Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / clr / src / BCL / System / Reflection / Emit / ParameterBuilder.cs / 1 / ParameterBuilder.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== /*============================================================ ** ** Class: ParameterBuilder ** ** ** ParameterBuilder is used to create/associate parameter information ** ** ===========================================================*/ namespace System.Reflection.Emit { using System.Runtime.InteropServices; using System; using System.Reflection; using System.Security.Permissions; [ClassInterface(ClassInterfaceType.None)] [ComDefaultInterface(typeof(_ParameterBuilder))] [System.Runtime.InteropServices.ComVisible(true)] public class ParameterBuilder : _ParameterBuilder { // set ParamMarshal [Obsolete("An alternate API is available: Emit the MarshalAs custom attribute instead. http://go.microsoft.com/fwlink/?linkid=14202")] public virtual void SetMarshal(UnmanagedMarshal unmanagedMarshal) { if (unmanagedMarshal == null) { throw new ArgumentNullException("unmanagedMarshal"); } byte [] ubMarshal = unmanagedMarshal.InternalGetBytes(); TypeBuilder.InternalSetMarshalInfo( m_methodBuilder.GetModule(), m_pdToken.Token, ubMarshal, ubMarshal.Length); } // Set the default value of the parameter public virtual void SetConstant(Object defaultValue) { TypeBuilder.SetConstantValue( m_methodBuilder.GetModule(), m_pdToken.Token, m_iPosition == 0 ? m_methodBuilder.m_returnType : m_methodBuilder.m_parameterTypes[m_iPosition-1], defaultValue); } // Use this function if client decides to form the custom attribute blob themselves [System.Runtime.InteropServices.ComVisible(true)] public void SetCustomAttribute(ConstructorInfo con, byte[] binaryAttribute) { if (con == null) throw new ArgumentNullException("con"); if (binaryAttribute == null) throw new ArgumentNullException("binaryAttribute"); TypeBuilder.InternalCreateCustomAttribute( m_pdToken.Token, ((ModuleBuilder )m_methodBuilder.GetModule()).GetConstructorToken(con).Token, binaryAttribute, m_methodBuilder.GetModule(), false); } // Use this function if client wishes to build CustomAttribute using CustomAttributeBuilder public void SetCustomAttribute(CustomAttributeBuilder customBuilder) { if (customBuilder == null) { throw new ArgumentNullException("customBuilder"); } customBuilder.CreateCustomAttribute((ModuleBuilder) (m_methodBuilder .GetModule()), m_pdToken.Token); } //******************************* // Make a private constructor so these cannot be constructed externally. //******************************* private ParameterBuilder() {} internal ParameterBuilder( MethodBuilder methodBuilder, int sequence, ParameterAttributes attributes, String strParamName) // can be NULL string { m_iPosition = sequence; m_strParamName = strParamName; m_methodBuilder = methodBuilder; m_strParamName = strParamName; m_attributes = attributes; m_pdToken = new ParameterToken( TypeBuilder.InternalSetParamInfo( m_methodBuilder.GetModule(), m_methodBuilder.GetToken().Token, sequence, attributes, strParamName)); } public virtual ParameterToken GetToken() { return m_pdToken; } void _ParameterBuilder.GetTypeInfoCount(out uint pcTInfo) { throw new NotImplementedException(); } void _ParameterBuilder.GetTypeInfo(uint iTInfo, uint lcid, IntPtr ppTInfo) { throw new NotImplementedException(); } void _ParameterBuilder.GetIDsOfNames([In] ref Guid riid, IntPtr rgszNames, uint cNames, uint lcid, IntPtr rgDispId) { throw new NotImplementedException(); } void _ParameterBuilder.Invoke(uint dispIdMember, [In] ref Guid riid, uint lcid, short wFlags, IntPtr pDispParams, IntPtr pVarResult, IntPtr pExcepInfo, IntPtr puArgErr) { throw new NotImplementedException(); } internal virtual int MetadataTokenInternal { get { return m_pdToken.Token; } } public virtual String Name { get {return m_strParamName;} } public virtual int Position { get {return m_iPosition;} } // < public virtual int Attributes { get {return (int) m_attributes;} } public bool IsIn { get {return ((m_attributes & ParameterAttributes.In) != 0);} } public bool IsOut { get {return ((m_attributes & ParameterAttributes.Out) != 0);} } public bool IsOptional { get {return ((m_attributes & ParameterAttributes.Optional) != 0);} } private String m_strParamName; private int m_iPosition; private ParameterAttributes m_attributes; private MethodBuilder m_methodBuilder; private ParameterToken m_pdToken; } }
Link Menu
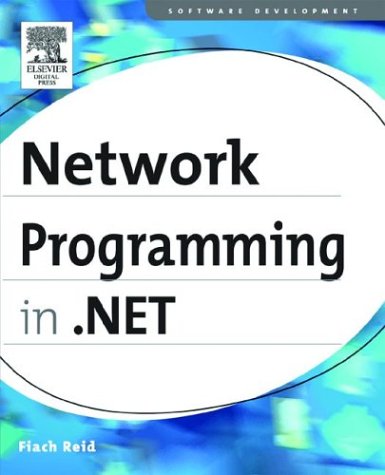
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- BufferModesCollection.cs
- SqlSupersetValidator.cs
- RC2CryptoServiceProvider.cs
- DashStyle.cs
- ReliableChannelFactory.cs
- DocumentGridContextMenu.cs
- SoapMessage.cs
- GridViewCommandEventArgs.cs
- __Error.cs
- MemoryRecordBuffer.cs
- WebEncodingValidator.cs
- FileLoadException.cs
- ColorAnimationUsingKeyFrames.cs
- ExtensionSimplifierMarkupObject.cs
- ContextBase.cs
- EventArgs.cs
- SmtpFailedRecipientException.cs
- XmlILIndex.cs
- DoubleAnimationBase.cs
- ObjectItemCollection.cs
- AssociationProvider.cs
- ColumnMap.cs
- InstancePersistenceContext.cs
- TableLayout.cs
- webeventbuffer.cs
- Matrix3D.cs
- NetSectionGroup.cs
- SQLBinaryStorage.cs
- HttpCachePolicy.cs
- TextEncodedRawTextWriter.cs
- DirectoryNotFoundException.cs
- InvalidComObjectException.cs
- CustomAttributeSerializer.cs
- TransactionFormatter.cs
- WorkflowFormatterBehavior.cs
- BinaryConverter.cs
- Logging.cs
- SerializationHelper.cs
- SiteMapHierarchicalDataSourceView.cs
- MobileControlPersister.cs
- TextChangedEventArgs.cs
- httpstaticobjectscollection.cs
- XmlUtf8RawTextWriter.cs
- SqlNodeTypeOperators.cs
- TextAutomationPeer.cs
- ThrowHelper.cs
- Block.cs
- XmlStringTable.cs
- AccessKeyManager.cs
- PanelStyle.cs
- DataServiceException.cs
- ChannelManagerHelpers.cs
- SqlException.cs
- ButtonChrome.cs
- validation.cs
- WindowClosedEventArgs.cs
- TcpServerChannel.cs
- CurrencyManager.cs
- UdpSocket.cs
- BoundColumn.cs
- HashHelpers.cs
- AttributeTable.cs
- DataGrid.cs
- Literal.cs
- TextMessageEncoder.cs
- PermissionToken.cs
- TemplateXamlTreeBuilder.cs
- EngineSite.cs
- LazyTextWriterCreator.cs
- FixedDocument.cs
- DataGridViewMethods.cs
- PermissionSet.cs
- ProviderUtil.cs
- LocatorPart.cs
- selecteditemcollection.cs
- DeclarationUpdate.cs
- _ConnectOverlappedAsyncResult.cs
- ConfigurationPermission.cs
- PathTooLongException.cs
- RegistryConfigurationProvider.cs
- StringToken.cs
- EncoderBestFitFallback.cs
- DataGridViewCellParsingEventArgs.cs
- invalidudtexception.cs
- DbExpressionVisitor.cs
- ArrayExtension.cs
- InputLanguage.cs
- CompiledAction.cs
- TemplatedAdorner.cs
- FastPropertyAccessor.cs
- WebPartAuthorizationEventArgs.cs
- DetailsViewInsertedEventArgs.cs
- WindowsFormsHost.cs
- dataSvcMapFileLoader.cs
- ActivityWithResultValueSerializer.cs
- DataServiceHost.cs
- FolderLevelBuildProvider.cs
- Bitmap.cs
- FileUtil.cs
- ListViewAutomationPeer.cs