Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / ndp / fx / src / DataWeb / Server / System / Data / Services / IgnorePropertiesAttribute.cs / 1 / IgnorePropertiesAttribute.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// CLR attribute to be annotated on types which indicate the list of properties // to ignore. // // // @owner [....] //--------------------------------------------------------------------- namespace System.Data.Services { using System; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Reflection; using System.Diagnostics; using System.Data.Services.Providers; ///Attribute to be annotated on types with ETags. [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Design", "CA1019:DefineAccessorsForAttributeArguments", Justification = "Processed values are available.")] [AttributeUsage(AttributeTargets.Class, AllowMultiple = false, Inherited = true)] public sealed class IgnorePropertiesAttribute : System.Attribute { ///Name of the properties that form the ETag. private readonly ReadOnlyCollectionpropertyNames; // This constructor was added since string[] is not a CLS-compliant type and // compiler gives a warning as error saying this attribute doesn't have any // constructor that takes CLS-compliant type /// /// Initializes a new instance of IgnoreProperties attribute with the property name /// that needs to be ignored /// /// Name of the property that form the ETag for the current type. public IgnorePropertiesAttribute(string propertyName) { WebUtil.CheckArgumentNull(propertyName, "propertyName"); this.propertyNames = new ReadOnlyCollection(new List (new string[1] { propertyName })); } /// /// Initializes a new instance of IgnoreProperties attribute with the list of property names /// that need to be ignored /// /// Name of the properties that form the ETag for the current type. public IgnorePropertiesAttribute(params string[] propertyNames) { WebUtil.CheckArgumentNull(propertyNames, "propertyNames"); if (propertyNames.Length == 0) { throw new ArgumentException(Strings.ETagAttribute_MustSpecifyAtleastOnePropertyName, "propertyNames"); } this.propertyNames = new ReadOnlyCollection(new List (propertyNames)); } /// Name of the properties that needs to be ignored for the current type. public ReadOnlyCollectionPropertyNames { get { return this.propertyNames; } } /// /// Validate and get the list of properties specified by this attribute on the given type. /// /// clr type on which this attribute must have defined. /// whether we need to inherit this attribute or not. /// For context types,we need to, since we can have one context dervied from another, and we want to ignore all the properties on the base ones too. /// For resource types, we don't need to, since we don't want derived types to know about ignore properties of the base type. Also /// from derived type, you cannot change the definition of the base type. /// binding flags to be used for validating property names. ///list of property names specified on IgnoreProperties on the given type. internal static IEnumerableGetProperties(Type type, bool inherit, BindingFlags bindingFlags) { IgnorePropertiesAttribute[] attributes = (IgnorePropertiesAttribute[])type.GetCustomAttributes(typeof(IgnorePropertiesAttribute), inherit); Debug.Assert(attributes.Length == 0 || attributes.Length == 1, "There should be atmost one IgnoreProperties specified"); if (attributes.Length == 1) { foreach (string propertyName in attributes[0].PropertyNames) { if (String.IsNullOrEmpty(propertyName)) { throw new InvalidOperationException(Strings.IgnorePropertiesAttribute_PropertyNameCannotBeNullOrEmpty); } PropertyInfo property = type.GetProperty(propertyName, bindingFlags); if (property == null) { throw new InvalidOperationException(Strings.IgnorePropertiesAttribute_InvalidPropertyName(propertyName, type.FullName)); } } return attributes[0].PropertyNames; } return WebUtil.EmptyStringArray; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // //// CLR attribute to be annotated on types which indicate the list of properties // to ignore. // // // @owner [....] //--------------------------------------------------------------------- namespace System.Data.Services { using System; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Reflection; using System.Diagnostics; using System.Data.Services.Providers; ///Attribute to be annotated on types with ETags. [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Design", "CA1019:DefineAccessorsForAttributeArguments", Justification = "Processed values are available.")] [AttributeUsage(AttributeTargets.Class, AllowMultiple = false, Inherited = true)] public sealed class IgnorePropertiesAttribute : System.Attribute { ///Name of the properties that form the ETag. private readonly ReadOnlyCollectionpropertyNames; // This constructor was added since string[] is not a CLS-compliant type and // compiler gives a warning as error saying this attribute doesn't have any // constructor that takes CLS-compliant type /// /// Initializes a new instance of IgnoreProperties attribute with the property name /// that needs to be ignored /// /// Name of the property that form the ETag for the current type. public IgnorePropertiesAttribute(string propertyName) { WebUtil.CheckArgumentNull(propertyName, "propertyName"); this.propertyNames = new ReadOnlyCollection(new List (new string[1] { propertyName })); } /// /// Initializes a new instance of IgnoreProperties attribute with the list of property names /// that need to be ignored /// /// Name of the properties that form the ETag for the current type. public IgnorePropertiesAttribute(params string[] propertyNames) { WebUtil.CheckArgumentNull(propertyNames, "propertyNames"); if (propertyNames.Length == 0) { throw new ArgumentException(Strings.ETagAttribute_MustSpecifyAtleastOnePropertyName, "propertyNames"); } this.propertyNames = new ReadOnlyCollection(new List (propertyNames)); } /// Name of the properties that needs to be ignored for the current type. public ReadOnlyCollectionPropertyNames { get { return this.propertyNames; } } /// /// Validate and get the list of properties specified by this attribute on the given type. /// /// clr type on which this attribute must have defined. /// whether we need to inherit this attribute or not. /// For context types,we need to, since we can have one context dervied from another, and we want to ignore all the properties on the base ones too. /// For resource types, we don't need to, since we don't want derived types to know about ignore properties of the base type. Also /// from derived type, you cannot change the definition of the base type. /// binding flags to be used for validating property names. ///list of property names specified on IgnoreProperties on the given type. internal static IEnumerableGetProperties(Type type, bool inherit, BindingFlags bindingFlags) { IgnorePropertiesAttribute[] attributes = (IgnorePropertiesAttribute[])type.GetCustomAttributes(typeof(IgnorePropertiesAttribute), inherit); Debug.Assert(attributes.Length == 0 || attributes.Length == 1, "There should be atmost one IgnoreProperties specified"); if (attributes.Length == 1) { foreach (string propertyName in attributes[0].PropertyNames) { if (String.IsNullOrEmpty(propertyName)) { throw new InvalidOperationException(Strings.IgnorePropertiesAttribute_PropertyNameCannotBeNullOrEmpty); } PropertyInfo property = type.GetProperty(propertyName, bindingFlags); if (property == null) { throw new InvalidOperationException(Strings.IgnorePropertiesAttribute_InvalidPropertyName(propertyName, type.FullName)); } } return attributes[0].PropertyNames; } return WebUtil.EmptyStringArray; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
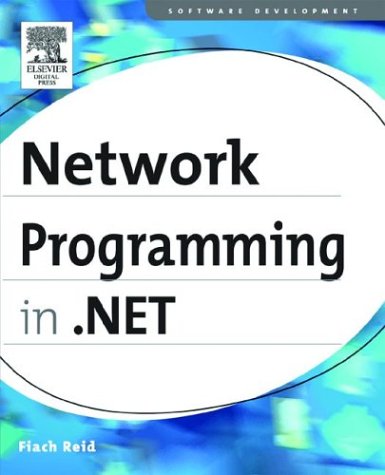
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- AffineTransform3D.cs
- EventLogEntry.cs
- ProtectedConfiguration.cs
- BuilderInfo.cs
- Tile.cs
- VirtualPath.cs
- Splitter.cs
- WeakEventManager.cs
- WebConfigurationHostFileChange.cs
- HwndStylusInputProvider.cs
- CounterNameConverter.cs
- WorkflowRuntimeService.cs
- ReflectionPermission.cs
- printdlgexmarshaler.cs
- XmlExceptionHelper.cs
- UnsafeNativeMethods.cs
- Profiler.cs
- ProfileSettingsCollection.cs
- PersonalizationAdministration.cs
- RadioButton.cs
- Monitor.cs
- OrderPreservingPipeliningSpoolingTask.cs
- SqlDataSourceConnectionPanel.cs
- MetadataSource.cs
- ImageDrawing.cs
- QueryTaskGroupState.cs
- AsyncStreamReader.cs
- StandardToolWindows.cs
- SqlPersonalizationProvider.cs
- RunWorkerCompletedEventArgs.cs
- ScrollProviderWrapper.cs
- DbMetaDataCollectionNames.cs
- Error.cs
- Missing.cs
- SmiEventStream.cs
- BindingCompleteEventArgs.cs
- TabControlEvent.cs
- TableParagraph.cs
- ZipIOCentralDirectoryBlock.cs
- keycontainerpermission.cs
- RelatedPropertyManager.cs
- AtlasWeb.Designer.cs
- CommandValueSerializer.cs
- HybridDictionary.cs
- Literal.cs
- HwndKeyboardInputProvider.cs
- ParseHttpDate.cs
- DataServiceKeyAttribute.cs
- VerificationAttribute.cs
- UxThemeWrapper.cs
- AuthenticationModuleElementCollection.cs
- DataSourceControlBuilder.cs
- DummyDataSource.cs
- ListControl.cs
- ProxyGenerator.cs
- RowUpdatedEventArgs.cs
- RequestQueue.cs
- SectionRecord.cs
- WmpBitmapEncoder.cs
- LingerOption.cs
- BuildProvider.cs
- OleDbRowUpdatingEvent.cs
- WebPartHelpVerb.cs
- WindowsEditBox.cs
- GetLastErrorDetailsRequest.cs
- AssemblyUtil.cs
- CodeTypeDelegate.cs
- ViewManagerAttribute.cs
- ContentType.cs
- DynamicUpdateCommand.cs
- ZoneMembershipCondition.cs
- IndexedSelectQueryOperator.cs
- CellIdBoolean.cs
- MatrixTransform.cs
- KnownColorTable.cs
- MimeWriter.cs
- SiteMapNodeItemEventArgs.cs
- StandardBindingImporter.cs
- HttpCacheParams.cs
- SplitterEvent.cs
- TraceHandlerErrorFormatter.cs
- BamlResourceContent.cs
- SecurityHelper.cs
- DefaultTextStoreTextComposition.cs
- DocumentApplicationJournalEntry.cs
- XmlSchemaCollection.cs
- ToolboxComponentsCreatedEventArgs.cs
- TransformDescriptor.cs
- SecurityPolicySection.cs
- WebPartUserCapability.cs
- Exceptions.cs
- EventHandlersStore.cs
- SignedInfo.cs
- SamlSubject.cs
- RectangleConverter.cs
- peersecuritysettings.cs
- RowUpdatedEventArgs.cs
- SubpageParaClient.cs
- Win32.cs
- ToolBarTray.cs