Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Framework / System / Windows / Setter.cs / 1 / Setter.cs
/****************************************************************************\ * * File: Setter.cs * * TargetType property setting class. * * Copyright (C) 2004 by Microsoft Corporation. All rights reserved. * \***************************************************************************/ using System; using System.ComponentModel; using System.Windows.Markup; #pragma warning disable 1634, 1691 // suppressing PreSharp warnings namespace System.Windows { ////// TargetType property setting class. /// public class Setter : SetterBase { ////// Property Setter construction - set everything to null or DependencyProperty.UnsetValue /// public Setter() { } ////// Property Setter construction - given property and value /// public Setter( DependencyProperty property, object value ) { Initialize( property, value, null ); } ////// Property Setter construction - given property, value, and string identifier for child node. /// public Setter( DependencyProperty property, object value, string targetName ) { Initialize( property, value, targetName ); } ////// Method that does all the initialization work for the constructors. /// private void Initialize( DependencyProperty property, object value, string target ) { if( value == DependencyProperty.UnsetValue ) { throw new ArgumentException(SR.Get(SRID.SetterValueCannotBeUnset)); } CheckValidProperty(property); // No null check for target since null is a valid value. _property = property; _value = value; _target = target; } private void CheckValidProperty( DependencyProperty property) { if (property == null) { throw new ArgumentNullException("property"); } if (property.ReadOnly) { // Read-only properties will not be consulting Style/Template/Trigger Setter for value. // Rather than silently do nothing, throw error. throw new ArgumentException(SR.Get(SRID.ReadOnlyPropertyNotAllowed, property.Name, GetType().Name)); } if( property == FrameworkElement.NameProperty) { throw new InvalidOperationException(SR.Get(SRID.CannotHavePropertyInStyle, FrameworkElement.NameProperty.Name)); } } ////// Seals this setter /// internal override void Seal() { // Do the validation that can't be done until we know all of the property // values. DependencyProperty dp = Property; object value = ValueInternal; if (dp == null) { throw new ArgumentException(SR.Get(SRID.NullPropertyIllegal, "Setter.Property")); } if( String.IsNullOrEmpty(TargetName)) { // Setter on container is not allowed to affect the StyleProperty. if (dp == FrameworkElement.StyleProperty) { throw new ArgumentException(SR.Get(SRID.StylePropertyInStyleNotAllowed)); } } // Value needs to be valid for the DP, or a deferred reference, or one of the supported // markup extensions. if (!dp.IsValidValue(value)) { // The only markup extensions supported by styles is resources and bindings. if (value is MarkupExtension) { if ( !(value is DynamicResourceExtension) && !(value is System.Windows.Data.BindingBase) ) { throw new ArgumentException(SR.Get(SRID.SetterValueOfMarkupExtensionNotSupported, value.GetType().Name)); } } else if (!(value is DeferredReference)) { throw new ArgumentException(SR.Get(SRID.InvalidSetterValue, value, dp.OwnerType, dp.Name)); } } // Styling the logical tree is not supported if (StyleHelper.IsStylingLogicalTree(dp, value)) { throw new NotSupportedException(SR.Get(SRID.ModifyingLogicalTreeViaStylesNotImplemented, "Setter.Value")); } // Freeze the value for the setter StyleHelper.SealIfSealable(_value); base.Seal(); } ////// Property that is being set by this setter /// [DefaultValue(null)] [Localizability(LocalizationCategory.None, Modifiability = Modifiability.Unmodifiable, Readability = Readability.Unreadable)] // Not localizable by-default public DependencyProperty Property { get { return _property; } set { CheckValidProperty(value); CheckSealed(); _property = value; } } ////// Property value that is being set by this setter /// [System.Windows.Markup.DependsOn("Property")] [System.Windows.Markup.DependsOn("TargetName")] [Localizability(LocalizationCategory.None, Readability = Readability.Unreadable)] // Not localizable by-default public object Value { get { // Inflate the deferred reference if the _value is one of those. DeferredReference deferredReference = _value as DeferredReference; if (deferredReference != null) { _value = deferredReference.GetValue(BaseValueSourceInternal.Unknown); } return _value; } set { if( value == DependencyProperty.UnsetValue ) { throw new ArgumentException(SR.Get(SRID.SetterValueCannotBeUnset)); } CheckSealed(); // Styling the logical tree is not supported // (We don't know the dp yet, so pass null) if (StyleHelper.IsStylingLogicalTree(null /*dp*/, value)) { throw new NotSupportedException(SR.Get(SRID.ModifyingLogicalTreeViaStylesNotImplemented, value, "Setter.Value")); } // No Expression support if( value is Expression ) { throw new ArgumentException(SR.Get(SRID.StyleValueOfExpressionNotSupported)); } _value = value; } } ////// Internal property used so that we obtain the value as /// is without having to inflate the DeferredReference. /// internal object ValueInternal { get { return _value; } } ////// When the set is directed at a child node, this string /// identifies the intended target child node. /// [DefaultValue(null)] public string TargetName { get { return _target; } set { // Setting to null is allowed, to clear out value. CheckSealed(); _target = value; } } private DependencyProperty _property = null; private object _value = DependencyProperty.UnsetValue; private string _target = null; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
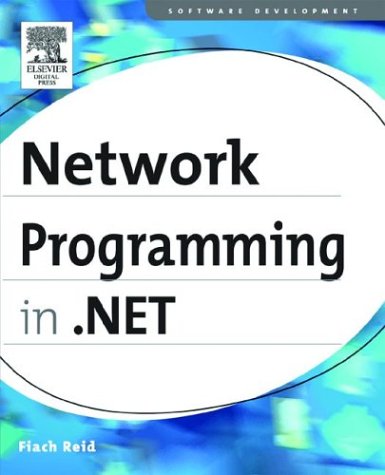
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- FixedDocumentSequencePaginator.cs
- UnsafeNativeMethods.cs
- Point.cs
- DBAsyncResult.cs
- KeyboardDevice.cs
- ViewRendering.cs
- XPathDocumentNavigator.cs
- Interlocked.cs
- FileDialog.cs
- LayoutEditorPart.cs
- BCryptNative.cs
- XmlCustomFormatter.cs
- NonBatchDirectoryCompiler.cs
- BevelBitmapEffect.cs
- TextTreeUndoUnit.cs
- XMLUtil.cs
- XmlElement.cs
- MetadataCollection.cs
- StandardBindingOptionalReliableSessionElement.cs
- EmptyEnumerable.cs
- MatrixAnimationUsingKeyFrames.cs
- Int16.cs
- DefaultValidator.cs
- SAPICategories.cs
- DoubleCollectionValueSerializer.cs
- TemplateAction.cs
- TwoPhaseCommitProxy.cs
- TypeDescriptorFilterService.cs
- ExpandCollapseProviderWrapper.cs
- Positioning.cs
- WorkItem.cs
- TimerTable.cs
- FastPropertyAccessor.cs
- WinFormsSecurity.cs
- ThemeableAttribute.cs
- CacheOutputQuery.cs
- XmlKeywords.cs
- sqlnorm.cs
- PackageDigitalSignatureManager.cs
- FragmentQuery.cs
- Metadata.cs
- WindowsRegion.cs
- ConfigurationCollectionAttribute.cs
- DataControlPagerLinkButton.cs
- CommonDialog.cs
- CancellationTokenRegistration.cs
- TextEditorTyping.cs
- DataBoundControl.cs
- WindowsScroll.cs
- HtmlProps.cs
- Deflater.cs
- SafeIUnknown.cs
- TimeEnumHelper.cs
- FlowDocumentPageViewerAutomationPeer.cs
- TemplateManager.cs
- CapabilitiesSection.cs
- FixedSOMTableCell.cs
- HttpListenerRequestTraceRecord.cs
- ComponentTray.cs
- WorkflowTerminatedException.cs
- ConsumerConnectionPointCollection.cs
- DescendantQuery.cs
- WinFormsSpinner.cs
- SqlUserDefinedAggregateAttribute.cs
- ComboBox.cs
- DateTimeOffset.cs
- AbsoluteQuery.cs
- FusionWrap.cs
- MatchAllMessageFilter.cs
- ZipIOExtraField.cs
- _NegotiateClient.cs
- AvTraceDetails.cs
- UnsettableComboBox.cs
- ResourceDictionary.cs
- DesignerDataSourceView.cs
- HttpChannelBindingToken.cs
- NodeFunctions.cs
- RegistrationContext.cs
- Journal.cs
- LiteralLink.cs
- CodeMethodInvokeExpression.cs
- SQLConvert.cs
- WSSecurityJan2004.cs
- CompilationSection.cs
- SrgsNameValueTag.cs
- ConfigXmlComment.cs
- ModelTypeConverter.cs
- SlotInfo.cs
- CommandValueSerializer.cs
- View.cs
- OutOfMemoryException.cs
- TcpStreams.cs
- GlobalProxySelection.cs
- ExtensionQuery.cs
- ZipIOExtraFieldZip64Element.cs
- SpStreamWrapper.cs
- IteratorFilter.cs
- ProtocolReflector.cs
- CodeMemberEvent.cs
- TextEditor.cs