Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / ndp / fx / src / DataEntity / System / Data / EntityClient / EntityParameterCollection.cs / 2 / EntityParameterCollection.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....], [....] //--------------------------------------------------------------------- using System.Collections; using System.Collections.Generic; using System.Text; using System.Data; using System.Data.Common; using System.Data.Metadata.Edm; namespace System.Data.EntityClient { ////// Class representing a parameter collection used in EntityCommand /// public sealed partial class EntityParameterCollection : DbParameterCollection { private static Type ItemType = typeof(EntityParameter); private bool _isDirty; ////// Constructs the EntityParameterCollection object /// internal EntityParameterCollection() : base() { } ////// Gets the parameter from the collection at the specified index /// /// The index of the parameter to retrieved ///The parameter at the index public new EntityParameter this[int index] { get { return (EntityParameter)this.GetParameter(index); } set { this.SetParameter(index, value); } } ////// Gets the parameter with the given name from the collection /// /// The name of the parameter to retrieved ///The parameter with the given name public new EntityParameter this[string parameterName] { get { return (EntityParameter)this.GetParameter(parameterName); } set { this.SetParameter(parameterName, value); } } ////// Gets whether this collection has been changes since the last reset /// internal bool IsDirty { get { if (_isDirty) { return true; } // Loop through and return true if any parameter is dirty foreach (EntityParameter parameter in this) { if (parameter.IsDirty) { return true; } } return false; } } ////// Add a EntityParameter to the collection /// /// The parameter to add to the collection ///The index of the new parameter within the collection public EntityParameter Add(EntityParameter value) { this.Add((object)value); return value; } ////// Add a EntityParameter with the given name and value to the collection /// /// The name of the parameter to add /// The value of the parameter to add ///The index of the new parameter within the collection public EntityParameter AddWithValue(string parameterName, object value) { EntityParameter param = new EntityParameter(); param.ParameterName = parameterName; param.Value = value; return this.Add(param); } ////// Adds a EntityParameter with the given name and type to the collection /// /// The name of the parameter to add /// The type of the parameter ///The index of the new parameter within the collection public EntityParameter Add(string parameterName, DbType dbType) { return this.Add(new EntityParameter(parameterName, dbType)); } ////// Add a EntityParameter with the given name, type, and size to the collection /// /// The name of the parameter to add /// The type of the parameter /// The size of the parameter ///The index of the new parameter within the collection public EntityParameter Add(string parameterName, DbType dbType, int size) { return this.Add(new EntityParameter(parameterName, dbType, size)); } ////// Adds a range of EntityParameter objects to this collection /// /// The arary of EntityParameter objects to add public void AddRange(EntityParameter[] values) { this.AddRange((Array)values); } ////// Check if the collection has a parameter with the given parameter name /// /// The parameter name to look for ///True if the collection has a parameter with the given name public override bool Contains(string parameterName) { return this.IndexOf(parameterName) != -1; } ////// Copies the given array of parameters into this collection /// /// The array to copy into /// The index in the array where the copy starts public void CopyTo(EntityParameter[] array, int index) { this.CopyTo((Array)array, index); } ////// Finds the index in the collection of the given parameter object /// /// The parameter to search for ///The index of the parameter, -1 if not found public int IndexOf(EntityParameter value) { return IndexOf((object)value); } ////// Add a EntityParameter with the given value to the collection at a location indicated by the index /// /// The index at which the parameter is to be inserted /// The value of the parameter public void Insert(int index, EntityParameter value) { this.Insert(index, (object)value); } ////// Marks that this collection has been changed /// private void OnChange() { _isDirty = true; } ////// Remove a EntityParameter with the given value from the collection /// /// The parameter to remove public void Remove(EntityParameter value) { this.Remove((object)value); } ////// Reset the dirty flag on the collection /// internal void ResetIsDirty() { _isDirty = false; // Loop through and reset each parameter foreach (EntityParameter parameter in this) { parameter.ResetIsDirty(); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....], [....] //--------------------------------------------------------------------- using System.Collections; using System.Collections.Generic; using System.Text; using System.Data; using System.Data.Common; using System.Data.Metadata.Edm; namespace System.Data.EntityClient { ////// Class representing a parameter collection used in EntityCommand /// public sealed partial class EntityParameterCollection : DbParameterCollection { private static Type ItemType = typeof(EntityParameter); private bool _isDirty; ////// Constructs the EntityParameterCollection object /// internal EntityParameterCollection() : base() { } ////// Gets the parameter from the collection at the specified index /// /// The index of the parameter to retrieved ///The parameter at the index public new EntityParameter this[int index] { get { return (EntityParameter)this.GetParameter(index); } set { this.SetParameter(index, value); } } ////// Gets the parameter with the given name from the collection /// /// The name of the parameter to retrieved ///The parameter with the given name public new EntityParameter this[string parameterName] { get { return (EntityParameter)this.GetParameter(parameterName); } set { this.SetParameter(parameterName, value); } } ////// Gets whether this collection has been changes since the last reset /// internal bool IsDirty { get { if (_isDirty) { return true; } // Loop through and return true if any parameter is dirty foreach (EntityParameter parameter in this) { if (parameter.IsDirty) { return true; } } return false; } } ////// Add a EntityParameter to the collection /// /// The parameter to add to the collection ///The index of the new parameter within the collection public EntityParameter Add(EntityParameter value) { this.Add((object)value); return value; } ////// Add a EntityParameter with the given name and value to the collection /// /// The name of the parameter to add /// The value of the parameter to add ///The index of the new parameter within the collection public EntityParameter AddWithValue(string parameterName, object value) { EntityParameter param = new EntityParameter(); param.ParameterName = parameterName; param.Value = value; return this.Add(param); } ////// Adds a EntityParameter with the given name and type to the collection /// /// The name of the parameter to add /// The type of the parameter ///The index of the new parameter within the collection public EntityParameter Add(string parameterName, DbType dbType) { return this.Add(new EntityParameter(parameterName, dbType)); } ////// Add a EntityParameter with the given name, type, and size to the collection /// /// The name of the parameter to add /// The type of the parameter /// The size of the parameter ///The index of the new parameter within the collection public EntityParameter Add(string parameterName, DbType dbType, int size) { return this.Add(new EntityParameter(parameterName, dbType, size)); } ////// Adds a range of EntityParameter objects to this collection /// /// The arary of EntityParameter objects to add public void AddRange(EntityParameter[] values) { this.AddRange((Array)values); } ////// Check if the collection has a parameter with the given parameter name /// /// The parameter name to look for ///True if the collection has a parameter with the given name public override bool Contains(string parameterName) { return this.IndexOf(parameterName) != -1; } ////// Copies the given array of parameters into this collection /// /// The array to copy into /// The index in the array where the copy starts public void CopyTo(EntityParameter[] array, int index) { this.CopyTo((Array)array, index); } ////// Finds the index in the collection of the given parameter object /// /// The parameter to search for ///The index of the parameter, -1 if not found public int IndexOf(EntityParameter value) { return IndexOf((object)value); } ////// Add a EntityParameter with the given value to the collection at a location indicated by the index /// /// The index at which the parameter is to be inserted /// The value of the parameter public void Insert(int index, EntityParameter value) { this.Insert(index, (object)value); } ////// Marks that this collection has been changed /// private void OnChange() { _isDirty = true; } ////// Remove a EntityParameter with the given value from the collection /// /// The parameter to remove public void Remove(EntityParameter value) { this.Remove((object)value); } ////// Reset the dirty flag on the collection /// internal void ResetIsDirty() { _isDirty = false; // Loop through and reset each parameter foreach (EntityParameter parameter in this) { parameter.ResetIsDirty(); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
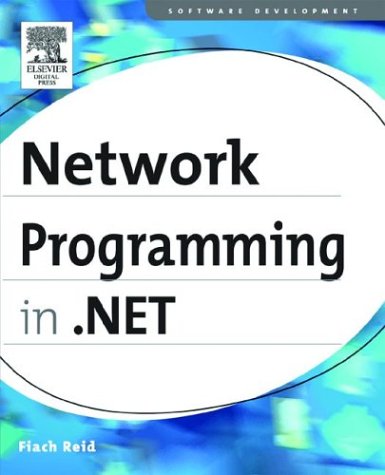
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- rsa.cs
- DataControlCommands.cs
- AppearanceEditorPart.cs
- Parsers.cs
- WindowsNonControl.cs
- Attributes.cs
- SQLByteStorage.cs
- DetailsViewRow.cs
- HandoffBehavior.cs
- DistributedTransactionPermission.cs
- DisplayNameAttribute.cs
- CornerRadius.cs
- WebExceptionStatus.cs
- SocketInformation.cs
- DependencyPropertyKey.cs
- FileDialog_Vista_Interop.cs
- ArrayWithOffset.cs
- Utilities.cs
- FixedSOMGroup.cs
- RuleElement.cs
- WindowsRichEdit.cs
- GestureRecognizer.cs
- SessionStateUtil.cs
- CategoryGridEntry.cs
- WindowsTooltip.cs
- BamlCollectionHolder.cs
- ReaderContextStackData.cs
- ValueUnavailableException.cs
- DbBuffer.cs
- RegexCompilationInfo.cs
- ProfileInfo.cs
- BufferAllocator.cs
- RSAPKCS1KeyExchangeDeformatter.cs
- ArrayWithOffset.cs
- EntityReference.cs
- COM2AboutBoxPropertyDescriptor.cs
- WebPartCollection.cs
- _FixedSizeReader.cs
- ReaderWriterLockSlim.cs
- DataListItem.cs
- DependencyPropertyAttribute.cs
- DeferredSelectedIndexReference.cs
- XpsS0ValidatingLoader.cs
- FrugalList.cs
- WebServiceReceiveDesigner.cs
- SettingsAttributeDictionary.cs
- CapabilitiesAssignment.cs
- RSAPKCS1KeyExchangeFormatter.cs
- WorkflowApplicationAbortedEventArgs.cs
- ObjectToken.cs
- DbTypeMap.cs
- ColumnWidthChangedEvent.cs
- ConfigXmlAttribute.cs
- BaseParser.cs
- DataGridViewCellConverter.cs
- Package.cs
- InternalRelationshipCollection.cs
- x509utils.cs
- ItemsControlAutomationPeer.cs
- AutomationAttributeInfo.cs
- SimpleHandlerFactory.cs
- DiagnosticTraceRecords.cs
- TextBoxDesigner.cs
- XsltConvert.cs
- TabPanel.cs
- ExpressionVisitor.cs
- PointLightBase.cs
- Activation.cs
- MemberNameValidator.cs
- InfoCardRSACryptoProvider.cs
- SqlMethodCallConverter.cs
- PropertyEmitter.cs
- EventLogInformation.cs
- TitleStyle.cs
- CompilationSection.cs
- MachineKeyConverter.cs
- WebRequest.cs
- newinstructionaction.cs
- TraceContext.cs
- AliasGenerator.cs
- ComponentCodeDomSerializer.cs
- NamespaceCollection.cs
- PackageRelationshipCollection.cs
- CmsInterop.cs
- DuplexClientBase.cs
- Model3D.cs
- OpenTypeCommon.cs
- StrokeNodeEnumerator.cs
- IriParsingElement.cs
- TextCollapsingProperties.cs
- ApplicationActivator.cs
- MultiSelectRootGridEntry.cs
- GlyphCollection.cs
- XmlLoader.cs
- DocumentGrid.cs
- XPathExpr.cs
- METAHEADER.cs
- Axis.cs
- DBDataPermission.cs
- ContainerControl.cs