Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Framework / System / Windows / Documents / TextTreeRootTextBlock.cs / 1 / TextTreeRootTextBlock.cs
//---------------------------------------------------------------------------- // // File: TextTreeRootTextBlock.cs // // Description: The root node of a TextBlock splay tree. // //--------------------------------------------------------------------------- using System; using MS.Internal; using System.Collections; namespace System.Windows.Documents { // The root node of a TextBlock splay tree. internal class TextTreeRootTextBlock : SplayTreeNode { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors // Creates a TextTreeRootTextBlock instance. internal TextTreeRootTextBlock() { TextTreeTextBlock block; // Allocate an initial block with just two characters -- one for // each edge of the root node. The block will grow when/if // additional content is added. block = new TextTreeTextBlock(2); block.InsertAtNode(this, ElementEdge.AfterStart); } #endregion Constructors //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- #region Public Methods #if DEBUG // Debug-only ToString override. public override string ToString() { return ("RootTextBlock Id=" + this.DebugId); } #endif // DEBUG #endregion Public Methods //------------------------------------------------------ // // Internal Properties // //------------------------------------------------------ #region Internal Properties // The root node never has a parent node. internal override SplayTreeNode ParentNode { get { return null; } set { Invariant.Assert(false, "Can't set ParentNode on TextBlock root!"); } } // Root node of a contained tree, if any. internal override SplayTreeNode ContainedNode { get { return _containedNode; } set { _containedNode = (TextTreeTextBlock)value; } } // The root node never has sibling nodes, so the LeftSymbolCount is a // constant zero. internal override int LeftSymbolCount { get { return 0; } set { Invariant.Assert(false, "TextContainer root is never a sibling!"); } } // Count of unicode chars of all siblings preceding this node. // This property is only used by TextTreeNodes. internal override int LeftCharCount { get { return 0; } set { Invariant.Assert(value == 0); } } // The root node never has siblings, so it never has child nodes. internal override SplayTreeNode LeftChildNode { get { return null; } set { Invariant.Assert(false, "TextBlock root never has sibling nodes!"); } } // The root node never has siblings, so it never has child nodes. internal override SplayTreeNode RightChildNode { get { return null; } set { Invariant.Assert(false, "TextBlock root never has sibling nodes!"); } } // The tree generation. Not used for TextTreeRootTextBlock. internal override uint Generation { get { return 0; } set { Invariant.Assert(false, "TextTreeRootTextBlock does not track Generation!"); } } // Cached symbol offset. The root node is always at offset zero. internal override int SymbolOffsetCache { get { return 0; } set { Invariant.Assert(false, "TextTreeRootTextBlock does not track SymbolOffsetCache!"); } } // Not used for TextTreeRootTextBlock. internal override int SymbolCount { get { return -1; } set { Invariant.Assert(false, "TextTreeRootTextBlock does not track symbol count!"); } } // Count of unicode chars covered by this node and any contained nodes. // This property is only used by TextTreeNodes. internal override int IMECharCount { get { return 0; } set { Invariant.Assert(value == 0); } } #endregion Internal Properties //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ #region Private Fields // Root node of a contained tree, if any. private TextTreeTextBlock _containedNode; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // File: TextTreeRootTextBlock.cs // // Description: The root node of a TextBlock splay tree. // //--------------------------------------------------------------------------- using System; using MS.Internal; using System.Collections; namespace System.Windows.Documents { // The root node of a TextBlock splay tree. internal class TextTreeRootTextBlock : SplayTreeNode { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors // Creates a TextTreeRootTextBlock instance. internal TextTreeRootTextBlock() { TextTreeTextBlock block; // Allocate an initial block with just two characters -- one for // each edge of the root node. The block will grow when/if // additional content is added. block = new TextTreeTextBlock(2); block.InsertAtNode(this, ElementEdge.AfterStart); } #endregion Constructors //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- #region Public Methods #if DEBUG // Debug-only ToString override. public override string ToString() { return ("RootTextBlock Id=" + this.DebugId); } #endif // DEBUG #endregion Public Methods //------------------------------------------------------ // // Internal Properties // //------------------------------------------------------ #region Internal Properties // The root node never has a parent node. internal override SplayTreeNode ParentNode { get { return null; } set { Invariant.Assert(false, "Can't set ParentNode on TextBlock root!"); } } // Root node of a contained tree, if any. internal override SplayTreeNode ContainedNode { get { return _containedNode; } set { _containedNode = (TextTreeTextBlock)value; } } // The root node never has sibling nodes, so the LeftSymbolCount is a // constant zero. internal override int LeftSymbolCount { get { return 0; } set { Invariant.Assert(false, "TextContainer root is never a sibling!"); } } // Count of unicode chars of all siblings preceding this node. // This property is only used by TextTreeNodes. internal override int LeftCharCount { get { return 0; } set { Invariant.Assert(value == 0); } } // The root node never has siblings, so it never has child nodes. internal override SplayTreeNode LeftChildNode { get { return null; } set { Invariant.Assert(false, "TextBlock root never has sibling nodes!"); } } // The root node never has siblings, so it never has child nodes. internal override SplayTreeNode RightChildNode { get { return null; } set { Invariant.Assert(false, "TextBlock root never has sibling nodes!"); } } // The tree generation. Not used for TextTreeRootTextBlock. internal override uint Generation { get { return 0; } set { Invariant.Assert(false, "TextTreeRootTextBlock does not track Generation!"); } } // Cached symbol offset. The root node is always at offset zero. internal override int SymbolOffsetCache { get { return 0; } set { Invariant.Assert(false, "TextTreeRootTextBlock does not track SymbolOffsetCache!"); } } // Not used for TextTreeRootTextBlock. internal override int SymbolCount { get { return -1; } set { Invariant.Assert(false, "TextTreeRootTextBlock does not track symbol count!"); } } // Count of unicode chars covered by this node and any contained nodes. // This property is only used by TextTreeNodes. internal override int IMECharCount { get { return 0; } set { Invariant.Assert(value == 0); } } #endregion Internal Properties //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ #region Private Fields // Root node of a contained tree, if any. private TextTreeTextBlock _containedNode; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
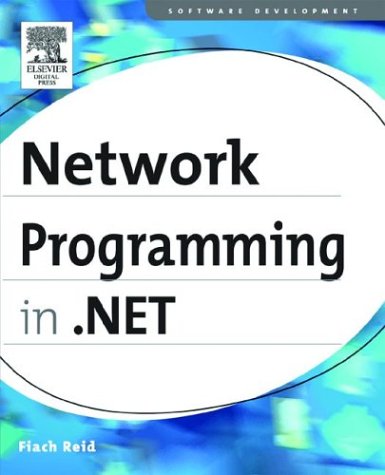
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ToolStripPanelRenderEventArgs.cs
- XmlIgnoreAttribute.cs
- DesignerView.Commands.cs
- Crc32Helper.cs
- SemanticBasicElement.cs
- WebDescriptionAttribute.cs
- SqlErrorCollection.cs
- FontFamilyIdentifier.cs
- ActivityExecutionContext.cs
- XhtmlBasicPanelAdapter.cs
- CodeArgumentReferenceExpression.cs
- HierarchicalDataBoundControl.cs
- GenericTextProperties.cs
- ExpandCollapseProviderWrapper.cs
- SqlConnectionManager.cs
- storepermission.cs
- X509Utils.cs
- SelectionList.cs
- RepeatButton.cs
- SelectionRange.cs
- ActivityInstanceMap.cs
- GeometryGroup.cs
- InternalCache.cs
- ExtendedProtectionPolicyElement.cs
- DataControlFieldHeaderCell.cs
- HtmlInputSubmit.cs
- HtmlForm.cs
- FastEncoderWindow.cs
- SimpleWebHandlerParser.cs
- ProfilePropertySettingsCollection.cs
- ModifiableIteratorCollection.cs
- BaseProcessor.cs
- TokenizerHelper.cs
- SequentialOutput.cs
- XPathNavigator.cs
- VerticalAlignConverter.cs
- PersistChildrenAttribute.cs
- StaticFileHandler.cs
- SqlBinder.cs
- WebPartConnectionsConnectVerb.cs
- HtmlTernaryTree.cs
- DbTransaction.cs
- MetaColumn.cs
- JapaneseCalendar.cs
- LabelLiteral.cs
- Debug.cs
- DashStyle.cs
- ParameterToken.cs
- TransportBindingElement.cs
- SchemaElement.cs
- UIElement.cs
- LineServicesCallbacks.cs
- CookieProtection.cs
- ButtonBaseAdapter.cs
- HttpDebugHandler.cs
- NativeMethodsCLR.cs
- TemplateBindingExpressionConverter.cs
- ChildDocumentBlock.cs
- SqlDataSource.cs
- EnumBuilder.cs
- DataTransferEventArgs.cs
- WebPartVerbsEventArgs.cs
- BindingBase.cs
- SelectionRange.cs
- CharacterMetricsDictionary.cs
- EventLogTraceListener.cs
- StartFileNameEditor.cs
- Empty.cs
- DelegateCompletionCallbackWrapper.cs
- InteropTrackingRecord.cs
- TimeSpanSecondsOrInfiniteConverter.cs
- InProcStateClientManager.cs
- SamlDoNotCacheCondition.cs
- XPathSelfQuery.cs
- ProviderCollection.cs
- BitHelper.cs
- GridViewPageEventArgs.cs
- ExtractedStateEntry.cs
- BigInt.cs
- EntityViewGenerator.cs
- ListViewInsertedEventArgs.cs
- SuppressMergeCheckAttribute.cs
- TypeDescriptorFilterService.cs
- LocalizableAttribute.cs
- OleDbConnectionPoolGroupProviderInfo.cs
- VectorValueSerializer.cs
- ChtmlTextWriter.cs
- RelatedCurrencyManager.cs
- FileAuthorizationModule.cs
- EncodingNLS.cs
- StyleSelector.cs
- HostDesigntimeLicenseContext.cs
- SmuggledIUnknown.cs
- ISAPIApplicationHost.cs
- HostVisual.cs
- SqlAliaser.cs
- IApplicationTrustManager.cs
- ProcessProtocolHandler.cs
- DataGridAutomationPeer.cs
- StringResourceManager.cs