Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / ndp / fx / src / DataEntity / System / Data / Common / Utils / KeyToListMap.cs / 1 / KeyToListMap.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.Diagnostics; using System.Text; using System.Collections.ObjectModel; namespace System.Data.Common.Utils { // This class contains an abstraction that map a key of type TKey to a // list of values (of type TValue). This is really a convenience abstraction internal class KeyToListMap: InternalBase { #region Constructors // effects: Creates an empty map with keys compared using comparer internal KeyToListMap(IEqualityComparer comparer) { Debug.Assert(null != comparer); m_map = new Dictionary >(comparer); } #endregion #region Fields // Just a regular dictionary private Dictionary > m_map; #endregion #region Properties // effects: Yields all the keys in this internal IEnumerable Keys { get { return m_map.Keys; } } // effects: Returns all the values for all keys with all the values // of a particular key adjacent to each other internal IEnumerable AllValues { get { foreach (TKey key in Keys) { foreach (TValue value in ListForKey(key)) { yield return value; } } } } // effects: Returns all the Dictionary Entries in this Map. internal IEnumerable >> KeyValuePairs { get { return m_map; } } #endregion #region Methods internal bool ContainsKey(TKey key) { return m_map.ContainsKey(key); } // effects: Adds to this. If the entry already exists, another one is added internal void Add(TKey key, TValue value) { // If entry for key already exists, add value to the list, else // create a new list and add the value to it List valueList; if (!m_map.TryGetValue(key, out valueList)) { valueList = new List (); m_map[key] = valueList; } valueList.Add(value); } // effects: Adds for each value in values to this. If the entry already exists, another one is added internal void AddRange(TKey key, IEnumerable values) { foreach (TValue value in values) { Add(key, value); } } // effects: Removes all entries corresponding to key // Returns true iff the key was removed internal bool RemoveKey(TKey key) { return m_map.Remove(key); } // requires: key exist in this // effects: Returns the values associated with key internal System.Collections.ObjectModel.ReadOnlyCollection ListForKey(TKey key) { Debug.Assert(m_map.ContainsKey(key), "key not registered in map"); return new System.Collections.ObjectModel.ReadOnlyCollection (m_map[key]); } // effects: Returns true if the key exists and false if not. // In case the Key exists, the out parameter is assigned the List for that key, // otherwise it is assigned a null value internal bool TryGetListForKey(TKey key, out System.Collections.ObjectModel.ReadOnlyCollection valueCollection) { List list; valueCollection = null; if (m_map.TryGetValue(key, out list)) { valueCollection = new System.Collections.ObjectModel.ReadOnlyCollection (list); return true; } return false; } // Returns all values for the given key. If no values have been added for the key, // yields no values. internal IEnumerable EnumerateValues(TKey key) { List values; if (m_map.TryGetValue(key, out values)) { foreach (TValue value in values) { yield return value; } } } internal override void ToCompactString(StringBuilder builder) { foreach (TKey key in Keys) { // Calling key's ToString here StringUtil.FormatStringBuilder(builder, "{0}", key); builder.Append(": "); IEnumerable values = ListForKey(key); StringUtil.ToSeparatedString(builder, values, ",", "null"); builder.Append("; "); } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.Diagnostics; using System.Text; using System.Collections.ObjectModel; namespace System.Data.Common.Utils { // This class contains an abstraction that map a key of type TKey to a // list of values (of type TValue). This is really a convenience abstraction internal class KeyToListMap: InternalBase { #region Constructors // effects: Creates an empty map with keys compared using comparer internal KeyToListMap(IEqualityComparer comparer) { Debug.Assert(null != comparer); m_map = new Dictionary >(comparer); } #endregion #region Fields // Just a regular dictionary private Dictionary > m_map; #endregion #region Properties // effects: Yields all the keys in this internal IEnumerable Keys { get { return m_map.Keys; } } // effects: Returns all the values for all keys with all the values // of a particular key adjacent to each other internal IEnumerable AllValues { get { foreach (TKey key in Keys) { foreach (TValue value in ListForKey(key)) { yield return value; } } } } // effects: Returns all the Dictionary Entries in this Map. internal IEnumerable >> KeyValuePairs { get { return m_map; } } #endregion #region Methods internal bool ContainsKey(TKey key) { return m_map.ContainsKey(key); } // effects: Adds to this. If the entry already exists, another one is added internal void Add(TKey key, TValue value) { // If entry for key already exists, add value to the list, else // create a new list and add the value to it List valueList; if (!m_map.TryGetValue(key, out valueList)) { valueList = new List (); m_map[key] = valueList; } valueList.Add(value); } // effects: Adds for each value in values to this. If the entry already exists, another one is added internal void AddRange(TKey key, IEnumerable values) { foreach (TValue value in values) { Add(key, value); } } // effects: Removes all entries corresponding to key // Returns true iff the key was removed internal bool RemoveKey(TKey key) { return m_map.Remove(key); } // requires: key exist in this // effects: Returns the values associated with key internal System.Collections.ObjectModel.ReadOnlyCollection ListForKey(TKey key) { Debug.Assert(m_map.ContainsKey(key), "key not registered in map"); return new System.Collections.ObjectModel.ReadOnlyCollection (m_map[key]); } // effects: Returns true if the key exists and false if not. // In case the Key exists, the out parameter is assigned the List for that key, // otherwise it is assigned a null value internal bool TryGetListForKey(TKey key, out System.Collections.ObjectModel.ReadOnlyCollection valueCollection) { List list; valueCollection = null; if (m_map.TryGetValue(key, out list)) { valueCollection = new System.Collections.ObjectModel.ReadOnlyCollection (list); return true; } return false; } // Returns all values for the given key. If no values have been added for the key, // yields no values. internal IEnumerable EnumerateValues(TKey key) { List values; if (m_map.TryGetValue(key, out values)) { foreach (TValue value in values) { yield return value; } } } internal override void ToCompactString(StringBuilder builder) { foreach (TKey key in Keys) { // Calling key's ToString here StringUtil.FormatStringBuilder(builder, "{0}", key); builder.Append(": "); IEnumerable values = ListForKey(key); StringUtil.ToSeparatedString(builder, values, ",", "null"); builder.Append("; "); } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
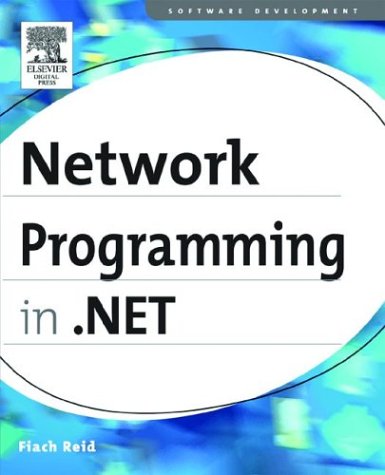
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CodeTypeReferenceExpression.cs
- ClipboardData.cs
- PersonalizablePropertyEntry.cs
- RectAnimationBase.cs
- DataRowCollection.cs
- OdbcUtils.cs
- SmiMetaDataProperty.cs
- DataSourceIDConverter.cs
- CapabilitiesState.cs
- UdpConstants.cs
- CodeSnippetTypeMember.cs
- NamedPermissionSet.cs
- HtmlInputPassword.cs
- CompositeControl.cs
- ImageFormatConverter.cs
- TcpSocketManager.cs
- VectorAnimationUsingKeyFrames.cs
- Process.cs
- DiagnosticsElement.cs
- SecurityUtils.cs
- RegistryKey.cs
- DataGridViewCellCollection.cs
- XmlJsonWriter.cs
- MethodExpression.cs
- Int16KeyFrameCollection.cs
- SimpleBitVector32.cs
- CodeDirectiveCollection.cs
- BufferedReadStream.cs
- EncodingNLS.cs
- PeerSecurityHelpers.cs
- TagPrefixInfo.cs
- OdbcConnection.cs
- AppDomainProtocolHandler.cs
- SystemGatewayIPAddressInformation.cs
- EntityReference.cs
- CheckBoxBaseAdapter.cs
- dbenumerator.cs
- XmlSchemaSimpleTypeRestriction.cs
- CallbackValidator.cs
- SqlXml.cs
- PointAnimationUsingPath.cs
- Char.cs
- TiffBitmapEncoder.cs
- WorkflowPersistenceService.cs
- TraceUtils.cs
- NullableFloatMinMaxAggregationOperator.cs
- InlineUIContainer.cs
- IndentedTextWriter.cs
- VoiceChangeEventArgs.cs
- CustomAttributeFormatException.cs
- Form.cs
- Helper.cs
- DataGridParentRows.cs
- VisualBrush.cs
- DelegatingConfigHost.cs
- RefreshEventArgs.cs
- ContractMapping.cs
- ValidationError.cs
- ListViewItem.cs
- SimpleApplicationHost.cs
- PropertyDescriptorComparer.cs
- counter.cs
- MethodToken.cs
- CommonDialog.cs
- WindowsListView.cs
- DataBindingHandlerAttribute.cs
- OperandQuery.cs
- XPathParser.cs
- Opcode.cs
- LinqDataSourceHelper.cs
- SmtpException.cs
- SystemIcmpV4Statistics.cs
- ObjectConverter.cs
- TreeNodeCollection.cs
- AttributeQuery.cs
- EntityDescriptor.cs
- Crc32.cs
- FileLevelControlBuilderAttribute.cs
- InspectionWorker.cs
- assemblycache.cs
- AlternateView.cs
- DirectoryObjectSecurity.cs
- CorePropertiesFilter.cs
- X509Utils.cs
- ResolveCriteriaApril2005.cs
- SparseMemoryStream.cs
- ProjectionPruner.cs
- FirstMatchCodeGroup.cs
- X509ChainPolicy.cs
- ScriptBehaviorDescriptor.cs
- OptimizerPatterns.cs
- SortExpressionBuilder.cs
- DataGridColumnFloatingHeader.cs
- ReferenceEqualityComparer.cs
- MatrixUtil.cs
- ZoneMembershipCondition.cs
- SqlDataSourceStatusEventArgs.cs
- PropertySegmentSerializer.cs
- PrincipalPermission.cs
- Missing.cs