Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / fx / src / CompMod / System / Diagnostics / TraceUtils.cs / 1 / TraceUtils.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- using System.Configuration; using System; using System.IO; using System.Reflection; using System.Globalization; using System.Collections; using System.Collections.Specialized; namespace System.Diagnostics { internal static class TraceUtils { internal static object GetRuntimeObject(string className, Type baseType, string initializeData) { Object newObject = null; Type objectType = null; if (className.Length == 0) { throw new ConfigurationErrorsException(SR.GetString(SR.EmptyTypeName_NotAllowed)); } objectType = Type.GetType(className); if (objectType == null) { throw new ConfigurationErrorsException(SR.GetString(SR.Could_not_find_type, className)); } if (!baseType.IsAssignableFrom(objectType)) throw new ConfigurationErrorsException(SR.GetString(SR.Incorrect_base_type, className, baseType.FullName)); Exception innerException = null; try { if (String.IsNullOrEmpty(initializeData)) { if (IsOwnedTextWriterTL(objectType)) throw new ConfigurationErrorsException(SR.GetString(SR.TextWriterTL_DefaultConstructor_NotSupported)); // create an object with parameterless constructor ConstructorInfo ctorInfo = objectType.GetConstructor(new Type[] {}); if (ctorInfo == null) throw new ConfigurationErrorsException(SR.GetString(SR.Could_not_get_constructor, className)); newObject = ctorInfo.Invoke(new object[] {}); } else { // create an object with a one-string constructor // first look for a string constructor ConstructorInfo ctorInfo = objectType.GetConstructor(new Type[] { typeof(string) }); if (ctorInfo != null) { // Special case to enable specifying relative path to trace file from config for // our own TextWriterTraceListener derivatives. We will prepend it with fullpath // prefix from config file location if (IsOwnedTextWriterTL(objectType)) { if ((initializeData[0] != Path.DirectorySeparatorChar) && (initializeData[0] != Path.AltDirectorySeparatorChar) && !Path.IsPathRooted(initializeData)) { string filePath = DiagnosticsConfiguration.ConfigFilePath; if (!String.IsNullOrEmpty(filePath)) { string dirPath = Path.GetDirectoryName(filePath); if (dirPath != null) initializeData = Path.Combine(dirPath, initializeData); } } } newObject = ctorInfo.Invoke(new object[] { initializeData }); } else { // now look for another 1 param constructor. ConstructorInfo[] ctorInfos = objectType.GetConstructors(); if (ctorInfos == null) throw new ConfigurationErrorsException(SR.GetString(SR.Could_not_get_constructor, className)); for (int i=0; i// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- using System.Configuration; using System; using System.IO; using System.Reflection; using System.Globalization; using System.Collections; using System.Collections.Specialized; namespace System.Diagnostics { internal static class TraceUtils { internal static object GetRuntimeObject(string className, Type baseType, string initializeData) { Object newObject = null; Type objectType = null; if (className.Length == 0) { throw new ConfigurationErrorsException(SR.GetString(SR.EmptyTypeName_NotAllowed)); } objectType = Type.GetType(className); if (objectType == null) { throw new ConfigurationErrorsException(SR.GetString(SR.Could_not_find_type, className)); } if (!baseType.IsAssignableFrom(objectType)) throw new ConfigurationErrorsException(SR.GetString(SR.Incorrect_base_type, className, baseType.FullName)); Exception innerException = null; try { if (String.IsNullOrEmpty(initializeData)) { if (IsOwnedTextWriterTL(objectType)) throw new ConfigurationErrorsException(SR.GetString(SR.TextWriterTL_DefaultConstructor_NotSupported)); // create an object with parameterless constructor ConstructorInfo ctorInfo = objectType.GetConstructor(new Type[] {}); if (ctorInfo == null) throw new ConfigurationErrorsException(SR.GetString(SR.Could_not_get_constructor, className)); newObject = ctorInfo.Invoke(new object[] {}); } else { // create an object with a one-string constructor // first look for a string constructor ConstructorInfo ctorInfo = objectType.GetConstructor(new Type[] { typeof(string) }); if (ctorInfo != null) { // Special case to enable specifying relative path to trace file from config for // our own TextWriterTraceListener derivatives. We will prepend it with fullpath // prefix from config file location if (IsOwnedTextWriterTL(objectType)) { if ((initializeData[0] != Path.DirectorySeparatorChar) && (initializeData[0] != Path.AltDirectorySeparatorChar) && !Path.IsPathRooted(initializeData)) { string filePath = DiagnosticsConfiguration.ConfigFilePath; if (!String.IsNullOrEmpty(filePath)) { string dirPath = Path.GetDirectoryName(filePath); if (dirPath != null) initializeData = Path.Combine(dirPath, initializeData); } } } newObject = ctorInfo.Invoke(new object[] { initializeData }); } else { // now look for another 1 param constructor. ConstructorInfo[] ctorInfos = objectType.GetConstructors(); if (ctorInfos == null) throw new ConfigurationErrorsException(SR.GetString(SR.Could_not_get_constructor, className)); for (int i=0; i
Link Menu
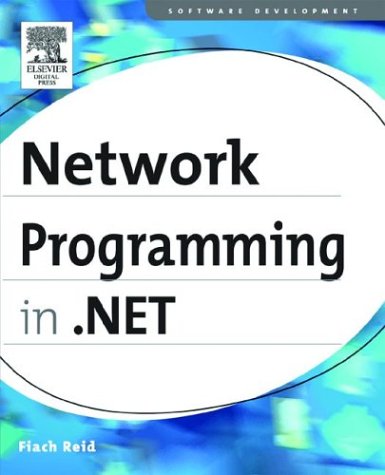
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- RedistVersionInfo.cs
- StaticFileHandler.cs
- ChildrenQuery.cs
- AssemblyAttributes.cs
- TextBoxBaseDesigner.cs
- WsatStrings.cs
- QilCloneVisitor.cs
- Size3DValueSerializer.cs
- WebPartConnectionCollection.cs
- InternalUserCancelledException.cs
- HttpListenerContext.cs
- MimeBasePart.cs
- AmbientValueAttribute.cs
- ListChunk.cs
- ValidationSummary.cs
- CapabilitiesSection.cs
- DefaultTypeArgumentAttribute.cs
- StickyNoteAnnotations.cs
- IRCollection.cs
- StrongNameUtility.cs
- SinglePhaseEnlistment.cs
- NullableDoubleMinMaxAggregationOperator.cs
- TriggerBase.cs
- LinkClickEvent.cs
- EntityContainerRelationshipSetEnd.cs
- HeaderCollection.cs
- ActivityBindForm.cs
- Ipv6Element.cs
- CodeObjectCreateExpression.cs
- basecomparevalidator.cs
- PrintController.cs
- MethodCallExpression.cs
- XmlReturnReader.cs
- SHA512Managed.cs
- SynchronizingStream.cs
- EntityContainerEntitySet.cs
- LinkedDataMemberFieldEditor.cs
- PathSegment.cs
- QuadraticBezierSegment.cs
- TransformProviderWrapper.cs
- DiagnosticsConfigurationHandler.cs
- ComponentConverter.cs
- CryptoProvider.cs
- CategoriesDocumentFormatter.cs
- LocalizableResourceBuilder.cs
- DataRecordObjectView.cs
- ConstraintStruct.cs
- BuildProvider.cs
- MemberDescriptor.cs
- WebPartTransformer.cs
- RealProxy.cs
- EmptyControlCollection.cs
- RangeValueProviderWrapper.cs
- InputEventArgs.cs
- ChineseLunisolarCalendar.cs
- XmlSchema.cs
- RawMouseInputReport.cs
- XmlChoiceIdentifierAttribute.cs
- FrameworkElementAutomationPeer.cs
- GenericWebPart.cs
- ReaderOutput.cs
- ProviderConnectionPoint.cs
- Vector3DKeyFrameCollection.cs
- CacheForPrimitiveTypes.cs
- TextWriterEngine.cs
- StringAnimationUsingKeyFrames.cs
- BaseDataListComponentEditor.cs
- NamespaceEmitter.cs
- DbProviderConfigurationHandler.cs
- CodeExporter.cs
- Transform.cs
- ListViewItemSelectionChangedEvent.cs
- SortedDictionary.cs
- MissingSatelliteAssemblyException.cs
- ClientSponsor.cs
- DataGridViewColumnTypePicker.cs
- DataGridViewRowsAddedEventArgs.cs
- ThicknessAnimationUsingKeyFrames.cs
- DataGridViewColumnConverter.cs
- HotSpot.cs
- HMACMD5.cs
- tooltip.cs
- BitmapEffectInputConnector.cs
- ToolboxItemAttribute.cs
- FastPropertyAccessor.cs
- SwitchExpression.cs
- DbgCompiler.cs
- HttpResponse.cs
- MetabaseServerConfig.cs
- HttpPostProtocolImporter.cs
- DebuggerService.cs
- InputLanguageSource.cs
- ContentDisposition.cs
- MeshGeometry3D.cs
- DeploymentExceptionMapper.cs
- GenericsInstances.cs
- ColumnHeader.cs
- OdbcConnectionFactory.cs
- IpcServerChannel.cs
- OpenTypeLayout.cs