Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / AddIn / AddIn / System / Addin / Hosting / Store / AddInAdapter.cs / 1305376 / AddInAdapter.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== /*============================================================ ** ** Class: AddInAdapter ** ** Purpose: Represents an add-in adapter on disk ** ===========================================================*/ using System; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Globalization; using System.Reflection; using System.Text; using System.AddIn.MiniReflection; using System.Diagnostics.Contracts; namespace System.AddIn { [Serializable] internal sealed class AddInAdapter : PipelineComponent { private List_contracts; private List _constructors; public AddInAdapter(TypeInfo typeInfo, String assemblyLocation) : base(typeInfo, assemblyLocation) { _contracts = new List (); _constructors = new List (); } public List Constructors { get { return _constructors; } } public List Contracts { get { return _contracts; } } public override String ToString() { return String.Format(CultureInfo.CurrentCulture, Res.AddInAdapterToString, Name, BestAvailableLocation); } internal override bool Validate(Type type, Collection warnings) { System.Diagnostics.Contracts.Contract.Assert(type.Assembly.ReflectionOnly && IContractInReflectionLoaderContext.Assembly.ReflectionOnly, "Both the type and IContract should be in the ReflectionOnly loader context"); if (!type.IsMarshalByRef) { warnings.Add(String.Format(CultureInfo.CurrentCulture, Res.AddInAdapterMustBeMBRO, type.AssemblyQualifiedName)); return false; } //if (!type.Implements(typeofIContract)) if (!IContractInReflectionLoaderContext.IsAssignableFrom(type)) { warnings.Add(String.Format(CultureInfo.CurrentCulture, Res.AddInAdapterMustImplementAnAddInContract, type.AssemblyQualifiedName)); return false; } foreach (Type contractInterface in type.GetInterfaces()) { //if (contractInterface.Implements(typeofIContract)) if (IContractInReflectionLoaderContext.IsAssignableFrom(contractInterface)) _contracts.Add(new TypeInfo(contractInterface)); } if (_contracts.Count == 0) { warnings.Add(String.Format(CultureInfo.CurrentCulture, Res.AddInAdapterMustImplementAnAddInContract, type.AssemblyQualifiedName)); return false; } foreach (ConstructorInfo ctor in type.GetConstructors(BindingFlags.Public | BindingFlags.NonPublic | BindingFlags.Instance)) { ParameterInfo[] pars = ctor.GetParameters(); if (pars.Length != 1) { warnings.Add(String.Format(CultureInfo.CurrentCulture, Res.AddInAdapterOneUnusableConstructor, type.AssemblyQualifiedName)); continue; } _constructors.Add(new TypeInfo(pars[0].ParameterType)); } if (_constructors.Count == 0) { warnings.Add(String.Format(CultureInfo.CurrentCulture, Res.AddInAdapterNoUsableConstructors, type.AssemblyQualifiedName)); return false; } return base.Validate(type, warnings); } // Imagine a generic AddInBase (AB ), and an AddInAdapter with a // constructor taking in AB . If we have IntAddIn : AB , // then we should be able to hook this up. internal bool CanConnectTo(AddInBase addInBase) { System.Diagnostics.Contracts.Contract.Requires(addInBase != null); if (!addInBase.TypeInfo.IsGeneric) { if (this.Constructors.Contains(addInBase.TypeInfo)) return true; // return true if we have a constructor that accepts one of addinBase's ActivatableAs base classes if (addInBase._activatableAs != null) { foreach (TypeInfo activatableAsTypeInfo in addInBase._activatableAs) { if (this.Constructors.Contains(activatableAsTypeInfo)) return true; } } } else { return false; } return false; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
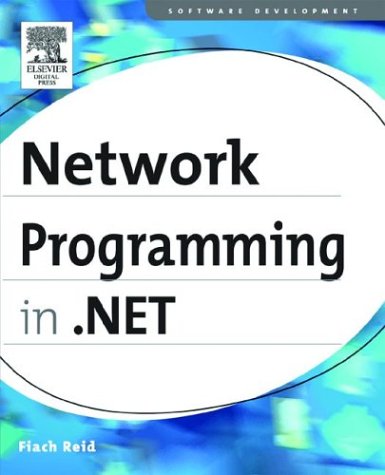
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SafeLocalMemHandle.cs
- RectKeyFrameCollection.cs
- RunWorkerCompletedEventArgs.cs
- FlatButtonAppearance.cs
- InputBindingCollection.cs
- URLIdentityPermission.cs
- SmtpReplyReaderFactory.cs
- _UriSyntax.cs
- CodeAttributeArgumentCollection.cs
- XmlConvert.cs
- XamlStyleSerializer.cs
- CapabilitiesAssignment.cs
- ControlBuilder.cs
- C14NUtil.cs
- infer.cs
- BinaryObjectReader.cs
- RegexRunnerFactory.cs
- CopyNamespacesAction.cs
- LinqToSqlWrapper.cs
- TraceContext.cs
- Util.cs
- VBIdentifierName.cs
- ButtonFieldBase.cs
- Point.cs
- RectangleHotSpot.cs
- OracleLob.cs
- AsyncPostBackTrigger.cs
- WebEventCodes.cs
- FileDialogPermission.cs
- TextMetrics.cs
- FormsAuthenticationModule.cs
- Vector3DConverter.cs
- XmlByteStreamWriter.cs
- PolygonHotSpot.cs
- FileLoadException.cs
- OperationBehaviorAttribute.cs
- OdbcConnection.cs
- DocobjHost.cs
- Int64Converter.cs
- DispatchRuntime.cs
- InsufficientMemoryException.cs
- FixedSOMTableRow.cs
- InlineUIContainer.cs
- AnimationTimeline.cs
- TriggerBase.cs
- HealthMonitoringSection.cs
- SafePEFileHandle.cs
- SettingsPropertyCollection.cs
- SettingsSavedEventArgs.cs
- CodeBinaryOperatorExpression.cs
- ControlPropertyNameConverter.cs
- TypeConverterValueSerializer.cs
- RadioButton.cs
- DataGridViewAutoSizeColumnsModeEventArgs.cs
- TitleStyle.cs
- RenderData.cs
- MenuItemBindingCollection.cs
- CheckStoreFileValidityRequest.cs
- HtmlProps.cs
- ErrorWebPart.cs
- MultiBindingExpression.cs
- AssemblyBuilderData.cs
- DropShadowBitmapEffect.cs
- UpWmlPageAdapter.cs
- CodeTryCatchFinallyStatement.cs
- OracleException.cs
- DragSelectionMessageFilter.cs
- FileSecurity.cs
- DmlSqlGenerator.cs
- DelimitedListTraceListener.cs
- Image.cs
- SymbolEqualComparer.cs
- TopClause.cs
- ResourcePart.cs
- ObjectResult.cs
- ScriptingScriptResourceHandlerSection.cs
- TextEmbeddedObject.cs
- SafePEFileHandle.cs
- SafeEventLogWriteHandle.cs
- DataGridLinkButton.cs
- BrowserCapabilitiesFactory35.cs
- CollectionTraceRecord.cs
- XmlSchemaInclude.cs
- FlagsAttribute.cs
- NetNamedPipeBindingCollectionElement.cs
- WindowsNonControl.cs
- EtwTrackingBehaviorElement.cs
- ControlLocalizer.cs
- TextServicesPropertyRanges.cs
- OperationFormatUse.cs
- StringReader.cs
- TraceListeners.cs
- DecoderBestFitFallback.cs
- DataGridViewSortCompareEventArgs.cs
- DataService.cs
- Vector3DCollectionValueSerializer.cs
- ComPlusServiceLoader.cs
- OleDbRowUpdatingEvent.cs
- TTSEngineTypes.cs
- TemplatedControlDesigner.cs