Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / Designer / WebForms / System / Web / UI / Design / WebParts / DeclarativeCatalogPartDesigner.cs / 1 / DeclarativeCatalogPartDesigner.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.Design.WebControls.WebParts { using System.ComponentModel; using System.ComponentModel.Design; using System.Diagnostics; using System.Design; using System.Web.UI.Design; using System.Web.UI.WebControls; using System.Web.UI.WebControls.WebParts; [System.Security.Permissions.SecurityPermission(System.Security.Permissions.SecurityAction.Demand, Flags=System.Security.Permissions.SecurityPermissionFlag.UnmanagedCode)] public class DeclarativeCatalogPartDesigner : CatalogPartDesigner { private const string templateName = "WebPartsTemplate"; private DeclarativeCatalogPart _catalogPart; private TemplateGroup _templateGroup; public override TemplateGroupCollection TemplateGroups { get { TemplateGroupCollection groups = base.TemplateGroups; if (_templateGroup == null) { _templateGroup = new TemplateGroup(templateName, _catalogPart.ControlStyle); _templateGroup.AddTemplateDefinition(new TemplateDefinition(this, templateName, _catalogPart, templateName, _catalogPart.ControlStyle)); } groups.Add(_templateGroup); return groups; } } public override string GetDesignTimeHtml() { if (!(_catalogPart.Parent is CatalogZoneBase)) { return CreateInvalidParentDesignTimeHtml(typeof(CatalogPart), typeof(CatalogZoneBase)); } string designTimeHtml = String.Empty; try { if (((DeclarativeCatalogPart)ViewControl).WebPartsTemplate == null) { designTimeHtml = GetEmptyDesignTimeHtml(); } else { // DeclarativeCatalogPart has no default runtime rendering, so GetDesignTimeHtml() should also // return String.Empty, so we don't get the '[Type "ID"]' rendered in the designer. // designTimeHtml = String.Empty; } } catch (Exception e) { designTimeHtml = GetErrorDesignTimeHtml(e); } return designTimeHtml; } protected override string GetEmptyDesignTimeHtml() { return CreatePlaceHolderDesignTimeHtml(SR.GetString(SR.DeclarativeCatalogPartDesigner_Empty)); } public override void Initialize(IComponent component) { VerifyInitializeArgument(component, typeof(DeclarativeCatalogPart)); base.Initialize(component); _catalogPart = (DeclarativeCatalogPart)component; if (View != null) { View.SetFlags(ViewFlags.TemplateEditing, true); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
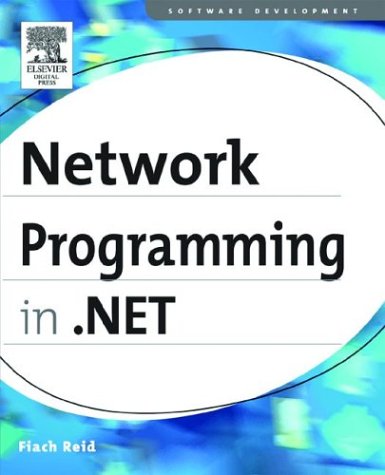
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- StaticFileHandler.cs
- ImageCollectionCodeDomSerializer.cs
- TextElement.cs
- XmlElementAttributes.cs
- PolicyManager.cs
- SvcMapFile.cs
- ParserStreamGeometryContext.cs
- ProcessModelSection.cs
- TypeHelpers.cs
- propertyentry.cs
- GridItemCollection.cs
- RequestSecurityTokenForRemoteTokenFactory.cs
- ViewPort3D.cs
- DataGridViewComboBoxCell.cs
- GenerateTemporaryAssemblyTask.cs
- RectangleGeometry.cs
- DynamicQueryableWrapper.cs
- MediaEntryAttribute.cs
- TextRunCache.cs
- _ServiceNameStore.cs
- FamilyCollection.cs
- FixedSOMElement.cs
- ThrowHelper.cs
- DataTransferEventArgs.cs
- XmlILOptimizerVisitor.cs
- PathTooLongException.cs
- AssemblyGen.cs
- BStrWrapper.cs
- Debug.cs
- Model3DCollection.cs
- BoundingRectTracker.cs
- PerformanceCounterPermissionAttribute.cs
- WindowsHyperlink.cs
- NumericExpr.cs
- ConfigurationStrings.cs
- FormatterServices.cs
- DesignerDataParameter.cs
- QueryOutputWriter.cs
- XmlSerializationGeneratedCode.cs
- ComboBoxItem.cs
- SortDescriptionCollection.cs
- ServiceDeploymentInfo.cs
- ExpressionBuilderCollection.cs
- DynamicPhysicalDiscoSearcher.cs
- XmlSchemaDocumentation.cs
- CriticalFinalizerObject.cs
- GridViewCommandEventArgs.cs
- CLSCompliantAttribute.cs
- XmlImplementation.cs
- DiffuseMaterial.cs
- CultureSpecificCharacterBufferRange.cs
- HierarchicalDataBoundControl.cs
- AlphaSortedEnumConverter.cs
- validationstate.cs
- OutputScopeManager.cs
- RectangleGeometry.cs
- CryptoStream.cs
- BooleanExpr.cs
- SeverityFilter.cs
- ListenerElementsCollection.cs
- RSAOAEPKeyExchangeDeformatter.cs
- ResourceExpressionEditorSheet.cs
- CacheRequest.cs
- IIS7WorkerRequest.cs
- UriPrefixTable.cs
- FunctionImportMapping.cs
- WebDisplayNameAttribute.cs
- RijndaelManaged.cs
- _BasicClient.cs
- ProcessManager.cs
- CodePageEncoding.cs
- ExeConfigurationFileMap.cs
- ConfigXmlSignificantWhitespace.cs
- Html32TextWriter.cs
- TraceUtils.cs
- ScrollableControlDesigner.cs
- PixelFormat.cs
- FormViewUpdatedEventArgs.cs
- mil_commands.cs
- PropertiesTab.cs
- CodeTypeReferenceExpression.cs
- DelegateInArgument.cs
- CommandField.cs
- X509Certificate2.cs
- DataControlPagerLinkButton.cs
- GridViewCancelEditEventArgs.cs
- WindowsEditBox.cs
- FontStretches.cs
- DocumentViewerBase.cs
- MouseGestureConverter.cs
- EnterpriseServicesHelper.cs
- FilteredXmlReader.cs
- TimeSpanParse.cs
- SqlParameterizer.cs
- CellParaClient.cs
- BrushMappingModeValidation.cs
- ListView.cs
- BitStream.cs
- EpmCustomContentSerializer.cs
- SecurityException.cs