Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / ndp / fx / src / xsp / System / Web / Extensions / Compilation / WCFModel / ClientOptions.cs / 3 / ClientOptions.cs
//------------------------------------------------------------------------------ //// Copyright (C) Microsoft Corporation. All Rights Reserved. // //----------------------------------------------------------------------------- using System; using System.Collections.Generic; using System.IO; using System.Text; using System.Xml; using System.Xml.Schema; using XmlSerialization = System.Xml.Serialization; #if WEB_EXTENSIONS_CODE namespace System.Web.Compilation.WCFModel #else namespace Microsoft.VSDesigner.WCFModel #endif { ////// ///#if WEB_EXTENSIONS_CODE internal class ClientOptions #else [CLSCompliant(true)] public class ClientOptions #endif { private bool m_GenerateAsynchronousMethods; private bool m_EnableDataBinding; private List m_ExcludedTypeList; private bool m_ImportXmlTypes; private bool m_GenerateInternalTypes; private bool m_GenerateMessageContracts; private List m_NamespaceMappingList; private List m_CollectionMappingList; private bool m_GenerateSerializableTypes; private ProxySerializerType m_Serializer; private bool m_ReferenceAllAssemblies; private List m_ReferencedAssemblyList; private List m_ReferencedDataContractTypeList; private List m_ServiceContractMappingList; private bool m_UseSerializerForFaults; private bool m_UseSerializerForFaultsSpecified; private bool m_Wrapped; private bool m_WrappedSpecified; /// /// Control whether asynchronous proxy will be generated /// ////// [XmlSerialization.XmlElement()] public bool GenerateAsynchronousMethods { get { return m_GenerateAsynchronousMethods; } set { m_GenerateAsynchronousMethods = value; } } /// /// control whether to generate INotifyPropertyChanged interface on data contract types /// ////// [XmlSerialization.XmlElement()] public bool EnableDataBinding { get { return m_EnableDataBinding; } set { m_EnableDataBinding = value; } } /// /// contains a list of types which will be excluded when the design time tool matches types automatically /// ////// [XmlSerialization.XmlArray(ElementName = "ExcludedTypes")] [XmlSerialization.XmlArrayItem("ExcludedType", typeof(ReferencedType))] public List ExcludedTypeList { get { if (m_ExcludedTypeList == null) { m_ExcludedTypeList = new List (); } return m_ExcludedTypeList; } } /// /// control whether the data contract serializer should import non-DataContract types as IXmlSerializable types /// ////// [XmlSerialization.XmlElement()] public bool ImportXmlTypes { get { return m_ImportXmlTypes; } set { m_ImportXmlTypes = value; } } /// /// control whether to generate internal types /// ////// [XmlSerialization.XmlElement()] public bool GenerateInternalTypes { get { return m_GenerateInternalTypes; } set { m_GenerateInternalTypes = value; } } /// /// control whether to generate message contract types /// ////// [XmlSerialization.XmlElement()] public bool GenerateMessageContracts { get { return m_GenerateMessageContracts; } set { m_GenerateMessageContracts = value; } } /// /// namespace mapping between metadata namespace and clr namespace /// ////// [XmlSerialization.XmlArray(ElementName = "NamespaceMappings")] [XmlSerialization.XmlArrayItem("NamespaceMapping", typeof(NamespaceMapping))] public List NamespaceMappingList { get { if (m_NamespaceMappingList == null) { m_NamespaceMappingList = new List (); } return m_NamespaceMappingList; } } /// /// known collection types which will be used by code generator /// ////// [XmlSerialization.XmlArray(ElementName = "CollectionMappings")] [XmlSerialization.XmlArrayItem("CollectionMapping", typeof(ReferencedCollectionType))] public List CollectionMappingList { get { if (m_CollectionMappingList == null) { m_CollectionMappingList = new List (); } return m_CollectionMappingList; } } /// /// whether class need be marked with Serializable attribute /// ////// [XmlSerialization.XmlElement()] public bool GenerateSerializableTypes { get { return m_GenerateSerializableTypes; } set { m_GenerateSerializableTypes = value; } } /// /// select serializer between DataContractSerializer or XmlSerializer /// ////// [XmlSerialization.XmlElement()] public ProxySerializerType Serializer { get { return m_Serializer; } set { m_Serializer = value; } } /// /// Control whether or not to UseSerializerForFaults. The System.ServiceModel.FaultImportOptions /// will set its UseMessageFormat Property using this value. /// ////// [XmlSerialization.XmlElement()] public bool UseSerializerForFaults{ get { if (m_UseSerializerForFaultsSpecified){ return m_UseSerializerForFaults; } else { return false; } } set { m_UseSerializerForFaultsSpecified = true; m_UseSerializerForFaults = value; } } /// /// Is UseSerializerForFaults specified? /// ////// [XmlSerialization.XmlIgnore()] public bool UseSerializerForFaultsSpecified { get { return m_UseSerializerForFaultsSpecified; } } /// /// Control whether or not to WrappedOption. The System.ServiceModel.Channels.WrappedOption /// will set its WrappedFlag Property using this value. /// ////// [XmlSerialization.XmlElement()] public bool Wrapped { get { if (m_WrappedSpecified) { return m_Wrapped; } else { return false; } } set { m_WrappedSpecified = true; m_Wrapped = value; } } /// /// Is WrappedOption specified? /// ////// [XmlSerialization.XmlIgnore()] public bool WrappedSpecified { get { return m_WrappedSpecified; } } /// /// Whether we will scan all dependent assemblies for type sharing /// ////// [XmlSerialization.XmlElement()] public bool ReferenceAllAssemblies { get { return m_ReferenceAllAssemblies; } set { m_ReferenceAllAssemblies = value; } } /// /// controll DataContract type sharing /// ////// [XmlSerialization.XmlArray(ElementName = "ReferencedAssemblies")] [XmlSerialization.XmlArrayItem("ReferencedAssembly", typeof(ReferencedAssembly))] public List ReferencedAssemblyList { get { if (m_ReferencedAssemblyList == null) { m_ReferencedAssemblyList = new List (); } return m_ReferencedAssemblyList; } } /// /// controll DataContract type sharing /// ////// [XmlSerialization.XmlArray(ElementName = "ReferencedDataContractTypes")] [XmlSerialization.XmlArrayItem("ReferencedDataContractType", typeof(ReferencedType))] public List ReferencedDataContractTypeList { get { if (m_ReferencedDataContractTypeList == null) { m_ReferencedDataContractTypeList = new List (); } return m_ReferencedDataContractTypeList; } } /// /// control service contract type sharing /// ////// [XmlSerialization.XmlArray(ElementName = "ServiceContractMappings")] [XmlSerialization.XmlArrayItem("ServiceContractMapping", typeof(ContractMapping))] public List ServiceContractMappingList { get { if (m_ServiceContractMappingList == null) { m_ServiceContractMappingList = new List (); } return m_ServiceContractMappingList; } } /// /// Serializer used in proxy generator /// ///public enum ProxySerializerType { [XmlSerialization.XmlEnum(Name = "Auto")] Auto = 0, [XmlSerialization.XmlEnum(Name = "DataContractSerializer")] DataContractSerializer = 1, [XmlSerialization.XmlEnum(Name = "XmlSerializer")] XmlSerializer = 2, } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ // // Copyright (C) Microsoft Corporation. All Rights Reserved. // //----------------------------------------------------------------------------- using System; using System.Collections.Generic; using System.IO; using System.Text; using System.Xml; using System.Xml.Schema; using XmlSerialization = System.Xml.Serialization; #if WEB_EXTENSIONS_CODE namespace System.Web.Compilation.WCFModel #else namespace Microsoft.VSDesigner.WCFModel #endif { ////// ///#if WEB_EXTENSIONS_CODE internal class ClientOptions #else [CLSCompliant(true)] public class ClientOptions #endif { private bool m_GenerateAsynchronousMethods; private bool m_EnableDataBinding; private List m_ExcludedTypeList; private bool m_ImportXmlTypes; private bool m_GenerateInternalTypes; private bool m_GenerateMessageContracts; private List m_NamespaceMappingList; private List m_CollectionMappingList; private bool m_GenerateSerializableTypes; private ProxySerializerType m_Serializer; private bool m_ReferenceAllAssemblies; private List m_ReferencedAssemblyList; private List m_ReferencedDataContractTypeList; private List m_ServiceContractMappingList; private bool m_UseSerializerForFaults; private bool m_UseSerializerForFaultsSpecified; private bool m_Wrapped; private bool m_WrappedSpecified; /// /// Control whether asynchronous proxy will be generated /// ////// [XmlSerialization.XmlElement()] public bool GenerateAsynchronousMethods { get { return m_GenerateAsynchronousMethods; } set { m_GenerateAsynchronousMethods = value; } } /// /// control whether to generate INotifyPropertyChanged interface on data contract types /// ////// [XmlSerialization.XmlElement()] public bool EnableDataBinding { get { return m_EnableDataBinding; } set { m_EnableDataBinding = value; } } /// /// contains a list of types which will be excluded when the design time tool matches types automatically /// ////// [XmlSerialization.XmlArray(ElementName = "ExcludedTypes")] [XmlSerialization.XmlArrayItem("ExcludedType", typeof(ReferencedType))] public List ExcludedTypeList { get { if (m_ExcludedTypeList == null) { m_ExcludedTypeList = new List (); } return m_ExcludedTypeList; } } /// /// control whether the data contract serializer should import non-DataContract types as IXmlSerializable types /// ////// [XmlSerialization.XmlElement()] public bool ImportXmlTypes { get { return m_ImportXmlTypes; } set { m_ImportXmlTypes = value; } } /// /// control whether to generate internal types /// ////// [XmlSerialization.XmlElement()] public bool GenerateInternalTypes { get { return m_GenerateInternalTypes; } set { m_GenerateInternalTypes = value; } } /// /// control whether to generate message contract types /// ////// [XmlSerialization.XmlElement()] public bool GenerateMessageContracts { get { return m_GenerateMessageContracts; } set { m_GenerateMessageContracts = value; } } /// /// namespace mapping between metadata namespace and clr namespace /// ////// [XmlSerialization.XmlArray(ElementName = "NamespaceMappings")] [XmlSerialization.XmlArrayItem("NamespaceMapping", typeof(NamespaceMapping))] public List NamespaceMappingList { get { if (m_NamespaceMappingList == null) { m_NamespaceMappingList = new List (); } return m_NamespaceMappingList; } } /// /// known collection types which will be used by code generator /// ////// [XmlSerialization.XmlArray(ElementName = "CollectionMappings")] [XmlSerialization.XmlArrayItem("CollectionMapping", typeof(ReferencedCollectionType))] public List CollectionMappingList { get { if (m_CollectionMappingList == null) { m_CollectionMappingList = new List (); } return m_CollectionMappingList; } } /// /// whether class need be marked with Serializable attribute /// ////// [XmlSerialization.XmlElement()] public bool GenerateSerializableTypes { get { return m_GenerateSerializableTypes; } set { m_GenerateSerializableTypes = value; } } /// /// select serializer between DataContractSerializer or XmlSerializer /// ////// [XmlSerialization.XmlElement()] public ProxySerializerType Serializer { get { return m_Serializer; } set { m_Serializer = value; } } /// /// Control whether or not to UseSerializerForFaults. The System.ServiceModel.FaultImportOptions /// will set its UseMessageFormat Property using this value. /// ////// [XmlSerialization.XmlElement()] public bool UseSerializerForFaults{ get { if (m_UseSerializerForFaultsSpecified){ return m_UseSerializerForFaults; } else { return false; } } set { m_UseSerializerForFaultsSpecified = true; m_UseSerializerForFaults = value; } } /// /// Is UseSerializerForFaults specified? /// ////// [XmlSerialization.XmlIgnore()] public bool UseSerializerForFaultsSpecified { get { return m_UseSerializerForFaultsSpecified; } } /// /// Control whether or not to WrappedOption. The System.ServiceModel.Channels.WrappedOption /// will set its WrappedFlag Property using this value. /// ////// [XmlSerialization.XmlElement()] public bool Wrapped { get { if (m_WrappedSpecified) { return m_Wrapped; } else { return false; } } set { m_WrappedSpecified = true; m_Wrapped = value; } } /// /// Is WrappedOption specified? /// ////// [XmlSerialization.XmlIgnore()] public bool WrappedSpecified { get { return m_WrappedSpecified; } } /// /// Whether we will scan all dependent assemblies for type sharing /// ////// [XmlSerialization.XmlElement()] public bool ReferenceAllAssemblies { get { return m_ReferenceAllAssemblies; } set { m_ReferenceAllAssemblies = value; } } /// /// controll DataContract type sharing /// ////// [XmlSerialization.XmlArray(ElementName = "ReferencedAssemblies")] [XmlSerialization.XmlArrayItem("ReferencedAssembly", typeof(ReferencedAssembly))] public List ReferencedAssemblyList { get { if (m_ReferencedAssemblyList == null) { m_ReferencedAssemblyList = new List (); } return m_ReferencedAssemblyList; } } /// /// controll DataContract type sharing /// ////// [XmlSerialization.XmlArray(ElementName = "ReferencedDataContractTypes")] [XmlSerialization.XmlArrayItem("ReferencedDataContractType", typeof(ReferencedType))] public List ReferencedDataContractTypeList { get { if (m_ReferencedDataContractTypeList == null) { m_ReferencedDataContractTypeList = new List (); } return m_ReferencedDataContractTypeList; } } /// /// control service contract type sharing /// ////// [XmlSerialization.XmlArray(ElementName = "ServiceContractMappings")] [XmlSerialization.XmlArrayItem("ServiceContractMapping", typeof(ContractMapping))] public List ServiceContractMappingList { get { if (m_ServiceContractMappingList == null) { m_ServiceContractMappingList = new List (); } return m_ServiceContractMappingList; } } /// /// Serializer used in proxy generator /// ///public enum ProxySerializerType { [XmlSerialization.XmlEnum(Name = "Auto")] Auto = 0, [XmlSerialization.XmlEnum(Name = "DataContractSerializer")] DataContractSerializer = 1, [XmlSerialization.XmlEnum(Name = "XmlSerializer")] XmlSerializer = 2, } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
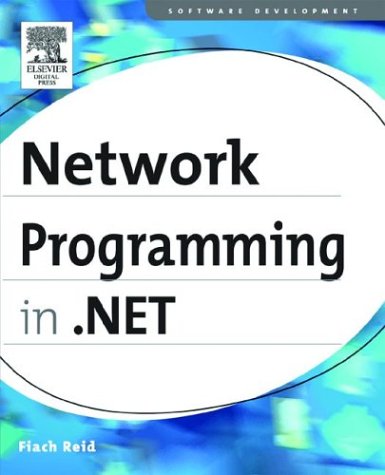
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- HttpRequestWrapper.cs
- LostFocusEventManager.cs
- SQLStringStorage.cs
- X509ChainElement.cs
- ProviderCommandInfoUtils.cs
- DllNotFoundException.cs
- XmlSecureResolver.cs
- OneOfConst.cs
- EventsTab.cs
- ToolStripDropDownButton.cs
- RegexRunner.cs
- ViewCellSlot.cs
- _SecureChannel.cs
- TextEffect.cs
- EventArgs.cs
- DesignerToolboxInfo.cs
- NumberFormatInfo.cs
- WebPartConnectionsConnectVerb.cs
- _AcceptOverlappedAsyncResult.cs
- IUnknownConstantAttribute.cs
- ISFTagAndGuidCache.cs
- TransactionFilter.cs
- EqualityComparer.cs
- ObjectQueryProvider.cs
- BuiltInExpr.cs
- SecurityPermission.cs
- VersionValidator.cs
- ListViewItem.cs
- ImageSource.cs
- PointLight.cs
- SafeMILHandle.cs
- TabletDeviceInfo.cs
- TemplatedWizardStep.cs
- ExpanderAutomationPeer.cs
- Activation.cs
- BamlTreeNode.cs
- DecoratedNameAttribute.cs
- NameSpaceExtractor.cs
- SafeLocalMemHandle.cs
- SymbolEqualComparer.cs
- AnnotationService.cs
- FocusWithinProperty.cs
- QueryInterceptorAttribute.cs
- DynamicActivityTypeDescriptor.cs
- ClientUrlResolverWrapper.cs
- CodeObjectCreateExpression.cs
- IndexedGlyphRun.cs
- TypefaceMetricsCache.cs
- MsmqIntegrationSecurityMode.cs
- Misc.cs
- StrokeDescriptor.cs
- FormsAuthenticationTicket.cs
- Registration.cs
- FloaterBaseParagraph.cs
- XamlStyleSerializer.cs
- CellQuery.cs
- Table.cs
- SystemWebExtensionsSectionGroup.cs
- ComboBox.cs
- DecoderExceptionFallback.cs
- XmlQueryTypeFactory.cs
- SchemaImporterExtensionsSection.cs
- UndoUnit.cs
- TypeElementCollection.cs
- CodeSnippetStatement.cs
- Rules.cs
- AssemblyFilter.cs
- SocketAddress.cs
- VoiceChangeEventArgs.cs
- PeerTransportElement.cs
- DateTimeParse.cs
- TransformedBitmap.cs
- HtmlFormParameterReader.cs
- UIElementIsland.cs
- BitmapImage.cs
- PersonalizationProvider.cs
- DateTimeEditor.cs
- ReliabilityContractAttribute.cs
- WindowsListViewGroupHelper.cs
- InboundActivityHelper.cs
- TypeDescriptionProvider.cs
- Label.cs
- XmlWrappingReader.cs
- PointCollection.cs
- XmlSchemaGroupRef.cs
- SecurityVerifiedMessage.cs
- FormViewModeEventArgs.cs
- MeasureItemEvent.cs
- ImageMetadata.cs
- AsymmetricKeyExchangeFormatter.cs
- BindingMemberInfo.cs
- SecondaryIndex.cs
- GatewayIPAddressInformationCollection.cs
- X509IssuerSerialKeyIdentifierClause.cs
- OrderedDictionary.cs
- Table.cs
- ButtonChrome.cs
- KeyValueSerializer.cs
- XmlCharCheckingReader.cs
- Maps.cs