Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / ndp / fx / src / DataEntity / System / Data / Metadata / CacheForPrimitiveTypes.cs / 1 / CacheForPrimitiveTypes.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....], [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Data.EntityModel; using System.Diagnostics; using System.Text; using System.Xml.Serialization; using System.Xml; using System.Xml.Schema; using System.IO; using System.Data.Common; using System.Globalization; namespace System.Data.Metadata.Edm { internal class CacheForPrimitiveTypes { #region Fields // The primitive type kind is a list of enum which the EDM model // Every specific instantiation of the model should map their // primitive types to the edm primitive types. // In this class, primitive type is to be cached // Key for the cache: primitive type kind // Value for the cache: List. A list is used because there an be multiple types mapping to the // same primitive type kind. For example, sqlserver has multiple string types. private List [] _primitiveTypeMap = new List [EdmConstants.NumPrimitiveTypes]; #endregion #region Methods /// /// Add the given primitive type to the primitive type cache /// /// The primitive type to add internal void Add(PrimitiveType type) { // Get to the list ListprimitiveTypes = EntityUtil.CheckArgumentOutOfRange(_primitiveTypeMap, (int)type.PrimitiveTypeKind, "primitiveTypeKind"); // If there isn't a list for the given model type, create one and add it if (primitiveTypes == null) { primitiveTypes = new List (); primitiveTypes.Add(type); _primitiveTypeMap[(int)type.PrimitiveTypeKind] = primitiveTypes; } else { primitiveTypes.Add(type); } } /// /// Try and get the mapped type for the given primitiveTypeKind in the given dataspace /// /// The primitive type kind of the primitive type to retrieve /// The facets to use in picking the primitive type /// The resulting type ///Whether a type was retrieved or not internal bool TryGetType(PrimitiveTypeKind primitiveTypeKind, IEnumerablefacets, out PrimitiveType type) { type = null; // Now, see if we have any types for this model type, if so, loop through to find the best matching one List primitiveTypes = EntityUtil.CheckArgumentOutOfRange(_primitiveTypeMap, (int)primitiveTypeKind, "primitiveTypeKind"); if ((null != primitiveTypes) && (0 < primitiveTypes.Count)) { if (primitiveTypes.Count == 1) { type = primitiveTypes[0]; return true; } if (facets == null) { FacetDescription[] facetDescriptions = EdmProviderManifest.GetInitialFacetDescriptions(primitiveTypeKind); if (facetDescriptions == null) { type = primitiveTypes[0]; return true; } Debug.Assert(facetDescriptions.Length > 0); facets = CacheForPrimitiveTypes.CreateInitialFacets(facetDescriptions); } Debug.Assert(type == null, "type must be null here"); bool isMaxLengthSentinel = false; // Create a dictionary of facets for easy lookup foreach (Facet facet in facets) { if ((primitiveTypeKind == PrimitiveTypeKind.String || primitiveTypeKind == PrimitiveTypeKind.Binary) && facet.Value != null && facet.Name == EdmProviderManifest.MaxLengthFacetName && Helper.IsUnboundedFacetValue(facet)) { // MaxLength has the sentinel value. So this facet need not be added. isMaxLengthSentinel = true; continue; } } int maxLength = 0; // Find a primitive type with the matching constraint foreach (PrimitiveType primitiveType in primitiveTypes) { if (isMaxLengthSentinel) { if (type == null) { type = primitiveType; maxLength = Helper.GetFacet(primitiveType.FacetDescriptions, EdmProviderManifest.MaxLengthFacetName).MaxValue.Value; } else { int newMaxLength = Helper.GetFacet(primitiveType.FacetDescriptions, EdmProviderManifest.MaxLengthFacetName).MaxValue.Value; if (newMaxLength > maxLength) { type = primitiveType; maxLength = newMaxLength; } } } else { type = primitiveType; break; } } Debug.Assert(type != null); return true; } return false; } private static Facet[] CreateInitialFacets(FacetDescription[] facetDescriptions) { Debug.Assert(facetDescriptions != null && facetDescriptions.Length > 0); Facet[] facets = new Facet[facetDescriptions.Length]; for (int i = 0; i < facetDescriptions.Length; ++i) { switch (facetDescriptions[i].FacetName) { case DbProviderManifest.MaxLengthFacetName: facets[i] = Facet.Create(facetDescriptions[i], TypeUsage.DefaultMaxLengthFacetValue); break; case DbProviderManifest.UnicodeFacetName: facets[i] = Facet.Create(facetDescriptions[i], TypeUsage.DefaultUnicodeFacetValue); break; case DbProviderManifest.FixedLengthFacetName: facets[i] = Facet.Create(facetDescriptions[i], TypeUsage.DefaultFixedLengthFacetValue); break; case DbProviderManifest.PrecisionFacetName: facets[i] = Facet.Create(facetDescriptions[i], TypeUsage.DefaultPrecisionFacetValue); break; case DbProviderManifest.ScaleFacetName: facets[i] = Facet.Create(facetDescriptions[i], TypeUsage.DefaultScaleFacetValue); break; default: Debug.Assert(false, "Unexpected facet"); break; } } return facets; } /// /// Get the list of the primitive types for the given dataspace /// ///internal System.Collections.ObjectModel.ReadOnlyCollection GetTypes() { List primitiveTypes = new List (); foreach (List types in _primitiveTypeMap) { if (null != types) { primitiveTypes.AddRange(types); } } return primitiveTypes.AsReadOnly(); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....], [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Data.EntityModel; using System.Diagnostics; using System.Text; using System.Xml.Serialization; using System.Xml; using System.Xml.Schema; using System.IO; using System.Data.Common; using System.Globalization; namespace System.Data.Metadata.Edm { internal class CacheForPrimitiveTypes { #region Fields // The primitive type kind is a list of enum which the EDM model // Every specific instantiation of the model should map their // primitive types to the edm primitive types. // In this class, primitive type is to be cached // Key for the cache: primitive type kind // Value for the cache: List. A list is used because there an be multiple types mapping to the // same primitive type kind. For example, sqlserver has multiple string types. private List [] _primitiveTypeMap = new List [EdmConstants.NumPrimitiveTypes]; #endregion #region Methods /// /// Add the given primitive type to the primitive type cache /// /// The primitive type to add internal void Add(PrimitiveType type) { // Get to the list ListprimitiveTypes = EntityUtil.CheckArgumentOutOfRange(_primitiveTypeMap, (int)type.PrimitiveTypeKind, "primitiveTypeKind"); // If there isn't a list for the given model type, create one and add it if (primitiveTypes == null) { primitiveTypes = new List (); primitiveTypes.Add(type); _primitiveTypeMap[(int)type.PrimitiveTypeKind] = primitiveTypes; } else { primitiveTypes.Add(type); } } /// /// Try and get the mapped type for the given primitiveTypeKind in the given dataspace /// /// The primitive type kind of the primitive type to retrieve /// The facets to use in picking the primitive type /// The resulting type ///Whether a type was retrieved or not internal bool TryGetType(PrimitiveTypeKind primitiveTypeKind, IEnumerablefacets, out PrimitiveType type) { type = null; // Now, see if we have any types for this model type, if so, loop through to find the best matching one List primitiveTypes = EntityUtil.CheckArgumentOutOfRange(_primitiveTypeMap, (int)primitiveTypeKind, "primitiveTypeKind"); if ((null != primitiveTypes) && (0 < primitiveTypes.Count)) { if (primitiveTypes.Count == 1) { type = primitiveTypes[0]; return true; } if (facets == null) { FacetDescription[] facetDescriptions = EdmProviderManifest.GetInitialFacetDescriptions(primitiveTypeKind); if (facetDescriptions == null) { type = primitiveTypes[0]; return true; } Debug.Assert(facetDescriptions.Length > 0); facets = CacheForPrimitiveTypes.CreateInitialFacets(facetDescriptions); } Debug.Assert(type == null, "type must be null here"); bool isMaxLengthSentinel = false; // Create a dictionary of facets for easy lookup foreach (Facet facet in facets) { if ((primitiveTypeKind == PrimitiveTypeKind.String || primitiveTypeKind == PrimitiveTypeKind.Binary) && facet.Value != null && facet.Name == EdmProviderManifest.MaxLengthFacetName && Helper.IsUnboundedFacetValue(facet)) { // MaxLength has the sentinel value. So this facet need not be added. isMaxLengthSentinel = true; continue; } } int maxLength = 0; // Find a primitive type with the matching constraint foreach (PrimitiveType primitiveType in primitiveTypes) { if (isMaxLengthSentinel) { if (type == null) { type = primitiveType; maxLength = Helper.GetFacet(primitiveType.FacetDescriptions, EdmProviderManifest.MaxLengthFacetName).MaxValue.Value; } else { int newMaxLength = Helper.GetFacet(primitiveType.FacetDescriptions, EdmProviderManifest.MaxLengthFacetName).MaxValue.Value; if (newMaxLength > maxLength) { type = primitiveType; maxLength = newMaxLength; } } } else { type = primitiveType; break; } } Debug.Assert(type != null); return true; } return false; } private static Facet[] CreateInitialFacets(FacetDescription[] facetDescriptions) { Debug.Assert(facetDescriptions != null && facetDescriptions.Length > 0); Facet[] facets = new Facet[facetDescriptions.Length]; for (int i = 0; i < facetDescriptions.Length; ++i) { switch (facetDescriptions[i].FacetName) { case DbProviderManifest.MaxLengthFacetName: facets[i] = Facet.Create(facetDescriptions[i], TypeUsage.DefaultMaxLengthFacetValue); break; case DbProviderManifest.UnicodeFacetName: facets[i] = Facet.Create(facetDescriptions[i], TypeUsage.DefaultUnicodeFacetValue); break; case DbProviderManifest.FixedLengthFacetName: facets[i] = Facet.Create(facetDescriptions[i], TypeUsage.DefaultFixedLengthFacetValue); break; case DbProviderManifest.PrecisionFacetName: facets[i] = Facet.Create(facetDescriptions[i], TypeUsage.DefaultPrecisionFacetValue); break; case DbProviderManifest.ScaleFacetName: facets[i] = Facet.Create(facetDescriptions[i], TypeUsage.DefaultScaleFacetValue); break; default: Debug.Assert(false, "Unexpected facet"); break; } } return facets; } /// /// Get the list of the primitive types for the given dataspace /// ///internal System.Collections.ObjectModel.ReadOnlyCollection GetTypes() { List primitiveTypes = new List (); foreach (List types in _primitiveTypeMap) { if (null != types) { primitiveTypes.AddRange(types); } } return primitiveTypes.AsReadOnly(); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
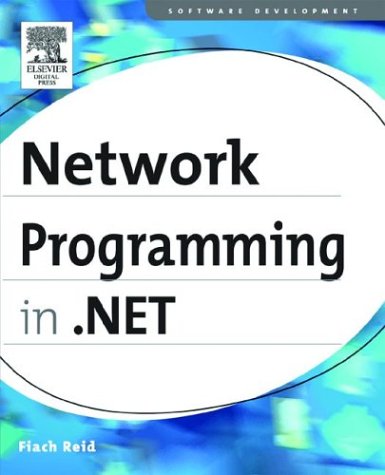
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- FormViewDeleteEventArgs.cs
- CollectionBuilder.cs
- TreeWalkHelper.cs
- SystemFonts.cs
- CounterCreationDataCollection.cs
- HostingPreferredMapPath.cs
- GeneratedView.cs
- VisemeEventArgs.cs
- DiffuseMaterial.cs
- ToolStripOverflowButton.cs
- DataRowIndexBuffer.cs
- selecteditemcollection.cs
- GradientBrush.cs
- WebPartTransformerAttribute.cs
- TabletDevice.cs
- ActivationProxy.cs
- CollectionView.cs
- UidManager.cs
- EpmAttributeNameBuilder.cs
- CustomErrorsSection.cs
- ObjectConverter.cs
- ProcessManager.cs
- CollectionViewGroup.cs
- controlskin.cs
- IpcChannelHelper.cs
- TagPrefixCollection.cs
- CreateUserWizardAutoFormat.cs
- CodeLinePragma.cs
- File.cs
- CodeMemberEvent.cs
- ExpressionBuilder.cs
- ContainsRowNumberChecker.cs
- SapiGrammar.cs
- nulltextnavigator.cs
- ToolboxDataAttribute.cs
- AttributeData.cs
- FirstQueryOperator.cs
- BindingMAnagerBase.cs
- OptimizedTemplateContentHelper.cs
- ObjectListDataBindEventArgs.cs
- CombinedGeometry.cs
- XmlText.cs
- SqlDependencyListener.cs
- WebRequestModuleElement.cs
- SafeCertificateContext.cs
- VarInfo.cs
- ProviderBase.cs
- recordstatescratchpad.cs
- EventLogTraceListener.cs
- PassportIdentity.cs
- WindowsFormsDesignerOptionService.cs
- XmlSchemaImporter.cs
- Directory.cs
- WinFormsSpinner.cs
- FileEnumerator.cs
- AsymmetricCryptoHandle.cs
- Component.cs
- Freezable.cs
- ToggleButton.cs
- XPathExpr.cs
- TransformConverter.cs
- LinqDataSourceDisposeEventArgs.cs
- TextModifier.cs
- StyleSheetRefUrlEditor.cs
- SplitterEvent.cs
- XmlBindingWorker.cs
- DataChangedEventManager.cs
- SourceFileInfo.cs
- SqlDataSourceDesigner.cs
- TrackBarRenderer.cs
- ComplexBindingPropertiesAttribute.cs
- DateBoldEvent.cs
- ImageListStreamer.cs
- DataBindingExpressionBuilder.cs
- GroupStyle.cs
- OutOfMemoryException.cs
- PropertyReferenceExtension.cs
- FontStretch.cs
- TextTreeFixupNode.cs
- EdgeProfileValidation.cs
- Pair.cs
- CachedBitmap.cs
- mansign.cs
- InputChannel.cs
- ProjectionRewriter.cs
- ClientRoleProvider.cs
- SqlFunctionAttribute.cs
- PermissionToken.cs
- Single.cs
- IPGlobalProperties.cs
- ApplicationDirectory.cs
- NavigationWindowAutomationPeer.cs
- StorageEntitySetMapping.cs
- SignedXml.cs
- KeyToListMap.cs
- PartitionResolver.cs
- SamlAuthorizationDecisionStatement.cs
- mediaeventargs.cs
- WebPartConnection.cs
- ISAPIRuntime.cs