Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / fx / src / CompMod / System / Diagnostics / Debug.cs / 1 / Debug.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ #define DEBUG namespace System.Diagnostics { using System; using System.Collections; using System.Security.Permissions; using System.Globalization; ////// public sealed class Debug { // not creatable... // private Debug() { } ///Provides a set of properties and /// methods /// for debugging code. ////// public static TraceListenerCollection Listeners { [SecurityPermission(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.UnmanagedCode)] [HostProtection(SharedState=true)] get { return TraceInternal.Listeners; } } ///Gets /// the collection of listeners that is monitoring the debug /// output. ////// public static bool AutoFlush { [SecurityPermission(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.UnmanagedCode)] get { return TraceInternal.AutoFlush; } [SecurityPermission(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.UnmanagedCode)] set { TraceInternal.AutoFlush = value; } } ///Gets or sets a value indicating whether ///should be called on the /// /// after every write. /// public static int IndentLevel { get { return TraceInternal.IndentLevel; } set { TraceInternal.IndentLevel = value; } } ///Gets or sets /// the indent level. ////// public static int IndentSize { get { return TraceInternal.IndentSize; } set { TraceInternal.IndentSize = value; } } ///Gets or sets the number of spaces in an indent. ////// [System.Diagnostics.Conditional("DEBUG")] public static void Flush() { TraceInternal.Flush(); } ///Clears the output buffer, and causes buffered data to /// be written to the ///. /// [System.Diagnostics.Conditional("DEBUG")] [SecurityPermission(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.UnmanagedCode)] public static void Close() { TraceInternal.Close(); } ///Clears the output buffer, and then closes the ///so that they no longer receive /// debugging output. /// [System.Diagnostics.Conditional("DEBUG")] public static void Assert(bool condition) { TraceInternal.Assert(condition); } ///Checks for a condition, and outputs the callstack if the condition is ///. /// [System.Diagnostics.Conditional("DEBUG")] public static void Assert(bool condition, string message) { TraceInternal.Assert(condition, message); } ///Checks for a condition, and displays a message if the condition is /// ///. /// [System.Diagnostics.Conditional("DEBUG")] public static void Assert(bool condition, string message, string detailMessage) { TraceInternal.Assert(condition, message, detailMessage); } ///Checks for a condition, and displays both the specified messages if the condition /// is ///. /// [System.Diagnostics.Conditional("DEBUG")] public static void Fail(string message) { TraceInternal.Fail(message); } ///Emits or displays a message for an assertion that always fails. ////// [System.Diagnostics.Conditional("DEBUG")] public static void Fail(string message, string detailMessage) { TraceInternal.Fail(message, detailMessage); } [System.Diagnostics.Conditional("DEBUG")] public static void Print(string message) { TraceInternal.WriteLine(message); } [System.Diagnostics.Conditional("DEBUG")] public static void Print(string format, params object[] args) { TraceInternal.WriteLine(String.Format(CultureInfo.InvariantCulture, format, args)); } ///Emits or displays both messages for an assertion that always fails. ////// [System.Diagnostics.Conditional("DEBUG")] public static void Write(string message) { TraceInternal.Write(message); } ///Writes a message to the trace listeners in the ///collection. /// [System.Diagnostics.Conditional("DEBUG")] public static void Write(object value) { TraceInternal.Write(value); } ///Writes the name of the value /// parameter to the trace listeners in the ///collection. /// [System.Diagnostics.Conditional("DEBUG")] public static void Write(string message, string category) { TraceInternal.Write(message, category); } ///Writes a category name and message /// to the trace listeners in the ///collection. /// [System.Diagnostics.Conditional("DEBUG")] public static void Write(object value, string category) { TraceInternal.Write(value, category); } ///Writes a category name and the name of the value parameter to the trace /// listeners in the ///collection. /// [System.Diagnostics.Conditional("DEBUG")] public static void WriteLine(string message) { TraceInternal.WriteLine(message); } ///Writes a message followed by a line terminator to the trace listeners in the /// ///collection. The default line terminator /// is a carriage return followed by a line feed (\r\n). /// [System.Diagnostics.Conditional("DEBUG")] public static void WriteLine(object value) { TraceInternal.WriteLine(value); } ///Writes the name of the value /// parameter followed by a line terminator to the /// trace listeners in the ///collection. The default line /// terminator is a carriage return followed by a line feed (\r\n). /// [System.Diagnostics.Conditional("DEBUG")] public static void WriteLine(string message, string category) { TraceInternal.WriteLine(message, category); } ///Writes a category name and message followed by a line terminator to the trace /// listeners in the ///collection. The default line /// terminator is a carriage return followed by a line feed (\r\n). /// [System.Diagnostics.Conditional("DEBUG")] public static void WriteLine(object value, string category) { TraceInternal.WriteLine(value, category); } ///Writes a category name and the name of the value /// parameter followed by a line /// terminator to the trace listeners in the ///collection. The /// default line terminator is a carriage return followed by a line feed (\r\n). /// [System.Diagnostics.Conditional("DEBUG")] public static void WriteIf(bool condition, string message) { TraceInternal.WriteIf(condition, message); } ///Writes a message to the trace listeners in the ///collection /// if a condition is /// . /// [System.Diagnostics.Conditional("DEBUG")] public static void WriteIf(bool condition, object value) { TraceInternal.WriteIf(condition, value); } ///Writes the name of the value /// parameter to the trace listeners in the ////// collection if a condition is /// . /// [System.Diagnostics.Conditional("DEBUG")] public static void WriteIf(bool condition, string message, string category) { TraceInternal.WriteIf(condition, message, category); } ///Writes a category name and message /// to the trace listeners in the ////// collection if a condition is /// . /// [System.Diagnostics.Conditional("DEBUG")] public static void WriteIf(bool condition, object value, string category) { TraceInternal.WriteIf(condition, value, category); } ///Writes a category name and the name of the value /// parameter to the trace /// listeners in the ///collection if a condition is /// . /// [System.Diagnostics.Conditional("DEBUG")] public static void WriteLineIf(bool condition, string message) { TraceInternal.WriteLineIf(condition, message); } ///Writes a message followed by a line terminator to the trace listeners in the /// ///collection if a condition is /// . The default line terminator is a carriage return followed /// by a line feed (\r\n). /// [System.Diagnostics.Conditional("DEBUG")] public static void WriteLineIf(bool condition, object value) { TraceInternal.WriteLineIf(condition, value); } ///Writes the name of the value /// parameter followed by a line terminator to the /// trace listeners in the ///collection if a condition is /// . The default line terminator is a carriage return followed /// by a line feed (\r\n). /// [System.Diagnostics.Conditional("DEBUG")] public static void WriteLineIf(bool condition, string message, string category) { TraceInternal.WriteLineIf(condition, message, category); } ///Writes a category name and message /// followed by a line terminator to the trace /// listeners in the ///collection if a condition is /// . The default line terminator is a carriage return followed /// by a line feed (\r\n). /// [System.Diagnostics.Conditional("DEBUG")] public static void WriteLineIf(bool condition, object value, string category) { TraceInternal.WriteLineIf(condition, value, category); } ///Writes a category name and the name of the value parameter followed by a line /// terminator to the trace listeners in the ///collection /// if a condition is . The default line terminator is a carriage /// return followed by a line feed (\r\n). /// [System.Diagnostics.Conditional("DEBUG")] public static void Indent() { TraceInternal.Indent(); } ///[To be supplied.] ////// [System.Diagnostics.Conditional("DEBUG")] public static void Unindent() { TraceInternal.Unindent(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //[To be supplied.] ///// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ #define DEBUG namespace System.Diagnostics { using System; using System.Collections; using System.Security.Permissions; using System.Globalization; ////// public sealed class Debug { // not creatable... // private Debug() { } ///Provides a set of properties and /// methods /// for debugging code. ////// public static TraceListenerCollection Listeners { [SecurityPermission(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.UnmanagedCode)] [HostProtection(SharedState=true)] get { return TraceInternal.Listeners; } } ///Gets /// the collection of listeners that is monitoring the debug /// output. ////// public static bool AutoFlush { [SecurityPermission(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.UnmanagedCode)] get { return TraceInternal.AutoFlush; } [SecurityPermission(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.UnmanagedCode)] set { TraceInternal.AutoFlush = value; } } ///Gets or sets a value indicating whether ///should be called on the /// /// after every write. /// public static int IndentLevel { get { return TraceInternal.IndentLevel; } set { TraceInternal.IndentLevel = value; } } ///Gets or sets /// the indent level. ////// public static int IndentSize { get { return TraceInternal.IndentSize; } set { TraceInternal.IndentSize = value; } } ///Gets or sets the number of spaces in an indent. ////// [System.Diagnostics.Conditional("DEBUG")] public static void Flush() { TraceInternal.Flush(); } ///Clears the output buffer, and causes buffered data to /// be written to the ///. /// [System.Diagnostics.Conditional("DEBUG")] [SecurityPermission(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.UnmanagedCode)] public static void Close() { TraceInternal.Close(); } ///Clears the output buffer, and then closes the ///so that they no longer receive /// debugging output. /// [System.Diagnostics.Conditional("DEBUG")] public static void Assert(bool condition) { TraceInternal.Assert(condition); } ///Checks for a condition, and outputs the callstack if the condition is ///. /// [System.Diagnostics.Conditional("DEBUG")] public static void Assert(bool condition, string message) { TraceInternal.Assert(condition, message); } ///Checks for a condition, and displays a message if the condition is /// ///. /// [System.Diagnostics.Conditional("DEBUG")] public static void Assert(bool condition, string message, string detailMessage) { TraceInternal.Assert(condition, message, detailMessage); } ///Checks for a condition, and displays both the specified messages if the condition /// is ///. /// [System.Diagnostics.Conditional("DEBUG")] public static void Fail(string message) { TraceInternal.Fail(message); } ///Emits or displays a message for an assertion that always fails. ////// [System.Diagnostics.Conditional("DEBUG")] public static void Fail(string message, string detailMessage) { TraceInternal.Fail(message, detailMessage); } [System.Diagnostics.Conditional("DEBUG")] public static void Print(string message) { TraceInternal.WriteLine(message); } [System.Diagnostics.Conditional("DEBUG")] public static void Print(string format, params object[] args) { TraceInternal.WriteLine(String.Format(CultureInfo.InvariantCulture, format, args)); } ///Emits or displays both messages for an assertion that always fails. ////// [System.Diagnostics.Conditional("DEBUG")] public static void Write(string message) { TraceInternal.Write(message); } ///Writes a message to the trace listeners in the ///collection. /// [System.Diagnostics.Conditional("DEBUG")] public static void Write(object value) { TraceInternal.Write(value); } ///Writes the name of the value /// parameter to the trace listeners in the ///collection. /// [System.Diagnostics.Conditional("DEBUG")] public static void Write(string message, string category) { TraceInternal.Write(message, category); } ///Writes a category name and message /// to the trace listeners in the ///collection. /// [System.Diagnostics.Conditional("DEBUG")] public static void Write(object value, string category) { TraceInternal.Write(value, category); } ///Writes a category name and the name of the value parameter to the trace /// listeners in the ///collection. /// [System.Diagnostics.Conditional("DEBUG")] public static void WriteLine(string message) { TraceInternal.WriteLine(message); } ///Writes a message followed by a line terminator to the trace listeners in the /// ///collection. The default line terminator /// is a carriage return followed by a line feed (\r\n). /// [System.Diagnostics.Conditional("DEBUG")] public static void WriteLine(object value) { TraceInternal.WriteLine(value); } ///Writes the name of the value /// parameter followed by a line terminator to the /// trace listeners in the ///collection. The default line /// terminator is a carriage return followed by a line feed (\r\n). /// [System.Diagnostics.Conditional("DEBUG")] public static void WriteLine(string message, string category) { TraceInternal.WriteLine(message, category); } ///Writes a category name and message followed by a line terminator to the trace /// listeners in the ///collection. The default line /// terminator is a carriage return followed by a line feed (\r\n). /// [System.Diagnostics.Conditional("DEBUG")] public static void WriteLine(object value, string category) { TraceInternal.WriteLine(value, category); } ///Writes a category name and the name of the value /// parameter followed by a line /// terminator to the trace listeners in the ///collection. The /// default line terminator is a carriage return followed by a line feed (\r\n). /// [System.Diagnostics.Conditional("DEBUG")] public static void WriteIf(bool condition, string message) { TraceInternal.WriteIf(condition, message); } ///Writes a message to the trace listeners in the ///collection /// if a condition is /// . /// [System.Diagnostics.Conditional("DEBUG")] public static void WriteIf(bool condition, object value) { TraceInternal.WriteIf(condition, value); } ///Writes the name of the value /// parameter to the trace listeners in the ////// collection if a condition is /// . /// [System.Diagnostics.Conditional("DEBUG")] public static void WriteIf(bool condition, string message, string category) { TraceInternal.WriteIf(condition, message, category); } ///Writes a category name and message /// to the trace listeners in the ////// collection if a condition is /// . /// [System.Diagnostics.Conditional("DEBUG")] public static void WriteIf(bool condition, object value, string category) { TraceInternal.WriteIf(condition, value, category); } ///Writes a category name and the name of the value /// parameter to the trace /// listeners in the ///collection if a condition is /// . /// [System.Diagnostics.Conditional("DEBUG")] public static void WriteLineIf(bool condition, string message) { TraceInternal.WriteLineIf(condition, message); } ///Writes a message followed by a line terminator to the trace listeners in the /// ///collection if a condition is /// . The default line terminator is a carriage return followed /// by a line feed (\r\n). /// [System.Diagnostics.Conditional("DEBUG")] public static void WriteLineIf(bool condition, object value) { TraceInternal.WriteLineIf(condition, value); } ///Writes the name of the value /// parameter followed by a line terminator to the /// trace listeners in the ///collection if a condition is /// . The default line terminator is a carriage return followed /// by a line feed (\r\n). /// [System.Diagnostics.Conditional("DEBUG")] public static void WriteLineIf(bool condition, string message, string category) { TraceInternal.WriteLineIf(condition, message, category); } ///Writes a category name and message /// followed by a line terminator to the trace /// listeners in the ///collection if a condition is /// . The default line terminator is a carriage return followed /// by a line feed (\r\n). /// [System.Diagnostics.Conditional("DEBUG")] public static void WriteLineIf(bool condition, object value, string category) { TraceInternal.WriteLineIf(condition, value, category); } ///Writes a category name and the name of the value parameter followed by a line /// terminator to the trace listeners in the ///collection /// if a condition is . The default line terminator is a carriage /// return followed by a line feed (\r\n). /// [System.Diagnostics.Conditional("DEBUG")] public static void Indent() { TraceInternal.Indent(); } ///[To be supplied.] ////// [System.Diagnostics.Conditional("DEBUG")] public static void Unindent() { TraceInternal.Unindent(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.[To be supplied.] ///
Link Menu
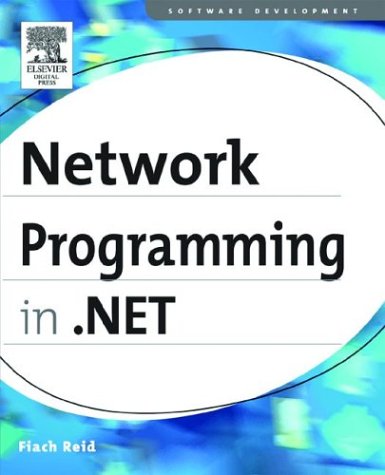
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- RSACryptoServiceProvider.cs
- ExtentKey.cs
- TransactionFlowProperty.cs
- FileDialog_Vista_Interop.cs
- Normalization.cs
- DesignerOptionService.cs
- SourceElementsCollection.cs
- XmlSchemaInfo.cs
- ExceptionNotification.cs
- SqlDataSourceConfigureFilterForm.cs
- ISO2022Encoding.cs
- WebServiceReceiveDesigner.cs
- TableItemPattern.cs
- ActivationServices.cs
- MergeFilterQuery.cs
- BoolExpressionVisitors.cs
- Configuration.cs
- WindowVisualStateTracker.cs
- HandledEventArgs.cs
- ArrayElementGridEntry.cs
- SpotLight.cs
- MissingManifestResourceException.cs
- CapabilitiesAssignment.cs
- serverconfig.cs
- SelectedGridItemChangedEvent.cs
- Conditional.cs
- Rotation3DAnimation.cs
- DataTableMappingCollection.cs
- ProviderIncompatibleException.cs
- DesignerLoader.cs
- LabelDesigner.cs
- BindingsCollection.cs
- ConnectionPool.cs
- RectAnimationUsingKeyFrames.cs
- NameValueConfigurationCollection.cs
- ContentElement.cs
- CqlQuery.cs
- SQLDecimal.cs
- MemberDescriptor.cs
- CqlLexerHelpers.cs
- IProducerConsumerCollection.cs
- ListBase.cs
- ZoneLinkButton.cs
- MultiSelectRootGridEntry.cs
- RayMeshGeometry3DHitTestResult.cs
- InternalBufferOverflowException.cs
- TimeSpanOrInfiniteConverter.cs
- SiteMapPath.cs
- StoragePropertyMapping.cs
- XmlSchemaComplexContentExtension.cs
- ClientRuntimeConfig.cs
- BevelBitmapEffect.cs
- Knowncolors.cs
- ClickablePoint.cs
- UrlMappingsSection.cs
- CodeGeneratorOptions.cs
- AttachedPropertyBrowsableForTypeAttribute.cs
- DataBoundControlHelper.cs
- AppearanceEditorPart.cs
- SevenBitStream.cs
- ToolStripControlHost.cs
- CalendarAutomationPeer.cs
- LicenseProviderAttribute.cs
- DataStreams.cs
- DataContractJsonSerializerOperationBehavior.cs
- MailWriter.cs
- TypeCollectionPropertyEditor.cs
- AcceptorSessionSymmetricTransportSecurityProtocol.cs
- Knowncolors.cs
- PreviewKeyDownEventArgs.cs
- ToolStripGripRenderEventArgs.cs
- ResourcesGenerator.cs
- QilStrConcatenator.cs
- securitycriticaldataClass.cs
- BrowserCapabilitiesFactoryBase.cs
- TraceLevelStore.cs
- RangeEnumerable.cs
- CurrencyManager.cs
- DoubleConverter.cs
- relpropertyhelper.cs
- DeferredRunTextReference.cs
- MetadataPropertyCollection.cs
- Renderer.cs
- ConfigXmlWhitespace.cs
- SqlDataSource.cs
- UserPreferenceChangedEventArgs.cs
- ContentType.cs
- ProfileSettingsCollection.cs
- ProfileGroupSettingsCollection.cs
- Validator.cs
- CodeCatchClause.cs
- GestureRecognizer.cs
- GenericNameHandler.cs
- WmpBitmapEncoder.cs
- BindingListCollectionView.cs
- CommandEventArgs.cs
- WebConfigurationHostFileChange.cs
- TextFindEngine.cs
- MdiWindowListItemConverter.cs
- Separator.cs