Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / clr / src / BCL / System / Nullable.cs / 1 / Nullable.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== using System; namespace System { using System.Globalization; using System.Reflection; using System.Collections.Generic; using System.Runtime.CompilerServices; using System.Security; // Warning, don't put System.Runtime.Serialization.On*Serializ*Attribute // on this class without first fixing ObjectClone::InvokeVtsCallbacks // Also, because we have special type system support that says a a boxed Nullable// can be used where a boxed is use, Nullable can not implement any intefaces // at all (since T may not). Do NOT add any interfaces to Nullable! // [TypeDependencyAttribute("System.Collections.Generic.NullableComparer`1")] [TypeDependencyAttribute("System.Collections.Generic.NullableEqualityComparer`1")] [Serializable()] public struct Nullable where T : struct { private bool hasValue; internal T value; public Nullable(T value) { this.value = value; this.hasValue = true; } public bool HasValue { get { return hasValue; } } public T Value { get { if (!HasValue) { ThrowHelper.ThrowInvalidOperationException(ExceptionResource.InvalidOperation_NoValue); } return value; } } public T GetValueOrDefault() { return value; } public T GetValueOrDefault(T defaultValue) { return HasValue ? value : defaultValue; } public override bool Equals(object other) { if (!HasValue) return other == null; if (other == null) return false; return value.Equals(other); } public override int GetHashCode() { return HasValue ? value.GetHashCode() : 0; } public override string ToString() { return HasValue ? value.ToString() : ""; } public static implicit operator Nullable (T value) { return new Nullable (value); } public static explicit operator T(Nullable value) { return value.Value; } // The following already obsoleted methods were removed: // public int CompareTo(object other) // public int CompareTo(Nullable other) // public bool Equals(Nullable other) // public static Nullable FromObject(object value) // public object ToObject() // public string ToString(string format) // public string ToString(IFormatProvider provider) // public string ToString(string format, IFormatProvider provider) // The following newly obsoleted methods were removed: // string IFormattable.ToString(string format, IFormatProvider provider) // int IComparable.CompareTo(object other) // int IComparable >.CompareTo(Nullable other) // bool IEquatable >.Equals(Nullable other) } [System.Runtime.InteropServices.ComVisible(true)] public static class Nullable { [System.Runtime.InteropServices.ComVisible(true)] public static int Compare (Nullable n1, Nullable n2) where T : struct { if (n1.HasValue) { if (n2.HasValue) return Comparer .Default.Compare(n1.value, n2.value); return 1; } if (n2.HasValue) return -1; return 0; } [System.Runtime.InteropServices.ComVisible(true)] public static bool Equals (Nullable n1, Nullable n2) where T : struct { if (n1.HasValue) { if (n2.HasValue) return EqualityComparer .Default.Equals(n1.value, n2.value); return false; } if (n2.HasValue) return false; return true; } // If the type provided is not a Nullable Type, return null. // Otherwise, returns the underlying type of the Nullable type public static Type GetUnderlyingType(Type nullableType) { if( nullableType == null) { throw new ArgumentNullException("nullableType"); } Type result = null; if( nullableType.IsGenericType && !nullableType.IsGenericTypeDefinition) { // instantiated generic type only Type genericType = nullableType.GetGenericTypeDefinition(); if( genericType == typeof(Nullable<>)) { result = nullableType.GetGenericArguments()[0]; } } return result; } // The following already obsoleted methods were removed: // public static bool HasValue (Nullable value) // public static T GetValueOrDefault (Nullable value) // public static T GetValueOrDefault (Nullable value, T valueWhenNull) // The following newly obsoleted methods were removed: // public static Nullable FromObject (object value) // public static object ToObject (Nullable value) // public static object Wrap(object value, Type type) // public static object Unwrap(object value) } }
Link Menu
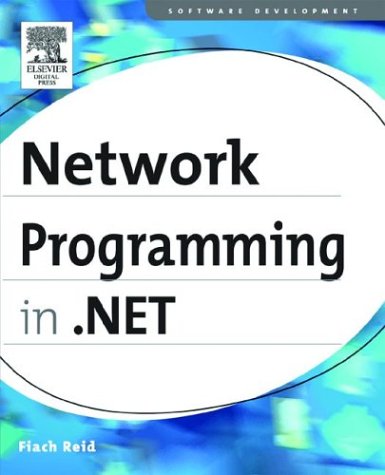
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- NumericPagerField.cs
- SemanticBasicElement.cs
- WebAdminConfigurationHelper.cs
- SqlUserDefinedTypeAttribute.cs
- SapiRecoInterop.cs
- Schema.cs
- CultureTable.cs
- FilterQueryOptionExpression.cs
- Recipient.cs
- returneventsaver.cs
- UnsafeNativeMethods.cs
- LicenseProviderAttribute.cs
- GridViewColumn.cs
- WebBrowserNavigatedEventHandler.cs
- SoapSchemaImporter.cs
- ListViewGroup.cs
- LineUtil.cs
- PageBorderless.cs
- PeerConnector.cs
- CodeIndexerExpression.cs
- IPAddressCollection.cs
- InputLanguageEventArgs.cs
- PrefixHandle.cs
- Menu.cs
- SqlRecordBuffer.cs
- XmlUnspecifiedAttribute.cs
- AppAction.cs
- ListBindableAttribute.cs
- RegexReplacement.cs
- HMAC.cs
- CaseInsensitiveComparer.cs
- TextTreeRootTextBlock.cs
- ComboBox.cs
- WsdlInspector.cs
- ConfigXmlCDataSection.cs
- _ProxyRegBlob.cs
- SystemResourceKey.cs
- EventWaitHandleSecurity.cs
- BoundingRectTracker.cs
- RtfToken.cs
- UriExt.cs
- assemblycache.cs
- TextBox.cs
- DesignerObjectListAdapter.cs
- HttpCapabilitiesSectionHandler.cs
- Bits.cs
- DataSetMappper.cs
- FlowLayout.cs
- SplashScreen.cs
- XmlEncoding.cs
- VScrollProperties.cs
- PropertyMapper.cs
- Empty.cs
- SuppressMergeCheckAttribute.cs
- ComponentGuaranteesAttribute.cs
- TextChangedEventArgs.cs
- ActiveXContainer.cs
- WebPartsPersonalization.cs
- IDispatchConstantAttribute.cs
- ContentPathSegment.cs
- Span.cs
- CodeDirectoryCompiler.cs
- propertyentry.cs
- Metafile.cs
- BamlLocalizationDictionary.cs
- SoapObjectWriter.cs
- ListenerSessionConnectionReader.cs
- CompilerCollection.cs
- EntitySetDataBindingList.cs
- CompiledQuery.cs
- StretchValidation.cs
- HtmlImage.cs
- DESCryptoServiceProvider.cs
- EntityDataReader.cs
- FileReader.cs
- RoleService.cs
- EmptyEnumerator.cs
- COM2EnumConverter.cs
- DataContractSerializer.cs
- SvcMapFile.cs
- WebDescriptionAttribute.cs
- HtmlShim.cs
- DateTimeSerializationSection.cs
- ComponentResourceKeyConverter.cs
- OleDbConnectionPoolGroupProviderInfo.cs
- ControlPropertyNameConverter.cs
- XpsPackagingException.cs
- PluralizationService.cs
- ScriptControlManager.cs
- PropertyNames.cs
- ExpressionEditorSheet.cs
- NamespaceEmitter.cs
- ApplicationActivator.cs
- DataViewManager.cs
- XPathExpr.cs
- XmlProcessingInstruction.cs
- Interop.cs
- ParserExtension.cs
- TheQuery.cs
- PtsHost.cs