Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / xsp / System / Web / UI / StringPropertyBuilder.cs / 1 / StringPropertyBuilder.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI { using System; using System.Collections; ////// Builds inner string properties. /// internal sealed class StringPropertyBuilder : ControlBuilder { private string _text; ////// Creates a new instance of StringPropertyBuilder. /// internal StringPropertyBuilder() { } internal StringPropertyBuilder(string text) { _text = text; } ////// Returns the inner text of the property. /// public string Text { get { return (_text == null) ? String.Empty : _text; } } ////// Gets the inner text of the property. /// public override void AppendLiteralString(string s) { if (ParentBuilder != null && ParentBuilder.HtmlDecodeLiterals()) s = HttpUtility.HtmlDecode(s); _text = s; } ////// Throws an exception - string properties cannot contain other objects. /// public override void AppendSubBuilder(ControlBuilder subBuilder) { throw new HttpException(SR.GetString(SR.StringPropertyBuilder_CannotHaveChildObjects, TagName, (ParentBuilder != null ? ParentBuilder.TagName : String.Empty))); } public override object BuildObject() { return Text; } public override void Init(TemplateParser parser, ControlBuilder parentBuilder, Type type, string tagName, string ID, IDictionary attribs) { base.Init(parser, parentBuilder, type /*type*/, tagName, ID, attribs); SetControlType(typeof(string)); } } }
Link Menu
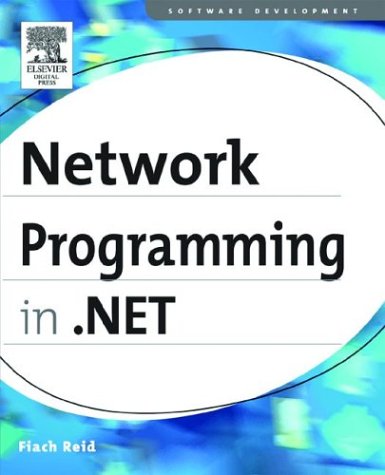
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SoapCodeExporter.cs
- PrinterSettings.cs
- GACMembershipCondition.cs
- GeneralTransform3DGroup.cs
- TabItemWrapperAutomationPeer.cs
- _NtlmClient.cs
- SmtpException.cs
- MarginCollapsingState.cs
- SerializationAttributes.cs
- WebPartTracker.cs
- ITextView.cs
- TextAdaptor.cs
- SortedSetDebugView.cs
- TypeGeneratedEventArgs.cs
- SectionRecord.cs
- TranslateTransform.cs
- CodeDefaultValueExpression.cs
- AutomationPatternInfo.cs
- SecurityKeyIdentifierClause.cs
- XmlDeclaration.cs
- Executor.cs
- LockCookie.cs
- compensatingcollection.cs
- ReflectPropertyDescriptor.cs
- TextBoxDesigner.cs
- HistoryEventArgs.cs
- ConfigurationStrings.cs
- loginstatus.cs
- MemberProjectedSlot.cs
- SecurityTokenProviderContainer.cs
- BoundsDrawingContextWalker.cs
- _AutoWebProxyScriptWrapper.cs
- AssemblyAttributes.cs
- Helper.cs
- HotCommands.cs
- AssociativeAggregationOperator.cs
- FormsAuthenticationTicket.cs
- DocobjHost.cs
- WebPartPersonalization.cs
- SqlTopReducer.cs
- PluralizationService.cs
- BypassElementCollection.cs
- DbUpdateCommandTree.cs
- DbProviderFactories.cs
- ConfigurationValues.cs
- SchemaMapping.cs
- TypeExtensionConverter.cs
- BufferedGraphics.cs
- QueryOutputWriterV1.cs
- DtrList.cs
- GuidTagList.cs
- TypeBuilder.cs
- ComplexType.cs
- FrameworkElementFactory.cs
- ClientConfigurationHost.cs
- PageCopyCount.cs
- CatalogPart.cs
- ObjectPersistData.cs
- CodeMemberMethod.cs
- Win32PrintDialog.cs
- CapabilitiesPattern.cs
- ConditionCollection.cs
- SqlConnectionManager.cs
- IteratorFilter.cs
- CodeCommentStatement.cs
- SqlInternalConnection.cs
- ProcessRequestArgs.cs
- SqlRemoveConstantOrderBy.cs
- Ray3DHitTestResult.cs
- HttpConfigurationContext.cs
- DiagnosticsConfigurationHandler.cs
- DataGridViewCheckBoxColumn.cs
- MultiByteCodec.cs
- ValueProviderWrapper.cs
- EntityClientCacheKey.cs
- Point3DValueSerializer.cs
- SchemaImporterExtensionElementCollection.cs
- KeyboardEventArgs.cs
- EncoderNLS.cs
- ServiceEndpoint.cs
- ThreadSafeList.cs
- LazyTextWriterCreator.cs
- FlowDocument.cs
- DateTimeSerializationSection.cs
- XmlTextWriter.cs
- DuplicateWaitObjectException.cs
- LabelLiteral.cs
- Bezier.cs
- SchemaObjectWriter.cs
- CroppedBitmap.cs
- ContentValidator.cs
- TriggerActionCollection.cs
- XslCompiledTransform.cs
- PersonalizationProviderHelper.cs
- AnimationClockResource.cs
- InstanceData.cs
- HttpDictionary.cs
- RuntimeIdentifierPropertyAttribute.cs
- PropertyInformationCollection.cs
- DataGridCommandEventArgs.cs