Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / ndp / fx / src / DataEntityDesign / Design / System / Data / Entity / Design / SSDLGenerator / FunctionDetailsReader.cs / 3 / FunctionDetailsReader.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Text; using System.Data.Common; using System.Data.EntityClient; using System.Diagnostics; using System.Data.Metadata.Edm; namespace System.Data.Entity.Design.SsdlGenerator { // the purpose of this class is to give us strongly typed access to // the results of the reader // // NOTE this class will dispose of the command when the reader is disposed // internal class FunctionDetailsReader : IDisposable { private DbDataReader _reader; private EntityCommand _command; private EntityConnection _connection; private object[] _currentRow; internal FunctionDetailsReader(FunctionDetailsReader.Memento memento) { _currentRow = memento.Values; } internal FunctionDetailsReader(EntityConnection connection, IEnumerablefilters) { Debug.Assert(connection != null, "the parameter connection is null"); Debug.Assert(connection.State == ConnectionState.Open, "the connection is not Open"); _connection = connection; _command = EntityStoreSchemaGeneratorDatabaseSchemaLoader.CreateFilteredCommand( _connection, FunctionDetailSql, FunctionOrderByClause, EntityStoreSchemaFilterObjectTypes.Function, new List (filters), new string [] {FunctionDetailAlias}); _reader = _command.ExecuteReader(CommandBehavior.SequentialAccess); } internal bool Read() { Debug.Assert(_reader != null, "don't Read() when it is created from a memento"); bool haveRow = _reader.Read(); if (haveRow) { if(_currentRow == null) { _currentRow = new object[COLUMN_COUNT]; } _reader.GetValues(_currentRow); } else { _currentRow = null; } return haveRow; } public void Dispose() { Debug.Assert(_reader != null, "don't Dispose() when it is created from a memento"); _reader.Dispose(); _command.Dispose(); } internal void Attach(Memento memento) { Debug.Assert(memento != null, "the parameter memento is null"); Debug.Assert(memento.Values != null, "the values in the memento are null"); Debug.Assert(_reader == null, "don't attach to a real reader"); _currentRow = memento.Values; } const int PROC_SCHEMA_INDEX = 0; const int PROC_NAME_INDEX = 1; const int PROC_RET_TYPE_INDEX = 2; const int PROC_ISAGGREGATE_INDEX = 3; const int PROC_ISCOMPOSABLE_INDEX = 4; const int PROC_ISBUILTIN_INDEX = 5; const int PROC_ISNILADIC_INDEX = 6; const int PARAM_NAME_INDEX = 7; const int PARAM_TYPE_INDEX = 8; const int PARAM_DIRECTION_INDEX = 9; const int COLUMN_COUNT = 10; internal bool IsSchemaNull { get { return Convert.IsDBNull(_currentRow[PROC_SCHEMA_INDEX]); } } internal string Schema { get { return (string)_currentRow[PROC_SCHEMA_INDEX]; } } internal string ProcedureName { get { return (string)_currentRow[PROC_NAME_INDEX]; } } internal string ReturnType { get { return (string)_currentRow[PROC_RET_TYPE_INDEX]; } } internal bool IsReturnTypeNull { get {return Convert.IsDBNull(_currentRow[PROC_RET_TYPE_INDEX]);} } internal bool IsIsAggregate { get { if(Convert.IsDBNull(_currentRow[PROC_ISAGGREGATE_INDEX])) return false; return (bool)_currentRow[PROC_ISAGGREGATE_INDEX]; } } internal bool IsBuiltIn { get { if (Convert.IsDBNull(_currentRow[PROC_ISBUILTIN_INDEX])) return false; return (bool)_currentRow[PROC_ISBUILTIN_INDEX]; } } internal bool IsComposable { get { if(Convert.IsDBNull(_currentRow[PROC_ISCOMPOSABLE_INDEX])) return false; return (bool)_currentRow[PROC_ISCOMPOSABLE_INDEX]; } } internal bool IsNiladic { get { if(Convert.IsDBNull(_currentRow[PROC_ISNILADIC_INDEX])) return false; return (bool)_currentRow[PROC_ISNILADIC_INDEX]; } } internal string ParameterName { get { return (string)_currentRow[PARAM_NAME_INDEX]; } } internal bool IsParameterNameNull { get {return Convert.IsDBNull(_currentRow[PARAM_TYPE_INDEX]);} } internal string ParameterType { get { return (string)_currentRow[PARAM_TYPE_INDEX]; } } internal bool IsParameterTypeNull { get {return Convert.IsDBNull(_currentRow[PARAM_TYPE_INDEX]);} } internal string ProcParameterMode { get { return (string)_currentRow[PARAM_DIRECTION_INDEX]; } } internal bool IsParameterModeNull { get { return Convert.IsDBNull(_currentRow[PARAM_DIRECTION_INDEX]); } } internal bool TryGetParameterMode(out ParameterMode mode) { if (IsParameterModeNull) { mode = (ParameterMode)(-1); return false; } switch(ProcParameterMode) { case "IN": mode = ParameterMode.In; return true; case "OUT": mode = ParameterMode.Out; return true; case "INOUT": mode = ParameterMode.InOut; return true; default: mode = (ParameterMode)(-1); return false; } } internal EntityStoreSchemaGenerator.DbObjectKey CreateDbObjectKey() { Debug.Assert(_currentRow != null, "don't call this method when you not reading"); return new EntityStoreSchemaGenerator.DbObjectKey(null, _currentRow[PROC_SCHEMA_INDEX], _currentRow[PROC_NAME_INDEX], EntityStoreSchemaGenerator.DbObjectType.Function); } internal Memento CreateMemento() { Debug.Assert(_currentRow != null, "don't call this method when you not reading"); return new Memento((object[])_currentRow.Clone()); } private static readonly string FunctionDetailAlias = "sp"; private static readonly string FunctionDetailSql = @" SELECT sp.SchemaName , sp.Name , sp.ReturnTypeName , sp.IsAggregate , sp.IsComposable , sp.IsBuiltIn , sp.IsNiladic , sp.ParameterName , sp.ParameterType , sp.Mode FROM ( (SELECT r.CatalogName as CatalogName , r.SchemaName as SchemaName , r.Name as Name , r.ReturnType.TypeName as ReturnTypeName , r.IsAggregate as IsAggregate , true as IsComposable , r.IsBuiltIn as IsBuiltIn , r.IsNiladic as IsNiladic , p.Name as ParameterName , p.ParameterType.TypeName as ParameterType , p.Mode as Mode , p.Ordinal as Ordinal FROM OfType(SchemaInformation.Functions, Store.ScalarFunction) as r OUTER APPLY r.Parameters as p) UNION ALL (SELECT r.CatalogName as CatalogName , r.SchemaName as SchemaName , r.Name as Name , CAST(NULL as string) as ReturnTypeName , false as IsAggregate , false as IsComposable , false as IsBuiltIn , false as IsNiladic , p.Name as ParameterName , p.ParameterType.TypeName as ParameterType , p.Mode as Mode , p.Ordinal as Ordinal FROM SchemaInformation.Procedures as r OUTER APPLY r.Parameters as p)) as sp "; private static readonly string FunctionOrderByClause = @" ORDER BY sp.SchemaName , sp.Name , sp.Ordinal "; internal class Memento { private object[] _values; internal Memento(object[] values) { _values = values; } internal object[] Values { get { return _values; } } internal FunctionDetailsReader CreateReader() { return new FunctionDetailsReader(this); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Text; using System.Data.Common; using System.Data.EntityClient; using System.Diagnostics; using System.Data.Metadata.Edm; namespace System.Data.Entity.Design.SsdlGenerator { // the purpose of this class is to give us strongly typed access to // the results of the reader // // NOTE this class will dispose of the command when the reader is disposed // internal class FunctionDetailsReader : IDisposable { private DbDataReader _reader; private EntityCommand _command; private EntityConnection _connection; private object[] _currentRow; internal FunctionDetailsReader(FunctionDetailsReader.Memento memento) { _currentRow = memento.Values; } internal FunctionDetailsReader(EntityConnection connection, IEnumerablefilters) { Debug.Assert(connection != null, "the parameter connection is null"); Debug.Assert(connection.State == ConnectionState.Open, "the connection is not Open"); _connection = connection; _command = EntityStoreSchemaGeneratorDatabaseSchemaLoader.CreateFilteredCommand( _connection, FunctionDetailSql, FunctionOrderByClause, EntityStoreSchemaFilterObjectTypes.Function, new List (filters), new string [] {FunctionDetailAlias}); _reader = _command.ExecuteReader(CommandBehavior.SequentialAccess); } internal bool Read() { Debug.Assert(_reader != null, "don't Read() when it is created from a memento"); bool haveRow = _reader.Read(); if (haveRow) { if(_currentRow == null) { _currentRow = new object[COLUMN_COUNT]; } _reader.GetValues(_currentRow); } else { _currentRow = null; } return haveRow; } public void Dispose() { Debug.Assert(_reader != null, "don't Dispose() when it is created from a memento"); _reader.Dispose(); _command.Dispose(); } internal void Attach(Memento memento) { Debug.Assert(memento != null, "the parameter memento is null"); Debug.Assert(memento.Values != null, "the values in the memento are null"); Debug.Assert(_reader == null, "don't attach to a real reader"); _currentRow = memento.Values; } const int PROC_SCHEMA_INDEX = 0; const int PROC_NAME_INDEX = 1; const int PROC_RET_TYPE_INDEX = 2; const int PROC_ISAGGREGATE_INDEX = 3; const int PROC_ISCOMPOSABLE_INDEX = 4; const int PROC_ISBUILTIN_INDEX = 5; const int PROC_ISNILADIC_INDEX = 6; const int PARAM_NAME_INDEX = 7; const int PARAM_TYPE_INDEX = 8; const int PARAM_DIRECTION_INDEX = 9; const int COLUMN_COUNT = 10; internal bool IsSchemaNull { get { return Convert.IsDBNull(_currentRow[PROC_SCHEMA_INDEX]); } } internal string Schema { get { return (string)_currentRow[PROC_SCHEMA_INDEX]; } } internal string ProcedureName { get { return (string)_currentRow[PROC_NAME_INDEX]; } } internal string ReturnType { get { return (string)_currentRow[PROC_RET_TYPE_INDEX]; } } internal bool IsReturnTypeNull { get {return Convert.IsDBNull(_currentRow[PROC_RET_TYPE_INDEX]);} } internal bool IsIsAggregate { get { if(Convert.IsDBNull(_currentRow[PROC_ISAGGREGATE_INDEX])) return false; return (bool)_currentRow[PROC_ISAGGREGATE_INDEX]; } } internal bool IsBuiltIn { get { if (Convert.IsDBNull(_currentRow[PROC_ISBUILTIN_INDEX])) return false; return (bool)_currentRow[PROC_ISBUILTIN_INDEX]; } } internal bool IsComposable { get { if(Convert.IsDBNull(_currentRow[PROC_ISCOMPOSABLE_INDEX])) return false; return (bool)_currentRow[PROC_ISCOMPOSABLE_INDEX]; } } internal bool IsNiladic { get { if(Convert.IsDBNull(_currentRow[PROC_ISNILADIC_INDEX])) return false; return (bool)_currentRow[PROC_ISNILADIC_INDEX]; } } internal string ParameterName { get { return (string)_currentRow[PARAM_NAME_INDEX]; } } internal bool IsParameterNameNull { get {return Convert.IsDBNull(_currentRow[PARAM_TYPE_INDEX]);} } internal string ParameterType { get { return (string)_currentRow[PARAM_TYPE_INDEX]; } } internal bool IsParameterTypeNull { get {return Convert.IsDBNull(_currentRow[PARAM_TYPE_INDEX]);} } internal string ProcParameterMode { get { return (string)_currentRow[PARAM_DIRECTION_INDEX]; } } internal bool IsParameterModeNull { get { return Convert.IsDBNull(_currentRow[PARAM_DIRECTION_INDEX]); } } internal bool TryGetParameterMode(out ParameterMode mode) { if (IsParameterModeNull) { mode = (ParameterMode)(-1); return false; } switch(ProcParameterMode) { case "IN": mode = ParameterMode.In; return true; case "OUT": mode = ParameterMode.Out; return true; case "INOUT": mode = ParameterMode.InOut; return true; default: mode = (ParameterMode)(-1); return false; } } internal EntityStoreSchemaGenerator.DbObjectKey CreateDbObjectKey() { Debug.Assert(_currentRow != null, "don't call this method when you not reading"); return new EntityStoreSchemaGenerator.DbObjectKey(null, _currentRow[PROC_SCHEMA_INDEX], _currentRow[PROC_NAME_INDEX], EntityStoreSchemaGenerator.DbObjectType.Function); } internal Memento CreateMemento() { Debug.Assert(_currentRow != null, "don't call this method when you not reading"); return new Memento((object[])_currentRow.Clone()); } private static readonly string FunctionDetailAlias = "sp"; private static readonly string FunctionDetailSql = @" SELECT sp.SchemaName , sp.Name , sp.ReturnTypeName , sp.IsAggregate , sp.IsComposable , sp.IsBuiltIn , sp.IsNiladic , sp.ParameterName , sp.ParameterType , sp.Mode FROM ( (SELECT r.CatalogName as CatalogName , r.SchemaName as SchemaName , r.Name as Name , r.ReturnType.TypeName as ReturnTypeName , r.IsAggregate as IsAggregate , true as IsComposable , r.IsBuiltIn as IsBuiltIn , r.IsNiladic as IsNiladic , p.Name as ParameterName , p.ParameterType.TypeName as ParameterType , p.Mode as Mode , p.Ordinal as Ordinal FROM OfType(SchemaInformation.Functions, Store.ScalarFunction) as r OUTER APPLY r.Parameters as p) UNION ALL (SELECT r.CatalogName as CatalogName , r.SchemaName as SchemaName , r.Name as Name , CAST(NULL as string) as ReturnTypeName , false as IsAggregate , false as IsComposable , false as IsBuiltIn , false as IsNiladic , p.Name as ParameterName , p.ParameterType.TypeName as ParameterType , p.Mode as Mode , p.Ordinal as Ordinal FROM SchemaInformation.Procedures as r OUTER APPLY r.Parameters as p)) as sp "; private static readonly string FunctionOrderByClause = @" ORDER BY sp.SchemaName , sp.Name , sp.Ordinal "; internal class Memento { private object[] _values; internal Memento(object[] values) { _values = values; } internal object[] Values { get { return _values; } } internal FunctionDetailsReader CreateReader() { return new FunctionDetailsReader(this); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
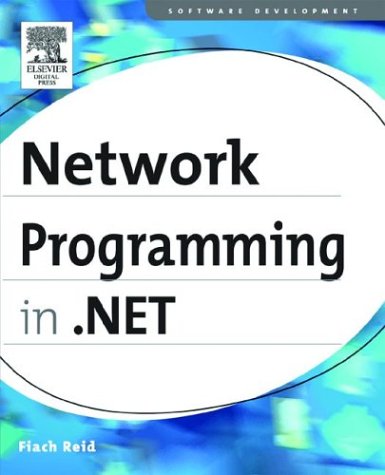
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ServiceProviders.cs
- SwitchElementsCollection.cs
- ButtonFlatAdapter.cs
- ContainerVisual.cs
- PreservationFileWriter.cs
- RegexCompilationInfo.cs
- DataGridViewTopLeftHeaderCell.cs
- SecurityPolicySection.cs
- Query.cs
- XComponentModel.cs
- TemplatePropertyEntry.cs
- ContainerVisual.cs
- Point3DValueSerializer.cs
- ReturnType.cs
- PreservationFileWriter.cs
- FileCodeGroup.cs
- ObjectParameterCollection.cs
- BitmapInitialize.cs
- DirectoryInfo.cs
- XmlSchemaElement.cs
- WebPartManagerDesigner.cs
- DetailsViewPageEventArgs.cs
- BooleanConverter.cs
- SecurityTokenContainer.cs
- ControlOperationBehavior.cs
- CookieProtection.cs
- KeyboardEventArgs.cs
- FlowLayout.cs
- JoinGraph.cs
- UInt32Storage.cs
- TagPrefixAttribute.cs
- HandleRef.cs
- XsdBuilder.cs
- StyleCollection.cs
- Operand.cs
- GestureRecognitionResult.cs
- StructuredTypeEmitter.cs
- DynamicPropertyHolder.cs
- Wow64ConfigurationLoader.cs
- BinaryParser.cs
- TriggerBase.cs
- HGlobalSafeHandle.cs
- ActivityScheduledQuery.cs
- IItemProperties.cs
- ConfigurationLocationCollection.cs
- ResourceDescriptionAttribute.cs
- List.cs
- ResourceWriter.cs
- KnownBoxes.cs
- MonitorWrapper.cs
- DataBindingExpressionBuilder.cs
- NavigationService.cs
- TextRangeBase.cs
- PageParserFilter.cs
- SqlBooleanMismatchVisitor.cs
- HttpCachePolicy.cs
- CustomPopupPlacement.cs
- CellQuery.cs
- PermissionAttributes.cs
- TrustSection.cs
- DatePickerAutomationPeer.cs
- WebPartTransformer.cs
- DependencyProperty.cs
- SqlCommandBuilder.cs
- InvalidAsynchronousStateException.cs
- ToolConsole.cs
- DotNetATv1WindowsLogEntryDeserializer.cs
- XmlSerializerObjectSerializer.cs
- Page.cs
- listitem.cs
- ImageBrush.cs
- Metadata.cs
- QueryCacheManager.cs
- RadioButtonList.cs
- UInt16Converter.cs
- ReferenceCountedObject.cs
- ColorConverter.cs
- EdmType.cs
- ListSortDescriptionCollection.cs
- DesignerSelectionListAdapter.cs
- WebBrowserProgressChangedEventHandler.cs
- StringUtil.cs
- CacheVirtualItemsEvent.cs
- XamlTypeMapper.cs
- Executor.cs
- Binding.cs
- FontWeight.cs
- PipelineModuleStepContainer.cs
- MouseBinding.cs
- CodeThrowExceptionStatement.cs
- ValidationHelpers.cs
- AccessText.cs
- CompositeDataBoundControl.cs
- RefreshEventArgs.cs
- TextViewSelectionProcessor.cs
- MultipleViewPattern.cs
- NestedContainer.cs
- PrintDocument.cs
- COM2ICategorizePropertiesHandler.cs
- WsatTransactionInfo.cs