Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Core / CSharp / System / Windows / Media / Animation / AnimationClockResource.cs / 1 / AnimationClockResource.cs
//---------------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // File: AnimationClockResource.cs // // Description: This file contains the implementation of AnimationClockResource. // An AnimationClockResource is used to tie together an AnimationClock // and a base value as a DUCE resource. This base class provides // Changed Events. // // History: // 04/29/2004 : adsmith - Created it. // //--------------------------------------------------------------------------- using System; using System.Windows; using System.Windows.Media; using System.Windows.Media.Composition; using System.Diagnostics; using System.Runtime.InteropServices; namespace System.Windows.Media.Animation { ////// AnimationClockResource class. /// AnimationClockResource classes refer to an AnimationClock and a base /// value. They implement DUCE.IResource, and thus can be used to produce /// a render-side resource which represents the current value of this /// AnimationClock. /// They subscribe to the Changed event on the AnimationClock and ensure /// that the resource's current value is up to date. /// internal abstract class AnimationClockResource: DUCE.IResource { ////// Protected constructor for AnimationClockResource. /// The derived class must provide a created duceResource. /// /// The AnimationClock for this resource. Can be null. protected AnimationClockResource(AnimationClock animationClock) { _animationClock = animationClock; if (_animationClock != null) { _animationClock.CurrentTimeInvalidated += new EventHandler(OnChanged); } } #region Public Properties ////// AnimationClock - accessor for the AnimationClock. /// public AnimationClock AnimationClock { get { return _animationClock; } } #endregion Public Properties ////// OnChanged - this is fired if any dependents change. /// In this case, that means the Clock, which means we can (and do) assert that the Clock isn't null. /// /// object - the origin of the change. /// EventArgs - ignored. protected void OnChanged(object sender, EventArgs args) { Debug.Assert(sender as System.Windows.Threading.DispatcherObject != null); Debug.Assert(((System.Windows.Threading.DispatcherObject)sender).Dispatcher != null); Debug.Assert(_animationClock != null); System.Windows.Threading.Dispatcher dispatcher = ((System.Windows.Threading.DispatcherObject)sender).Dispatcher; MediaContext mediaContext = MediaContext.From(dispatcher); DUCE.Channel channel = mediaContext.Channel; // Only register for an update if this resource is currently on channel and // isn't already registered. if (!IsResourceInvalid && _duceResource.IsOnAnyChannel) { // Add this handler to this event means that the handler will be // called on the next UIThread render for this Dispatcher. mediaContext.ResourcesUpdated += new MediaContext.ResourcesUpdatedHandler(UpdateResourceFromMediaContext); IsResourceInvalid = true; } } ////// Propagagtes handler to the _animationClock. /// /// /// EventHandler - the EventHandle to associate with the mutable dependents. /// /// bool - if true, we're adding the new handler, if false we're removing it. internal virtual void PropagateChangedHandlersCore(EventHandler handler, bool adding) { // Nothing to do if the clock is null. if (_animationClock != null) { if (adding) { _animationClock.CurrentTimeInvalidated += handler; } else { _animationClock.CurrentTimeInvalidated -= handler; } } } #region DUCE ////// UpdateResourceFromMediaContext - this is called by the MediaContext /// to validate the render-thread resource. /// Sender is the MediaContext. /// private void UpdateResourceFromMediaContext(DUCE.Channel channel, bool skipOnChannelCheck) { // If we're told we can skip the channel check, then we must be on channel Debug.Assert(!skipOnChannelCheck || _duceResource.IsOnChannel(channel)); // Check to see if we're on the channel and if we're invalid. // Only perform the update if this resource is currently on channel. if (IsResourceInvalid && (skipOnChannelCheck || _duceResource.IsOnChannel(channel))) { UpdateResource(_duceResource.GetHandle(channel), channel); // This resource is now valid. IsResourceInvalid = false; } } ////// UpdateResource - This method is called to update the render-thread /// resource on a given channel. /// /// The DUCE.ResourceHandle for this resource on this channel. /// The channel on which to update the render-thread resource. protected abstract void UpdateResource(DUCE.ResourceHandle handle, DUCE.Channel channel); DUCE.ResourceHandle DUCE.IResource.AddRefOnChannel(DUCE.Channel channel) { using (CompositionEngineLock.Acquire()) { if (_duceResource.CreateOrAddRefOnChannel(channel, ResourceType)) { UpdateResource(_duceResource.GetHandle(channel), channel); } return _duceResource.GetHandle(channel); } } void DUCE.IResource.ReleaseOnChannel(DUCE.Channel channel) { using (CompositionEngineLock.Acquire()) { Debug.Assert(_duceResource.IsOnChannel(channel)); _duceResource.ReleaseOnChannel(channel); } } DUCE.ResourceHandle DUCE.IResource.GetHandle(DUCE.Channel channel) { DUCE.ResourceHandle handle; // Reconsider the need for this lock when removing the MultiChannelResource. using (CompositionEngineLock.Acquire()) { // This method is a short cut and must only be called while the ref count // of this resource on this channel is non-zero. Thus we assert that this // resource is already on this channel. Debug.Assert(_duceResource.IsOnChannel(channel)); handle = _duceResource.GetHandle(channel); } return handle; } int DUCE.IResource.GetChannelCount() { return _duceResource.GetChannelCount(); } DUCE.Channel DUCE.IResource.GetChannel(int index) { return _duceResource.GetChannel(index); } ////// This is only implemented by Visual and Visual3D. /// void DUCE.IResource.RemoveChildFromParent(DUCE.IResource parent, DUCE.Channel channel) { throw new NotImplementedException(); } ////// This is only implemented by Visual and Visual3D. /// DUCE.ResourceHandle DUCE.IResource.Get3DHandle(DUCE.Channel channel) { throw new NotImplementedException(); } #endregion DUCE #region Protected Method protected bool IsResourceInvalid { get { return _isResourceInvalid; } set { _isResourceInvalid = value; } } // // Method which returns the DUCE type of this class. // The base class needs this type when calling CreateOrAddRefOnChannel. // By providing this via a virtual, we avoid a per-instance storage cost. // protected abstract DUCE.ResourceType ResourceType { get; } #endregion Protected Method // DUCE resource // It is provided via the constructor. private DUCE.MultiChannelResource _duceResource = new DUCE.MultiChannelResource(); // This bool keeps track of whether or not this resource is valid // on its channel. private bool _isResourceInvalid; // This AnimationClock is the animation associated with this resource. // It is provided via the constructor. protected AnimationClock _animationClock; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // File: AnimationClockResource.cs // // Description: This file contains the implementation of AnimationClockResource. // An AnimationClockResource is used to tie together an AnimationClock // and a base value as a DUCE resource. This base class provides // Changed Events. // // History: // 04/29/2004 : adsmith - Created it. // //--------------------------------------------------------------------------- using System; using System.Windows; using System.Windows.Media; using System.Windows.Media.Composition; using System.Diagnostics; using System.Runtime.InteropServices; namespace System.Windows.Media.Animation { ////// AnimationClockResource class. /// AnimationClockResource classes refer to an AnimationClock and a base /// value. They implement DUCE.IResource, and thus can be used to produce /// a render-side resource which represents the current value of this /// AnimationClock. /// They subscribe to the Changed event on the AnimationClock and ensure /// that the resource's current value is up to date. /// internal abstract class AnimationClockResource: DUCE.IResource { ////// Protected constructor for AnimationClockResource. /// The derived class must provide a created duceResource. /// /// The AnimationClock for this resource. Can be null. protected AnimationClockResource(AnimationClock animationClock) { _animationClock = animationClock; if (_animationClock != null) { _animationClock.CurrentTimeInvalidated += new EventHandler(OnChanged); } } #region Public Properties ////// AnimationClock - accessor for the AnimationClock. /// public AnimationClock AnimationClock { get { return _animationClock; } } #endregion Public Properties ////// OnChanged - this is fired if any dependents change. /// In this case, that means the Clock, which means we can (and do) assert that the Clock isn't null. /// /// object - the origin of the change. /// EventArgs - ignored. protected void OnChanged(object sender, EventArgs args) { Debug.Assert(sender as System.Windows.Threading.DispatcherObject != null); Debug.Assert(((System.Windows.Threading.DispatcherObject)sender).Dispatcher != null); Debug.Assert(_animationClock != null); System.Windows.Threading.Dispatcher dispatcher = ((System.Windows.Threading.DispatcherObject)sender).Dispatcher; MediaContext mediaContext = MediaContext.From(dispatcher); DUCE.Channel channel = mediaContext.Channel; // Only register for an update if this resource is currently on channel and // isn't already registered. if (!IsResourceInvalid && _duceResource.IsOnAnyChannel) { // Add this handler to this event means that the handler will be // called on the next UIThread render for this Dispatcher. mediaContext.ResourcesUpdated += new MediaContext.ResourcesUpdatedHandler(UpdateResourceFromMediaContext); IsResourceInvalid = true; } } ////// Propagagtes handler to the _animationClock. /// /// /// EventHandler - the EventHandle to associate with the mutable dependents. /// /// bool - if true, we're adding the new handler, if false we're removing it. internal virtual void PropagateChangedHandlersCore(EventHandler handler, bool adding) { // Nothing to do if the clock is null. if (_animationClock != null) { if (adding) { _animationClock.CurrentTimeInvalidated += handler; } else { _animationClock.CurrentTimeInvalidated -= handler; } } } #region DUCE ////// UpdateResourceFromMediaContext - this is called by the MediaContext /// to validate the render-thread resource. /// Sender is the MediaContext. /// private void UpdateResourceFromMediaContext(DUCE.Channel channel, bool skipOnChannelCheck) { // If we're told we can skip the channel check, then we must be on channel Debug.Assert(!skipOnChannelCheck || _duceResource.IsOnChannel(channel)); // Check to see if we're on the channel and if we're invalid. // Only perform the update if this resource is currently on channel. if (IsResourceInvalid && (skipOnChannelCheck || _duceResource.IsOnChannel(channel))) { UpdateResource(_duceResource.GetHandle(channel), channel); // This resource is now valid. IsResourceInvalid = false; } } ////// UpdateResource - This method is called to update the render-thread /// resource on a given channel. /// /// The DUCE.ResourceHandle for this resource on this channel. /// The channel on which to update the render-thread resource. protected abstract void UpdateResource(DUCE.ResourceHandle handle, DUCE.Channel channel); DUCE.ResourceHandle DUCE.IResource.AddRefOnChannel(DUCE.Channel channel) { using (CompositionEngineLock.Acquire()) { if (_duceResource.CreateOrAddRefOnChannel(channel, ResourceType)) { UpdateResource(_duceResource.GetHandle(channel), channel); } return _duceResource.GetHandle(channel); } } void DUCE.IResource.ReleaseOnChannel(DUCE.Channel channel) { using (CompositionEngineLock.Acquire()) { Debug.Assert(_duceResource.IsOnChannel(channel)); _duceResource.ReleaseOnChannel(channel); } } DUCE.ResourceHandle DUCE.IResource.GetHandle(DUCE.Channel channel) { DUCE.ResourceHandle handle; // Reconsider the need for this lock when removing the MultiChannelResource. using (CompositionEngineLock.Acquire()) { // This method is a short cut and must only be called while the ref count // of this resource on this channel is non-zero. Thus we assert that this // resource is already on this channel. Debug.Assert(_duceResource.IsOnChannel(channel)); handle = _duceResource.GetHandle(channel); } return handle; } int DUCE.IResource.GetChannelCount() { return _duceResource.GetChannelCount(); } DUCE.Channel DUCE.IResource.GetChannel(int index) { return _duceResource.GetChannel(index); } ////// This is only implemented by Visual and Visual3D. /// void DUCE.IResource.RemoveChildFromParent(DUCE.IResource parent, DUCE.Channel channel) { throw new NotImplementedException(); } ////// This is only implemented by Visual and Visual3D. /// DUCE.ResourceHandle DUCE.IResource.Get3DHandle(DUCE.Channel channel) { throw new NotImplementedException(); } #endregion DUCE #region Protected Method protected bool IsResourceInvalid { get { return _isResourceInvalid; } set { _isResourceInvalid = value; } } // // Method which returns the DUCE type of this class. // The base class needs this type when calling CreateOrAddRefOnChannel. // By providing this via a virtual, we avoid a per-instance storage cost. // protected abstract DUCE.ResourceType ResourceType { get; } #endregion Protected Method // DUCE resource // It is provided via the constructor. private DUCE.MultiChannelResource _duceResource = new DUCE.MultiChannelResource(); // This bool keeps track of whether or not this resource is valid // on its channel. private bool _isResourceInvalid; // This AnimationClock is the animation associated with this resource. // It is provided via the constructor. protected AnimationClock _animationClock; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
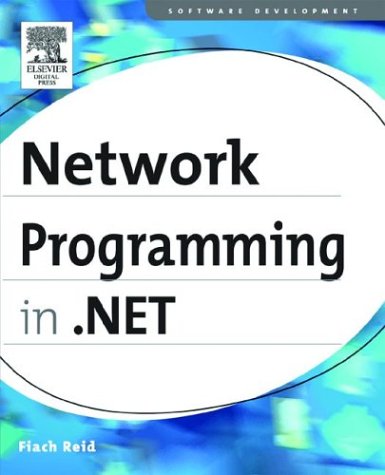
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DbProviderServices.cs
- DifferencingCollection.cs
- CharacterShapingProperties.cs
- XhtmlBasicControlAdapter.cs
- TypographyProperties.cs
- _Win32.cs
- CrossContextChannel.cs
- EntitySqlQueryCacheEntry.cs
- WebBrowsableAttribute.cs
- UInt64.cs
- ImpersonateTokenRef.cs
- DataBinding.cs
- InfoCardProofToken.cs
- DotExpr.cs
- LinqDataSourceContextEventArgs.cs
- DateRangeEvent.cs
- RIPEMD160Managed.cs
- TextWriter.cs
- BufferedGraphics.cs
- SID.cs
- ClickablePoint.cs
- SqlDataRecord.cs
- AmbientLight.cs
- TimeSpanConverter.cs
- InputLanguageProfileNotifySink.cs
- EntityDataSourceValidationException.cs
- VirtualPath.cs
- ColumnMapProcessor.cs
- PathSegment.cs
- RegexInterpreter.cs
- BinaryObjectInfo.cs
- SiteMapNode.cs
- CompositeTypefaceMetrics.cs
- ItemContainerGenerator.cs
- UnsafeNativeMethods.cs
- DbConnectionPoolGroup.cs
- TextBox.cs
- UInt32Storage.cs
- Thread.cs
- FacetValues.cs
- XmlSortKey.cs
- LogExtent.cs
- ZipPackagePart.cs
- CollectionChangeEventArgs.cs
- XmlSignatureProperties.cs
- DataBinder.cs
- UInt32Converter.cs
- VirtualDirectoryMappingCollection.cs
- WebPartCloseVerb.cs
- RightNameExpirationInfoPair.cs
- FixedPosition.cs
- EndEvent.cs
- DataGridViewControlCollection.cs
- RequestQueue.cs
- ExceptionList.cs
- AnchoredBlock.cs
- SignedInfo.cs
- FrameworkPropertyMetadata.cs
- RuntimeHelpers.cs
- Closure.cs
- ContractTypeNameElement.cs
- TrackingSection.cs
- RepeatInfo.cs
- TrustSection.cs
- TemplatedAdorner.cs
- HttpModuleAction.cs
- TypefaceMetricsCache.cs
- BeginStoryboard.cs
- Condition.cs
- CellParaClient.cs
- SqlAliasesReferenced.cs
- CollectionMarkupSerializer.cs
- DataGridPagerStyle.cs
- XLinq.cs
- WSTrust.cs
- DataGridPagingPage.cs
- HttpListenerException.cs
- FontInfo.cs
- SerializationInfo.cs
- StreamGeometryContext.cs
- RefType.cs
- securestring.cs
- ConfigXmlSignificantWhitespace.cs
- CompatibleIComparer.cs
- DataGridColumnCollection.cs
- AspNetCacheProfileAttribute.cs
- CompilerErrorCollection.cs
- MonitoringDescriptionAttribute.cs
- CollectionViewGroupRoot.cs
- AnnotationMap.cs
- ControlValuePropertyAttribute.cs
- EmptyStringExpandableObjectConverter.cs
- DefaultValueAttribute.cs
- InstanceDataCollection.cs
- ToolStripDesignerUtils.cs
- ServiceProviders.cs
- SecurityUtils.cs
- HtmlTitle.cs
- DesignerVerbToolStripMenuItem.cs
- SByte.cs