Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Framework / System / Windows / Documents / ValidationHelper.cs / 1 / ValidationHelper.cs
//---------------------------------------------------------------------------- // // File: ValidationHelper.cs // // Copyright (C) Microsoft Corporation. All rights reserved. // // Description: Helpers for TOM parameter validation. // //--------------------------------------------------------------------------- namespace System.Windows.Documents { using MS.Internal; // Invariant.Assert using System.ComponentModel; using System.Windows; using System.Windows.Media; internal static class ValidationHelper { //----------------------------------------------------- // // Internal Methods // //----------------------------------------------------- #region Internal Methods // Verifies a TextPointer is non-null and // is associated with a given TextContainer. // // Throws an appropriate exception if a test fails. internal static void VerifyPosition(ITextContainer tree, ITextPointer position) { VerifyPosition(tree, position, "position"); } // Verifies a TextPointer is non-null and is associated with a given TextContainer. // // Throws an appropriate exception if a test fails. internal static void VerifyPosition(ITextContainer container, ITextPointer position, string paramName) { if (position == null) { throw new ArgumentNullException(paramName); } if (position.TextContainer != container) { throw new ArgumentException(SR.Get(SRID.NotInAssociatedTree, paramName)); } } // Verifies two positions are safe to use as a logical text span. // // Throws ArgumentNullException if startPosition == null || endPosition == null // ArgumentException if startPosition.TextContainer != endPosition.TextContainer or // startPosition > endPosition internal static void VerifyPositionPair(ITextPointer startPosition, ITextPointer endPosition) { if (startPosition == null) { throw new ArgumentNullException("startPosition"); } if (endPosition == null) { throw new ArgumentNullException("endPosition"); } if (startPosition.TextContainer != endPosition.TextContainer) { throw new ArgumentException(SR.Get(SRID.InDifferentTextContainers, "startPosition", "endPosition")); } if (startPosition.CompareTo(endPosition) > 0) { throw new ArgumentException(SR.Get(SRID.BadTextPositionOrder, "startPosition", "endPosition")); } } // Throws an ArgumentException if direction is not a valid enum. internal static void VerifyDirection(LogicalDirection direction, string argumentName) { if (direction != LogicalDirection.Forward && direction != LogicalDirection.Backward) { throw new InvalidEnumArgumentException(argumentName, (int)direction, typeof(LogicalDirection)); } } // Throws an ArgumentException if edge is not a valid enum. internal static void VerifyElementEdge(ElementEdge edge, string param) { if (edge != ElementEdge.BeforeStart && edge != ElementEdge.AfterStart && edge != ElementEdge.BeforeEnd && edge != ElementEdge.AfterEnd) { throw new InvalidEnumArgumentException(param, (int)edge, typeof(ElementEdge)); } } // ............................................................... // // TextSchema Validation // // ............................................................... // Checks whether it is valid to insert the child object at passed position. internal static void ValidateChild(TextPointer position, object child, string paramName) { Invariant.Assert(position != null); Invariant.Assert(position.Parent != null); if (child == null) { throw new ArgumentNullException(paramName); } if (!TextSchema.IsValidChild(/*position:*/position, /*childType:*/child.GetType())) { throw new ArgumentException(SR.Get(SRID.TextSchema_ChildTypeIsInvalid, position.Parent.GetType().Name, child.GetType().Name)); } // The new child should not be currently in other text tree if (child is TextElement) { if (((TextElement)child).Parent != null) { throw new ArgumentException(SR.Get(SRID.TextSchema_TheChildElementBelongsToAnotherTreeAlready, child.GetType().Name)); } } else { Invariant.Assert(child is UIElement); // Cannot call UIElement.Parent across assembly boundary. So skip this part of validation. This condition will be checked elsewhere anyway. //if (((UIElement)child).Parent != null) //{ // throw new ArgumentException(SR.Get(SRID.TextSchema_TheChildElementBelongsToAnotherTreeAlready, child.GetType().Name)); //} } } #endregion Internal methods } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
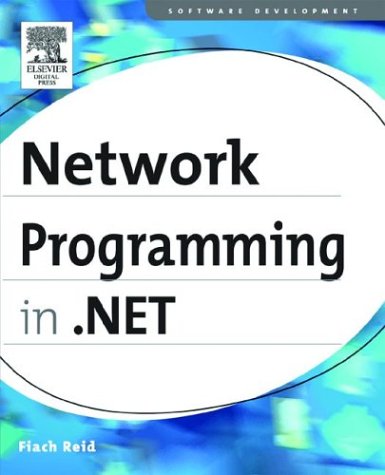
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- WCFServiceClientProxyGenerator.cs
- CheckBoxList.cs
- DBDataPermissionAttribute.cs
- ResourceDisplayNameAttribute.cs
- UpdateProgress.cs
- BufferBuilder.cs
- DependencyPropertyHelper.cs
- FormViewDeleteEventArgs.cs
- PathData.cs
- DefaultSection.cs
- XmlQueryCardinality.cs
- Expander.cs
- SamlSerializer.cs
- MergeLocalizationDirectives.cs
- BufferedStream.cs
- DesignTimeDataBinding.cs
- UpdateCommand.cs
- ScalarRestriction.cs
- ScrollChangedEventArgs.cs
- MachineKeyValidationConverter.cs
- StreamResourceInfo.cs
- SmtpClient.cs
- LinkedList.cs
- PackageDigitalSignature.cs
- SubqueryTrackingVisitor.cs
- invalidudtexception.cs
- XPathSelfQuery.cs
- NetworkCredential.cs
- SqlDataSource.cs
- BinaryFormatterWriter.cs
- DropShadowBitmapEffect.cs
- GuidelineSet.cs
- ResXBuildProvider.cs
- Native.cs
- TraceFilter.cs
- SqlUserDefinedAggregateAttribute.cs
- WsatServiceCertificate.cs
- UTF8Encoding.cs
- SafeFileMappingHandle.cs
- EntityProviderServices.cs
- BlurBitmapEffect.cs
- TableLayoutSettings.cs
- WebPartConnectionsConfigureVerb.cs
- TcpTransportSecurity.cs
- NameValueConfigurationElement.cs
- XmlIncludeAttribute.cs
- Parameter.cs
- SessionStateModule.cs
- MenuEventArgs.cs
- SqlDataSourceCache.cs
- StandardMenuStripVerb.cs
- DateTimeSerializationSection.cs
- ZoomPercentageConverter.cs
- AnnotationAdorner.cs
- EntityDataSourceView.cs
- DrawingState.cs
- Queue.cs
- __TransparentProxy.cs
- BrowserCapabilitiesFactory.cs
- SchemaImporter.cs
- MobileContainerDesigner.cs
- ExtensionQuery.cs
- FieldNameLookup.cs
- EntityContainerEmitter.cs
- SafeCancelMibChangeNotify.cs
- QueryContext.cs
- EntityDataSourceWrapper.cs
- UIElementParaClient.cs
- RandomNumberGenerator.cs
- DiffuseMaterial.cs
- TextBoxAutomationPeer.cs
- SecurityTokenParametersEnumerable.cs
- ContentType.cs
- ResourceDictionary.cs
- MaskedTextBox.cs
- DataGridDetailsPresenter.cs
- DbConnectionStringBuilder.cs
- SpellCheck.cs
- SecurityPermission.cs
- ProxyElement.cs
- DbUpdateCommandTree.cs
- ConstraintConverter.cs
- XmlNodeChangedEventManager.cs
- ListBox.cs
- PlainXmlSerializer.cs
- Grant.cs
- PageCatalogPart.cs
- DbConnectionPoolIdentity.cs
- CorePropertiesFilter.cs
- SqlTrackingWorkflowInstance.cs
- EncodingDataItem.cs
- ShaperBuffers.cs
- MetadataArtifactLoaderFile.cs
- WebPartZoneBase.cs
- CodeAttributeDeclarationCollection.cs
- AccessText.cs
- TrackBar.cs
- HttpCookiesSection.cs
- RecordBuilder.cs
- NumericExpr.cs