Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / SMSvcHost / System / ServiceModel / Activation / AppAction.cs / 1 / AppAction.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.ServiceModel.Activation { using System; using System.Collections.Generic; enum AppActionType { // An App is deleted Deleted, // Binding, or AppPool, or RequestsBlocked is changed SettingsChanged } class AppAction { AppActionType actionType; string path; string appPoolId; NullablerequestsBlocked; string[] bindings; AppAction(AppActionType actionType) { this.actionType = actionType; } public static AppAction CreateDeletedAction() { return new AppAction(AppActionType.Deleted); } public static AppAction CreateBindingsChangedAction(string[] bindings) { AppAction action = new AppAction(AppActionType.SettingsChanged); action.bindings = bindings; return action; } public static AppAction CreateAppPoolChangedAction(string appPoolId) { AppAction action = new AppAction(AppActionType.SettingsChanged); action.appPoolId = appPoolId; return action; } public AppActionType ActionType { get { return this.actionType; } } public string Path { get { return this.path; } } public string AppPoolId { get { return this.appPoolId; } } public string[] Bindings { get { return this.bindings; } } public Nullable RequestsBlocked { get { return this.requestsBlocked; } } public void MergeFromCreatedAction(string path, int siteId, string appPoolId, bool requestsBlocked, string[] bindings) { DiagnosticUtility.DebugAssert(this.ActionType == AppActionType.Deleted, "We should get ApplicationCreated notification only when the App is to be deleted."); // Delete + Created = SettingsChanged this.actionType = AppActionType.SettingsChanged; SetSettings(path, appPoolId, requestsBlocked, bindings); // SiteId is ignored because the siteId can't be changed for the same appKey. } public void MergeFromDeletedAction() { DiagnosticUtility.DebugAssert(this.ActionType == AppActionType.SettingsChanged, "We should not get two consecutive ApplicationDeleted notifications."); this.actionType = AppActionType.Deleted; } public void MergeFromBindingChangedAction(string[] bindings) { DiagnosticUtility.DebugAssert(this.ActionType == AppActionType.SettingsChanged, "We should not get two consecutive ApplicationDeleted notifications."); this.bindings = bindings; } public void MergeFromAppPoolChangedAction(string appPoolId) { DiagnosticUtility.DebugAssert(this.ActionType == AppActionType.SettingsChanged, "We should not get two consecutive ApplicationDeleted notifications."); this.appPoolId = appPoolId; } public void MergeFromRequestsBlockedAction(bool requestsBlocked) { DiagnosticUtility.DebugAssert(this.ActionType == AppActionType.SettingsChanged, "We should not get two consecutive ApplicationDeleted notifications."); this.requestsBlocked = requestsBlocked; } void SetSettings(string path, string appPoolId, bool requestsBlocked, string[] bindings) { this.path = path; this.appPoolId = appPoolId; this.requestsBlocked = requestsBlocked; this.bindings = bindings; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
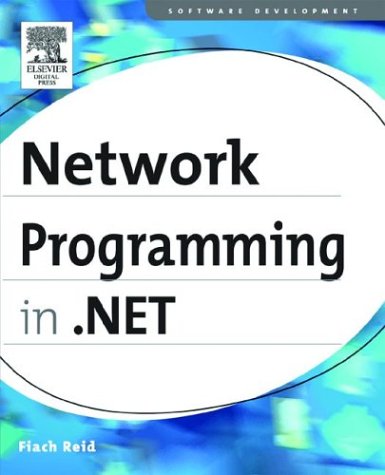
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Propagator.cs
- MetadataPropertyAttribute.cs
- ConfigurationValidatorAttribute.cs
- GlobalProxySelection.cs
- SoapInteropTypes.cs
- ISAPIWorkerRequest.cs
- ConnectionsZone.cs
- ImageListStreamer.cs
- Switch.cs
- CornerRadius.cs
- SourceElementsCollection.cs
- PriorityQueue.cs
- DataGridPreparingCellForEditEventArgs.cs
- EntityClassGenerator.cs
- NegatedCellConstant.cs
- ProfileEventArgs.cs
- RunWorkerCompletedEventArgs.cs
- PrimaryKeyTypeConverter.cs
- DialogResultConverter.cs
- DesignerHelpers.cs
- SessionIDManager.cs
- Section.cs
- SearchExpression.cs
- CollectionViewProxy.cs
- ListViewGroupItemCollection.cs
- sqlnorm.cs
- CodeIdentifier.cs
- VectorAnimation.cs
- InvalidWMPVersionException.cs
- Missing.cs
- HtmlControl.cs
- BlockCollection.cs
- ChangeInterceptorAttribute.cs
- GraphicsPath.cs
- PackagingUtilities.cs
- TextProperties.cs
- Set.cs
- CustomValidator.cs
- PolyLineSegment.cs
- ToolStripScrollButton.cs
- ContentHostHelper.cs
- ProviderConnectionPoint.cs
- DocumentViewerAutomationPeer.cs
- BamlRecordHelper.cs
- PeerDuplexChannel.cs
- Oid.cs
- GridViewAutoFormat.cs
- TraceHwndHost.cs
- ElasticEase.cs
- HttpListenerContext.cs
- _TransmitFileOverlappedAsyncResult.cs
- XmlSchemaRedefine.cs
- DrawingDrawingContext.cs
- MergablePropertyAttribute.cs
- BamlCollectionHolder.cs
- DataAdapter.cs
- SafeNativeMethodsOther.cs
- FlowchartSizeFeature.cs
- SerializationHelper.cs
- Binding.cs
- Evaluator.cs
- TdsEnums.cs
- EditorOptionAttribute.cs
- Wizard.cs
- HitTestParameters3D.cs
- PreProcessor.cs
- BinaryUtilClasses.cs
- _BaseOverlappedAsyncResult.cs
- ProcessModelSection.cs
- LazyTextWriterCreator.cs
- Errors.cs
- OleDbConnectionInternal.cs
- AssociatedControlConverter.cs
- MetaForeignKeyColumn.cs
- CustomAttributeBuilder.cs
- RootBuilder.cs
- Invariant.cs
- BufferedGraphicsContext.cs
- CommandBindingCollection.cs
- DrawingContextWalker.cs
- RadioButtonAutomationPeer.cs
- CustomErrorCollection.cs
- XmlDataCollection.cs
- CodeArrayCreateExpression.cs
- SizeConverter.cs
- OperationFormatUse.cs
- ScriptResourceMapping.cs
- DefaultObjectMappingItemCollection.cs
- ValidationSummary.cs
- TreeView.cs
- XslTransform.cs
- NonSerializedAttribute.cs
- COAUTHIDENTITY.cs
- CDSCollectionETWBCLProvider.cs
- _ContextAwareResult.cs
- ImageCodecInfo.cs
- DesignParameter.cs
- WrappedKeySecurityToken.cs
- ListViewUpdateEventArgs.cs
- FlowDocumentPage.cs