Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / xsp / System / Web / HttpPostedFile.cs / 1 / HttpPostedFile.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* * HttpCookie - collection + name + path * * Copyright (c) 1998 Microsoft Corporation */ namespace System.Web { using System.IO; using System.Security.Permissions; using System.Configuration; using System.Web.Configuration; using System.Web.Management; ////// [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public sealed class HttpPostedFile { private String _filename; private String _contentType; private HttpInputStream _stream; internal HttpPostedFile(String filename, String contentType, HttpInputStream stream) { _filename = filename; _contentType = contentType; _stream = stream; } /* * File name */ ////// Provides a way to /// access files uploaded by a client. /// ////// public String FileName { get { return _filename;} } /* * Content type */ ////// Returns the full path of a file on the local browser's machine (for /// example, "c:\temp\test.txt"). /// ////// public String ContentType { get { return _contentType;} } /* * Content length */ ////// Returns the MIME content type of an incoming file sent by a client. /// ////// public int ContentLength { get { return (int)_stream.Length;} } /* * Stream */ ////// Returns the size of an uploaded file, in bytes. /// ////// public Stream InputStream { get { return _stream;} } /* * Save into file */ ////// Provides raw access to /// contents of an uploaded file. /// ////// public void SaveAs(String filename) { // VSWhidbey 82855 if (!Path.IsPathRooted(filename)) { HttpRuntimeSection config = RuntimeConfig.GetConfig().HttpRuntime; if (config.RequireRootedSaveAsPath) { throw new HttpException(SR.GetString(SR.SaveAs_requires_rooted_path, filename)); } } FileStream f = new FileStream(filename, FileMode.Create); try { _stream.WriteTo(f); f.Flush(); } finally { f.Close(); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ ///// Initiates a utility method to save an uploaded file to disk. /// ///// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* * HttpCookie - collection + name + path * * Copyright (c) 1998 Microsoft Corporation */ namespace System.Web { using System.IO; using System.Security.Permissions; using System.Configuration; using System.Web.Configuration; using System.Web.Management; ////// [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public sealed class HttpPostedFile { private String _filename; private String _contentType; private HttpInputStream _stream; internal HttpPostedFile(String filename, String contentType, HttpInputStream stream) { _filename = filename; _contentType = contentType; _stream = stream; } /* * File name */ ////// Provides a way to /// access files uploaded by a client. /// ////// public String FileName { get { return _filename;} } /* * Content type */ ////// Returns the full path of a file on the local browser's machine (for /// example, "c:\temp\test.txt"). /// ////// public String ContentType { get { return _contentType;} } /* * Content length */ ////// Returns the MIME content type of an incoming file sent by a client. /// ////// public int ContentLength { get { return (int)_stream.Length;} } /* * Stream */ ////// Returns the size of an uploaded file, in bytes. /// ////// public Stream InputStream { get { return _stream;} } /* * Save into file */ ////// Provides raw access to /// contents of an uploaded file. /// ////// public void SaveAs(String filename) { // VSWhidbey 82855 if (!Path.IsPathRooted(filename)) { HttpRuntimeSection config = RuntimeConfig.GetConfig().HttpRuntime; if (config.RequireRootedSaveAsPath) { throw new HttpException(SR.GetString(SR.SaveAs_requires_rooted_path, filename)); } } FileStream f = new FileStream(filename, FileMode.Create); try { _stream.WriteTo(f); f.Flush(); } finally { f.Close(); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007./// Initiates a utility method to save an uploaded file to disk. /// ///
Link Menu
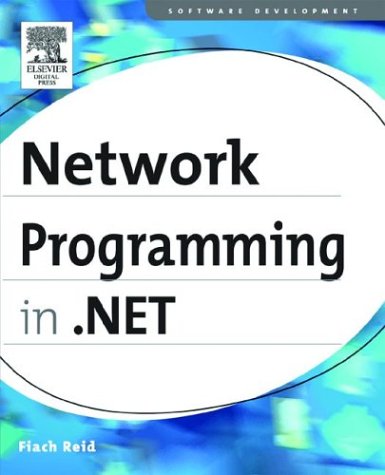
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Compiler.cs
- ObjectListCommand.cs
- X509IssuerSerialKeyIdentifierClause.cs
- XmlSchemaSequence.cs
- EncodingStreamWrapper.cs
- ListBoxAutomationPeer.cs
- DesignerProperties.cs
- ResizeGrip.cs
- XmlSchemaSearchPattern.cs
- ComponentResourceKey.cs
- TextBoxLine.cs
- FileChangesMonitor.cs
- TableCell.cs
- ObjectConverter.cs
- VariableReference.cs
- DrawingContext.cs
- FixedSOMElement.cs
- ILGenerator.cs
- InfiniteTimeSpanConverter.cs
- Point.cs
- _IPv6Address.cs
- ConstraintConverter.cs
- DbCommandDefinition.cs
- DtdParser.cs
- ValidationResult.cs
- DataSvcMapFile.cs
- MultilineStringConverter.cs
- SvcMapFileLoader.cs
- ConfigurationStrings.cs
- SID.cs
- DtdParser.cs
- SetIterators.cs
- WebRequestModuleElement.cs
- WebPartConnectionsConfigureVerb.cs
- DefaultPerformanceCounters.cs
- XmlLanguage.cs
- SqlProviderManifest.cs
- SafeMarshalContext.cs
- XmlReflectionImporter.cs
- GeneralTransform3D.cs
- QueryContinueDragEvent.cs
- GenericPrincipal.cs
- CalendarAutomationPeer.cs
- HierarchicalDataSourceIDConverter.cs
- HtmlControl.cs
- ClientBuildManager.cs
- PublisherMembershipCondition.cs
- RevocationPoint.cs
- ModelFunctionTypeElement.cs
- DataTableNewRowEvent.cs
- COM2PropertyDescriptor.cs
- XmlSchemaAny.cs
- ReadOnlyTernaryTree.cs
- HtmlImage.cs
- KerberosSecurityTokenProvider.cs
- ErrorInfoXmlDocument.cs
- MouseCaptureWithinProperty.cs
- Knowncolors.cs
- ContentDefinition.cs
- EventTrigger.cs
- TemplateEditingService.cs
- SupportsEventValidationAttribute.cs
- StylusButtonEventArgs.cs
- SchemaNotation.cs
- EdmSchemaAttribute.cs
- TTSEngineProxy.cs
- StreamResourceInfo.cs
- DetailsViewRow.cs
- UIPropertyMetadata.cs
- TextRangeBase.cs
- GridViewUpdatedEventArgs.cs
- PageThemeCodeDomTreeGenerator.cs
- InitiatorSessionSymmetricTransportSecurityProtocol.cs
- NewArrayExpression.cs
- ProcessModelInfo.cs
- FocusManager.cs
- IdentityModelDictionary.cs
- BinaryWriter.cs
- ExecutionContext.cs
- XmlNodeChangedEventManager.cs
- QueryStringParameter.cs
- TemplatedAdorner.cs
- PolicyException.cs
- StatusStrip.cs
- TextBoxRenderer.cs
- COM2ExtendedBrowsingHandler.cs
- InfoCardClaim.cs
- MultiPropertyDescriptorGridEntry.cs
- ButtonFieldBase.cs
- CodeDOMUtility.cs
- WorkflowPageSetupDialog.cs
- VisualStyleInformation.cs
- NumberFunctions.cs
- ManifestResourceInfo.cs
- StackOverflowException.cs
- HttpHeaderCollection.cs
- ArgumentException.cs
- EntityViewGenerator.cs
- basemetadatamappingvisitor.cs
- SharedUtils.cs