Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Data / Microsoft / SqlServer / Server / SmiConnection.cs / 1305376 / SmiConnection.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //[....] //----------------------------------------------------------------------------- namespace Microsoft.SqlServer.Server { using System; using System.Data; internal abstract class SmiConnection : IDisposable { // // Miscellaneous directives / accessors // internal abstract string GetCurrentDatabase( SmiEventSink eventSink ); internal abstract void SetCurrentDatabase ( string databaseName, SmiEventSink eventSink ); // // IDisposable // public virtual void Dispose( ) { // Obsoleting from SMI -- use Close( SmiEventSink ) instead. // Intended to be removed (along with inheriting IDisposable) prior to RTM. // Implement body with throw because there are only a couple of ways to get to this code: // 1) Client is calling this method even though the server negotiated for V3+ and dropped support for V2-. // 2) Server didn't implement V2- on some interface and negotiated V2-. System.Data.Common.ADP.InternalError( System.Data.Common.ADP.InternalErrorCode.UnimplementedSMIMethod ); } public virtual void Close( SmiEventSink eventSink ) { // Adding as of V3 // Implement body with throw because there are only a couple of ways to get to this code: // 1) Client is calling this method even though the server negotiated for V2- and hasn't implemented V3 yet. // 2) Server didn't implement V3 on some interface, but negotiated V3+. System.Data.Common.ADP.InternalError( System.Data.Common.ADP.InternalErrorCode.UnimplementedSMIMethod ); } // // Transaction API (should we encapsulate in it's own class or interface?) // internal abstract void BeginTransaction ( string name, IsolationLevel level, SmiEventSink eventSink ); internal abstract void CommitTransaction ( long transactionId, SmiEventSink eventSink ); internal abstract void CreateTransactionSavePoint ( long transactionId, string name, SmiEventSink eventSink ); internal abstract byte[] GetDTCAddress( // better buffer management needed? I.e. non-allocating call needed/possible? SmiEventSink eventSink ); internal abstract void EnlistTransaction ( byte[] token, // better buffer management needed? I.e. non-allocating call needed/possible? SmiEventSink eventSink ); internal abstract byte[] PromoteTransaction ( // better buffer management needed? I.e. non-allocating call needed/possible? long transactionId, SmiEventSink eventSink ); internal abstract void RollbackTransaction ( long transactionId, string savePointName, // only roll back to save point if name non-null SmiEventSink eventSink ); } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
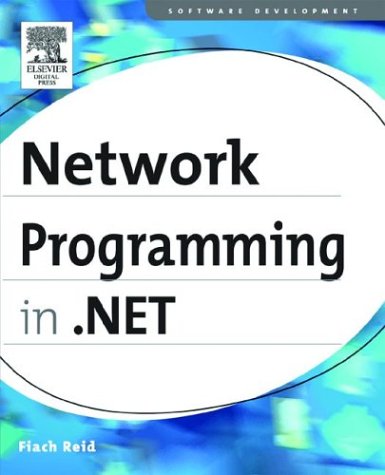
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- RestClientProxyHandler.cs
- ByValueEqualityComparer.cs
- CodeDelegateInvokeExpression.cs
- odbcmetadatafactory.cs
- PenThreadWorker.cs
- OracleDateTime.cs
- DataGridViewCheckBoxColumn.cs
- CustomErrorsSectionWrapper.cs
- DateTimePicker.cs
- Event.cs
- DbInsertCommandTree.cs
- XmlDataSourceView.cs
- HandleScope.cs
- SchemaImporterExtensionElementCollection.cs
- FixedSchema.cs
- AccessedThroughPropertyAttribute.cs
- Binding.cs
- metadatamappinghashervisitor.cs
- StrokeRenderer.cs
- GridViewAutoFormat.cs
- SessionStateItemCollection.cs
- TerminateDesigner.cs
- GetWinFXPath.cs
- OrderByBuilder.cs
- ButtonStandardAdapter.cs
- FtpWebRequest.cs
- BamlBinaryWriter.cs
- KeyInterop.cs
- RegexCompilationInfo.cs
- OutputWindow.cs
- RegexStringValidator.cs
- ExpressionQuoter.cs
- _SecureChannel.cs
- _RegBlobWebProxyDataBuilder.cs
- AccessDataSourceView.cs
- ShellProvider.cs
- ZipIOModeEnforcingStream.cs
- InputGestureCollection.cs
- ComEventsMethod.cs
- InfoCardRSAPKCS1KeyExchangeFormatter.cs
- VariantWrapper.cs
- FormClosingEvent.cs
- WorkflowTransactionOptions.cs
- Directory.cs
- RevocationPoint.cs
- XmlObjectSerializerWriteContext.cs
- Permission.cs
- ClientFormsIdentity.cs
- PathGeometry.cs
- BindingNavigator.cs
- DiagnosticTraceRecords.cs
- NavigatingCancelEventArgs.cs
- SchemaElementLookUpTableEnumerator.cs
- SoapFaultCodes.cs
- VariableAction.cs
- ByteViewer.cs
- XmlSchemaIdentityConstraint.cs
- RealizationDrawingContextWalker.cs
- wmiprovider.cs
- Directory.cs
- OdbcEnvironment.cs
- ScriptServiceAttribute.cs
- TreeBuilderBamlTranslator.cs
- XComponentModel.cs
- WindowsGraphicsWrapper.cs
- UnsafeNetInfoNativeMethods.cs
- SetIndexBinder.cs
- GlobalItem.cs
- DataGridViewCheckBoxColumn.cs
- GridEntryCollection.cs
- ExpressionBuilderContext.cs
- DataGridViewImageColumn.cs
- PropertyFilter.cs
- RevocationPoint.cs
- LinkUtilities.cs
- RestHandler.cs
- fixedPageContentExtractor.cs
- DataKeyCollection.cs
- Missing.cs
- SqlClientFactory.cs
- GridViewSortEventArgs.cs
- ItemsControl.cs
- StreamWriter.cs
- DataGridCellsPresenter.cs
- OleDbParameterCollection.cs
- MULTI_QI.cs
- XmlAutoDetectWriter.cs
- FixedSOMGroup.cs
- _Semaphore.cs
- TextElement.cs
- securitycriticaldata.cs
- XPathDocument.cs
- StringDictionaryCodeDomSerializer.cs
- GenericPrincipal.cs
- ToolStripAdornerWindowService.cs
- ThumbAutomationPeer.cs
- ForeignConstraint.cs
- CodeDirectoryCompiler.cs
- DataDesignUtil.cs
- FocusChangedEventArgs.cs