Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / TransactionBridge / Microsoft / Transactions / Wsat / Messaging / Registration.cs / 1 / Registration.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- // Define the interfaces and infrastructure needed to receive registration messages using System; using System.ServiceModel.Channels; using System.Diagnostics; using System.ServiceModel; using System.Transactions; using Microsoft.Transactions.Wsat.Protocol; using DiagnosticUtility = Microsoft.Transactions.Bridge.DiagnosticUtility; namespace Microsoft.Transactions.Wsat.Messaging { enum ControlProtocol { None, Completion, Volatile2PC, Durable2PC, } interface IRegistrationCoordinator { void Register (Message message, RequestAsyncResult result); } class RegistrationCoordinatorDispatcher : RequestMessageDispatcher { CoordinationService service; IRegistrationCoordinator dispatch; public RegistrationCoordinatorDispatcher (CoordinationService service, IRegistrationCoordinator dispatch) { this.service = service; this.dispatch = dispatch; } protected override void SendFaultReply (RequestAsyncResult result, Fault fault) { RegistrationProxy.SendFaultResponse (result, fault); } public IAsyncResult BeginRegister(Message message, AsyncCallback callback, object state) { if (DebugTrace.Verbose) { DebugTrace.Trace(TraceLevel.Verbose, "Dispatching Register request"); if (DebugTrace.Pii) DebugTrace.TracePii(TraceLevel.Verbose, "Sender is {0}", CoordinationServiceSecurity.GetSenderName(message)); } RequestAsyncResult result = new RequestAsyncResult(message, callback, state); try { this.dispatch.Register(message, result); } catch (InvalidMessageException e) { DiagnosticUtility.ExceptionUtility.TraceHandledException(e, TraceEventType.Warning); this.OnMessageException(result, message, e, Faults.Version(this.service.ProtocolVersion).InvalidParameters); } catch (CommunicationException e) { DiagnosticUtility.ExceptionUtility.TraceHandledException(e, TraceEventType.Warning); this.OnMessageException(result, message, e, Faults.Version(this.service.ProtocolVersion).RegistrationDispatchFailed); } #pragma warning suppress 56500 // Only catch Exception for InvokeFinalHandler catch (Exception e) { DebugTrace.Trace( TraceLevel.Error, "Unhandled exception {0} dispatching Register message: {1}", e.GetType().Name, e ); // Contractually the dispatch object can only throw CommunicationException. // Our implementation is very careful to only throw this type of exception // (e.g. when deserializing a malformed message body or header...) // Any other exception indicates that things have gone seriously wrong, // so we cannot be trusted to process further messages. This is a QFE-worthy event. DiagnosticUtility.InvokeFinalHandler(e); } return result; } public Message EndRegister(IAsyncResult ar) { RequestAsyncResult result = (RequestAsyncResult) ar; result.End(); return result.Reply; } public static IWSRegistrationCoordinator Instance(CoordinationService service, IRegistrationCoordinator dispatch) { ProtocolVersionHelper.AssertProtocolVersion(service.ProtocolVersion, typeof(RegistrationCoordinatorDispatcher), "V"); //assert valid protocol version switch (service.ProtocolVersion) { case ProtocolVersion.Version10: return new RegistrationCoordinatorDispatcher10(service, dispatch); case ProtocolVersion.Version11: return new RegistrationCoordinatorDispatcher11(service, dispatch); default: return null; // inaccessible path because we have asserted the protocol version } } } [ServiceBehavior( InstanceContextMode = InstanceContextMode.Single, ConcurrencyMode = ConcurrencyMode.Multiple)] class RegistrationCoordinatorDispatcher10 : IWSRegistrationCoordinator10 { RegistrationCoordinatorDispatcher registrationCoordinatorDispatcher; public RegistrationCoordinatorDispatcher10(CoordinationService service, IRegistrationCoordinator dispatch) { ProtocolVersionHelper.AssertProtocolVersion10(service.ProtocolVersion, typeof(RegistrationCoordinatorDispatcher10), "constr"); this.registrationCoordinatorDispatcher = new RegistrationCoordinatorDispatcher(service, dispatch); } public Type ContractType { get { return typeof(IWSRegistrationCoordinator10); } } public IAsyncResult BeginRegister(Message message, AsyncCallback callback, object state) { return this.registrationCoordinatorDispatcher.BeginRegister(message, callback, state); } public Message EndRegister(IAsyncResult ar) { return this.registrationCoordinatorDispatcher.EndRegister(ar); } } [ServiceBehavior( InstanceContextMode = InstanceContextMode.Single, ConcurrencyMode = ConcurrencyMode.Multiple)] class RegistrationCoordinatorDispatcher11 : IWSRegistrationCoordinator11 { RegistrationCoordinatorDispatcher registrationCoordinatorDispatcher; public RegistrationCoordinatorDispatcher11(CoordinationService service, IRegistrationCoordinator dispatch) { ProtocolVersionHelper.AssertProtocolVersion11(service.ProtocolVersion, typeof(RegistrationCoordinatorDispatcher11), "constr"); this.registrationCoordinatorDispatcher = new RegistrationCoordinatorDispatcher(service, dispatch); } public Type ContractType { get { return typeof(IWSRegistrationCoordinator11); } } public IAsyncResult BeginRegister(Message message, AsyncCallback callback, object state) { return this.registrationCoordinatorDispatcher.BeginRegister(message, callback, state); } public Message EndRegister(IAsyncResult ar) { return this.registrationCoordinatorDispatcher.EndRegister(ar); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
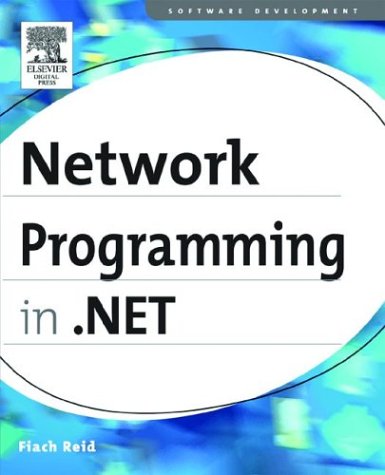
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- EdmComplexTypeAttribute.cs
- RuntimeConfigurationRecord.cs
- UseAttributeSetsAction.cs
- WorkItem.cs
- XmlDeclaration.cs
- iisPickupDirectory.cs
- safex509handles.cs
- Crypto.cs
- GPStream.cs
- BindUriHelper.cs
- Gdiplus.cs
- LinearGradientBrush.cs
- WinFormsComponentEditor.cs
- EmptyElement.cs
- TransformerConfigurationWizardBase.cs
- Effect.cs
- HttpContext.cs
- RawStylusInputCustomData.cs
- SchemaRegistration.cs
- PanelStyle.cs
- AncillaryOps.cs
- SymmetricKeyWrap.cs
- ControlValuePropertyAttribute.cs
- COM2IProvidePropertyBuilderHandler.cs
- CalendarDayButton.cs
- PostBackOptions.cs
- TimeoutException.cs
- DBCSCodePageEncoding.cs
- PnrpPeerResolver.cs
- StaticDataManager.cs
- ExecutionContext.cs
- WindowsClaimSet.cs
- ExtendedPropertyDescriptor.cs
- XmlnsCache.cs
- TransactionInformation.cs
- TextEditorSelection.cs
- List.cs
- Logging.cs
- NullableDoubleMinMaxAggregationOperator.cs
- SessionEndingCancelEventArgs.cs
- ClientScriptManagerWrapper.cs
- NameValueConfigurationCollection.cs
- ManipulationInertiaStartingEventArgs.cs
- xdrvalidator.cs
- AlphabeticalEnumConverter.cs
- StorageRoot.cs
- ProfileGroupSettings.cs
- AQNBuilder.cs
- DataContractFormatAttribute.cs
- CreatingCookieEventArgs.cs
- ScrollBarAutomationPeer.cs
- FileDataSourceCache.cs
- CodeTypeDeclaration.cs
- WebServiceData.cs
- PropertyMap.cs
- ToolStripDropDownButton.cs
- DependentTransaction.cs
- HScrollProperties.cs
- Math.cs
- Pkcs9Attribute.cs
- ErrorWrapper.cs
- RestHandlerFactory.cs
- exports.cs
- PointLightBase.cs
- DPCustomTypeDescriptor.cs
- WindowsServiceCredential.cs
- DbProviderConfigurationHandler.cs
- TypeDelegator.cs
- AsynchronousChannel.cs
- MiniParameterInfo.cs
- SplitContainer.cs
- SessionEndingCancelEventArgs.cs
- OdbcParameterCollection.cs
- TypeElement.cs
- DataShape.cs
- XmlCharCheckingWriter.cs
- EnvelopedSignatureTransform.cs
- BaseProcessor.cs
- EdmItemError.cs
- _SslSessionsCache.cs
- BitConverter.cs
- SiteMapNodeCollection.cs
- ScopedKnownTypes.cs
- ImageListStreamer.cs
- IsolatedStoragePermission.cs
- DbTransaction.cs
- DataServiceQueryException.cs
- StatusStrip.cs
- Int32.cs
- TemplateInstanceAttribute.cs
- HelpKeywordAttribute.cs
- Subset.cs
- DeclarativeConditionsCollection.cs
- ClientSponsor.cs
- XhtmlMobileTextWriter.cs
- JsonUriDataContract.cs
- EncryptedReference.cs
- Collection.cs
- CacheHelper.cs
- UnitySerializationHolder.cs