Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / ServiceModel / System / ServiceModel / Security / WSSecurityXXX2005.cs / 1 / WSSecurityXXX2005.cs
//------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace System.ServiceModel.Security { using System; using System.ServiceModel; using System.Collections; using System.Collections.Generic; using System.Diagnostics; using System.Globalization; using System.IO; using System.Text; using System.Threading; using System.Xml; using System.IdentityModel.Claims; using System.IdentityModel.Policy; using System.IdentityModel.Tokens; using System.Security.Cryptography.X509Certificates; using System.ServiceModel.Security.Tokens; using HexBinary = System.Runtime.Remoting.Metadata.W3cXsd2001.SoapHexBinary; using System.ServiceModel.Channels; using System.ServiceModel.Security; using System.Runtime.Serialization; using KeyIdentifierEntry = WSSecurityTokenSerializer.KeyIdentifierEntry; using KeyIdentifierClauseEntry = WSSecurityTokenSerializer.KeyIdentifierClauseEntry; using TokenEntry = WSSecurityTokenSerializer.TokenEntry; using StrEntry = WSSecurityTokenSerializer.StrEntry; class WSSecurityXXX2005 : WSSecurityJan2004 { public WSSecurityXXX2005(WSSecurityTokenSerializer tokenSerializer, SamlSerializer samlSerializer) : base(tokenSerializer, samlSerializer) { } public override void PopulateStrEntries(IListstrEntries) { PopulateJan2004StrEntries(strEntries); strEntries.Add(new SamlXXX2005KeyIdentifierStrEntry()); strEntries.Add(new SamlDirectStrEntry()); strEntries.Add(new X509ThumbprintStrEntry(this.WSSecurityTokenSerializer)); strEntries.Add(new EncryptedKeyHashStrEntry(this.WSSecurityTokenSerializer)); } public override void PopulateTokenEntries(IList tokenEntryList) { PopulateJan2004TokenEntries(tokenEntryList); tokenEntryList.Add(new WSSecurityXXX2005.WrappedKeyTokenEntry(this.WSSecurityTokenSerializer)); tokenEntryList.Add(new WSSecurityXXX2005.SamlTokenEntry(this.WSSecurityTokenSerializer, this.SamlSerializer)); } public override void PopulateKeyIdentifierClauseEntries(IList clauseEntries) { List strEntries = new List (); this.WSSecurityTokenSerializer.PopulateStrEntries(strEntries); SecurityTokenReferenceXXX2005ClauseEntry strClause = new SecurityTokenReferenceXXX2005ClauseEntry(this.WSSecurityTokenSerializer, strEntries); clauseEntries.Add(strClause); } new class SamlTokenEntry : WSSecurityJan2004.SamlTokenEntry { public SamlTokenEntry(WSSecurityTokenSerializer tokenSerializer, SamlSerializer samlSerializer) : base(tokenSerializer, samlSerializer) { } public override string TokenTypeUri { get { return SecurityXXX2005Strings.SamlTokenType; } } } new class WrappedKeyTokenEntry : WSSecurityJan2004.WrappedKeyTokenEntry { public WrappedKeyTokenEntry(WSSecurityTokenSerializer tokenSerializer) : base(tokenSerializer) { } public override string TokenTypeUri { get { return SecurityXXX2005Strings.EncryptedKeyTokenType; } } } class SecurityTokenReferenceXXX2005ClauseEntry : SecurityTokenReferenceJan2004ClauseEntry { public SecurityTokenReferenceXXX2005ClauseEntry(WSSecurityTokenSerializer tokenSerializer, IList strEntries) : base(tokenSerializer, strEntries) { } protected override string ReadTokenType(XmlDictionaryReader reader) { return reader.GetAttribute(XD.SecurityXXX2005Dictionary.TokenTypeAttribute, XD.SecurityXXX2005Dictionary.Namespace); } } class EncryptedKeyHashStrEntry : WSSecurityJan2004.KeyIdentifierStrEntry { protected override Type ClauseType { get { return typeof(EncryptedKeyHashIdentifierClause); } } public override Type TokenType { get { return typeof(WrappedKeySecurityToken); } } protected override string ValueTypeUri { get { return SecurityXXX2005Strings.EncryptedKeyHashValueType; } } public EncryptedKeyHashStrEntry(WSSecurityTokenSerializer tokenSerializer) : base(tokenSerializer) { } public override bool CanReadClause(XmlDictionaryReader reader, string tokenType) { // Backward compatible with V1. Accept if missing. if (tokenType != null && tokenType != SecurityXXX2005Strings.EncryptedKeyTokenType) { return false; } return base.CanReadClause(reader, tokenType); } protected override SecurityKeyIdentifierClause CreateClause(byte[] bytes, byte[] derivationNonce, int derivationLength) { return new EncryptedKeyHashIdentifierClause(bytes, true, derivationNonce, derivationLength); } // WS-Security 1.1's EncryptedKey external ref requires wsse11:TokenType public override void WriteContent(XmlDictionaryWriter writer, SecurityKeyIdentifierClause clause) { writer.WriteAttributeString(XD.SecurityXXX2005Dictionary.Prefix.Value, XD.SecurityXXX2005Dictionary.TokenTypeAttribute, XD.SecurityXXX2005Dictionary.Namespace, SecurityXXX2005Strings.EncryptedKeyTokenType); base.WriteContent(writer, clause); } } class X509ThumbprintStrEntry : WSSecurityJan2004.KeyIdentifierStrEntry { protected override Type ClauseType { get { return typeof(X509ThumbprintKeyIdentifierClause); } } public override Type TokenType { get { return typeof(X509SecurityToken); } } protected override string ValueTypeUri { get { return SecurityXXX2005Strings.ThumbprintSha1ValueType; } } public X509ThumbprintStrEntry(WSSecurityTokenSerializer tokenSerializer) : base(tokenSerializer) { } protected override SecurityKeyIdentifierClause CreateClause(byte[] bytes, byte[] derivationNonce, int derivationLength) { return new X509ThumbprintKeyIdentifierClause(bytes); } } class SamlXXX2005KeyIdentifierStrEntry : SamlJan2004KeyIdentifierStrEntry { protected override bool IsMatchingValueType(string valueType) { return (base.IsMatchingValueType(valueType) || valueType == XD.SecurityXXX2005Dictionary.Saml11AssertionValueType.Value); } public override void WriteContent(XmlDictionaryWriter writer, SecurityKeyIdentifierClause clause) { SamlAssertionKeyIdentifierClause samlClause = (SamlAssertionKeyIdentifierClause) clause; // write the token type as part of the STR if (samlClause.TokenTypeUri != null) { writer.WriteAttributeString(XD.SecurityXXX2005Dictionary.Prefix.Value, XD.SecurityXXX2005Dictionary.TokenTypeAttribute, XD.SecurityXXX2005Dictionary.Namespace, samlClause.TokenTypeUri); } base.WriteContent(writer, clause); } } class SamlDirectStrEntry : StrEntry { public override bool CanReadClause(XmlDictionaryReader reader, string tokenType) { if (tokenType != XD.SecurityXXX2005Dictionary.Saml20TokenType.Value) { return false; } return (reader.IsStartElement(XD.SecurityJan2004Dictionary.Reference, XD.SecurityJan2004Dictionary.Namespace)); } public override Type GetTokenType(SecurityKeyIdentifierClause clause) { return null; } public override SecurityKeyIdentifierClause ReadClause(XmlDictionaryReader reader, byte[] derivationNone, int derivationLength, string tokenType) { string samlUri = reader.GetAttribute(XD.SecurityJan2004Dictionary.URI, null); if (reader.IsEmptyElement) { reader.Read(); } else { reader.ReadStartElement(); reader.ReadEndElement(); } return new SamlAssertionDirectKeyIdentifierClause(samlUri, derivationNone, derivationLength); } public override bool SupportsCore(SecurityKeyIdentifierClause clause) { return typeof(SamlAssertionDirectKeyIdentifierClause).IsAssignableFrom(clause.GetType()); } public override void WriteContent(XmlDictionaryWriter writer, SecurityKeyIdentifierClause clause) { writer.WriteAttributeString(XD.SecurityXXX2005Dictionary.Prefix.Value, XD.SecurityXXX2005Dictionary.TokenTypeAttribute, XD.SecurityXXX2005Dictionary.Namespace, XD.SecurityXXX2005Dictionary.Saml20TokenType.Value); SamlAssertionDirectKeyIdentifierClause samlClause = clause as SamlAssertionDirectKeyIdentifierClause; writer.WriteStartElement(XD.SecurityJan2004Dictionary.Prefix.Value, XD.SecurityJan2004Dictionary.Reference, XD.SecurityJan2004Dictionary.Namespace); writer.WriteAttributeString(XD.SecurityJan2004Dictionary.URI, null, samlClause.SamlUri); writer.WriteEndElement(); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
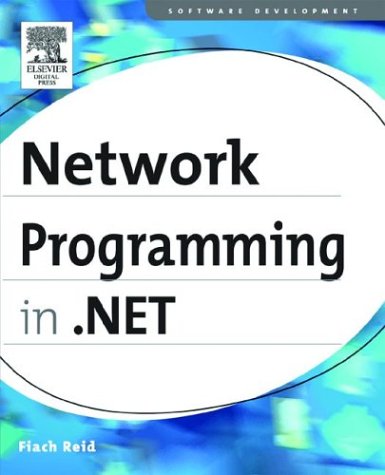
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- GeometryGroup.cs
- UserNameSecurityTokenAuthenticator.cs
- DataKeyArray.cs
- DomNameTable.cs
- CreateUserWizard.cs
- TPLETWProvider.cs
- XmlSchemaValidationException.cs
- QueryStringConverter.cs
- WorkerRequest.cs
- FrameworkReadOnlyPropertyMetadata.cs
- ListMarkerLine.cs
- UnmanagedMemoryStreamWrapper.cs
- PagesChangedEventArgs.cs
- DictionaryTraceRecord.cs
- TemplateXamlTreeBuilder.cs
- RSAPKCS1KeyExchangeFormatter.cs
- BitmapPalettes.cs
- ControlIdConverter.cs
- OverlappedAsyncResult.cs
- XXXInfos.cs
- DbConnectionHelper.cs
- GregorianCalendarHelper.cs
- ListComponentEditor.cs
- GlobalAllocSafeHandle.cs
- UriTemplateClientFormatter.cs
- AspCompat.cs
- QilReplaceVisitor.cs
- SchemaLookupTable.cs
- WebPartDisplayModeCancelEventArgs.cs
- QilPatternFactory.cs
- MemoryPressure.cs
- TypeResolver.cs
- AudioFormatConverter.cs
- BinaryObjectInfo.cs
- Compiler.cs
- ListControlStringCollectionEditor.cs
- Logging.cs
- DatePickerTextBox.cs
- OrderByBuilder.cs
- ScriptResourceAttribute.cs
- __FastResourceComparer.cs
- Encoder.cs
- ApplicationSecurityManager.cs
- ConnectionProviderAttribute.cs
- DataTemplate.cs
- _ReceiveMessageOverlappedAsyncResult.cs
- Splitter.cs
- IntPtr.cs
- XmlSchemaIdentityConstraint.cs
- PriorityBinding.cs
- SplitContainer.cs
- TemplateField.cs
- StorageMappingItemLoader.cs
- ListBindingConverter.cs
- SqlDataSourceTableQuery.cs
- TextParaLineResult.cs
- WebPartChrome.cs
- CompressedStack.cs
- WebBrowserBase.cs
- AngleUtil.cs
- NamespaceEmitter.cs
- HttpProcessUtility.cs
- LocatorPartList.cs
- ExpressionBuilderContext.cs
- WorkflowInstanceUnhandledExceptionRecord.cs
- DataComponentMethodGenerator.cs
- ContentValidator.cs
- TemplateXamlParser.cs
- PartBasedPackageProperties.cs
- CreateInstanceBinder.cs
- XmlCodeExporter.cs
- ProfileGroupSettingsCollection.cs
- CryptoKeySecurity.cs
- UnSafeCharBuffer.cs
- WebCategoryAttribute.cs
- TextureBrush.cs
- ConfigXmlCDataSection.cs
- XmlComment.cs
- Logging.cs
- DesignerVerbCollection.cs
- SecurityContext.cs
- PermissionAttributes.cs
- EventProviderBase.cs
- FieldNameLookup.cs
- SineEase.cs
- TreeViewImageGenerator.cs
- WebPartConnectionsCancelVerb.cs
- CompilerScopeManager.cs
- WebPartEditorOkVerb.cs
- PagesChangedEventArgs.cs
- OptimalTextSource.cs
- SafeArrayTypeMismatchException.cs
- XmlStringTable.cs
- Guid.cs
- GetRecipientRequest.cs
- NativeActivityAbortContext.cs
- ControlBuilder.cs
- NetworkInterface.cs
- ThrowHelper.cs
- TargetPerspective.cs