Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Wmi / managed / System / Management / ManagementEventArgs.cs / 1305376 / ManagementEventArgs.cs
using System; namespace System.Management { internal class IdentifierChangedEventArgs : EventArgs { internal IdentifierChangedEventArgs () {} } internal class InternalObjectPutEventArgs : EventArgs { private ManagementPath path; internal InternalObjectPutEventArgs (ManagementPath path) { this.path = path.Clone(); } internal ManagementPath Path { get { return path; } } } ////// public abstract class ManagementEventArgs : EventArgs { private object context; ///Represents the virtual base class to hold event data for WMI events. ////// Constructor. This is not callable directly by applications. /// /// The operation context which is echoed back /// from the operation which trigerred the event. internal ManagementEventArgs (object context) { this.context = context; } ////// ///Gets the operation context echoed back /// from the operation that triggered the event. ////// A WMI context object containing /// context information provided by the operation that triggered the event. /// public object Context { get { return context; } } } ////// public class ObjectReadyEventArgs : ManagementEventArgs { private ManagementBaseObject wmiObject; ///Holds event data for the ///event. /// Constructor. /// /// The operation context which is echoed back /// from the operation which triggerred the event. /// The newly arrived WmiObject. internal ObjectReadyEventArgs ( object context, ManagementBaseObject wmiObject ) : base (context) { this.wmiObject = wmiObject; } ////// ///Gets the newly-returned object. ////// public ManagementBaseObject NewObject { get { return wmiObject; } } } ///A ///representing the /// newly-returned object. /// public class CompletedEventArgs : ManagementEventArgs { private readonly int status; private readonly ManagementBaseObject wmiObject; ///Holds event data for the ///event. /// Constructor. /// /// The operation context which is echoed back /// from the operation which trigerred the event. /// The completion status of the operation. /// Additional status information /// encapsulated within a WmiObject. This may be null. internal CompletedEventArgs ( object context, int status, ManagementBaseObject wmiStatusObject ) : base (context) { wmiObject = wmiStatusObject; this.status = status; } ////// ///Gets or sets additional status information /// within a WMI object. This may be null. ////// public ManagementBaseObject StatusObject { get { return wmiObject; } } ////// if an error did not occur. Otherwise, may be non-null if the provider /// supports extended error information. /// ///Gets the completion status of the operation. ////// public ManagementStatus Status { get { return (ManagementStatus) status; } } } ///A ///value /// indicating the return code of the operation. /// public class ObjectPutEventArgs : ManagementEventArgs { private ManagementPath wmiPath; ///Holds event data for the ///event. /// Constructor /// /// The operation context which is echoed back /// from the operation which trigerred the event. /// The WmiPath representing the identity of the /// object that has been put. internal ObjectPutEventArgs ( object context, ManagementPath path ) : base (context) { wmiPath = path; } ////// ///Gets the identity of the /// object that has been put. ////// public ManagementPath Path { get { return wmiPath; } } } ///A ///containing the path of the object that has /// been put. /// public class ProgressEventArgs : ManagementEventArgs { private int upperBound; private int current; private string message; ///Holds event data for the ///event. /// Constructor /// /// The operation context which is echoed back /// from the operation which trigerred the event. /// A quantity representing the total /// amount of work required to be done by the operation. /// A quantity representing the current /// amount of work required to be done by the operation. This is /// always less than or equal to upperBound. /// Optional additional information regarding /// operation progress. internal ProgressEventArgs ( object context, int upperBound, int current, string message ) : base (context) { this.upperBound = upperBound; this.current = current; this.message = message; } ////// ///Gets the total /// amount of work required to be done by the operation. ////// An integer representing the total /// amount of work for the operation. /// public int UpperBound { get { return upperBound; } } ////// ///Gets the current amount of work /// done by the operation. This is always less than or equal to ///. /// public int Current { get { return current; } } ///An integer representing the current amount of work /// already completed by the operation. ////// ///Gets or sets optional additional information regarding the operation's progress. ////// A string containing additional /// information regarding the operation's progress. /// public string Message { get { return (null != message) ? message : String.Empty; } } } ////// public class EventArrivedEventArgs : ManagementEventArgs { private ManagementBaseObject eventObject; internal EventArrivedEventArgs ( object context, ManagementBaseObject eventObject) : base (context) { this.eventObject = eventObject; } ///Holds event data for the ///event. /// ///Gets the WMI event that was delivered. ////// The object representing the WMI event. /// public ManagementBaseObject NewEvent { get { return this.eventObject; } } } ////// public class StoppedEventArgs : ManagementEventArgs { private int status; internal StoppedEventArgs ( object context, int status) : base (context) { this.status = status; } ///Holds event data for the ///event. /// ///Gets the completion status of the operation. ////// public ManagementStatus Status { get { return (ManagementStatus) status; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.A ///value representing the status of the /// operation.
Link Menu
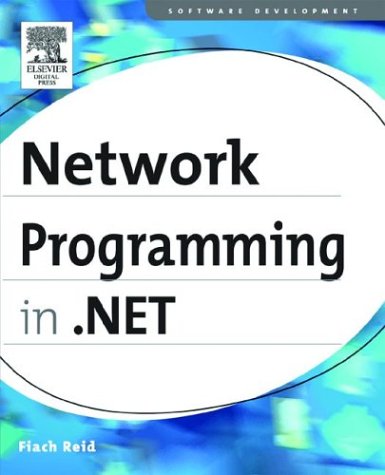
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- NullableBoolConverter.cs
- ProfileModule.cs
- IntegrationExceptionEventArgs.cs
- LinearGradientBrush.cs
- ZipIOModeEnforcingStream.cs
- Setter.cs
- XmlWrappingReader.cs
- assertwrapper.cs
- XPathNavigatorKeyComparer.cs
- WindowsGraphics2.cs
- Freezable.cs
- SHA384.cs
- ErrorRuntimeConfig.cs
- DocumentViewerBase.cs
- BindingManagerDataErrorEventArgs.cs
- QilCloneVisitor.cs
- FixedSOMElement.cs
- NativeObjectSecurity.cs
- RefreshPropertiesAttribute.cs
- FrameworkTextComposition.cs
- SmtpTransport.cs
- XmlNamespaceMappingCollection.cs
- DataKey.cs
- CrossAppDomainChannel.cs
- RequestContext.cs
- PixelShader.cs
- ListViewCommandEventArgs.cs
- oledbmetadatacollectionnames.cs
- Image.cs
- EncryptedPackage.cs
- TextParagraphProperties.cs
- IgnoreFileBuildProvider.cs
- DataReceivedEventArgs.cs
- LogicalTreeHelper.cs
- DocumentPageHost.cs
- OracleColumn.cs
- SignatureHelper.cs
- ObjectQueryState.cs
- TextureBrush.cs
- ConfigsHelper.cs
- TreeNode.cs
- CodeArrayIndexerExpression.cs
- KeyValuePair.cs
- Stroke2.cs
- OrderedEnumerableRowCollection.cs
- ControlParameter.cs
- SoapIgnoreAttribute.cs
- UnsignedPublishLicense.cs
- XmlElementAttribute.cs
- ServiceHost.cs
- GeneratedCodeAttribute.cs
- AuthorizationBehavior.cs
- LookupBindingPropertiesAttribute.cs
- ACL.cs
- XmlSchemaImport.cs
- XsltQilFactory.cs
- Monitor.cs
- GridViewRowCollection.cs
- AddInEnvironment.cs
- DbFunctionCommandTree.cs
- Console.cs
- TimeSpanParse.cs
- NullableConverter.cs
- _LazyAsyncResult.cs
- RC2.cs
- SplitterPanelDesigner.cs
- Metadata.cs
- UInt64Storage.cs
- PlatformNotSupportedException.cs
- SafePEFileHandle.cs
- FeatureManager.cs
- ButtonChrome.cs
- ExpressionBuilderContext.cs
- RepeatInfo.cs
- Point3DCollection.cs
- StringArrayConverter.cs
- ToolStripOverflowButton.cs
- RolePrincipal.cs
- DateTimeOffset.cs
- StorageScalarPropertyMapping.cs
- TextElementEnumerator.cs
- ExcCanonicalXml.cs
- RelatedImageListAttribute.cs
- Win32PrintDialog.cs
- Speller.cs
- MonthChangedEventArgs.cs
- SqlConnectionStringBuilder.cs
- DataGridViewColumnEventArgs.cs
- CodeDomConfigurationHandler.cs
- Point3DValueSerializer.cs
- PackageProperties.cs
- HybridDictionary.cs
- BrowserCapabilitiesCompiler.cs
- ObjectDataSourceView.cs
- RegexRunnerFactory.cs
- MemberRelationshipService.cs
- PathGeometry.cs
- HttpWebRequest.cs
- XmlExceptionHelper.cs
- RefType.cs