Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / xsp / System / Web / UI / HTMLTagNameToTypeMapper.cs / 1 / HTMLTagNameToTypeMapper.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* * Mapper of html tags to control types. * * Copyright (c) 1998 Microsoft Corporation */ namespace System.Web.UI { using System.ComponentModel; using System; using System.Collections; using System.Collections.Specialized; using System.Reflection; using System.Web.UI.HtmlControls; using System.Web.Util; using System.Globalization; internal class HtmlTagNameToTypeMapper : ITagNameToTypeMapper { static Hashtable _tagMap; static Hashtable _inputTypes; internal HtmlTagNameToTypeMapper() { } /*public*/ Type ITagNameToTypeMapper.GetControlType(string tagName, IDictionary attributeBag) { Type controlType; if (_tagMap == null) { Hashtable t = new Hashtable(10, StringComparer.OrdinalIgnoreCase); t.Add("a", typeof(HtmlAnchor)); t.Add("button", typeof(HtmlButton)); t.Add("form", typeof(HtmlForm)); t.Add("head", typeof(HtmlHead)); t.Add("img", typeof(HtmlImage)); t.Add("textarea", typeof(HtmlTextArea)); t.Add("select", typeof(HtmlSelect)); t.Add("table", typeof(HtmlTable)); t.Add("tr", typeof(HtmlTableRow)); t.Add("td", typeof(HtmlTableCell)); t.Add("th", typeof(HtmlTableCell)); _tagMap = t; } if (_inputTypes == null) { Hashtable t = new Hashtable(10, StringComparer.OrdinalIgnoreCase); t.Add("text", typeof(HtmlInputText)); t.Add("password", typeof(HtmlInputPassword)); t.Add("button", typeof(HtmlInputButton)); t.Add("submit", typeof(HtmlInputSubmit)); t.Add("reset", typeof(HtmlInputReset)); t.Add("image", typeof(HtmlInputImage)); t.Add("checkbox", typeof(HtmlInputCheckBox)); t.Add("radio", typeof(HtmlInputRadioButton)); t.Add("hidden", typeof(HtmlInputHidden)); t.Add("file", typeof(HtmlInputFile)); _inputTypes = t; } if (StringUtil.EqualsIgnoreCase("input", tagName)) { string type = (string)attributeBag["type"]; if (type == null) type = "text"; controlType = (Type)_inputTypes[type]; if (controlType == null) throw new HttpException( SR.GetString(SR.Invalid_type_for_input_tag, type)); } else { controlType = (Type)_tagMap[tagName]; if (controlType == null) controlType = typeof(HtmlGenericControl); } return controlType; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* * Mapper of html tags to control types. * * Copyright (c) 1998 Microsoft Corporation */ namespace System.Web.UI { using System.ComponentModel; using System; using System.Collections; using System.Collections.Specialized; using System.Reflection; using System.Web.UI.HtmlControls; using System.Web.Util; using System.Globalization; internal class HtmlTagNameToTypeMapper : ITagNameToTypeMapper { static Hashtable _tagMap; static Hashtable _inputTypes; internal HtmlTagNameToTypeMapper() { } /*public*/ Type ITagNameToTypeMapper.GetControlType(string tagName, IDictionary attributeBag) { Type controlType; if (_tagMap == null) { Hashtable t = new Hashtable(10, StringComparer.OrdinalIgnoreCase); t.Add("a", typeof(HtmlAnchor)); t.Add("button", typeof(HtmlButton)); t.Add("form", typeof(HtmlForm)); t.Add("head", typeof(HtmlHead)); t.Add("img", typeof(HtmlImage)); t.Add("textarea", typeof(HtmlTextArea)); t.Add("select", typeof(HtmlSelect)); t.Add("table", typeof(HtmlTable)); t.Add("tr", typeof(HtmlTableRow)); t.Add("td", typeof(HtmlTableCell)); t.Add("th", typeof(HtmlTableCell)); _tagMap = t; } if (_inputTypes == null) { Hashtable t = new Hashtable(10, StringComparer.OrdinalIgnoreCase); t.Add("text", typeof(HtmlInputText)); t.Add("password", typeof(HtmlInputPassword)); t.Add("button", typeof(HtmlInputButton)); t.Add("submit", typeof(HtmlInputSubmit)); t.Add("reset", typeof(HtmlInputReset)); t.Add("image", typeof(HtmlInputImage)); t.Add("checkbox", typeof(HtmlInputCheckBox)); t.Add("radio", typeof(HtmlInputRadioButton)); t.Add("hidden", typeof(HtmlInputHidden)); t.Add("file", typeof(HtmlInputFile)); _inputTypes = t; } if (StringUtil.EqualsIgnoreCase("input", tagName)) { string type = (string)attributeBag["type"]; if (type == null) type = "text"; controlType = (Type)_inputTypes[type]; if (controlType == null) throw new HttpException( SR.GetString(SR.Invalid_type_for_input_tag, type)); } else { controlType = (Type)_tagMap[tagName]; if (controlType == null) controlType = typeof(HtmlGenericControl); } return controlType; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
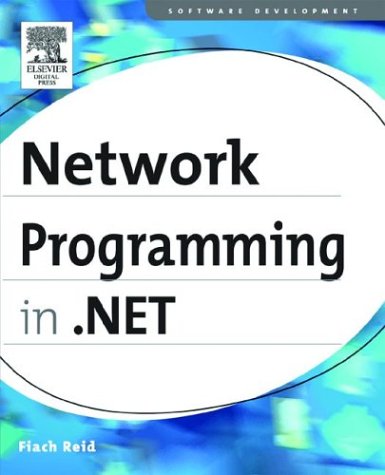
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SimpleApplicationHost.cs
- ValidatedControlConverter.cs
- HitTestParameters.cs
- DataGridViewRowStateChangedEventArgs.cs
- LinqDataSourceValidationException.cs
- PropertyPathConverter.cs
- StringComparer.cs
- ThreadPool.cs
- ClientScriptManagerWrapper.cs
- StdValidatorsAndConverters.cs
- FileSecurity.cs
- ColorBlend.cs
- FirewallWrapper.cs
- WindowsSecurityTokenAuthenticator.cs
- CodeCastExpression.cs
- processwaithandle.cs
- Vector3DConverter.cs
- PropertyMetadata.cs
- ValueTable.cs
- HtmlInputImage.cs
- MetafileHeaderEmf.cs
- ScaleTransform3D.cs
- UITypeEditor.cs
- SafeRightsManagementSessionHandle.cs
- CancellationHandlerDesigner.cs
- IItemContainerGenerator.cs
- ControlAdapter.cs
- RestClientProxyHandler.cs
- HttpWrapper.cs
- METAHEADER.cs
- FusionWrap.cs
- FormViewPagerRow.cs
- PropertyGeneratedEventArgs.cs
- ToolStripPanelRenderEventArgs.cs
- XmlHierarchicalEnumerable.cs
- TransportManager.cs
- ComponentEvent.cs
- ResourceManagerWrapper.cs
- ValidationRuleCollection.cs
- PolyBezierSegment.cs
- MulticastNotSupportedException.cs
- PassportAuthenticationModule.cs
- HtmlToClrEventProxy.cs
- XmlDataProvider.cs
- MonthChangedEventArgs.cs
- ContractListAdapter.cs
- WindowPatternIdentifiers.cs
- SimpleHandlerBuildProvider.cs
- NumericUpDownAccelerationCollection.cs
- AddressAccessDeniedException.cs
- UserPersonalizationStateInfo.cs
- PathParser.cs
- OutArgument.cs
- SHA384.cs
- GAC.cs
- COM2Properties.cs
- SoapIgnoreAttribute.cs
- StructuredTypeEmitter.cs
- SafeProcessHandle.cs
- PropertyInformation.cs
- PartialList.cs
- HealthMonitoringSectionHelper.cs
- TraceLevelStore.cs
- DesignerVerbCollection.cs
- WebAdminConfigurationHelper.cs
- ImageAttributes.cs
- DataObject.cs
- DataGridViewAutoSizeModeEventArgs.cs
- PhysicalOps.cs
- SelectionPatternIdentifiers.cs
- NativeRightsManagementAPIsStructures.cs
- Accessors.cs
- MediaEntryAttribute.cs
- TheQuery.cs
- Configuration.cs
- FormViewUpdateEventArgs.cs
- EdmToObjectNamespaceMap.cs
- PointHitTestResult.cs
- CustomError.cs
- RightsManagementPermission.cs
- CellPartitioner.cs
- AsyncInvokeOperation.cs
- FamilyTypeface.cs
- ServiceDesigner.xaml.cs
- XmlRawWriter.cs
- WebPartEditorOkVerb.cs
- CompositionDesigner.cs
- PermissionSetEnumerator.cs
- SessionIDManager.cs
- InkPresenter.cs
- DateTimeOffsetConverter.cs
- RequestCacheEntry.cs
- PersistChildrenAttribute.cs
- RuleValidation.cs
- ColumnHeader.cs
- TreeBuilder.cs
- TypeSystem.cs
- TransformDescriptor.cs
- StrongNamePublicKeyBlob.cs
- ParallelActivityDesigner.cs