Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / UIAutomation / Win32Providers / MS / Internal / AutomationProxies / SafeProcessHandle.cs / 1305600 / SafeProcessHandle.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // // History: // 10/04/2003 : [....] Created //--------------------------------------------------------------------------- using System; using System.Security; using System.Security.Permissions; using System.Runtime.InteropServices; using System.Runtime.CompilerServices; using System.Windows.Automation; using Microsoft.Win32.SafeHandles; using MS.Win32; namespace MS.Internal.AutomationProxies { internal sealed class SafeProcessHandle : SafeHandleZeroOrMinusOneIsInvalid { // This constructor is used by the P/Invoke marshaling layer // to allocate a SafeHandle instance. P/Invoke then does the // appropriate method call, storing the handle in this class. private SafeProcessHandle() : base(true) {} internal SafeProcessHandle(IntPtr hwnd) : base(true) { uint processId; if (hwnd == IntPtr.Zero) { processId = UnsafeNativeMethods.GetCurrentProcessId(); } else { // Get process id... Misc.GetWindowThreadProcessId(hwnd, out processId); } // handle might be used to query for Wow64 information (_QUERY_), or to do cross-process allocs (VM_*) SetHandle(Misc.OpenProcess(NativeMethods.PROCESS_QUERY_INFORMATION | NativeMethods.PROCESS_VM_OPERATION | NativeMethods.PROCESS_VM_READ | NativeMethods.PROCESS_VM_WRITE, false, processId, hwnd)); } // Uncomment this if & only if we need a constructor // that takes a handle from external code //internal SafeProcessHandle(IntPtr preexistingHandle, bool ownsHandle) : base(ownsHandle) //{ // SetHandle(preexistingHandle); //} // protected override bool ReleaseHandle() { return Misc.CloseHandle(handle); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // // History: // 10/04/2003 : [....] Created //--------------------------------------------------------------------------- using System; using System.Security; using System.Security.Permissions; using System.Runtime.InteropServices; using System.Runtime.CompilerServices; using System.Windows.Automation; using Microsoft.Win32.SafeHandles; using MS.Win32; namespace MS.Internal.AutomationProxies { internal sealed class SafeProcessHandle : SafeHandleZeroOrMinusOneIsInvalid { // This constructor is used by the P/Invoke marshaling layer // to allocate a SafeHandle instance. P/Invoke then does the // appropriate method call, storing the handle in this class. private SafeProcessHandle() : base(true) {} internal SafeProcessHandle(IntPtr hwnd) : base(true) { uint processId; if (hwnd == IntPtr.Zero) { processId = UnsafeNativeMethods.GetCurrentProcessId(); } else { // Get process id... Misc.GetWindowThreadProcessId(hwnd, out processId); } // handle might be used to query for Wow64 information (_QUERY_), or to do cross-process allocs (VM_*) SetHandle(Misc.OpenProcess(NativeMethods.PROCESS_QUERY_INFORMATION | NativeMethods.PROCESS_VM_OPERATION | NativeMethods.PROCESS_VM_READ | NativeMethods.PROCESS_VM_WRITE, false, processId, hwnd)); } // Uncomment this if & only if we need a constructor // that takes a handle from external code //internal SafeProcessHandle(IntPtr preexistingHandle, bool ownsHandle) : base(ownsHandle) //{ // SetHandle(preexistingHandle); //} // protected override bool ReleaseHandle() { return Misc.CloseHandle(handle); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
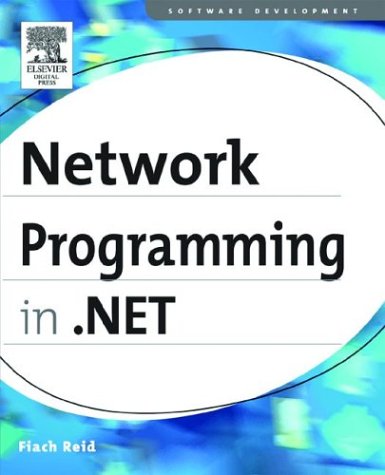
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SqlExpressionNullability.cs
- ConfigXmlSignificantWhitespace.cs
- PersonalizationProviderHelper.cs
- RawStylusActions.cs
- SourceElementsCollection.cs
- BitmapEffectvisualstate.cs
- EventSinkHelperWriter.cs
- panel.cs
- CollectionChangeEventArgs.cs
- SqlProfileProvider.cs
- TextBox.cs
- MessageProperties.cs
- HttpRawResponse.cs
- SynchronizedDispatch.cs
- Camera.cs
- UntrustedRecipientException.cs
- BmpBitmapDecoder.cs
- ListViewItemMouseHoverEvent.cs
- PagerSettings.cs
- VisualBrush.cs
- QuarticEase.cs
- RsaKeyGen.cs
- ComPlusAuthorization.cs
- GlyphingCache.cs
- ProfileServiceManager.cs
- TCEAdapterGenerator.cs
- QueryOutputWriterV1.cs
- ScriptReferenceEventArgs.cs
- MembershipPasswordException.cs
- ExplicitDiscriminatorMap.cs
- StateWorkerRequest.cs
- PersonalizationState.cs
- KeyGesture.cs
- webeventbuffer.cs
- ObjectStateEntryBaseUpdatableDataRecord.cs
- ResourceManagerWrapper.cs
- TableItemProviderWrapper.cs
- DataGridViewAccessibleObject.cs
- SettingsSavedEventArgs.cs
- LinearKeyFrames.cs
- TokenBasedSet.cs
- BindingList.cs
- ComplexType.cs
- ToolStripOverflowButton.cs
- Events.cs
- XamlPoint3DCollectionSerializer.cs
- RegexInterpreter.cs
- DropShadowEffect.cs
- DrawingImage.cs
- XPathQilFactory.cs
- ColorConverter.cs
- oledbconnectionstring.cs
- RolePrincipal.cs
- ColumnReorderedEventArgs.cs
- OverrideMode.cs
- StoreItemCollection.cs
- JsonDeserializer.cs
- Int64AnimationUsingKeyFrames.cs
- VisualTreeUtils.cs
- PropertyIDSet.cs
- CommandBindingCollection.cs
- DesignerContextDescriptor.cs
- FixedDocument.cs
- ColorTranslator.cs
- WarningException.cs
- Model3D.cs
- FileLoadException.cs
- IIS7ConfigurationLoader.cs
- BufferedStream.cs
- ThreadInterruptedException.cs
- EditingCoordinator.cs
- SessionStateSection.cs
- BitmapPalette.cs
- OrderByExpression.cs
- ByteStream.cs
- Util.cs
- hwndwrapper.cs
- CompositeControlDesigner.cs
- Helpers.cs
- ModifiableIteratorCollection.cs
- ClientUtils.cs
- GroupBox.cs
- PointConverter.cs
- StyleXamlParser.cs
- QueryComponents.cs
- ImageDrawing.cs
- WebReferencesBuildProvider.cs
- StateDesigner.Layouts.cs
- FilePrompt.cs
- VoiceInfo.cs
- IIS7UserPrincipal.cs
- CommandEventArgs.cs
- XamlDesignerSerializationManager.cs
- XmlArrayAttribute.cs
- DataSourceView.cs
- CollectionType.cs
- PrintingPermissionAttribute.cs
- NativeMethods.cs
- GridItemProviderWrapper.cs
- MarkupWriter.cs