Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / WinForms / Managed / System / WinForms / DataGridCell.cs / 1 / DataGridCell.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System.Diagnostics; using System.Diagnostics.CodeAnalysis; using System; using System.Globalization; ////// /// [SuppressMessage("Microsoft.Performance", "CA1815:OverrideEqualsAndOperatorEqualsOnValueTypes")] public struct DataGridCell { private int rowNumber; private int columnNumber; ///Identifies a cell in the grid. ////// /// public int ColumnNumber { get { return columnNumber; } set { columnNumber = value; } } ///Gets or sets the number of a column in the ///control. /// /// public int RowNumber { get { return rowNumber; } set { rowNumber = value; } } ///Gets or sets the number of a row in the ///control. /// /// public DataGridCell(int r, int c) { this.rowNumber = r; this.columnNumber = c; } ////// Initializes a new instance of the ///class. /// /// /// [SuppressMessage("Microsoft.Usage", "CA2231:OverrideOperatorEqualsOnOverridingValueTypeEquals")] public override bool Equals(object o) { if (o is DataGridCell) { DataGridCell rhs = (DataGridCell)o; return (rhs.RowNumber == RowNumber && rhs.ColumnNumber == ColumnNumber); } else return false; } ////// Gets a value indicating whether the ///is identical to a second /// . /// /// /// public override int GetHashCode() { return ((~rowNumber * (columnNumber+1)) & 0x00ffff00) >> 8; } ////// Gets /// a hash value that uniquely identifies the cell. /// ////// /// public override string ToString() { return "DataGridCell {RowNumber = " + RowNumber.ToString(CultureInfo.CurrentCulture) + ", ColumnNumber = " + ColumnNumber.ToString(CultureInfo.CurrentCulture) + "}"; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved./// Gets the row number and column number of the cell. /// ///
Link Menu
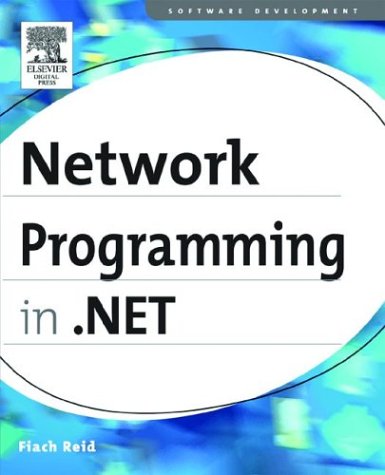
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- IISUnsafeMethods.cs
- OpenTypeLayoutCache.cs
- TypeLibConverter.cs
- GenericXmlSecurityToken.cs
- ClientConvert.cs
- FacetChecker.cs
- TextChange.cs
- WebCategoryAttribute.cs
- DirectoryObjectSecurity.cs
- HandledMouseEvent.cs
- DeploymentSection.cs
- ControlHelper.cs
- FixedSOMPageConstructor.cs
- IsolatedStorage.cs
- PriorityBindingExpression.cs
- Timer.cs
- WindowsListViewGroup.cs
- HostedAspNetEnvironment.cs
- AdornerDecorator.cs
- ColumnPropertiesGroup.cs
- NeutralResourcesLanguageAttribute.cs
- PreviewPrintController.cs
- QuadraticBezierSegment.cs
- ToolStripProfessionalLowResolutionRenderer.cs
- EventLogPermissionHolder.cs
- HierarchicalDataTemplate.cs
- ServiceReference.cs
- panel.cs
- GuidelineSet.cs
- EntitySetBaseCollection.cs
- IconHelper.cs
- ConfigErrorGlyph.cs
- MetafileHeaderWmf.cs
- ContextProperty.cs
- ZipIOFileItemStream.cs
- objectquery_tresulttype.cs
- ClickablePoint.cs
- FlowDocumentReader.cs
- DynamicActivityProperty.cs
- _FtpControlStream.cs
- ErrorFormatterPage.cs
- OdbcEnvironment.cs
- BufferedStream.cs
- FamilyCollection.cs
- RestClientProxyHandler.cs
- PinnedBufferMemoryStream.cs
- MessagePropertyAttribute.cs
- QueryRewriter.cs
- IPAddressCollection.cs
- FileDialog_Vista.cs
- SparseMemoryStream.cs
- TargetParameterCountException.cs
- EditorPartChrome.cs
- HyperLink.cs
- BufferedGraphicsManager.cs
- PreDigestedSignedInfo.cs
- MultipartContentParser.cs
- ScrollChrome.cs
- DesignerDataView.cs
- OracleRowUpdatingEventArgs.cs
- ControlParameter.cs
- Lease.cs
- HGlobalSafeHandle.cs
- FileDialogCustomPlaces.cs
- WindowPatternIdentifiers.cs
- recordstatefactory.cs
- Stroke.cs
- ProcessingInstructionAction.cs
- DebugHandleTracker.cs
- TextDecorationUnitValidation.cs
- DataGridBoundColumn.cs
- LockCookie.cs
- ServicePrincipalNameElement.cs
- DbMetaDataColumnNames.cs
- FrameworkElement.cs
- ListControlStringCollectionEditor.cs
- PageFunction.cs
- ConnectionOrientedTransportChannelListener.cs
- NetworkInformationPermission.cs
- AssemblyResolver.cs
- GenericRootAutomationPeer.cs
- Dump.cs
- CompositeFontParser.cs
- ObfuscationAttribute.cs
- FileSystemEventArgs.cs
- ExpandedProjectionNode.cs
- SuppressIldasmAttribute.cs
- ConfigurationPropertyCollection.cs
- ImageButton.cs
- BaseCAMarshaler.cs
- PieceNameHelper.cs
- DataControlLinkButton.cs
- ApplicationServiceHelper.cs
- UTF8Encoding.cs
- ToolStripPanelRow.cs
- CommonRemoteMemoryBlock.cs
- PageRouteHandler.cs
- FaultBookmark.cs
- EventLogPermissionEntryCollection.cs
- IPEndPointCollection.cs