Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / MS / Internal / Controls / ActiveXContainer.cs / 1305600 / ActiveXContainer.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // // // // Description: Implements ActiveXContainer interfaces to host // ActiveX controls // // Source copied from AxContainer.cs // // History // 04/17/05 KusumaV Created // //----------------------------------------------------------------------------- using System; using System.Collections; using System.Diagnostics; using Microsoft.Win32; using System.Windows.Interop; using MS.Win32; using System.Security ; using System.Windows.Controls; namespace MS.Internal.Controls { #region class ActiveXContainer //This implements the basic container interfaces. Other siting related interfaces are //implemented on the ActiveXSite object e.g. IOleClientSite, IOleInPlaceSite, IOleControlSite etc. internal class ActiveXContainer : UnsafeNativeMethods.IOleContainer, UnsafeNativeMethods.IOleInPlaceFrame { #region Constructor ////// Critical - accesses critical _host member. /// [ SecurityCritical ] internal ActiveXContainer(ActiveXHost host) { this._host = host; Invariant.Assert(_host != null); } #endregion Constructor //----------------------------------------------------- // // Internal Methods // //----------------------------------------------------- #region Internal Methods #region IOleContainer // // IOleContainer methods: // int UnsafeNativeMethods.IOleContainer.ParseDisplayName(Object pbc, string pszDisplayName, int[] pchEaten, Object[] ppmkOut) { if (ppmkOut != null) ppmkOut[0] = null; return NativeMethods.E_NOTIMPL; } ////// Critical - calls _host.ActiveXInstance /// [SecurityCritical] int UnsafeNativeMethods.IOleContainer.EnumObjects(int grfFlags, out UnsafeNativeMethods.IEnumUnknown ppenum) { ppenum = null; Debug.Assert(_host != null, "gotta have the avalon activex host"); object ax = _host.ActiveXInstance; //We support only one control, return that here //How does one add multiple controls to a container? if (ax != null && ( ((grfFlags & NativeMethods.OLECONTF_EMBEDDINGS) != 0) || ((grfFlags & NativeMethods.OLECONTF_ONLYIFRUNNING) != 0 && _host.ActiveXState == ActiveXHelper.ActiveXState.Running )) ) { Object[] temp = new Object[1]; temp[0]= ax; ppenum = new EnumUnknown(temp); return NativeMethods.S_OK; } ppenum = new EnumUnknown(null); return NativeMethods.S_OK; } int UnsafeNativeMethods.IOleContainer.LockContainer(bool fLock) { return NativeMethods.E_NOTIMPL; } #endregion IOleContainer #region IOleInPlaceFrame // // IOleInPlaceFrame methods: // ////// Critical - accesses critical _host member. /// [SecurityCritical] IntPtr UnsafeNativeMethods.IOleInPlaceFrame.GetWindow() { return _host.ParentHandle.Handle; } int UnsafeNativeMethods.IOleInPlaceFrame.ContextSensitiveHelp(int fEnterMode) { return NativeMethods.S_OK; } int UnsafeNativeMethods.IOleInPlaceFrame.GetBorder(NativeMethods.COMRECT lprectBorder) { return NativeMethods.E_NOTIMPL; } int UnsafeNativeMethods.IOleInPlaceFrame.RequestBorderSpace(NativeMethods.COMRECT pborderwidths) { return NativeMethods.E_NOTIMPL; } int UnsafeNativeMethods.IOleInPlaceFrame.SetBorderSpace(NativeMethods.COMRECT pborderwidths) { return NativeMethods.E_NOTIMPL; } int UnsafeNativeMethods.IOleInPlaceFrame.SetActiveObject(UnsafeNativeMethods.IOleInPlaceActiveObject pActiveObject, string pszObjName) { //[....] has code to remove selection handler around the active object //and add it around the new one // //Since we don't have anything like that in Avalon, we do nothing // //For future reference, added skeletal code on how to get to the internal hosting //objects incase they are needed here. /* ActiveXHost host = null; if (pActiveObject is UnsafeNativeMethods.IOleObject) { UnsafeNativeMethods.IOleObject oleObject = (UnsafeNativeMethods.IOleObject)pActiveObject; UnsafeNativeMethods.IOleClientSite clientSite = null; try { clientSite = oleObject.GetClientSite(); if ((clientSite as ActiveXSite) != null) { ctl = ((ActiveXSite)(clientSite)).GetActiveXHost(); } } catch (COMException t) { Debug.Fail(t.ToString()); } } */ return NativeMethods.S_OK; } int UnsafeNativeMethods.IOleInPlaceFrame.InsertMenus(IntPtr hmenuShared, NativeMethods.tagOleMenuGroupWidths lpMenuWidths) { return NativeMethods.S_OK; } int UnsafeNativeMethods.IOleInPlaceFrame.SetMenu(IntPtr hmenuShared, IntPtr holemenu, IntPtr hwndActiveObject) { return NativeMethods.E_NOTIMPL; } int UnsafeNativeMethods.IOleInPlaceFrame.RemoveMenus(IntPtr hmenuShared) { return NativeMethods.E_NOTIMPL; } int UnsafeNativeMethods.IOleInPlaceFrame.SetStatusText(string pszStatusText) { return NativeMethods.E_NOTIMPL; } int UnsafeNativeMethods.IOleInPlaceFrame.EnableModeless(bool fEnable) { return NativeMethods.E_NOTIMPL; } int UnsafeNativeMethods.IOleInPlaceFrame.TranslateAccelerator(ref MSG lpmsg, short wID) { return NativeMethods.S_FALSE; } #endregion IOleInPlaceFrame ////// Critical - calls ActiveXHost. /// TreatAsSafe - transitioning to UIActive is ok. /// [ SecurityCritical, SecurityTreatAsSafe ] internal void OnUIActivate(ActiveXHost site) { // The ShDocVw control repeatedly calls OnUIActivate() with the same // site. This causes the assert below to fire. // if (_siteUIActive == site) return; if (_siteUIActive != null ) { //[....] WebOC also uses ActiveXHost instead of ActiveXSite. //Ideally it should have been the site but since its a 1-1 relationship //for hosting the webOC, it will work ActiveXHost tempSite = _siteUIActive; tempSite.ActiveXInPlaceObject.UIDeactivate(); } Debug.Assert(_siteUIActive == null, "Object did not call OnUIDeactivate"); _siteUIActive = site; // } ////// Critical - calls ActiveXHost. /// TreatAsSafe - transitioning away from UI-Active is ok. /// [SecurityCritical, SecurityTreatAsSafe ] internal void OnUIDeactivate(ActiveXHost site) { #if DEBUG if (_siteUIActive != null) { // Debug.Assert(this.ActiveXHost == site, "deactivating when not active..."); } #endif // DEBUG // _siteUIActive = null; } ////// Critical - accesses critical data. /// TreatAsSafe - Transitioning away from in-place is ok. /// Clearing the active site is ok. /// [ SecurityCritical, SecurityTreatAsSafe ] internal void OnInPlaceDeactivate(ActiveXHost site) { // if (this.ActiveXHost == site) { } } #endregion Internal Methods //------------------------------------------------------ // // Internal Properties // //----------------------------------------------------- #region Internal Properties ////// Critical - accesses critical _host member. /// internal ActiveXHost ActiveXHost { [ SecurityCritical ] get { return _host; } } #endregion Internal Properties //------------------------------------------------------ // // Private Fields // //------------------------------------------------------ #region Private Fields ////// Critical - data - ptr. to control. /// [ SecurityCritical ] private ActiveXHost _host; ////// Critical - data - ptr. to control. /// [ SecurityCritical ] private ActiveXHost _siteUIActive; #endregion Private Fields } #endregion class ActiveXContainer } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // // // // Description: Implements ActiveXContainer interfaces to host // ActiveX controls // // Source copied from AxContainer.cs // // History // 04/17/05 KusumaV Created // //----------------------------------------------------------------------------- using System; using System.Collections; using System.Diagnostics; using Microsoft.Win32; using System.Windows.Interop; using MS.Win32; using System.Security ; using System.Windows.Controls; namespace MS.Internal.Controls { #region class ActiveXContainer //This implements the basic container interfaces. Other siting related interfaces are //implemented on the ActiveXSite object e.g. IOleClientSite, IOleInPlaceSite, IOleControlSite etc. internal class ActiveXContainer : UnsafeNativeMethods.IOleContainer, UnsafeNativeMethods.IOleInPlaceFrame { #region Constructor ////// Critical - accesses critical _host member. /// [ SecurityCritical ] internal ActiveXContainer(ActiveXHost host) { this._host = host; Invariant.Assert(_host != null); } #endregion Constructor //----------------------------------------------------- // // Internal Methods // //----------------------------------------------------- #region Internal Methods #region IOleContainer // // IOleContainer methods: // int UnsafeNativeMethods.IOleContainer.ParseDisplayName(Object pbc, string pszDisplayName, int[] pchEaten, Object[] ppmkOut) { if (ppmkOut != null) ppmkOut[0] = null; return NativeMethods.E_NOTIMPL; } ////// Critical - calls _host.ActiveXInstance /// [SecurityCritical] int UnsafeNativeMethods.IOleContainer.EnumObjects(int grfFlags, out UnsafeNativeMethods.IEnumUnknown ppenum) { ppenum = null; Debug.Assert(_host != null, "gotta have the avalon activex host"); object ax = _host.ActiveXInstance; //We support only one control, return that here //How does one add multiple controls to a container? if (ax != null && ( ((grfFlags & NativeMethods.OLECONTF_EMBEDDINGS) != 0) || ((grfFlags & NativeMethods.OLECONTF_ONLYIFRUNNING) != 0 && _host.ActiveXState == ActiveXHelper.ActiveXState.Running )) ) { Object[] temp = new Object[1]; temp[0]= ax; ppenum = new EnumUnknown(temp); return NativeMethods.S_OK; } ppenum = new EnumUnknown(null); return NativeMethods.S_OK; } int UnsafeNativeMethods.IOleContainer.LockContainer(bool fLock) { return NativeMethods.E_NOTIMPL; } #endregion IOleContainer #region IOleInPlaceFrame // // IOleInPlaceFrame methods: // ////// Critical - accesses critical _host member. /// [SecurityCritical] IntPtr UnsafeNativeMethods.IOleInPlaceFrame.GetWindow() { return _host.ParentHandle.Handle; } int UnsafeNativeMethods.IOleInPlaceFrame.ContextSensitiveHelp(int fEnterMode) { return NativeMethods.S_OK; } int UnsafeNativeMethods.IOleInPlaceFrame.GetBorder(NativeMethods.COMRECT lprectBorder) { return NativeMethods.E_NOTIMPL; } int UnsafeNativeMethods.IOleInPlaceFrame.RequestBorderSpace(NativeMethods.COMRECT pborderwidths) { return NativeMethods.E_NOTIMPL; } int UnsafeNativeMethods.IOleInPlaceFrame.SetBorderSpace(NativeMethods.COMRECT pborderwidths) { return NativeMethods.E_NOTIMPL; } int UnsafeNativeMethods.IOleInPlaceFrame.SetActiveObject(UnsafeNativeMethods.IOleInPlaceActiveObject pActiveObject, string pszObjName) { //[....] has code to remove selection handler around the active object //and add it around the new one // //Since we don't have anything like that in Avalon, we do nothing // //For future reference, added skeletal code on how to get to the internal hosting //objects incase they are needed here. /* ActiveXHost host = null; if (pActiveObject is UnsafeNativeMethods.IOleObject) { UnsafeNativeMethods.IOleObject oleObject = (UnsafeNativeMethods.IOleObject)pActiveObject; UnsafeNativeMethods.IOleClientSite clientSite = null; try { clientSite = oleObject.GetClientSite(); if ((clientSite as ActiveXSite) != null) { ctl = ((ActiveXSite)(clientSite)).GetActiveXHost(); } } catch (COMException t) { Debug.Fail(t.ToString()); } } */ return NativeMethods.S_OK; } int UnsafeNativeMethods.IOleInPlaceFrame.InsertMenus(IntPtr hmenuShared, NativeMethods.tagOleMenuGroupWidths lpMenuWidths) { return NativeMethods.S_OK; } int UnsafeNativeMethods.IOleInPlaceFrame.SetMenu(IntPtr hmenuShared, IntPtr holemenu, IntPtr hwndActiveObject) { return NativeMethods.E_NOTIMPL; } int UnsafeNativeMethods.IOleInPlaceFrame.RemoveMenus(IntPtr hmenuShared) { return NativeMethods.E_NOTIMPL; } int UnsafeNativeMethods.IOleInPlaceFrame.SetStatusText(string pszStatusText) { return NativeMethods.E_NOTIMPL; } int UnsafeNativeMethods.IOleInPlaceFrame.EnableModeless(bool fEnable) { return NativeMethods.E_NOTIMPL; } int UnsafeNativeMethods.IOleInPlaceFrame.TranslateAccelerator(ref MSG lpmsg, short wID) { return NativeMethods.S_FALSE; } #endregion IOleInPlaceFrame ////// Critical - calls ActiveXHost. /// TreatAsSafe - transitioning to UIActive is ok. /// [ SecurityCritical, SecurityTreatAsSafe ] internal void OnUIActivate(ActiveXHost site) { // The ShDocVw control repeatedly calls OnUIActivate() with the same // site. This causes the assert below to fire. // if (_siteUIActive == site) return; if (_siteUIActive != null ) { //[....] WebOC also uses ActiveXHost instead of ActiveXSite. //Ideally it should have been the site but since its a 1-1 relationship //for hosting the webOC, it will work ActiveXHost tempSite = _siteUIActive; tempSite.ActiveXInPlaceObject.UIDeactivate(); } Debug.Assert(_siteUIActive == null, "Object did not call OnUIDeactivate"); _siteUIActive = site; // } ////// Critical - calls ActiveXHost. /// TreatAsSafe - transitioning away from UI-Active is ok. /// [SecurityCritical, SecurityTreatAsSafe ] internal void OnUIDeactivate(ActiveXHost site) { #if DEBUG if (_siteUIActive != null) { // Debug.Assert(this.ActiveXHost == site, "deactivating when not active..."); } #endif // DEBUG // _siteUIActive = null; } ////// Critical - accesses critical data. /// TreatAsSafe - Transitioning away from in-place is ok. /// Clearing the active site is ok. /// [ SecurityCritical, SecurityTreatAsSafe ] internal void OnInPlaceDeactivate(ActiveXHost site) { // if (this.ActiveXHost == site) { } } #endregion Internal Methods //------------------------------------------------------ // // Internal Properties // //----------------------------------------------------- #region Internal Properties ////// Critical - accesses critical _host member. /// internal ActiveXHost ActiveXHost { [ SecurityCritical ] get { return _host; } } #endregion Internal Properties //------------------------------------------------------ // // Private Fields // //------------------------------------------------------ #region Private Fields ////// Critical - data - ptr. to control. /// [ SecurityCritical ] private ActiveXHost _host; ////// Critical - data - ptr. to control. /// [ SecurityCritical ] private ActiveXHost _siteUIActive; #endregion Private Fields } #endregion class ActiveXContainer } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
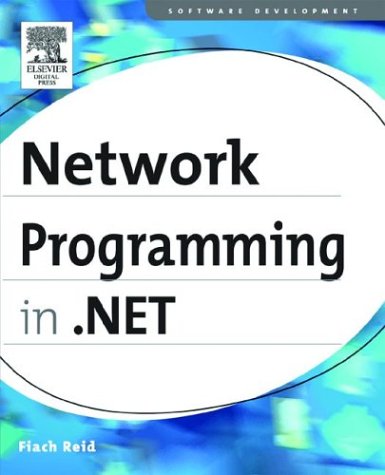
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- _LazyAsyncResult.cs
- XmlValidatingReader.cs
- DataContext.cs
- TypedElement.cs
- FixedPosition.cs
- FtpWebResponse.cs
- ImmutableObjectAttribute.cs
- StartUpEventArgs.cs
- Triplet.cs
- PasswordTextNavigator.cs
- WindowsGraphics.cs
- RelativeSource.cs
- versioninfo.cs
- OleDbConnectionPoolGroupProviderInfo.cs
- _FixedSizeReader.cs
- DiscoveryExceptionDictionary.cs
- RequestCachingSection.cs
- PointCollectionValueSerializer.cs
- ServiceContractListItem.cs
- BindingSource.cs
- CompilerErrorCollection.cs
- CompilerInfo.cs
- SendMessageRecord.cs
- RuleInfoComparer.cs
- BulletedList.cs
- LoginCancelEventArgs.cs
- SpeechSynthesizer.cs
- XPathNavigatorKeyComparer.cs
- SynchronizedDispatch.cs
- AssemblyNameUtility.cs
- CompileLiteralTextParser.cs
- EntityTypeBase.cs
- NaturalLanguageHyphenator.cs
- Monitor.cs
- SHA384Cng.cs
- XhtmlBasicTextViewAdapter.cs
- AsyncOperationContext.cs
- PointConverter.cs
- ResourceDescriptionAttribute.cs
- DataGridViewCheckBoxColumn.cs
- CommandEventArgs.cs
- ReadOnlyTernaryTree.cs
- DynamicContractTypeBuilder.cs
- SqlCommandSet.cs
- WindowsSysHeader.cs
- PreviewKeyDownEventArgs.cs
- CodeExpressionCollection.cs
- DataSourceSelectArguments.cs
- DataObjectMethodAttribute.cs
- ByteAnimationBase.cs
- RowSpanVector.cs
- MailAddress.cs
- PackageRelationshipCollection.cs
- SqlDuplicator.cs
- FocusChangedEventArgs.cs
- TypeUtil.cs
- XmlNodeWriter.cs
- TransformerInfoCollection.cs
- InlinedLocationReference.cs
- SchemaNotation.cs
- ParsedAttributeCollection.cs
- OleDbRowUpdatedEvent.cs
- DivideByZeroException.cs
- NavigatorInvalidBodyAccessException.cs
- HttpCapabilitiesSectionHandler.cs
- WindowsStatusBar.cs
- DBCSCodePageEncoding.cs
- MemberHolder.cs
- FloaterParaClient.cs
- ArrayTypeMismatchException.cs
- OLEDB_Util.cs
- IsolatedStorageFileStream.cs
- SafeNativeMethods.cs
- dbdatarecord.cs
- TabPage.cs
- Executor.cs
- InkPresenter.cs
- AttributeProviderAttribute.cs
- Stacktrace.cs
- httpapplicationstate.cs
- RSAOAEPKeyExchangeFormatter.cs
- UnknownBitmapDecoder.cs
- webeventbuffer.cs
- SparseMemoryStream.cs
- SqlAliaser.cs
- MonthChangedEventArgs.cs
- BidOverLoads.cs
- VirtualPathUtility.cs
- DataGridViewSortCompareEventArgs.cs
- AnnotationResourceCollection.cs
- ELinqQueryState.cs
- Vector3DAnimationUsingKeyFrames.cs
- CompositionAdorner.cs
- SecurityResources.cs
- GridItemProviderWrapper.cs
- RotateTransform.cs
- ServerProtocol.cs
- TextViewSelectionProcessor.cs
- CustomError.cs
- DBConcurrencyException.cs