Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / xsp / System / Web / Util / SystemInfo.cs / 1 / SystemInfo.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Util { internal static class SystemInfo { static int _trueNumberOfProcessors; static internal int GetNumProcessCPUs() { if (_trueNumberOfProcessors == 0) { UnsafeNativeMethods.SYSTEM_INFO si; UnsafeNativeMethods.GetSystemInfo(out si); if (si.dwNumberOfProcessors == 1) { _trueNumberOfProcessors = 1; } else { // KERNEL32.DLL:GetCurrentProcess() always returns -1 under NT // Note: not really a handle (no need to CloseHandle()) IntPtr processHandle = UnsafeNativeMethods.INVALID_HANDLE_VALUE; IntPtr processAffinityMask; IntPtr systemAffinityMask; int returnCode = UnsafeNativeMethods.GetProcessAffinityMask( processHandle, out processAffinityMask, out systemAffinityMask); if (returnCode == 0) { _trueNumberOfProcessors = 1; } else { // if cpu affinity is set to a single processor busy waiting is a waste of time int numProcessors = 0; if (IntPtr.Size == 4) { uint mask = (uint) processAffinityMask; for (; mask != 0; mask >>= 1) { if ((mask & 1) == 1) { ++numProcessors; } } } else { ulong mask = (ulong) processAffinityMask; for (; mask != 0; mask >>= 1) { if ((mask & 1) == 1) { ++numProcessors; } } } _trueNumberOfProcessors = numProcessors; } } } Debug.Assert(_trueNumberOfProcessors > 0); return _trueNumberOfProcessors; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Util { internal static class SystemInfo { static int _trueNumberOfProcessors; static internal int GetNumProcessCPUs() { if (_trueNumberOfProcessors == 0) { UnsafeNativeMethods.SYSTEM_INFO si; UnsafeNativeMethods.GetSystemInfo(out si); if (si.dwNumberOfProcessors == 1) { _trueNumberOfProcessors = 1; } else { // KERNEL32.DLL:GetCurrentProcess() always returns -1 under NT // Note: not really a handle (no need to CloseHandle()) IntPtr processHandle = UnsafeNativeMethods.INVALID_HANDLE_VALUE; IntPtr processAffinityMask; IntPtr systemAffinityMask; int returnCode = UnsafeNativeMethods.GetProcessAffinityMask( processHandle, out processAffinityMask, out systemAffinityMask); if (returnCode == 0) { _trueNumberOfProcessors = 1; } else { // if cpu affinity is set to a single processor busy waiting is a waste of time int numProcessors = 0; if (IntPtr.Size == 4) { uint mask = (uint) processAffinityMask; for (; mask != 0; mask >>= 1) { if ((mask & 1) == 1) { ++numProcessors; } } } else { ulong mask = (ulong) processAffinityMask; for (; mask != 0; mask >>= 1) { if ((mask & 1) == 1) { ++numProcessors; } } } _trueNumberOfProcessors = numProcessors; } } } Debug.Assert(_trueNumberOfProcessors > 0); return _trueNumberOfProcessors; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
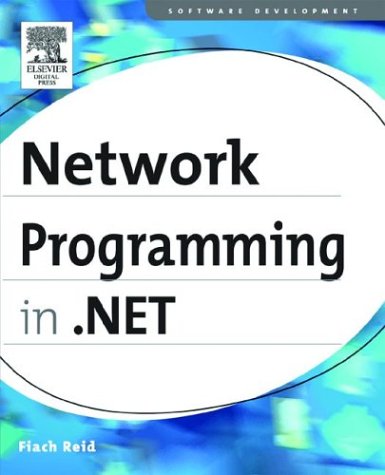
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Base64Stream.cs
- HtmlInputHidden.cs
- RuleConditionDialog.cs
- CustomAttributeBuilder.cs
- CodeGenerator.cs
- ServicePointManager.cs
- BuiltInExpr.cs
- AuthenticationSchemesHelper.cs
- x509utils.cs
- RequestSecurityToken.cs
- AnonymousIdentificationSection.cs
- FlowDocumentReaderAutomationPeer.cs
- OrderedDictionaryStateHelper.cs
- DateTimeUtil.cs
- GlyphRunDrawing.cs
- PolygonHotSpot.cs
- DataDocumentXPathNavigator.cs
- UrlPath.cs
- SelectionGlyphBase.cs
- Types.cs
- AnonymousIdentificationSection.cs
- Scanner.cs
- PhysicalAddress.cs
- Dictionary.cs
- SecurityUtils.cs
- PopupControlService.cs
- ObjectDataSourceWizardForm.cs
- SchemaSetCompiler.cs
- ColorMap.cs
- BamlLocalizabilityResolver.cs
- DependencyObjectPropertyDescriptor.cs
- Range.cs
- BufferedGraphicsContext.cs
- BindingList.cs
- PathFigure.cs
- KnownIds.cs
- AssemblyAttributes.cs
- CompressedStack.cs
- Baml2006Reader.cs
- TypeLibConverter.cs
- GCHandleCookieTable.cs
- ColorBlend.cs
- ClientBuildManager.cs
- ToolboxBitmapAttribute.cs
- ExpandoObject.cs
- WorkflowMessageEventArgs.cs
- Restrictions.cs
- Triplet.cs
- IgnoreDeviceFilterElement.cs
- OdbcDataReader.cs
- ExpressionParser.cs
- UpdatePanel.cs
- Lock.cs
- BooleanProjectedSlot.cs
- QilChoice.cs
- HtmlControl.cs
- SHA1Managed.cs
- PathSegmentCollection.cs
- XamlSerializerUtil.cs
- RC2CryptoServiceProvider.cs
- X509LogoTypeExtension.cs
- CqlQuery.cs
- StringFreezingAttribute.cs
- DataSet.cs
- CheckBox.cs
- MouseBinding.cs
- MatrixValueSerializer.cs
- LineBreak.cs
- ThumbAutomationPeer.cs
- XmlRawWriter.cs
- SegmentInfo.cs
- ZipPackage.cs
- DirectionalLight.cs
- DataProtection.cs
- SourceElementsCollection.cs
- UserNamePasswordValidator.cs
- DataGridViewCellPaintingEventArgs.cs
- SelectedCellsChangedEventArgs.cs
- ToolStripDropDownMenu.cs
- MimeObjectFactory.cs
- WizardStepBase.cs
- FlowDocumentReaderAutomationPeer.cs
- LightweightCodeGenerator.cs
- SortFieldComparer.cs
- ConfigurationException.cs
- XamlVector3DCollectionSerializer.cs
- DrawingGroup.cs
- SHA512Managed.cs
- AmbiguousMatchException.cs
- DependencyObject.cs
- ExpressionParser.cs
- FacetValueContainer.cs
- WeakReadOnlyCollection.cs
- SplashScreen.cs
- PersonalizationProviderHelper.cs
- SystemUdpStatistics.cs
- TemplateControlBuildProvider.cs
- SafeArrayRankMismatchException.cs
- TextDecorations.cs
- IconBitmapDecoder.cs