Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Data / System / Data / OleDb / OleDbPermission.cs / 1305376 / OleDbPermission.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //[....] //----------------------------------------------------------------------------- namespace System.Data.OleDb { using System.Collections; using System.Collections.Generic; using System.Data.Common; using System.Globalization; using System.Runtime.Serialization; using System.Security; using System.Security.Permissions; [Serializable] public sealed class OleDbPermission : DBDataPermission { private String[] _providerRestriction; // should never be string[0] private String _providers; [ Obsolete("OleDbPermission() has been deprecated. Use the OleDbPermission(PermissionState.None) constructor. http://go.microsoft.com/fwlink/?linkid=14202", true) ] // MDAC 86034 public OleDbPermission() : this(PermissionState.None) { } public OleDbPermission(PermissionState state) : base(state) { } [ Obsolete("OleDbPermission(PermissionState state, Boolean allowBlankPassword) has been deprecated. Use the OleDbPermission(PermissionState.None) constructor. http://go.microsoft.com/fwlink/?linkid=14202", true) ] // MDAC 86034 public OleDbPermission(PermissionState state, bool allowBlankPassword) : this(state) { AllowBlankPassword = allowBlankPassword; } private OleDbPermission(OleDbPermission permission) : base(permission) { // for Copy } internal OleDbPermission(OleDbPermissionAttribute permissionAttribute) : base(permissionAttribute) { // for CreatePermission } internal OleDbPermission(OleDbConnectionString constr) : base(constr) { // for Open if ((null == constr) || constr.IsEmpty) { base.Add(ADP.StrEmpty, ADP.StrEmpty, KeyRestrictionBehavior.AllowOnly); } } [System.ComponentModel.Browsable(false)] [System.ComponentModel.EditorBrowsableAttribute(System.ComponentModel.EditorBrowsableState.Never)] [Obsolete("Provider property has been deprecated. Use the Add method. http://go.microsoft.com/fwlink/?linkid=14202")] public string Provider { get { string providers = _providers; // MDAC 83103 if (null == providers) { string[] restrictions = _providerRestriction; if (null != restrictions) { if (0 < restrictions.Length) { providers = restrictions[0]; for (int i = 1; i < restrictions.Length; ++i) { providers += ";" + restrictions[i]; } } } } return ((null != providers) ? providers : ADP.StrEmpty); } set { // MDAC 61263 string[] restrictions = null; if (!ADP.IsEmpty(value)) { restrictions = value.Split(new char[1] { ';' }); restrictions = DBConnectionString.RemoveDuplicates(restrictions); } _providerRestriction = restrictions; _providers = value; } } override public IPermission Copy () { return new OleDbPermission(this); } } [AttributeUsage(AttributeTargets.Method | AttributeTargets.Constructor | AttributeTargets.Class | AttributeTargets.Struct | AttributeTargets.Assembly, AllowMultiple = true, Inherited = false )] [Serializable] public sealed class OleDbPermissionAttribute : DBDataPermissionAttribute { private String _providers; public OleDbPermissionAttribute(SecurityAction action) : base(action) { } [System.ComponentModel.Browsable(false)] [System.ComponentModel.EditorBrowsableAttribute(System.ComponentModel.EditorBrowsableState.Never)] [Obsolete("Provider property has been deprecated. Use the Add method. http://go.microsoft.com/fwlink/?linkid=14202")] public String Provider { get { string providers = _providers; return ((null != providers) ? providers : ADP.StrEmpty); } set { _providers = value; } } override public IPermission CreatePermission() { return new OleDbPermission(this); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //[....] //----------------------------------------------------------------------------- namespace System.Data.OleDb { using System.Collections; using System.Collections.Generic; using System.Data.Common; using System.Globalization; using System.Runtime.Serialization; using System.Security; using System.Security.Permissions; [Serializable] public sealed class OleDbPermission : DBDataPermission { private String[] _providerRestriction; // should never be string[0] private String _providers; [ Obsolete("OleDbPermission() has been deprecated. Use the OleDbPermission(PermissionState.None) constructor. http://go.microsoft.com/fwlink/?linkid=14202", true) ] // MDAC 86034 public OleDbPermission() : this(PermissionState.None) { } public OleDbPermission(PermissionState state) : base(state) { } [ Obsolete("OleDbPermission(PermissionState state, Boolean allowBlankPassword) has been deprecated. Use the OleDbPermission(PermissionState.None) constructor. http://go.microsoft.com/fwlink/?linkid=14202", true) ] // MDAC 86034 public OleDbPermission(PermissionState state, bool allowBlankPassword) : this(state) { AllowBlankPassword = allowBlankPassword; } private OleDbPermission(OleDbPermission permission) : base(permission) { // for Copy } internal OleDbPermission(OleDbPermissionAttribute permissionAttribute) : base(permissionAttribute) { // for CreatePermission } internal OleDbPermission(OleDbConnectionString constr) : base(constr) { // for Open if ((null == constr) || constr.IsEmpty) { base.Add(ADP.StrEmpty, ADP.StrEmpty, KeyRestrictionBehavior.AllowOnly); } } [System.ComponentModel.Browsable(false)] [System.ComponentModel.EditorBrowsableAttribute(System.ComponentModel.EditorBrowsableState.Never)] [Obsolete("Provider property has been deprecated. Use the Add method. http://go.microsoft.com/fwlink/?linkid=14202")] public string Provider { get { string providers = _providers; // MDAC 83103 if (null == providers) { string[] restrictions = _providerRestriction; if (null != restrictions) { if (0 < restrictions.Length) { providers = restrictions[0]; for (int i = 1; i < restrictions.Length; ++i) { providers += ";" + restrictions[i]; } } } } return ((null != providers) ? providers : ADP.StrEmpty); } set { // MDAC 61263 string[] restrictions = null; if (!ADP.IsEmpty(value)) { restrictions = value.Split(new char[1] { ';' }); restrictions = DBConnectionString.RemoveDuplicates(restrictions); } _providerRestriction = restrictions; _providers = value; } } override public IPermission Copy () { return new OleDbPermission(this); } } [AttributeUsage(AttributeTargets.Method | AttributeTargets.Constructor | AttributeTargets.Class | AttributeTargets.Struct | AttributeTargets.Assembly, AllowMultiple = true, Inherited = false )] [Serializable] public sealed class OleDbPermissionAttribute : DBDataPermissionAttribute { private String _providers; public OleDbPermissionAttribute(SecurityAction action) : base(action) { } [System.ComponentModel.Browsable(false)] [System.ComponentModel.EditorBrowsableAttribute(System.ComponentModel.EditorBrowsableState.Never)] [Obsolete("Provider property has been deprecated. Use the Add method. http://go.microsoft.com/fwlink/?linkid=14202")] public String Provider { get { string providers = _providers; return ((null != providers) ? providers : ADP.StrEmpty); } set { _providers = value; } } override public IPermission CreatePermission() { return new OleDbPermission(this); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
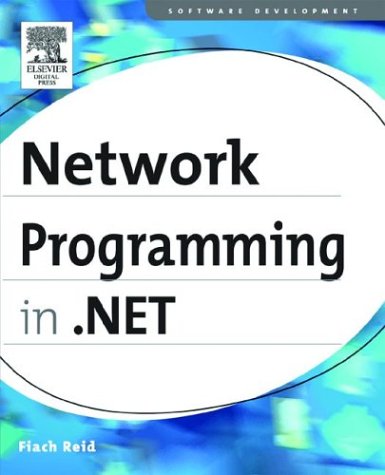
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SchemaAttDef.cs
- CodeConstructor.cs
- TextWriterTraceListener.cs
- SQLMoneyStorage.cs
- FileVersionInfo.cs
- UTF8Encoding.cs
- ExceptionRoutedEventArgs.cs
- AssociatedControlConverter.cs
- AnnotationResourceCollection.cs
- LocalIdKeyIdentifierClause.cs
- XmlDataDocument.cs
- loginstatus.cs
- MultitargetingHelpers.cs
- InvalidCastException.cs
- WpfPayload.cs
- Soap.cs
- SqlMethodCallConverter.cs
- InputScopeNameConverter.cs
- ListMarkerLine.cs
- XPathParser.cs
- GridItem.cs
- ListViewSortEventArgs.cs
- SqlExpander.cs
- MessagePropertyFilter.cs
- SiblingIterators.cs
- Scene3D.cs
- CodeGenerator.cs
- TaskFileService.cs
- WindowsListViewGroup.cs
- InputReport.cs
- FileNotFoundException.cs
- DetailsViewRow.cs
- TimelineCollection.cs
- ShutDownListener.cs
- GraphicsContainer.cs
- WSSecurityXXX2005.cs
- NavigateEvent.cs
- UserMapPath.cs
- NetNamedPipeSecurity.cs
- WebRequest.cs
- TypeUtils.cs
- SqlCommandSet.cs
- SubpageParaClient.cs
- JsonEnumDataContract.cs
- GetWinFXPath.cs
- SmtpReplyReader.cs
- DataGridTextBox.cs
- DataGridItem.cs
- CodeLabeledStatement.cs
- PeerHopCountAttribute.cs
- XmlDataSourceView.cs
- SubtreeProcessor.cs
- ConnectionManagementElement.cs
- WinEventHandler.cs
- PriorityQueue.cs
- ContentPlaceHolder.cs
- DateRangeEvent.cs
- ConnectionPoolManager.cs
- MetadataItemEmitter.cs
- EventWaitHandleSecurity.cs
- PerformanceCounters.cs
- PermissionListSet.cs
- DesignTimeTemplateParser.cs
- ChannelDemuxer.cs
- SqlReorderer.cs
- WasHttpHandlersInstallComponent.cs
- BitArray.cs
- PackageStore.cs
- TableProvider.cs
- DependentList.cs
- XmlStringTable.cs
- BrowserTree.cs
- EntitySqlQueryCacheKey.cs
- InternalBase.cs
- JsonByteArrayDataContract.cs
- XamlStackWriter.cs
- PreProcessInputEventArgs.cs
- ListBindingConverter.cs
- ISFTagAndGuidCache.cs
- PackageProperties.cs
- TextViewBase.cs
- DelegateArgumentReference.cs
- Boolean.cs
- PropertyGridView.cs
- ServiceBehaviorAttribute.cs
- SemanticResultValue.cs
- FilterException.cs
- SerializeAbsoluteContext.cs
- SiteMapDataSourceView.cs
- ClientProxyGenerator.cs
- FontDialog.cs
- ActionMismatchAddressingException.cs
- BitmapSource.cs
- CapiHashAlgorithm.cs
- NotifyParentPropertyAttribute.cs
- ValidationUtility.cs
- EntityExpressionVisitor.cs
- SlipBehavior.cs
- GregorianCalendar.cs
- HtmlControlPersistable.cs