Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / System / Windows / Ink / Events.cs / 1305600 / Events.cs
//---------------------------------------------------------------------------- // // File: Events.cs // // Description: // Defined our Delegates, EventHandlers and EventArgs // // Authors: samgeo // // Copyright (C) 2003 by Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.Collections.ObjectModel; using System.ComponentModel; using System.Windows; using System.Windows.Media; using System.Windows.Documents; using System.Windows.Ink; using Swi = System.Windows.Ink; using MS.Utility; namespace System.Windows.Controls { ////// The delegate to use for the StrokeCollected event /// public delegate void InkCanvasStrokeCollectedEventHandler(object sender, InkCanvasStrokeCollectedEventArgs e); ////// InkCanvasStrokeCollectedEventArgs /// public class InkCanvasStrokeCollectedEventArgs : RoutedEventArgs { ////// [TBS] /// public InkCanvasStrokeCollectedEventArgs(Swi.Stroke stroke) : base(InkCanvas.StrokeCollectedEvent) { if (stroke == null) { throw new ArgumentNullException("stroke"); } _stroke = stroke; } ////// [TBS] /// public Swi.Stroke Stroke { get { return _stroke; } } ////// The mechanism used to call the type-specific handler on the /// target. /// /// /// The generic handler to call in a type-specific way. /// /// /// The target to call the handler on. /// protected override void InvokeEventHandler(Delegate genericHandler, object genericTarget) { InkCanvasStrokeCollectedEventHandler handler = (InkCanvasStrokeCollectedEventHandler)genericHandler; handler(genericTarget, this); } private Swi.Stroke _stroke; } ////// The delegate to use for the StrokesChanged event /// public delegate void InkCanvasStrokesReplacedEventHandler(object sender, InkCanvasStrokesReplacedEventArgs e); ////// InkCanvasStrokesChangedEventArgs /// public class InkCanvasStrokesReplacedEventArgs : EventArgs { ////// InkCanvasStrokesReplacedEventArgs /// internal InkCanvasStrokesReplacedEventArgs(Swi.StrokeCollection newStrokes, Swi.StrokeCollection previousStrokes) { if (newStrokes == null) { throw new ArgumentNullException("newStrokes"); } if (previousStrokes == null) { throw new ArgumentNullException("previousStrokes"); } _newStrokes = newStrokes; _previousStrokes = previousStrokes; } ////// [TBS] /// public Swi.StrokeCollection NewStrokes { get { return _newStrokes; } } ////// [TBS] /// public Swi.StrokeCollection PreviousStrokes { get { return _previousStrokes; } } private Swi.StrokeCollection _newStrokes; private Swi.StrokeCollection _previousStrokes; } ////// The delegate to use for the SelectionChanging event /// /// This event is only thrown when you change the selection programmatically (through our APIs, not the SelectionService's /// or through our lasso selection behavior /// public delegate void InkCanvasSelectionChangingEventHandler(object sender, InkCanvasSelectionChangingEventArgs e); ////// Event arguments sent when the SelectionChanging event is raised. /// public class InkCanvasSelectionChangingEventArgs : CancelEventArgs { private StrokeCollection _strokes; private List_elements; private bool _strokesChanged; private bool _elementsChanged; /// /// Constructor /// internal InkCanvasSelectionChangingEventArgs(StrokeCollection selectedStrokes, IEnumerableselectedElements) { if (selectedStrokes == null) { throw new ArgumentNullException("selectedStrokes"); } if (selectedElements == null) { throw new ArgumentNullException("selectedElements"); } _strokes = selectedStrokes; List elements = new List (selectedElements); _elements = elements; _strokesChanged = false; _elementsChanged = false; } /// /// An internal flag which indicates the Strokes has changed. /// internal bool StrokesChanged { get { return _strokesChanged; } } ////// An internal flag which indicates the Elements has changed. /// internal bool ElementsChanged { get { return _elementsChanged; } } ////// Set the selected elements /// /// The new selected elements public void SetSelectedElements(IEnumerableselectedElements) { if ( selectedElements == null ) { throw new ArgumentNullException("selectedElements"); } List elements = new List (selectedElements); _elements = elements; _elementsChanged = true; } /// /// Get the selected elements /// ///The selected elements public ReadOnlyCollectionGetSelectedElements() { return new ReadOnlyCollection (_elements); } /// /// Set the selected strokes /// /// The new selected strokes public void SetSelectedStrokes(StrokeCollection selectedStrokes) { if ( selectedStrokes == null ) { throw new ArgumentNullException("selectedStrokes"); } _strokes = selectedStrokes; _strokesChanged = true; } ////// Get the selected strokes /// ///The selected strokes public StrokeCollection GetSelectedStrokes() { // // make a copy of out internal collection. // StrokeCollection sc = new StrokeCollection(); sc.Add(_strokes); return sc; } } ////// The delegate to use for the SelectionMoving, SelectionResizing events /// public delegate void InkCanvasSelectionEditingEventHandler(object sender, InkCanvasSelectionEditingEventArgs e); ////// Event arguments sent when the SelectionChanging event is raised. /// public class InkCanvasSelectionEditingEventArgs : CancelEventArgs { private Rect _oldRectangle; private Rect _newRectangle; ////// Constructor /// internal InkCanvasSelectionEditingEventArgs(Rect oldRectangle, Rect newRectangle) { _oldRectangle = oldRectangle; _newRectangle = newRectangle; } ////// Read access to the OldRectangle, from before the edit. /// public Rect OldRectangle { get { return _oldRectangle;} } ////// Read access to the NewRectangle, resulting from this edit. /// public Rect NewRectangle { get { return _newRectangle;} set {_newRectangle = value;} } } ////// The delegate to use for the InkErasing event /// public delegate void InkCanvasStrokeErasingEventHandler(object sender, InkCanvasStrokeErasingEventArgs e); ////// Event arguments sent when the SelectionChanging event is raised. /// public class InkCanvasStrokeErasingEventArgs : CancelEventArgs { private Swi.Stroke _stroke; ////// Constructor /// internal InkCanvasStrokeErasingEventArgs(Swi.Stroke stroke) { if (stroke == null) { throw new ArgumentNullException("stroke"); } _stroke = stroke; } ////// Read access to the stroke about to be deleted /// public Stroke Stroke { get { return _stroke;} } } ////// TBD /// /// sender /// e public delegate void InkCanvasGestureEventHandler(object sender, InkCanvasGestureEventArgs e); ////// ApplicationGestureEventArgs /// public class InkCanvasGestureEventArgs : RoutedEventArgs { private StrokeCollection _strokes; private List_gestureRecognitionResults; private bool _cancel; /// /// TBD /// /// strokes /// gestureRecognitionResults public InkCanvasGestureEventArgs(StrokeCollection strokes, IEnumerablegestureRecognitionResults) : base(InkCanvas.GestureEvent) { if (strokes == null) { throw new ArgumentNullException("strokes"); } if (strokes.Count < 1) { throw new ArgumentException(SR.Get(SRID.InvalidEmptyStrokeCollection), "strokes"); } if (gestureRecognitionResults == null) { throw new ArgumentNullException("strokes"); } List results = new List (gestureRecognitionResults); if (results.Count == 0) { throw new ArgumentException(SR.Get(SRID.InvalidEmptyArray), "gestureRecognitionResults"); } _strokes = strokes; _gestureRecognitionResults = results; } /// /// TBD /// public StrokeCollection Strokes { get { return _strokes; } } ////// TBD /// ///public ReadOnlyCollection GetGestureRecognitionResults() { return new ReadOnlyCollection (_gestureRecognitionResults); } /// /// Indicates whether the Gesture event needs to be cancelled. /// public bool Cancel { get { return _cancel; } set { _cancel = value; } } ////// The mechanism used to call the type-specific handler on the /// target. /// /// /// The generic handler to call in a type-specific way. /// /// /// The target to call the handler on. /// protected override void InvokeEventHandler(Delegate genericHandler, object genericTarget) { InkCanvasGestureEventHandler handler = (InkCanvasGestureEventHandler)genericHandler; handler(genericTarget, this); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
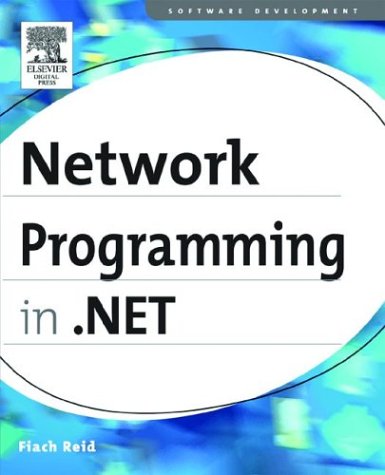
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- InkCollectionBehavior.cs
- DataGridViewButtonCell.cs
- WebPartCloseVerb.cs
- RoleServiceManager.cs
- XmlSchemaImporter.cs
- DataConnectionHelper.cs
- QueryResults.cs
- SelectionChangedEventArgs.cs
- AssemblyBuilder.cs
- ClassDataContract.cs
- Parser.cs
- FamilyTypefaceCollection.cs
- FamilyCollection.cs
- NamespaceEmitter.cs
- WebPartDeleteVerb.cs
- RecordsAffectedEventArgs.cs
- CheckBoxField.cs
- RoleExceptions.cs
- HandleTable.cs
- ZoneMembershipCondition.cs
- Compiler.cs
- Label.cs
- LinkLabel.cs
- OleDbCommandBuilder.cs
- TransactionManager.cs
- DefaultTraceListener.cs
- InfoCardClaim.cs
- ModelItemCollection.cs
- AuthenticateEventArgs.cs
- ControlHelper.cs
- PassportAuthenticationEventArgs.cs
- FileUpload.cs
- ArglessEventHandlerProxy.cs
- TaiwanLunisolarCalendar.cs
- EventLogPermission.cs
- Label.cs
- SiteMapNodeItemEventArgs.cs
- XmlObjectSerializerWriteContextComplexJson.cs
- arclist.cs
- ImmutableObjectAttribute.cs
- SystemFonts.cs
- ZeroOpNode.cs
- CodeCommentStatement.cs
- CompositeFontFamily.cs
- EntityCommand.cs
- CommonDialog.cs
- _ListenerRequestStream.cs
- WindowsListViewSubItem.cs
- DataGridHyperlinkColumn.cs
- EditableTreeList.cs
- SchemaImporterExtensionElement.cs
- AppDomainGrammarProxy.cs
- Converter.cs
- WebHttpSecurity.cs
- ViewUtilities.cs
- Light.cs
- MatchingStyle.cs
- Light.cs
- SmiConnection.cs
- VariableAction.cs
- QilVisitor.cs
- ConstrainedGroup.cs
- CompositeDataBoundControl.cs
- SafeSecurityHandles.cs
- SafeNativeMethodsMilCoreApi.cs
- MsmqProcessProtocolHandler.cs
- Pen.cs
- ExternalDataExchangeService.cs
- CallbackHandler.cs
- EntitySqlQueryState.cs
- DataKeyCollection.cs
- SmtpLoginAuthenticationModule.cs
- XPathDocumentBuilder.cs
- HtmlInputFile.cs
- VisualStateManager.cs
- HyperLinkStyle.cs
- Typeface.cs
- DrawListViewSubItemEventArgs.cs
- PersonalizationStateInfoCollection.cs
- FieldBuilder.cs
- Span.cs
- SettingsBindableAttribute.cs
- DiscardableAttribute.cs
- wmiprovider.cs
- TabControl.cs
- MemoryStream.cs
- SchemaNotation.cs
- CatalogPartChrome.cs
- NetNamedPipeBindingElement.cs
- Registry.cs
- SoapHelper.cs
- ObjectStorage.cs
- DesignerDataConnection.cs
- WCFModelStrings.Designer.cs
- Errors.cs
- HiddenFieldPageStatePersister.cs
- BindableAttribute.cs
- EntityViewGenerator.cs
- XmlSchemaComplexContentExtension.cs
- DataGridViewCellEventArgs.cs