Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / ndp / fx / src / DataEntity / System / Data / Common / internal / materialization / shaperfactory.cs / 3 / shaperfactory.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //[....] //----------------------------------------------------------------------------- using System.Data.Common.QueryCache; using System.Data.Metadata.Edm; using System.Data.Objects; using System.Data.Objects.Internal; using System.Data.Query.InternalTrees; using System.Diagnostics; namespace System.Data.Common.Internal.Materialization { ////// An immutable type used to generate Shaper instances. /// internal abstract class ShaperFactory { internal static ShaperFactory Create(Type elementType, QueryCacheManager cacheManager, ColumnMap columnMap, MetadataWorkspace metadata, SpanIndex spanInfo, MergeOption mergeOption, bool valueLayer) { ShaperFactoryCreator creator = (ShaperFactoryCreator)Activator.CreateInstance(typeof(TypedShaperFactoryCreator<>).MakeGenericType(elementType)); return creator.TypedCreate(cacheManager, columnMap, metadata, spanInfo, mergeOption, valueLayer); } private abstract class ShaperFactoryCreator { internal abstract ShaperFactory TypedCreate(QueryCacheManager cacheManager, ColumnMap columnMap, MetadataWorkspace metadata, SpanIndex spanInfo, MergeOption mergeOption, bool valueLayer); } private sealed class TypedShaperFactoryCreator: ShaperFactoryCreator { public TypedShaperFactoryCreator() {} internal override ShaperFactory TypedCreate(QueryCacheManager cacheManager, ColumnMap columnMap, MetadataWorkspace metadata, SpanIndex spanInfo, MergeOption mergeOption, bool valueLayer) { return Translator.TranslateColumnMap (cacheManager, columnMap, metadata, spanInfo, mergeOption, valueLayer); } } } /// /// Typed ShaperFactory /// internal class ShaperFactory: ShaperFactory { private readonly int _stateCount; private readonly CoordinatorFactory _rootCoordinatorFactory; private readonly Action _checkPermissions; private readonly MergeOption _mergeOption; internal ShaperFactory(int stateCount, CoordinatorFactory rootCoordinatorFactory, Action checkPermissions, MergeOption mergeOption) { _stateCount = stateCount; _rootCoordinatorFactory = rootCoordinatorFactory; _checkPermissions = checkPermissions; _mergeOption = mergeOption; } /// /// Factory method to create the Shaper for Object Layer queries. /// internal ShaperCreate(DbDataReader reader, ObjectContext context, MetadataWorkspace workspace, MergeOption mergeOption) { Debug.Assert(mergeOption == _mergeOption, "executing a query with a different mergeOption than was used to compile the delegate"); return new Shaper (reader, context, workspace, mergeOption, _stateCount, _rootCoordinatorFactory, _checkPermissions); } /// /// Factory method to create the Shaper for Value Layer queries. /// internal ShaperCreate(DbDataReader reader, MetadataWorkspace workspace) { Debug.Assert(MergeOption.NoTracking == _mergeOption, "executing a query with a different mergeOption than was used to compile the delegate"); return new Shaper (reader, null, workspace, MergeOption.NoTracking, _stateCount, _rootCoordinatorFactory, _checkPermissions); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //[....] //----------------------------------------------------------------------------- using System.Data.Common.QueryCache; using System.Data.Metadata.Edm; using System.Data.Objects; using System.Data.Objects.Internal; using System.Data.Query.InternalTrees; using System.Diagnostics; namespace System.Data.Common.Internal.Materialization { ////// An immutable type used to generate Shaper instances. /// internal abstract class ShaperFactory { internal static ShaperFactory Create(Type elementType, QueryCacheManager cacheManager, ColumnMap columnMap, MetadataWorkspace metadata, SpanIndex spanInfo, MergeOption mergeOption, bool valueLayer) { ShaperFactoryCreator creator = (ShaperFactoryCreator)Activator.CreateInstance(typeof(TypedShaperFactoryCreator<>).MakeGenericType(elementType)); return creator.TypedCreate(cacheManager, columnMap, metadata, spanInfo, mergeOption, valueLayer); } private abstract class ShaperFactoryCreator { internal abstract ShaperFactory TypedCreate(QueryCacheManager cacheManager, ColumnMap columnMap, MetadataWorkspace metadata, SpanIndex spanInfo, MergeOption mergeOption, bool valueLayer); } private sealed class TypedShaperFactoryCreator: ShaperFactoryCreator { public TypedShaperFactoryCreator() {} internal override ShaperFactory TypedCreate(QueryCacheManager cacheManager, ColumnMap columnMap, MetadataWorkspace metadata, SpanIndex spanInfo, MergeOption mergeOption, bool valueLayer) { return Translator.TranslateColumnMap (cacheManager, columnMap, metadata, spanInfo, mergeOption, valueLayer); } } } /// /// Typed ShaperFactory /// internal class ShaperFactory: ShaperFactory { private readonly int _stateCount; private readonly CoordinatorFactory _rootCoordinatorFactory; private readonly Action _checkPermissions; private readonly MergeOption _mergeOption; internal ShaperFactory(int stateCount, CoordinatorFactory rootCoordinatorFactory, Action checkPermissions, MergeOption mergeOption) { _stateCount = stateCount; _rootCoordinatorFactory = rootCoordinatorFactory; _checkPermissions = checkPermissions; _mergeOption = mergeOption; } /// /// Factory method to create the Shaper for Object Layer queries. /// internal ShaperCreate(DbDataReader reader, ObjectContext context, MetadataWorkspace workspace, MergeOption mergeOption) { Debug.Assert(mergeOption == _mergeOption, "executing a query with a different mergeOption than was used to compile the delegate"); return new Shaper (reader, context, workspace, mergeOption, _stateCount, _rootCoordinatorFactory, _checkPermissions); } /// /// Factory method to create the Shaper for Value Layer queries. /// internal ShaperCreate(DbDataReader reader, MetadataWorkspace workspace) { Debug.Assert(MergeOption.NoTracking == _mergeOption, "executing a query with a different mergeOption than was used to compile the delegate"); return new Shaper (reader, null, workspace, MergeOption.NoTracking, _stateCount, _rootCoordinatorFactory, _checkPermissions); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
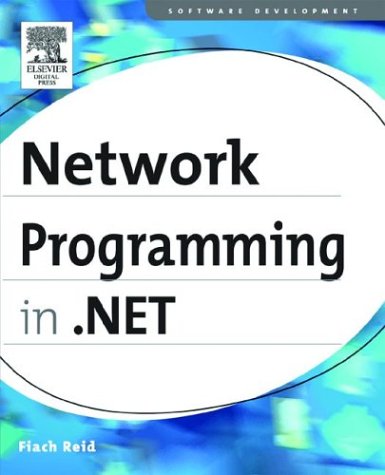
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CustomValidator.cs
- TemplateLookupAction.cs
- EntityDataSourceViewSchema.cs
- XhtmlTextWriter.cs
- XmlSchemaSequence.cs
- InfiniteTimeSpanConverter.cs
- SourceLineInfo.cs
- StrokeNode.cs
- MultiPartWriter.cs
- SQLMoney.cs
- DataBindingCollectionEditor.cs
- InputBindingCollection.cs
- VisualStyleInformation.cs
- DesignParameter.cs
- CodePageEncoding.cs
- CompositeActivityCodeGenerator.cs
- WebHttpEndpoint.cs
- HashMembershipCondition.cs
- CapabilitiesSection.cs
- ComponentEvent.cs
- ProxyManager.cs
- UnknownExceptionActionHelper.cs
- ConstraintConverter.cs
- FixedSOMSemanticBox.cs
- StyleCollection.cs
- EntityCollectionChangedParams.cs
- ListViewHitTestInfo.cs
- CompilerCollection.cs
- SkinBuilder.cs
- CrossSiteScriptingValidation.cs
- CaretElement.cs
- DataMisalignedException.cs
- TextParagraphProperties.cs
- NotificationContext.cs
- WorkerRequest.cs
- ProjectionPathSegment.cs
- DataGridItemAttachedStorage.cs
- XamlTypeMapper.cs
- FrameworkElement.cs
- CollectionEditorDialog.cs
- TypeUnloadedException.cs
- ViewBase.cs
- ReadOnlyPropertyMetadata.cs
- ExpressionReplacer.cs
- SerializationHelper.cs
- ViewRendering.cs
- TranslateTransform3D.cs
- Int64AnimationUsingKeyFrames.cs
- CaseInsensitiveOrdinalStringComparer.cs
- Label.cs
- ComponentResourceManager.cs
- TaskHelper.cs
- UnsafeNativeMethods.cs
- Mutex.cs
- DataGridRow.cs
- QueryRewriter.cs
- SspiNegotiationTokenProvider.cs
- KeyFrames.cs
- InvalidCommandTreeException.cs
- RegisteredExpandoAttribute.cs
- PixelShader.cs
- EvidenceTypeDescriptor.cs
- XmlTextWriter.cs
- DataControlHelper.cs
- GenerateTemporaryAssemblyTask.cs
- AssemblyCache.cs
- controlskin.cs
- NTAccount.cs
- X509KeyIdentifierClauseType.cs
- ComponentCommands.cs
- DelayedRegex.cs
- WebPartRestoreVerb.cs
- CrossContextChannel.cs
- Quaternion.cs
- DrawListViewItemEventArgs.cs
- DBSchemaTable.cs
- versioninfo.cs
- ProviderMetadata.cs
- WebBodyFormatMessageProperty.cs
- X509Chain.cs
- TickBar.cs
- SharedStatics.cs
- SecurityManager.cs
- XmlName.cs
- XamlInt32CollectionSerializer.cs
- WebResourceAttribute.cs
- OAVariantLib.cs
- MergablePropertyAttribute.cs
- BitStack.cs
- PeerNameRecordCollection.cs
- DecimalConverter.cs
- FormsAuthentication.cs
- Funcletizer.cs
- SafeFileMappingHandle.cs
- Stacktrace.cs
- XmlSortKeyAccumulator.cs
- CodeGenerator.cs
- InfoCardBaseException.cs
- _FtpControlStream.cs
- CodeCatchClauseCollection.cs