Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / System / Windows / Input / Command / MouseBinding.cs / 1305600 / MouseBinding.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: The MouseBinding class is used by the developer to create Mouse Input Bindings // // See spec at : http://avalon/coreui/Specs/Commanding(new).mht // //* MouseBinding class serves the purpose of Input Bindings for Mouse Device. // // History: // 06/01/2003 : chandras - Created // 05/01/2004 : chandras- changed to new design. //--------------------------------------------------------------------------- using System; using System.Windows.Input; using System.Windows; using System.Windows.Markup; using System.ComponentModel; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Input { ////// MouseBinding - Implements InputBinding (generic InputGesture-Command map) /// MouseBinding acts like a map for MouseGesture and Commands. /// Most of the logic is in InputBinding and MouseGesture, this only /// facilitates user to specify MouseAction directly without going in /// MouseGesture path. Also it provides the MouseGestureTypeConverter /// on the Gesture property to have MouseGesture, like "RightClick" /// defined in Markup as Gesture="RightClick" working. /// public class MouseBinding : InputBinding { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors ////// constructor /// public MouseBinding() : base() { } ////// Constructor /// /// Command Associated /// Mouse Action internal MouseBinding(ICommand command, MouseAction mouseAction) : this(command, new MouseGesture(mouseAction)) { } ////// Constructor /// /// Command Associated /// Mmouse Gesture associated public MouseBinding(ICommand command, MouseGesture gesture) : base(command, gesture) { SynchronizePropertiesFromGesture(gesture); // Hooking the handler explicitly becuase base constructor uses _gesture // It cannot use Gesture property itself because it is a virtual gesture.PropertyChanged += new PropertyChangedEventHandler(OnMouseGesturePropertyChanged); } #endregion Constructors //------------------------------------------------------ // // Properties // //----------------------------------------------------- #region Properties ////// MouseGesture /// [TypeConverter(typeof(MouseGestureConverter))] [ValueSerializer(typeof(MouseGestureValueSerializer))] public override InputGesture Gesture { get { return base.Gesture as MouseGesture; } set { MouseGesture oldMouseGesture = Gesture as MouseGesture; MouseGesture mouseGesture = value as MouseGesture; if (mouseGesture != null) { base.Gesture = mouseGesture; SynchronizePropertiesFromGesture(mouseGesture); if (oldMouseGesture != mouseGesture) { if (oldMouseGesture != null) { oldMouseGesture.PropertyChanged -= new PropertyChangedEventHandler(OnMouseGesturePropertyChanged); } mouseGesture.PropertyChanged += new PropertyChangedEventHandler(OnMouseGesturePropertyChanged); } } else { throw new ArgumentException(SR.Get(SRID.InputBinding_ExpectedInputGesture, typeof(MouseGesture))); } } } ////// Dependency property for MouseAction /// public static readonly DependencyProperty MouseActionProperty = DependencyProperty.Register("MouseAction", typeof(MouseAction), typeof(MouseBinding), new UIPropertyMetadata(MouseAction.None, new PropertyChangedCallback(OnMouseActionPropertyChanged))); ////// MouseAction /// public MouseAction MouseAction { get { return (MouseAction)GetValue(MouseActionProperty); } set { SetValue(MouseActionProperty, value); } } private static void OnMouseActionPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { MouseBinding mouseBinding = (MouseBinding)d; mouseBinding.SynchronizeGestureFromProperties((MouseAction)(e.NewValue)); } #endregion #region Freezable protected override Freezable CreateInstanceCore() { return new MouseBinding(); } protected override void CloneCore(Freezable sourceFreezable) { base.CloneCore(sourceFreezable); CloneGesture(); } protected override void CloneCurrentValueCore(Freezable sourceFreezable) { base.CloneCurrentValueCore(sourceFreezable); CloneGesture(); } protected override void GetAsFrozenCore(Freezable sourceFreezable) { base.GetAsFrozenCore(sourceFreezable); CloneGesture(); } protected override void GetCurrentValueAsFrozenCore(Freezable sourceFreezable) { base.GetCurrentValueAsFrozenCore(sourceFreezable); CloneGesture(); } #endregion #region Private Methods ////// Synchronized Properties from Gesture /// private void SynchronizePropertiesFromGesture(MouseGesture mouseGesture) { if (!_settingGesture) { _settingGesture = true; try { MouseAction = mouseGesture.MouseAction; } finally { _settingGesture = false; } } } ////// Synchronized Gesture from properties /// private void SynchronizeGestureFromProperties(MouseAction mouseAction) { if (!_settingGesture) { _settingGesture = true; try { if (Gesture == null) { Gesture = new MouseGesture(mouseAction); } else { ((MouseGesture)Gesture).MouseAction = mouseAction; } } finally { _settingGesture = false; } } } private void OnMouseGesturePropertyChanged(object sender, PropertyChangedEventArgs e) { if (string.Compare(e.PropertyName, "MouseAction", StringComparison.Ordinal) == 0) { MouseGesture mouseGesture = Gesture as MouseGesture; if (mouseGesture != null) { SynchronizePropertiesFromGesture(mouseGesture); } } } private void CloneGesture() { MouseGesture mouseGesture = Gesture as MouseGesture; if (mouseGesture != null) { mouseGesture.PropertyChanged += new PropertyChangedEventHandler(OnMouseGesturePropertyChanged); } } #endregion //------------------------------------------------------ // // Private Fields // //------------------------------------------------------ #region Data private bool _settingGesture = false; #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
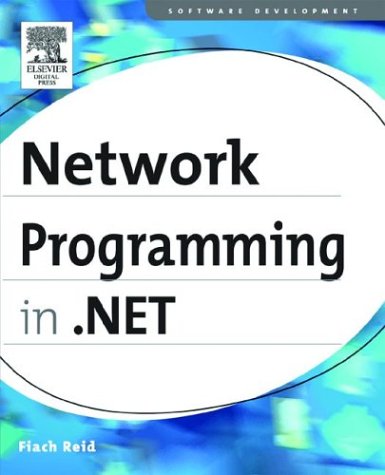
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ReplacementText.cs
- StructuralComparisons.cs
- FontCollection.cs
- SQLBinaryStorage.cs
- RuntimeHandles.cs
- SrgsGrammarCompiler.cs
- IndicShape.cs
- ScrollItemPattern.cs
- CustomCategoryAttribute.cs
- InvalidEnumArgumentException.cs
- ProfileSettings.cs
- AxDesigner.cs
- FontStyleConverter.cs
- KeyProperty.cs
- CacheOutputQuery.cs
- SQLCharsStorage.cs
- XPathNavigator.cs
- XmlProcessingInstruction.cs
- XmlQualifiedName.cs
- GradientStop.cs
- Parsers.cs
- NativeRecognizer.cs
- RelationshipConverter.cs
- ExceptionHandlers.cs
- DynamicDiscoSearcher.cs
- GeneratedContractType.cs
- Point3DAnimationUsingKeyFrames.cs
- UnsafeNativeMethods.cs
- DoubleKeyFrameCollection.cs
- WebPartDeleteVerb.cs
- EntityDataSourceEntityTypeFilterConverter.cs
- XmlSerializerVersionAttribute.cs
- compensatingcollection.cs
- IntegerValidator.cs
- SetterBaseCollection.cs
- SubMenuStyleCollectionEditor.cs
- EventBuilder.cs
- DecimalAverageAggregationOperator.cs
- AlphabeticalEnumConverter.cs
- AddInProcess.cs
- XmlSchemaComplexType.cs
- Form.cs
- StorageScalarPropertyMapping.cs
- EntityConnectionStringBuilder.cs
- SqlTransaction.cs
- ColumnResizeUndoUnit.cs
- SoapAttributeOverrides.cs
- ProfileSection.cs
- BulletedListDesigner.cs
- Guid.cs
- XmlILIndex.cs
- ReversePositionQuery.cs
- OledbConnectionStringbuilder.cs
- MessageDirection.cs
- StringAnimationBase.cs
- Errors.cs
- ResXResourceReader.cs
- XmlnsCompatibleWithAttribute.cs
- MatrixKeyFrameCollection.cs
- PtsPage.cs
- WebPartsPersonalizationAuthorization.cs
- PropertyRecord.cs
- TrackingSection.cs
- PathSegmentCollection.cs
- PropertyManager.cs
- SystemDropShadowChrome.cs
- OutputCacheProfileCollection.cs
- KnownTypesProvider.cs
- SqlBulkCopy.cs
- HwndSource.cs
- UserInitiatedRoutedEventPermission.cs
- BindingListCollectionView.cs
- Iis7Helper.cs
- AnimatedTypeHelpers.cs
- ResXResourceSet.cs
- ContextStaticAttribute.cs
- TabControl.cs
- SqlWorkflowInstanceStore.cs
- DesignerActionPanel.cs
- xamlnodes.cs
- RoleService.cs
- ActiveXSerializer.cs
- Comparer.cs
- FusionWrap.cs
- BitmapEffectInputData.cs
- LineGeometry.cs
- CollectionTraceRecord.cs
- ToolboxItem.cs
- DataViewListener.cs
- HtmlMeta.cs
- HtmlTextArea.cs
- PhysicalAddress.cs
- Types.cs
- CodeGeneratorAttribute.cs
- ClientTargetSection.cs
- ColorInterpolationModeValidation.cs
- StrongNameKeyPair.cs
- TextElementAutomationPeer.cs
- KnownAssemblyEntry.cs
- SqlExpressionNullability.cs