Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / Tools / xws_reg / System / ServiceModel / Install / Configuration / WasHttpHandlersInstallComponent.cs / 1 / WasHttpHandlersInstallComponent.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.ServiceModel.Install.Configuration { using WebAdmin = Microsoft.Web.Administration; using System; using System.Collections; using System.Collections.Generic; using System.Configuration; using System.Globalization; using System.Text; using System.Web.Configuration; internal class WasHttpHandlersInstallComponent : ServiceModelInstallComponent { IIS7ConfigurationLoader configLoader; string displayString; ConfigElementInfo integratedHttpHandler; ListisapiHttpHandlers; WasHttpHandlersInstallComponent(IIS7ConfigurationLoader configLoader) { if (!IisHelper.ShouldInstallWas || !IisHelper.ShouldInstallApplicationHost) { throw new WasNotInstalledException(SR.GetString(SR.WasNotInstalled, SR.GetString(SR.HttpHandlersComponentNameWAS))); } else if (!IisHelper.ShouldInstallIis) { throw new IisNotInstalledException(SR.GetString(SR.IisNotInstalled, SR.GetString(SR.HttpHandlersComponentNameWAS))); } this.configLoader = configLoader; CreateDefaultHttpHandlers(); } void CreateDefaultHttpHandlers() { // Integrated HTTP Handler this.integratedHttpHandler = new ConfigElementInfo(); this.integratedHttpHandler.RawAttributes.Add(ServiceModelInstallStrings.Name, ServiceModelInstallStrings.IIS7IntegratedHttpHandlerName); this.integratedHttpHandler.RawAttributes.Add(ServiceModelInstallStrings.Path, ServiceModelInstallStrings.HttpHandlersPath); this.integratedHttpHandler.RawAttributes.Add(ServiceModelInstallStrings.Verb, ServiceModelInstallStrings.HttpHandlersVerb); this.integratedHttpHandler.RawAttributes.Add(ServiceModelInstallStrings.Type, ServiceModelInstallStrings.HttpHandlersType); this.integratedHttpHandler.RawAttributes.Add(ServiceModelInstallStrings.PreCondition, ServiceModelInstallStrings.IIS7IntegratedMode); // ISAPI HTTP Handlers this.isapiHttpHandlers = new List (); // ISAPI 32-bit Handler ConfigElementInfo handler = new ConfigElementInfo(); handler.RawAttributes.Add(ServiceModelInstallStrings.Name, ServiceModelInstallStrings.IIS7IsapiHttpHandlerName); handler.RawAttributes.Add(ServiceModelInstallStrings.Path, ServiceModelInstallStrings.HttpHandlersPath); handler.RawAttributes.Add(ServiceModelInstallStrings.Verb, ServiceModelInstallStrings.HttpHandlersVerb); handler.RawAttributes.Add(ServiceModelInstallStrings.Modules, ServiceModelInstallStrings.IIS7IsapiModule); if (!InstallHelper.Is64BitMachine()) { handler.RawAttributes.Add(ServiceModelInstallStrings.ScriptProcessor, InstallHelper.GetNativeIsapiFilter(true)); } else { handler.RawAttributes.Add(ServiceModelInstallStrings.ScriptProcessor, InstallHelper.GetWow64IsapiFilter(true)); } handler.RawAttributes.Add(ServiceModelInstallStrings.PreCondition, ServiceModelInstallStrings.IIS7ClassicModePreCondition); this.isapiHttpHandlers.Add(handler); // ISAPI 64-bit Handler if (InstallHelper.Is64BitMachine()) { handler = new ConfigElementInfo(); handler.RawAttributes.Add(ServiceModelInstallStrings.Name, ServiceModelInstallStrings.IIS7Isapi64HttpHandlerName); handler.RawAttributes.Add(ServiceModelInstallStrings.Path, ServiceModelInstallStrings.HttpHandlersPath); handler.RawAttributes.Add(ServiceModelInstallStrings.Verb, ServiceModelInstallStrings.HttpHandlersVerb); handler.RawAttributes.Add(ServiceModelInstallStrings.Modules, ServiceModelInstallStrings.IIS7IsapiModule); handler.RawAttributes.Add(ServiceModelInstallStrings.ScriptProcessor, InstallHelper.GetNativeIsapiFilter(true)); handler.RawAttributes.Add(ServiceModelInstallStrings.PreCondition, ServiceModelInstallStrings.IIS7ClassicMode64PreCondition); this.isapiHttpHandlers.Add(handler); } } internal override string DisplayName { get {return this.displayString; } } protected override string InstallActionMessage { get {return SR.GetString(SR.HttpHandlersComponentInstall, ServiceModelInstallStrings.IIS7IntegratedHttpHandlerName); } } internal override string[] InstalledVersions { get { List installedVersions = new List (); if (null != this.configLoader.HttpHandlersSection) { WebAdmin.ConfigurationElement httpHandler = this.GetHttpHandlerFromCollection(); Type type = HttpHandlersInstallComponent.GetHandlerType((string)httpHandler.GetAttribute(ServiceModelInstallStrings.Type).Value); string versionString = null; if (type != null) { versionString = InstallHelper.GetVersionStringFromTypeString(type.AssemblyQualifiedName); } else { versionString = (string)httpHandler.GetAttribute(ServiceModelInstallStrings.Type).Value; } string preCondition = String.Empty; if(null != httpHandler.GetAttribute(ServiceModelInstallStrings.PreCondition)) { preCondition = (string)httpHandler.GetAttribute(ServiceModelInstallStrings.PreCondition).Value; } versionString = string.Format(CultureInfo.InvariantCulture, "{0} : {1} ({2})", (string)httpHandler.GetAttribute(ServiceModelInstallStrings.Path).Value, versionString, preCondition); if (!installedVersions.Contains(versionString)) { installedVersions.Add(versionString); } } return installedVersions.ToArray(); } } internal override bool IsInstalled { get { bool isInstalled = false; WebAdmin.ConfigurationElement httpHandler = this.GetHttpHandlerFromCollection(); if (null != httpHandler && null != httpHandler.GetAttribute(ServiceModelInstallStrings.Type) && !((string)httpHandler.GetAttribute(ServiceModelInstallStrings.Type).Value).StartsWith(ServiceModelInstallStrings.HttpForbiddenHandlerType, StringComparison.OrdinalIgnoreCase) && !((string)httpHandler.GetAttribute(ServiceModelInstallStrings.Type).Value).StartsWith(ServiceModelInstallStrings.HttpNotFoundHandlerType, StringComparison.OrdinalIgnoreCase)) { isInstalled = true; } return isInstalled; } } protected override string ReinstallActionMessage { get {return SR.GetString(SR.HttpHandlersComponentReinstall, ServiceModelInstallStrings.IIS7IntegratedHttpHandlerName); } } protected override string UninstallActionMessage { get {return SR.GetString(SR.HttpHandlersComponentUninstall, ServiceModelInstallStrings.IIS7IntegratedHttpHandlerName); } } internal static WasHttpHandlersInstallComponent CreateNativeWasHttpHandlersInstallComponent() { WasHttpHandlersInstallComponent wasHttpHandlersInstallComponent = new WasHttpHandlersInstallComponent(new IIS7ConfigurationLoader(new NativeConfigurationLoader())); wasHttpHandlersInstallComponent.displayString = SR.GetString(SR.HttpHandlersComponentNameWAS); return wasHttpHandlersInstallComponent; } WebAdmin.ConfigurationElement GetHttpHandlerFromCollection() { WebAdmin.ConfigurationElement httpHandler = null; if (null != this.configLoader.HttpHandlersSection) { WebAdmin.ConfigurationElementCollection httpHandlersCollection = this.configLoader.HttpHandlersSection.GetCollection(); // HttpHandlersCollection does not have a unique key, thus need to enumerate collection rather than check for key foreach (WebAdmin.ConfigurationElement element in httpHandlersCollection) { if (((string)element.GetAttribute(ServiceModelInstallStrings.Name).Value).Equals(ServiceModelInstallStrings.IIS7IntegratedHttpHandlerName, StringComparison.OrdinalIgnoreCase)) { httpHandler = element; break; } } } return httpHandler; } internal override void Install(OutputLevel outputLevel) { if (!this.IsInstalled) { if (null != this.configLoader.HttpHandlersSection) { AddSvcHandlers(); configLoader.Save(); } else { throw new InvalidOperationException(SR.GetString(SR.IIS7ConfigurationSectionNotFound, this.configLoader.HttpHandlersSectionPath)); } } else { EventLogger.LogWarning(SR.GetString(SR.HttpHandlersComponentAlreadyExists, ServiceModelInstallStrings.IIS7IntegratedHttpHandlerName), (OutputLevel.Verbose == outputLevel)); } } void CopyHandlerAttributes(WebAdmin.ConfigurationElement element, IDictionary attributes) { foreach (KeyValuePair attribute in attributes) { element.GetAttribute(attribute.Key).Value = attribute.Value; } } void AddSvcHandlers() { WebAdmin.ConfigurationElementCollection httpHandlersCollection = this.configLoader.HttpHandlersSection.GetCollection(); List oldSvcHandlers = new List (); for (int i = 0; i < httpHandlersCollection.Count; i++) { if (string.Compare((string)httpHandlersCollection[i].GetAttribute(ServiceModelInstallStrings.Path).Value, ServiceModelInstallStrings.HttpHandlersPath, StringComparison.OrdinalIgnoreCase) == 0) { oldSvcHandlers.Add(httpHandlersCollection[i]); } } // Remove existing svc handlers for (int i = 0; i < oldSvcHandlers.Count; i++) { httpHandlersCollection.Remove(oldSvcHandlers[i]); } // Add our handler before the first wildcard handler WebAdmin.ConfigurationElement newHandlerAction = httpHandlersCollection.CreateElement(); CopyHandlerAttributes(newHandlerAction, integratedHttpHandler.RawAttributes); // Always install to the top of the config so that we are before wildcard "*" handlers. httpHandlersCollection.AddAt(0, newHandlerAction); for (int i = 0; i < isapiHttpHandlers.Count; i++) { newHandlerAction = httpHandlersCollection.CreateElement(); CopyHandlerAttributes(newHandlerAction, isapiHttpHandlers[i].RawAttributes); httpHandlersCollection.AddAt(i + 1, newHandlerAction); } } internal override void Uninstall(OutputLevel outputLevel) { if (this.IsInstalled) { // Remove the httpHandlers node WebAdmin.ConfigurationElement httpHandler = this.GetHttpHandlerFromCollection(); httpHandler.GetAttribute(ServiceModelInstallStrings.Type).Value = ServiceModelInstallStrings.HttpForbiddenHandlerType; configLoader.Save(); } else { EventLogger.LogWarning(SR.GetString(SR.HttpHandlersComponentNotInstalled, ServiceModelInstallStrings.IIS7IntegratedHttpHandlerName), (OutputLevel.Verbose == outputLevel)); } } internal override InstallationState VerifyInstall() { InstallationState installState = InstallationState.Unknown; if (this.IsInstalled) { WebAdmin.ConfigurationElement httpHandler = this.GetHttpHandlerFromCollection(); if (((string)httpHandler.GetAttribute(ServiceModelInstallStrings.Path).Value).Equals(ServiceModelInstallStrings.HttpHandlersPath, StringComparison.OrdinalIgnoreCase) && ((string)httpHandler.GetAttribute(ServiceModelInstallStrings.Verb).Value).Equals(ServiceModelInstallStrings.HttpHandlersVerb, StringComparison.OrdinalIgnoreCase) && ((string)httpHandler.GetAttribute(ServiceModelInstallStrings.Type).Value).Equals(ServiceModelInstallStrings.HttpHandlersType, StringComparison.OrdinalIgnoreCase) && null != httpHandler.GetAttribute(ServiceModelInstallStrings.PreCondition) && ((string)httpHandler.GetAttribute(ServiceModelInstallStrings.PreCondition).Value).Equals(ServiceModelInstallStrings.IIS7IntegratedMode, StringComparison.OrdinalIgnoreCase)) { installState = InstallationState.InstalledDefaults; } else { installState = InstallationState.InstalledCustom; } } else { installState = InstallationState.NotInstalled; } return installState; } class ConfigElementInfo { IDictionary rawAttributes; public ConfigElementInfo() { rawAttributes = new Dictionary (); } public IDictionary RawAttributes { get { return this.rawAttributes; } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
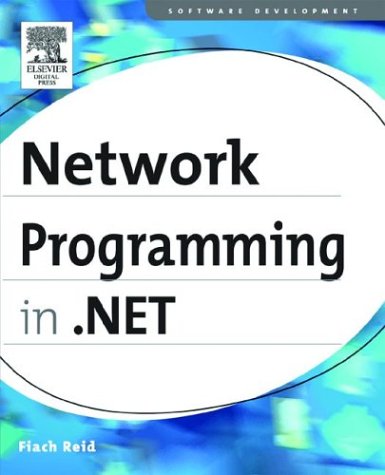
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- EnumerableCollectionView.cs
- TreeNodeBindingCollection.cs
- CodeTypeOfExpression.cs
- keycontainerpermission.cs
- ConfigXmlSignificantWhitespace.cs
- EnumValAlphaComparer.cs
- _HTTPDateParse.cs
- VisualTreeHelper.cs
- AttributeCollection.cs
- printdlgexmarshaler.cs
- OdbcConnectionString.cs
- ReflectionTypeLoadException.cs
- RequestCachePolicyConverter.cs
- TextTreeInsertUndoUnit.cs
- XPathDescendantIterator.cs
- XPathBuilder.cs
- BinaryUtilClasses.cs
- AppearanceEditorPart.cs
- EpmCustomContentDeSerializer.cs
- ChangesetResponse.cs
- RemotingConfiguration.cs
- __Filters.cs
- AdornerDecorator.cs
- VariantWrapper.cs
- WmfPlaceableFileHeader.cs
- JumpTask.cs
- ManagementClass.cs
- GridViewHeaderRowPresenter.cs
- DataBindingExpressionBuilder.cs
- MLangCodePageEncoding.cs
- PagerStyle.cs
- DictionaryChange.cs
- HTTPNotFoundHandler.cs
- SamlSubjectStatement.cs
- SqlConnectionStringBuilder.cs
- XmlParser.cs
- ResourceProperty.cs
- PowerModeChangedEventArgs.cs
- MemoryRecordBuffer.cs
- ValidationRuleCollection.cs
- ProxyOperationRuntime.cs
- BooleanExpr.cs
- ReachPageContentSerializerAsync.cs
- DebuggerAttributes.cs
- PasswordRecoveryAutoFormat.cs
- AnnotationComponentManager.cs
- MailWebEventProvider.cs
- SrgsElementFactoryCompiler.cs
- LoginCancelEventArgs.cs
- UIElement.cs
- RequestStatusBarUpdateEventArgs.cs
- ArrayElementGridEntry.cs
- SqlDependencyUtils.cs
- OciLobLocator.cs
- BufferBuilder.cs
- SchemaCollectionCompiler.cs
- RewritingSimplifier.cs
- HMAC.cs
- SetterBase.cs
- PackageRelationshipSelector.cs
- RuntimeConfigLKG.cs
- Codec.cs
- HttpServerVarsCollection.cs
- DigestComparer.cs
- TraceFilter.cs
- ValidationRule.cs
- AutomationPropertyInfo.cs
- DefaultValueTypeConverter.cs
- HostedElements.cs
- ApplicationSecurityInfo.cs
- EditorZoneBase.cs
- Triplet.cs
- SymDocumentType.cs
- DataBoundControlHelper.cs
- PositiveTimeSpanValidator.cs
- PersistChildrenAttribute.cs
- XmlSchemaSubstitutionGroup.cs
- TagMapCollection.cs
- GroupDescription.cs
- QueryOperationResponseOfT.cs
- UnmanagedHandle.cs
- BuildProvidersCompiler.cs
- MultiBindingExpression.cs
- Maps.cs
- ConnectionPoint.cs
- PrintDialogException.cs
- SectionInput.cs
- DataListItemEventArgs.cs
- ButtonChrome.cs
- XPathExpr.cs
- ServiceBuildProvider.cs
- WebResourceAttribute.cs
- ReflectTypeDescriptionProvider.cs
- DelegateSerializationHolder.cs
- TextClipboardData.cs
- TableColumn.cs
- InstanceData.cs
- IISUnsafeMethods.cs
- ConfigUtil.cs
- HostedHttpTransportManager.cs