Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Core / CSharp / MS / Internal / Ink / StrokeFIndices.cs / 1 / StrokeFIndices.cs
//------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------- using MS.Utility; using MS.Internal; using System; using System.Windows; using System.Collections.Generic; using System.Globalization; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace MS.Internal.Ink { #region StrokeFIndices ////// A helper struct that represents a fragment of a stroke spine. /// internal struct StrokeFIndices : IEquatable{ #region Private statics private static StrokeFIndices s_empty = new StrokeFIndices(AfterLast, BeforeFirst); private static StrokeFIndices s_full = new StrokeFIndices(BeforeFirst, AfterLast); #endregion #region Internal API /// /// BeforeFirst /// ///internal static double BeforeFirst { get { return double.MinValue; } } /// /// AfterLast /// ///internal static double AfterLast { get { return double.MaxValue; } } /// /// StrokeFIndices /// /// beginFIndex /// endFIndex internal StrokeFIndices(double beginFIndex, double endFIndex) { _beginFIndex = beginFIndex; _endFIndex = endFIndex; } ////// BeginFIndex /// ///internal double BeginFIndex { get { return _beginFIndex; } set { _beginFIndex = value; } } /// /// EndFIndex /// ///internal double EndFIndex { get { return _endFIndex; } set { _endFIndex = value;} } /// /// ToString /// public override string ToString() { return "{" + GetStringRepresentation(_beginFIndex) + "," + GetStringRepresentation(_endFIndex) + "}"; } ////// Equals /// /// ///public bool Equals(StrokeFIndices strokeFIndices) { return (strokeFIndices == this); } /// /// Equals /// /// ///public override bool Equals(Object obj) { // Check for null and compare run-time types if (obj == null || GetType() != obj.GetType()) return false; return ((StrokeFIndices)obj == this); } /// /// GetHashCode /// ///public override int GetHashCode() { return _beginFIndex.GetHashCode() ^ _endFIndex.GetHashCode(); } /// /// operator == /// /// /// ///public static bool operator ==(StrokeFIndices sfiLeft, StrokeFIndices sfiRight) { return (DoubleUtil.AreClose(sfiLeft._beginFIndex, sfiRight._beginFIndex) && DoubleUtil.AreClose(sfiLeft._endFIndex, sfiRight._endFIndex)); } /// /// operator != /// /// /// ///public static bool operator !=(StrokeFIndices sfiLeft, StrokeFIndices sfiRight) { return !(sfiLeft == sfiRight); } internal static string GetStringRepresentation(double fIndex) { if (DoubleUtil.AreClose(fIndex, StrokeFIndices.BeforeFirst)) { return "BeforeFirst"; } if (DoubleUtil.AreClose(fIndex, StrokeFIndices.AfterLast)) { return "AfterLast"; } return fIndex.ToString(CultureInfo.InvariantCulture); } /// /// /// internal static StrokeFIndices Empty { get { return s_empty; } } ////// /// internal static StrokeFIndices Full { get { return s_full; } } ////// /// internal bool IsEmpty { get { return DoubleUtil.GreaterThanOrClose(_beginFIndex, _endFIndex); } } ////// /// internal bool IsFull { get { return ((DoubleUtil.AreClose(_beginFIndex, BeforeFirst)) && (DoubleUtil.AreClose(_endFIndex,AfterLast))); } } #if DEBUG ////// /// private bool IsValid { get { return !double.IsNaN(_beginFIndex) && !double.IsNaN(_endFIndex) && _beginFIndex < _endFIndex; } } #endif ////// Compare StrokeFIndices based on the BeinFIndex /// /// ///internal int CompareTo(StrokeFIndices fIndices) { #if DEBUG System.Diagnostics.Debug.Assert(!double.IsNaN(_beginFIndex) && !double.IsNaN(_endFIndex) && DoubleUtil.LessThan(_beginFIndex, _endFIndex)); #endif if (DoubleUtil.AreClose(BeginFIndex, fIndices.BeginFIndex)) { return 0; } else if (DoubleUtil.GreaterThan(BeginFIndex, fIndices.BeginFIndex)) { return 1; } else { return -1; } } #endregion #region Fields private double _beginFIndex; private double _endFIndex; #endregion } #endregion } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------- using MS.Utility; using MS.Internal; using System; using System.Windows; using System.Collections.Generic; using System.Globalization; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace MS.Internal.Ink { #region StrokeFIndices ////// A helper struct that represents a fragment of a stroke spine. /// internal struct StrokeFIndices : IEquatable{ #region Private statics private static StrokeFIndices s_empty = new StrokeFIndices(AfterLast, BeforeFirst); private static StrokeFIndices s_full = new StrokeFIndices(BeforeFirst, AfterLast); #endregion #region Internal API /// /// BeforeFirst /// ///internal static double BeforeFirst { get { return double.MinValue; } } /// /// AfterLast /// ///internal static double AfterLast { get { return double.MaxValue; } } /// /// StrokeFIndices /// /// beginFIndex /// endFIndex internal StrokeFIndices(double beginFIndex, double endFIndex) { _beginFIndex = beginFIndex; _endFIndex = endFIndex; } ////// BeginFIndex /// ///internal double BeginFIndex { get { return _beginFIndex; } set { _beginFIndex = value; } } /// /// EndFIndex /// ///internal double EndFIndex { get { return _endFIndex; } set { _endFIndex = value;} } /// /// ToString /// public override string ToString() { return "{" + GetStringRepresentation(_beginFIndex) + "," + GetStringRepresentation(_endFIndex) + "}"; } ////// Equals /// /// ///public bool Equals(StrokeFIndices strokeFIndices) { return (strokeFIndices == this); } /// /// Equals /// /// ///public override bool Equals(Object obj) { // Check for null and compare run-time types if (obj == null || GetType() != obj.GetType()) return false; return ((StrokeFIndices)obj == this); } /// /// GetHashCode /// ///public override int GetHashCode() { return _beginFIndex.GetHashCode() ^ _endFIndex.GetHashCode(); } /// /// operator == /// /// /// ///public static bool operator ==(StrokeFIndices sfiLeft, StrokeFIndices sfiRight) { return (DoubleUtil.AreClose(sfiLeft._beginFIndex, sfiRight._beginFIndex) && DoubleUtil.AreClose(sfiLeft._endFIndex, sfiRight._endFIndex)); } /// /// operator != /// /// /// ///public static bool operator !=(StrokeFIndices sfiLeft, StrokeFIndices sfiRight) { return !(sfiLeft == sfiRight); } internal static string GetStringRepresentation(double fIndex) { if (DoubleUtil.AreClose(fIndex, StrokeFIndices.BeforeFirst)) { return "BeforeFirst"; } if (DoubleUtil.AreClose(fIndex, StrokeFIndices.AfterLast)) { return "AfterLast"; } return fIndex.ToString(CultureInfo.InvariantCulture); } /// /// /// internal static StrokeFIndices Empty { get { return s_empty; } } ////// /// internal static StrokeFIndices Full { get { return s_full; } } ////// /// internal bool IsEmpty { get { return DoubleUtil.GreaterThanOrClose(_beginFIndex, _endFIndex); } } ////// /// internal bool IsFull { get { return ((DoubleUtil.AreClose(_beginFIndex, BeforeFirst)) && (DoubleUtil.AreClose(_endFIndex,AfterLast))); } } #if DEBUG ////// /// private bool IsValid { get { return !double.IsNaN(_beginFIndex) && !double.IsNaN(_endFIndex) && _beginFIndex < _endFIndex; } } #endif ////// Compare StrokeFIndices based on the BeinFIndex /// /// ///internal int CompareTo(StrokeFIndices fIndices) { #if DEBUG System.Diagnostics.Debug.Assert(!double.IsNaN(_beginFIndex) && !double.IsNaN(_endFIndex) && DoubleUtil.LessThan(_beginFIndex, _endFIndex)); #endif if (DoubleUtil.AreClose(BeginFIndex, fIndices.BeginFIndex)) { return 0; } else if (DoubleUtil.GreaterThan(BeginFIndex, fIndices.BeginFIndex)) { return 1; } else { return -1; } } #endregion #region Fields private double _beginFIndex; private double _endFIndex; #endregion } #endregion } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
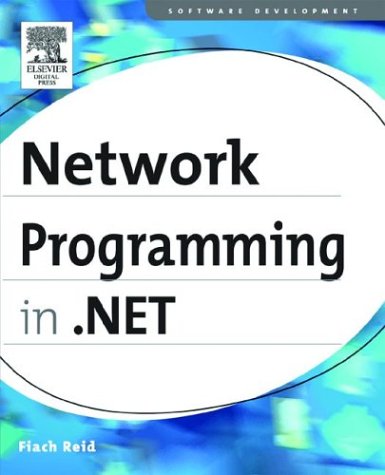
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SchemaNotation.cs
- JavaScriptObjectDeserializer.cs
- ExpressionVisitor.cs
- XmlILConstructAnalyzer.cs
- COM2TypeInfoProcessor.cs
- KeyMatchBuilder.cs
- ProcessModelInfo.cs
- SecurityHeaderLayout.cs
- InheritanceRules.cs
- ConfigurationStrings.cs
- DeadCharTextComposition.cs
- ObjectPropertyMapping.cs
- XamlReader.cs
- TCPClient.cs
- Environment.cs
- DetailsViewPagerRow.cs
- ToolStripPanel.cs
- UnknownBitmapEncoder.cs
- SimpleMailWebEventProvider.cs
- WriteableBitmap.cs
- ClientTarget.cs
- XmlTextWriter.cs
- ProxyWebPartManager.cs
- PropagatorResult.cs
- WpfGeneratedKnownTypes.cs
- DependencyPropertyDescriptor.cs
- MarkupCompiler.cs
- User.cs
- DateTimeValueSerializer.cs
- DataGridParentRows.cs
- InputLanguageEventArgs.cs
- FusionWrap.cs
- KeyToListMap.cs
- SiteMapNodeCollection.cs
- AssociativeAggregationOperator.cs
- OleDbError.cs
- SafeIUnknown.cs
- OpacityConverter.cs
- WebServiceErrorEvent.cs
- Utils.cs
- Point3D.cs
- ToolStripProgressBar.cs
- XmlQueryTypeFactory.cs
- FormViewPagerRow.cs
- AxImporter.cs
- RangeContentEnumerator.cs
- ProfessionalColors.cs
- SystemResourceHost.cs
- IteratorDescriptor.cs
- MruCache.cs
- Size3D.cs
- CodeLabeledStatement.cs
- MimeBasePart.cs
- SecurityTokenAuthenticator.cs
- UserControlParser.cs
- EventSetter.cs
- HatchBrush.cs
- LogoValidationException.cs
- Clipboard.cs
- ObjectContext.cs
- DataGrid.cs
- DispatchWrapper.cs
- TextSelectionHighlightLayer.cs
- MenuScrollingVisibilityConverter.cs
- ExpressionVisitorHelpers.cs
- DBConnection.cs
- PointConverter.cs
- COM2EnumConverter.cs
- UdpDiscoveryEndpointProvider.cs
- VirtualizingStackPanel.cs
- ResourceAttributes.cs
- ListChangedEventArgs.cs
- CellCreator.cs
- MemoryRecordBuffer.cs
- NavigationHelper.cs
- AnnotationDocumentPaginator.cs
- TableRow.cs
- ArraySortHelper.cs
- TextWriter.cs
- LinqDataSourceUpdateEventArgs.cs
- XmlDomTextWriter.cs
- ContainerParagraph.cs
- PropertyChangingEventArgs.cs
- _BasicClient.cs
- WindowClosedEventArgs.cs
- KeyValueSerializer.cs
- IPGlobalProperties.cs
- Stylus.cs
- ConnectionInterfaceCollection.cs
- MenuItemStyle.cs
- CodePageEncoding.cs
- RegexCompiler.cs
- HWStack.cs
- SubMenuStyle.cs
- ToolStripContentPanel.cs
- ViewKeyConstraint.cs
- ConnectionPointGlyph.cs
- Hash.cs
- SponsorHelper.cs
- FramingChannels.cs