Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Framework / System / Windows / Media / Animation / Generated / DiscreteKeyFrames.cs / 1 / DiscreteKeyFrames.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see http://wiki/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- using MS.Internal; using System; using System.Collections; using System.ComponentModel; using System.Diagnostics; using System.Windows.Media; using System.Windows.Media.Media3D; using MS.Internal.PresentationFramework; namespace System.Windows.Media.Animation { ////// This class is used as part of a ThicknessKeyFrameCollection in /// conjunction with a KeyFrameThicknessAnimation to animate a /// Thickness property value along a set of key frames. /// /// This ThicknessKeyFrame changes from the Thickness Value of /// the previous key frame to its own Value without interpolation. The /// change occurs at the KeyTime. /// public class DiscreteThicknessKeyFrame : ThicknessKeyFrame { #region Constructors ////// Creates a new DiscreteThicknessKeyFrame. /// public DiscreteThicknessKeyFrame() : base() { } ////// Creates a new DiscreteThicknessKeyFrame. /// public DiscreteThicknessKeyFrame(Thickness value) : base(value) { } ////// Creates a new DiscreteThicknessKeyFrame. /// public DiscreteThicknessKeyFrame(Thickness value, KeyTime keyTime) : base(value, keyTime) { } #endregion #region Freezable ////// Implementation of ///Freezable.CreateInstanceCore . ///The new Freezable. protected override Freezable CreateInstanceCore() { return new DiscreteThicknessKeyFrame(); } // We don't need to override CloneCore because it won't do anything #endregion #region ThicknessKeyFrame ////// Implemented to linearly interpolate between the baseValue and the /// Value of this KeyFrame using the keyFrameProgress. /// protected override Thickness InterpolateValueCore(Thickness baseValue, double keyFrameProgress) { if (keyFrameProgress < 1.0) { return baseValue; } else { return Value; } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see http://wiki/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- using MS.Internal; using System; using System.Collections; using System.ComponentModel; using System.Diagnostics; using System.Windows.Media; using System.Windows.Media.Media3D; using MS.Internal.PresentationFramework; namespace System.Windows.Media.Animation { ////// This class is used as part of a ThicknessKeyFrameCollection in /// conjunction with a KeyFrameThicknessAnimation to animate a /// Thickness property value along a set of key frames. /// /// This ThicknessKeyFrame changes from the Thickness Value of /// the previous key frame to its own Value without interpolation. The /// change occurs at the KeyTime. /// public class DiscreteThicknessKeyFrame : ThicknessKeyFrame { #region Constructors ////// Creates a new DiscreteThicknessKeyFrame. /// public DiscreteThicknessKeyFrame() : base() { } ////// Creates a new DiscreteThicknessKeyFrame. /// public DiscreteThicknessKeyFrame(Thickness value) : base(value) { } ////// Creates a new DiscreteThicknessKeyFrame. /// public DiscreteThicknessKeyFrame(Thickness value, KeyTime keyTime) : base(value, keyTime) { } #endregion #region Freezable ////// Implementation of ///Freezable.CreateInstanceCore . ///The new Freezable. protected override Freezable CreateInstanceCore() { return new DiscreteThicknessKeyFrame(); } // We don't need to override CloneCore because it won't do anything #endregion #region ThicknessKeyFrame ////// Implemented to linearly interpolate between the baseValue and the /// Value of this KeyFrame using the keyFrameProgress. /// protected override Thickness InterpolateValueCore(Thickness baseValue, double keyFrameProgress) { if (keyFrameProgress < 1.0) { return baseValue; } else { return Value; } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
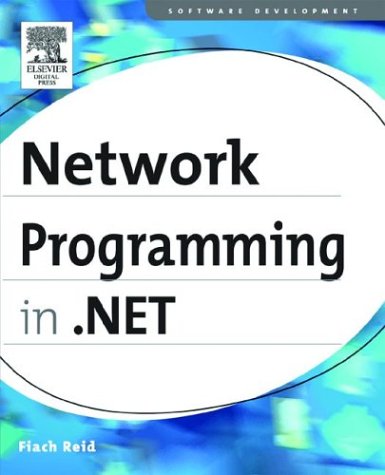
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TagMapCollection.cs
- TextTreeNode.cs
- PeerTransportElement.cs
- InkCanvasSelectionAdorner.cs
- httpserverutility.cs
- EventArgs.cs
- BitmapEffectState.cs
- Boolean.cs
- SqlResolver.cs
- GlobalProxySelection.cs
- COM2Enum.cs
- DbConnectionHelper.cs
- DLinqDataModelProvider.cs
- XhtmlBasicPanelAdapter.cs
- PassportIdentity.cs
- DataRelation.cs
- TextDecorations.cs
- NestPullup.cs
- CodeCastExpression.cs
- LabelDesigner.cs
- EntityContainer.cs
- WorkflowInstanceUnhandledExceptionRecord.cs
- InplaceBitmapMetadataWriter.cs
- AdornerDecorator.cs
- CharConverter.cs
- OleDbDataReader.cs
- DataGridCommandEventArgs.cs
- XmlSchemaAll.cs
- PageAdapter.cs
- RelationshipFixer.cs
- CodeAttachEventStatement.cs
- SqlTopReducer.cs
- ToolboxItemCollection.cs
- BitmapFrameEncode.cs
- ListItemConverter.cs
- RtfFormatStack.cs
- PageBuildProvider.cs
- RecommendedAsConfigurableAttribute.cs
- OutputCacheProfile.cs
- SqlProviderUtilities.cs
- CompilerGlobalScopeAttribute.cs
- QuaternionAnimation.cs
- MarkupWriter.cs
- EventLogPermissionEntry.cs
- FrameworkContentElement.cs
- WebPartConnectionsEventArgs.cs
- SharedPerformanceCounter.cs
- SHA384Cng.cs
- EventListenerClientSide.cs
- ContentHostHelper.cs
- SafeIUnknown.cs
- ListSortDescriptionCollection.cs
- SerialErrors.cs
- HMACSHA512.cs
- UnmanagedMarshal.cs
- sqlmetadatafactory.cs
- DataList.cs
- AttachedPropertyBrowsableAttribute.cs
- RequestCacheValidator.cs
- DataGridViewCellValidatingEventArgs.cs
- AddInDeploymentState.cs
- TraceContextRecord.cs
- ChannelBinding.cs
- XDeferredAxisSource.cs
- ExceptionValidationRule.cs
- PagePropertiesChangingEventArgs.cs
- TextBox.cs
- SmtpReplyReaderFactory.cs
- ToolTipService.cs
- BitmapEffectCollection.cs
- EntityCommandCompilationException.cs
- SqlDataReader.cs
- StringPropertyBuilder.cs
- ListControlBoundActionList.cs
- GenericTextProperties.cs
- ShapingEngine.cs
- ping.cs
- EventProviderWriter.cs
- EditorZone.cs
- ListSortDescriptionCollection.cs
- Int32KeyFrameCollection.cs
- FamilyMap.cs
- StylusButton.cs
- RectAnimationUsingKeyFrames.cs
- TransactionFilter.cs
- UserControlCodeDomTreeGenerator.cs
- DialogResultConverter.cs
- TransformCryptoHandle.cs
- SoapHeaderAttribute.cs
- PenThreadPool.cs
- WebPartConnection.cs
- GridItem.cs
- _NegoState.cs
- Trace.cs
- UdpSocketReceiveManager.cs
- JavaScriptString.cs
- AssemblyBuilder.cs
- WebBrowserDocumentCompletedEventHandler.cs
- SqlFunctionAttribute.cs
- TypeConvertions.cs