Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / System / Windows / Media3D / GeneralTransform3DGroup.cs / 1305600 / GeneralTransform3DGroup.cs
//------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation, 2001 // // File: GeneralTransform3DGroup.cs //----------------------------------------------------------------------------- using System; using System.Windows; using System.Windows.Media; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Reflection; using System.Collections; using System.Collections.Generic; using MS.Internal; using System.Windows.Media.Animation; using System.Globalization; using System.Text; using System.Runtime.InteropServices; using System.Windows.Markup; using System.Windows.Media.Composition; using System.Diagnostics; using MS.Internal.PresentationCore; using SR = MS.Internal.PresentationCore.SR; using SRID = MS.Internal.PresentationCore.SRID; namespace System.Windows.Media.Media3D { ////// GeneralTransform3D group /// [ContentProperty("Children")] [Localizability(LocalizationCategory.None, Readability = Readability.Unreadable)] public sealed partial class GeneralTransform3DGroup : GeneralTransform3D { #region Constructors ////// Default Constructor /// public GeneralTransform3DGroup() { } #endregion ////// Transform a point /// /// input point /// output point ///True if the point is transformed successfully public override bool TryTransform(Point3D inPoint, out Point3D result) { result = inPoint; // cache the children to avoid a repeated DP access GeneralTransform3DCollection children = Children; if ((children == null) || (children.Count == 0)) { return false; } bool pointTransformed = true; // transform the point through each of the transforms for (int i = 0, count = children.Count; i < count; i++) { if (children._collection[i].TryTransform(inPoint, out result) == false) { pointTransformed = false; break; } inPoint = result; } return pointTransformed; } ////// Transforms the bounding box to the smallest axis aligned bounding box /// that contains all the points in the original bounding box /// /// Input bounding rect ///Transformed bounding rect public override Rect3D TransformBounds(Rect3D rect) { // cache the children to avoid a repeated DP access GeneralTransform3DCollection children = Children; if ((children == null) || (children.Count == 0)) { return rect; } Rect3D result = rect; for (int i = 0, count = children.Count; i < count; i++) { result = children._collection[i].TransformBounds(result); } return result; } ////// Returns the inverse transform if it has an inverse, null otherwise /// public override GeneralTransform3D Inverse { get { // cache the children to avoid a repeated DP access GeneralTransform3DCollection children = Children; if ((children == null) || (children.Count == 0)) { return null; } GeneralTransform3DGroup group = new GeneralTransform3DGroup(); for (int i = children.Count - 1; i >= 0; i--) { GeneralTransform3D g = children._collection[i].Inverse; // if any of the transforms does not have an inverse, // then the entire group does not have one if (g == null) return null; group.Children.Add(g); } return group; } } ////// Returns a best effort affine transform /// internal override Transform3D AffineTransform { [FriendAccessAllowed] // Built into Core, also used by Framework. get { // cache the children to avoid a repeated DP access GeneralTransform3DCollection children = Children; if ((children == null) || (children.Count == 0)) { return null; } Matrix3D matrix = Matrix3D.Identity; for (int i = 0, count = children.Count; i < count; i++) { Transform3D t = children._collection[i].AffineTransform; t.Append(ref matrix); } return new MatrixTransform3D(matrix); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation, 2001 // // File: GeneralTransform3DGroup.cs //----------------------------------------------------------------------------- using System; using System.Windows; using System.Windows.Media; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Reflection; using System.Collections; using System.Collections.Generic; using MS.Internal; using System.Windows.Media.Animation; using System.Globalization; using System.Text; using System.Runtime.InteropServices; using System.Windows.Markup; using System.Windows.Media.Composition; using System.Diagnostics; using MS.Internal.PresentationCore; using SR = MS.Internal.PresentationCore.SR; using SRID = MS.Internal.PresentationCore.SRID; namespace System.Windows.Media.Media3D { ////// GeneralTransform3D group /// [ContentProperty("Children")] [Localizability(LocalizationCategory.None, Readability = Readability.Unreadable)] public sealed partial class GeneralTransform3DGroup : GeneralTransform3D { #region Constructors ////// Default Constructor /// public GeneralTransform3DGroup() { } #endregion ////// Transform a point /// /// input point /// output point ///True if the point is transformed successfully public override bool TryTransform(Point3D inPoint, out Point3D result) { result = inPoint; // cache the children to avoid a repeated DP access GeneralTransform3DCollection children = Children; if ((children == null) || (children.Count == 0)) { return false; } bool pointTransformed = true; // transform the point through each of the transforms for (int i = 0, count = children.Count; i < count; i++) { if (children._collection[i].TryTransform(inPoint, out result) == false) { pointTransformed = false; break; } inPoint = result; } return pointTransformed; } ////// Transforms the bounding box to the smallest axis aligned bounding box /// that contains all the points in the original bounding box /// /// Input bounding rect ///Transformed bounding rect public override Rect3D TransformBounds(Rect3D rect) { // cache the children to avoid a repeated DP access GeneralTransform3DCollection children = Children; if ((children == null) || (children.Count == 0)) { return rect; } Rect3D result = rect; for (int i = 0, count = children.Count; i < count; i++) { result = children._collection[i].TransformBounds(result); } return result; } ////// Returns the inverse transform if it has an inverse, null otherwise /// public override GeneralTransform3D Inverse { get { // cache the children to avoid a repeated DP access GeneralTransform3DCollection children = Children; if ((children == null) || (children.Count == 0)) { return null; } GeneralTransform3DGroup group = new GeneralTransform3DGroup(); for (int i = children.Count - 1; i >= 0; i--) { GeneralTransform3D g = children._collection[i].Inverse; // if any of the transforms does not have an inverse, // then the entire group does not have one if (g == null) return null; group.Children.Add(g); } return group; } } ////// Returns a best effort affine transform /// internal override Transform3D AffineTransform { [FriendAccessAllowed] // Built into Core, also used by Framework. get { // cache the children to avoid a repeated DP access GeneralTransform3DCollection children = Children; if ((children == null) || (children.Count == 0)) { return null; } Matrix3D matrix = Matrix3D.Identity; for (int i = 0, count = children.Count; i < count; i++) { Transform3D t = children._collection[i].AffineTransform; t.Append(ref matrix); } return new MatrixTransform3D(matrix); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
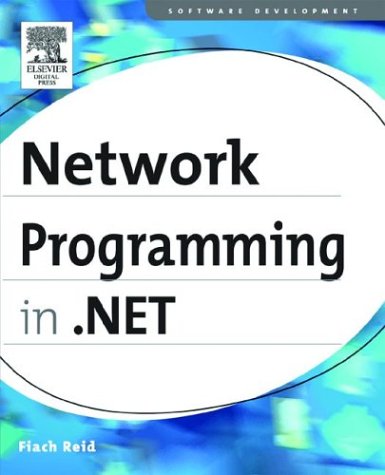
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TemplateBindingExtensionConverter.cs
- CompressStream.cs
- NetCodeGroup.cs
- FixedSOMFixedBlock.cs
- ListCollectionView.cs
- CookieHandler.cs
- TabRenderer.cs
- BooleanFacetDescriptionElement.cs
- figurelengthconverter.cs
- FlowDocumentReaderAutomationPeer.cs
- OrderedDictionary.cs
- DataSysAttribute.cs
- TagElement.cs
- PathSegmentCollection.cs
- UserControlCodeDomTreeGenerator.cs
- EpmSourcePathSegment.cs
- HitTestWithPointDrawingContextWalker.cs
- RpcAsyncResult.cs
- TypeDescriptionProviderAttribute.cs
- TemplateBamlRecordReader.cs
- HwndSource.cs
- AuthStoreRoleProvider.cs
- FamilyMapCollection.cs
- SubpageParaClient.cs
- TextInfo.cs
- SiteMapDesignerDataSourceView.cs
- XmlConvert.cs
- UnsignedPublishLicense.cs
- XXXOnTypeBuilderInstantiation.cs
- FloaterParagraph.cs
- FileDialogCustomPlace.cs
- Page.cs
- CodeExpressionCollection.cs
- FieldTemplateFactory.cs
- QuaternionRotation3D.cs
- OptimalBreakSession.cs
- CodeGroup.cs
- MDIClient.cs
- ItemCollection.cs
- ConnectionStringsExpressionBuilder.cs
- PrimitiveDataContract.cs
- TemplateBindingExpressionConverter.cs
- PeerDefaultCustomResolverClient.cs
- DbMetaDataFactory.cs
- SiteMapProvider.cs
- NullableLongMinMaxAggregationOperator.cs
- CommandCollectionEditor.cs
- XmlnsDictionary.cs
- NotifyIcon.cs
- Schema.cs
- DataGridPageChangedEventArgs.cs
- ListViewEditEventArgs.cs
- dtdvalidator.cs
- ObjectViewListener.cs
- WindowsFormsSynchronizationContext.cs
- namescope.cs
- Debugger.cs
- MessageContractAttribute.cs
- RepeatButtonAutomationPeer.cs
- SafeNativeMethods.cs
- SmiEventSink_Default.cs
- MemberAssignment.cs
- ListViewAutomationPeer.cs
- ListViewCommandEventArgs.cs
- FlowDocumentScrollViewerAutomationPeer.cs
- Array.cs
- NominalTypeEliminator.cs
- DateTimePicker.cs
- XmlDataSourceView.cs
- ImageField.cs
- XmlNamespaceManager.cs
- ParamArrayAttribute.cs
- SemanticBasicElement.cs
- ResourceDescriptionAttribute.cs
- TempFiles.cs
- DSASignatureDeformatter.cs
- ImageListUtils.cs
- TypeAccessException.cs
- LinqDataSourceSelectEventArgs.cs
- RangeContentEnumerator.cs
- PrimitiveList.cs
- AsymmetricSignatureDeformatter.cs
- RegionIterator.cs
- DtrList.cs
- BrowserDefinition.cs
- FixedPageProcessor.cs
- DbConvert.cs
- ContextProperty.cs
- SpecialTypeDataContract.cs
- UserControlBuildProvider.cs
- StateMachineSubscriptionManager.cs
- InstanceCompleteException.cs
- XmlSchemaInferenceException.cs
- ListenDesigner.cs
- MethodInfo.cs
- MULTI_QI.cs
- StyleXamlTreeBuilder.cs
- XmlSchemaImporter.cs
- PageContent.cs
- ParserExtension.cs