Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Base / System / IO / Packaging / PackageRelationshipSelector.cs / 1 / PackageRelationshipSelector.cs
//------------------------------------------------------------------------------ // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // This class represents a PackageRelationshipSelector. // // History: // 07/27/2005: SarjanaS: Initial creation. // //----------------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Diagnostics; // for Debug.Assert using MS.Internal.IO.Packaging; using System.Windows; // For Exception strings - SRID namespace System.IO.Packaging { ////// This class is used to represent a PackageRelationship selector. PackageRelationships can be /// selected based on their Type or ID. This class will specify what the selection is based on and /// what the actual criteria is. public sealed class PackageRelationshipSelector { //----------------------------------------------------- // // Public Constructors // //----------------------------------------------------- #region Public Constructor ////// Constructor /// /// Source Uri of the PackagePart or PackageRoot ("/") that owns the relationship /// PackageRelationshipSelectorType enum representing the type of the selectionCriteria /// The actual string that is used to select the relationships ///If sourceUri is null ///If selectionCriteria is null ///If selectorType Enumeration does not have a valid value ///If PackageRelationshipSelectorType.Id and selection criteria is not valid Xsd Id ///If PackageRelationshipSelectorType.Type and selection criteria is not valid relationship type ///If sourceUri is not "/" to indicate the PackageRoot, then it must conform to the /// valid PartUri syntax public PackageRelationshipSelector(Uri sourceUri, PackageRelationshipSelectorType selectorType, string selectionCriteria) { if (sourceUri == null) throw new ArgumentNullException("sourceUri"); if (selectionCriteria == null) throw new ArgumentNullException("selectionCriteria"); //If the sourceUri is not equal to "/", it must be a valid part name. if(Uri.Compare(sourceUri,PackUriHelper.PackageRootUri, UriComponents.SerializationInfoString, UriFormat.UriEscaped, StringComparison.Ordinal) != 0) sourceUri = PackUriHelper.ValidatePartUri(sourceUri); //selectionCriteria is tested here as per the value of the selectorType. //If selectionCriteria is empty string we will throw the appropriate error message. if(selectorType == PackageRelationshipSelectorType.Type) InternalRelationshipCollection.ThrowIfInvalidRelationshipType(selectionCriteria); else if (selectorType == PackageRelationshipSelectorType.Id) InternalRelationshipCollection.ThrowIfInvalidXsdId(selectionCriteria); else throw new ArgumentOutOfRangeException("selectorType"); _sourceUri = sourceUri; _selectionCriteria = selectionCriteria; _selectorType = selectorType; } #endregion Public Constructor //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- #region Public Properties ////// This is a uri to the parent PackagePart to which this relationship belongs. /// ///PackagePart public Uri SourceUri { get { return _sourceUri; } } ////// Enumeration value indicating the interpretations of the SelectionCriteria. /// ///public PackageRelationshipSelectorType SelectorType { get { return _selectorType; } } /// /// Selection Criteria - actual value (could be ID or type) on which the selection is based /// ///public string SelectionCriteria { get { return _selectionCriteria; } } #endregion Public Properties //------------------------------------------------------ // // Public Methods // //------------------------------------------------------ #region Public Methods /// /// This method returns the list of selected PackageRelationships as per the /// given criteria, from a part in the Package provided /// /// Package object from which we get the relationsips ////// If package parameter is null public ListSelect(Package package) { if(package == null) { throw new ArgumentNullException("package"); } List relationships = new List (0); switch (SelectorType) { case PackageRelationshipSelectorType.Id: if (SourceUri.Equals(PackUriHelper.PackageRootUri)) { if (package.RelationshipExists(SelectionCriteria)) relationships.Add(package.GetRelationship(SelectionCriteria)); } else { if (package.PartExists(SourceUri)) { PackagePart part = package.GetPart(SourceUri); if (part.RelationshipExists(SelectionCriteria)) relationships.Add(part.GetRelationship(SelectionCriteria)); } } break; case PackageRelationshipSelectorType.Type: if (SourceUri.Equals(PackUriHelper.PackageRootUri)) { foreach (PackageRelationship r in package.GetRelationshipsByType(SelectionCriteria)) relationships.Add(r); } else { if (package.PartExists(SourceUri)) { foreach (PackageRelationship r in package.GetPart(SourceUri).GetRelationshipsByType(SelectionCriteria)) relationships.Add(r); } } break; default: //Debug.Assert is fine here since the parameters have already been validated. And all the properties are //readonly Debug.Assert(false, "This option should never be called"); break; } return relationships; } #endregion Public Methods //----------------------------------------------------- // // Public Events // //------------------------------------------------------ // None //----------------------------------------------------- // // Internal Constructors // //----------------------------------------------------- // None //----------------------------------------------------- // // Internal Properties // //------------------------------------------------------ // None //----------------------------------------------------- // // Internal Methods // //------------------------------------------------------ // None //------------------------------------------------------ // // Internal Events // //----------------------------------------------------- // None //------------------------------------------------------ // // Private Methods // //----------------------------------------------------- // None //----------------------------------------------------- // // Private Fields // //----------------------------------------------------- #region Private Members private Uri _sourceUri; private string _selectionCriteria; private PackageRelationshipSelectorType _selectorType; #endregion Private Members } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ // // // Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // This class represents a PackageRelationshipSelector. // // History: // 07/27/2005: SarjanaS: Initial creation. // //----------------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Diagnostics; // for Debug.Assert using MS.Internal.IO.Packaging; using System.Windows; // For Exception strings - SRID namespace System.IO.Packaging { ////// This class is used to represent a PackageRelationship selector. PackageRelationships can be /// selected based on their Type or ID. This class will specify what the selection is based on and /// what the actual criteria is. public sealed class PackageRelationshipSelector { //----------------------------------------------------- // // Public Constructors // //----------------------------------------------------- #region Public Constructor ////// Constructor /// /// Source Uri of the PackagePart or PackageRoot ("/") that owns the relationship /// PackageRelationshipSelectorType enum representing the type of the selectionCriteria /// The actual string that is used to select the relationships ///If sourceUri is null ///If selectionCriteria is null ///If selectorType Enumeration does not have a valid value ///If PackageRelationshipSelectorType.Id and selection criteria is not valid Xsd Id ///If PackageRelationshipSelectorType.Type and selection criteria is not valid relationship type ///If sourceUri is not "/" to indicate the PackageRoot, then it must conform to the /// valid PartUri syntax public PackageRelationshipSelector(Uri sourceUri, PackageRelationshipSelectorType selectorType, string selectionCriteria) { if (sourceUri == null) throw new ArgumentNullException("sourceUri"); if (selectionCriteria == null) throw new ArgumentNullException("selectionCriteria"); //If the sourceUri is not equal to "/", it must be a valid part name. if(Uri.Compare(sourceUri,PackUriHelper.PackageRootUri, UriComponents.SerializationInfoString, UriFormat.UriEscaped, StringComparison.Ordinal) != 0) sourceUri = PackUriHelper.ValidatePartUri(sourceUri); //selectionCriteria is tested here as per the value of the selectorType. //If selectionCriteria is empty string we will throw the appropriate error message. if(selectorType == PackageRelationshipSelectorType.Type) InternalRelationshipCollection.ThrowIfInvalidRelationshipType(selectionCriteria); else if (selectorType == PackageRelationshipSelectorType.Id) InternalRelationshipCollection.ThrowIfInvalidXsdId(selectionCriteria); else throw new ArgumentOutOfRangeException("selectorType"); _sourceUri = sourceUri; _selectionCriteria = selectionCriteria; _selectorType = selectorType; } #endregion Public Constructor //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- #region Public Properties ////// This is a uri to the parent PackagePart to which this relationship belongs. /// ///PackagePart public Uri SourceUri { get { return _sourceUri; } } ////// Enumeration value indicating the interpretations of the SelectionCriteria. /// ///public PackageRelationshipSelectorType SelectorType { get { return _selectorType; } } /// /// Selection Criteria - actual value (could be ID or type) on which the selection is based /// ///public string SelectionCriteria { get { return _selectionCriteria; } } #endregion Public Properties //------------------------------------------------------ // // Public Methods // //------------------------------------------------------ #region Public Methods /// /// This method returns the list of selected PackageRelationships as per the /// given criteria, from a part in the Package provided /// /// Package object from which we get the relationsips ////// If package parameter is null public ListSelect(Package package) { if(package == null) { throw new ArgumentNullException("package"); } List relationships = new List (0); switch (SelectorType) { case PackageRelationshipSelectorType.Id: if (SourceUri.Equals(PackUriHelper.PackageRootUri)) { if (package.RelationshipExists(SelectionCriteria)) relationships.Add(package.GetRelationship(SelectionCriteria)); } else { if (package.PartExists(SourceUri)) { PackagePart part = package.GetPart(SourceUri); if (part.RelationshipExists(SelectionCriteria)) relationships.Add(part.GetRelationship(SelectionCriteria)); } } break; case PackageRelationshipSelectorType.Type: if (SourceUri.Equals(PackUriHelper.PackageRootUri)) { foreach (PackageRelationship r in package.GetRelationshipsByType(SelectionCriteria)) relationships.Add(r); } else { if (package.PartExists(SourceUri)) { foreach (PackageRelationship r in package.GetPart(SourceUri).GetRelationshipsByType(SelectionCriteria)) relationships.Add(r); } } break; default: //Debug.Assert is fine here since the parameters have already been validated. And all the properties are //readonly Debug.Assert(false, "This option should never be called"); break; } return relationships; } #endregion Public Methods //----------------------------------------------------- // // Public Events // //------------------------------------------------------ // None //----------------------------------------------------- // // Internal Constructors // //----------------------------------------------------- // None //----------------------------------------------------- // // Internal Properties // //------------------------------------------------------ // None //----------------------------------------------------- // // Internal Methods // //------------------------------------------------------ // None //------------------------------------------------------ // // Internal Events // //----------------------------------------------------- // None //------------------------------------------------------ // // Private Methods // //----------------------------------------------------- // None //----------------------------------------------------- // // Private Fields // //----------------------------------------------------- #region Private Members private Uri _sourceUri; private string _selectionCriteria; private PackageRelationshipSelectorType _selectorType; #endregion Private Members } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
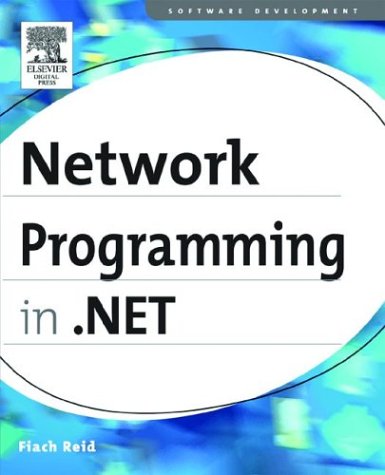
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CompiledAction.cs
- PeerNameRegistration.cs
- SectionXmlInfo.cs
- OleDbEnumerator.cs
- XmlTextReader.cs
- ChtmlLinkAdapter.cs
- ForeignKeyConstraint.cs
- FileEnumerator.cs
- EventKeyword.cs
- SystemEvents.cs
- DBDataPermissionAttribute.cs
- BamlResourceSerializer.cs
- FormatControl.cs
- DelegatingTypeDescriptionProvider.cs
- ContainerParaClient.cs
- SecUtil.cs
- CodeEntryPointMethod.cs
- CollectionEditVerbManager.cs
- PenThreadPool.cs
- KeyTime.cs
- ExpressionParser.cs
- InternalControlCollection.cs
- TextTreeTextNode.cs
- JulianCalendar.cs
- WindowShowOrOpenTracker.cs
- JsonGlobals.cs
- TableCell.cs
- HtmlElementErrorEventArgs.cs
- ResXResourceSet.cs
- AddInAdapter.cs
- ScriptReferenceEventArgs.cs
- PowerModeChangedEventArgs.cs
- Collection.cs
- TransformCryptoHandle.cs
- ObjectDataSourceStatusEventArgs.cs
- Trace.cs
- ZoneLinkButton.cs
- LocalBuilder.cs
- ThreadTrace.cs
- ThrowHelper.cs
- CodeAccessPermission.cs
- LinkedResource.cs
- XmlTextAttribute.cs
- SpecialNameAttribute.cs
- RbTree.cs
- TextEffect.cs
- FrameworkElementAutomationPeer.cs
- GeometryHitTestParameters.cs
- EdmProviderManifest.cs
- DictionaryContent.cs
- MembershipSection.cs
- RegisteredArrayDeclaration.cs
- Helper.cs
- SQLInt16.cs
- TextPenaltyModule.cs
- EnumMember.cs
- GenericPrincipal.cs
- TransformerInfoCollection.cs
- ContentAlignmentEditor.cs
- SecureStringHasher.cs
- COM2Enum.cs
- AssemblyCacheEntry.cs
- HttpContext.cs
- ChannelFactoryBase.cs
- FrameworkEventSource.cs
- BaseTemplateParser.cs
- WebWorkflowRole.cs
- Journal.cs
- PreviewPageInfo.cs
- XmlSchemaSimpleType.cs
- ActivationProxy.cs
- WinCategoryAttribute.cs
- StringInfo.cs
- LogLogRecordEnumerator.cs
- BehaviorEditorPart.cs
- FlowDocumentView.cs
- CustomSignedXml.cs
- ContentType.cs
- MemberAssignment.cs
- ChannelDispatcherBase.cs
- TagPrefixCollection.cs
- DeploymentSectionCache.cs
- ObjectDataSource.cs
- AsynchronousChannel.cs
- InlinedAggregationOperatorEnumerator.cs
- PhonemeEventArgs.cs
- NativeMethods.cs
- ToolStripGripRenderEventArgs.cs
- Buffer.cs
- Border.cs
- XmlBindingWorker.cs
- CodeIdentifiers.cs
- BaseTransportHeaders.cs
- ConfigXmlDocument.cs
- ExpressionBindingCollection.cs
- GridViewActionList.cs
- ComNativeDescriptor.cs
- ButtonBase.cs
- ConfigXmlText.cs
- WorkerRequest.cs