfo.cs
- Screen.cs
- OperatorExpressions.cs
- TreeNodeClickEventArgs.cs
- ResourceReferenceKeyNotFoundException.cs
- TraceHandlerErrorFormatter.cs
- LinearGradientBrush.cs
- PackUriHelper.cs
- RemoteHelper.cs
- ApplicationTrust.cs
- JsonFormatReaderGenerator.cs
- Rectangle.cs
- EventLogger.cs
- PagesSection.cs
- ButtonRenderer.cs
- _AutoWebProxyScriptHelper.cs
- TextEditorTyping.cs
- ConfigsHelper.cs
- URLEditor.cs
- RegexTree.cs
- ApplicationGesture.cs
- PeerContact.cs
- ExclusiveTcpTransportManager.cs
- TreeView.cs
- SimpleBitVector32.cs