Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / CompMod / System / ComponentModel / LicenseProviderAttribute.cs / 1 / LicenseProviderAttribute.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.ComponentModel { using System; using System.Security.Permissions; ////// [AttributeUsage(AttributeTargets.Class, AllowMultiple = false, Inherited = false)] public sealed class LicenseProviderAttribute : Attribute { ///Specifies the ////// to use with a class. /// public static readonly LicenseProviderAttribute Default = new LicenseProviderAttribute(); private Type licenseProviderType = null; private string licenseProviderName = null; ////// Specifies the default value, which is no provider. This ///field is read-only. /// /// public LicenseProviderAttribute() : this((string)null) { } ///Initializes a new instance of the ///class without a license /// provider. /// public LicenseProviderAttribute(string typeName) { licenseProviderName = typeName; } ////// Initializes a new instance of the ///class with /// the specified type. /// /// public LicenseProviderAttribute(Type type) { licenseProviderType = type; } ////// Initializes a new instance of the ///class with /// the specified type of license provider. /// /// public Type LicenseProvider { // SECREVIEW: Remove this attribute once bug#411910 is fixed. [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Security", "CA2113:SecureLateBindingMethods")] get { if (licenseProviderType == null && licenseProviderName != null) { licenseProviderType = Type.GetType(licenseProviderName); } return licenseProviderType; } } ///Gets the license provider to use with the associated class. ////// /// public override object TypeId { get { string typeName = licenseProviderName; if (typeName == null && licenseProviderType != null) { typeName = licenseProviderType.FullName; } return GetType().FullName + typeName; } } ////// This defines a unique ID for this attribute type. It is used /// by filtering algorithms to identify two attributes that are /// the same type. For most attributes, this just returns the /// Type instance for the attribute. LicenseProviderAttribute overrides this to include the type name and the /// provider type name. /// ////// /// public override bool Equals(object value) { if (value is LicenseProviderAttribute && value != null) { Type type = ((LicenseProviderAttribute)value).LicenseProvider; if (type == LicenseProvider) { return true; } else { if (type != null && type.Equals(LicenseProvider)) { return true; } } } return false; } ////// public override int GetHashCode() { return base.GetHashCode(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ ///// Returns the hashcode for this object. /// ///// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.ComponentModel { using System; using System.Security.Permissions; ////// [AttributeUsage(AttributeTargets.Class, AllowMultiple = false, Inherited = false)] public sealed class LicenseProviderAttribute : Attribute { ///Specifies the ////// to use with a class. /// public static readonly LicenseProviderAttribute Default = new LicenseProviderAttribute(); private Type licenseProviderType = null; private string licenseProviderName = null; ////// Specifies the default value, which is no provider. This ///field is read-only. /// /// public LicenseProviderAttribute() : this((string)null) { } ///Initializes a new instance of the ///class without a license /// provider. /// public LicenseProviderAttribute(string typeName) { licenseProviderName = typeName; } ////// Initializes a new instance of the ///class with /// the specified type. /// /// public LicenseProviderAttribute(Type type) { licenseProviderType = type; } ////// Initializes a new instance of the ///class with /// the specified type of license provider. /// /// public Type LicenseProvider { // SECREVIEW: Remove this attribute once bug#411910 is fixed. [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Security", "CA2113:SecureLateBindingMethods")] get { if (licenseProviderType == null && licenseProviderName != null) { licenseProviderType = Type.GetType(licenseProviderName); } return licenseProviderType; } } ///Gets the license provider to use with the associated class. ////// /// public override object TypeId { get { string typeName = licenseProviderName; if (typeName == null && licenseProviderType != null) { typeName = licenseProviderType.FullName; } return GetType().FullName + typeName; } } ////// This defines a unique ID for this attribute type. It is used /// by filtering algorithms to identify two attributes that are /// the same type. For most attributes, this just returns the /// Type instance for the attribute. LicenseProviderAttribute overrides this to include the type name and the /// provider type name. /// ////// /// public override bool Equals(object value) { if (value is LicenseProviderAttribute && value != null) { Type type = ((LicenseProviderAttribute)value).LicenseProvider; if (type == LicenseProvider) { return true; } else { if (type != null && type.Equals(LicenseProvider)) { return true; } } } return false; } ////// public override int GetHashCode() { return base.GetHashCode(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007./// Returns the hashcode for this object. /// ///
Link Menu
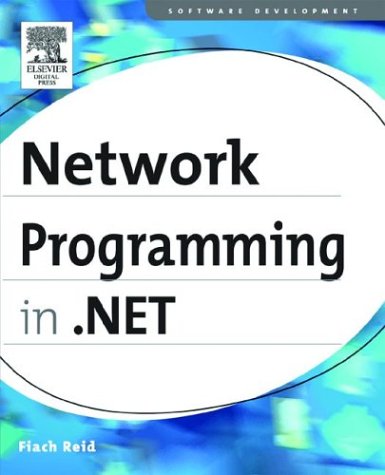
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TextUtf8RawTextWriter.cs
- ResourceDescriptionAttribute.cs
- FileDetails.cs
- AddInBase.cs
- RuntimeConfigLKG.cs
- XmlDigitalSignatureProcessor.cs
- TransformerTypeCollection.cs
- GridItem.cs
- FixedPageProcessor.cs
- unsafenativemethodstextservices.cs
- Table.cs
- BypassElement.cs
- TogglePattern.cs
- UnmanagedMemoryStream.cs
- ThemeDictionaryExtension.cs
- MetaDataInfo.cs
- ValidationSummary.cs
- PreservationFileReader.cs
- ConfigXmlComment.cs
- TypeSystem.cs
- SchemaCreator.cs
- SkipQueryOptionExpression.cs
- Context.cs
- ObjectStateManagerMetadata.cs
- SmtpCommands.cs
- ParallelDesigner.cs
- XPathMultyIterator.cs
- DragEvent.cs
- DefaultPrintController.cs
- InvalidAsynchronousStateException.cs
- NotCondition.cs
- DecimalAnimationBase.cs
- ListBox.cs
- OutgoingWebResponseContext.cs
- StateInitializationDesigner.cs
- UInt64Storage.cs
- SHA1Cng.cs
- ZoneMembershipCondition.cs
- CompleteWizardStep.cs
- TableSectionStyle.cs
- TypeNameConverter.cs
- SiteMapNodeItem.cs
- Util.cs
- PropertyItemInternal.cs
- OutputBuffer.cs
- IPAddress.cs
- ArrayConverter.cs
- CacheOutputQuery.cs
- EntityModelBuildProvider.cs
- XMLSchema.cs
- FixedLineResult.cs
- TextSpan.cs
- WebPartEditVerb.cs
- AssociationProvider.cs
- BitmapImage.cs
- DocumentSignatureManager.cs
- ThemeDirectoryCompiler.cs
- DirectoryRootQuery.cs
- TextEndOfSegment.cs
- Graphics.cs
- TraceUtility.cs
- Transaction.cs
- RelatedPropertyManager.cs
- TreeViewBindingsEditorForm.cs
- Soap.cs
- UnwrappedTypesXmlSerializerManager.cs
- TextSegment.cs
- WeakReferenceList.cs
- EventEntry.cs
- DbExpressionRules.cs
- DirectoryRootQuery.cs
- Byte.cs
- HtmlInputRadioButton.cs
- Base64Decoder.cs
- DomNameTable.cs
- PropertyToken.cs
- TextSelectionHelper.cs
- MimeParameterWriter.cs
- Random.cs
- DataGridCell.cs
- RelationshipEndMember.cs
- ParseElement.cs
- ContainerParaClient.cs
- HttpApplicationFactory.cs
- EmptyStringExpandableObjectConverter.cs
- ImageResources.Designer.cs
- PopupControlService.cs
- SystemIPInterfaceProperties.cs
- CustomErrorsSectionWrapper.cs
- ProfessionalColorTable.cs
- ExceptionUtil.cs
- XsdCachingReader.cs
- SqlDelegatedTransaction.cs
- TrackingParameters.cs
- OdbcEnvironment.cs
- ListViewItem.cs
- EndpointAddressMessageFilter.cs
- HtmlEmptyTagControlBuilder.cs
- RelationshipEndCollection.cs
- RowSpanVector.cs