Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / xsp / System / Web / UI / WebControls / DataBoundControlHelper.cs / 2 / DataBoundControlHelper.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls { using System; using System.Collections; using System.ComponentModel; using System.Data; using System.Security.Permissions; using System.Web.Util; ////// Helper class for DataBoundControls and v1 data controls. /// This is also used by ControlParameter to find its associated /// control. /// internal static class DataBoundControlHelper { ////// Walks up the stack of NamingContainers starting at 'control' to find a control with the ID 'controlID'. /// public static Control FindControl(Control control, string controlID) { Debug.Assert(control != null, "control should not be null"); Debug.Assert(!String.IsNullOrEmpty(controlID), "controlID should not be empty"); Control currentContainer = control; Control foundControl = null; if (control == control.Page) { // If we get to the Page itself while we're walking up the // hierarchy, just return whatever item we find (if anything) // since we can't walk any higher. return control.FindControl(controlID); } while (foundControl == null && currentContainer != control.Page) { currentContainer = currentContainer.NamingContainer; if (currentContainer == null) { throw new HttpException(SR.GetString(SR.DataBoundControlHelper_NoNamingContainer, control.GetType().Name, control.ID)); } foundControl = currentContainer.FindControl(controlID); } return foundControl; } ///// return true if the two string arrays have the same members /// public static bool CompareStringArrays(string[] stringA, string[] stringB) { if (stringA == null && stringB == null) { return true; } if (stringA == null || stringB == null) { return false; } if (stringA.Length != stringB.Length) { return false; } for (int i = 0; i < stringA.Length; i++) { if (!String.Equals(stringA[i], stringB[i], StringComparison.Ordinal)) { return false; } } return true; } // Returns true for types that can be automatically databound in controls such as // GridView and DetailsView. Bindable types are simple types, such as primitives, strings, // and nullable primitives. public static bool IsBindableType(Type type) { if (type == null) { return false; } Type underlyingType = Nullable.GetUnderlyingType(type); if (underlyingType != null) { // If the type is Nullable then it has an underlying type, in which case // we want to check the underlying type for bindability. type = underlyingType; } return (type.IsPrimitive || (type == typeof(string)) || (type == typeof(DateTime)) || (type == typeof(Decimal)) || (type == typeof(Guid))); } } }
Link Menu
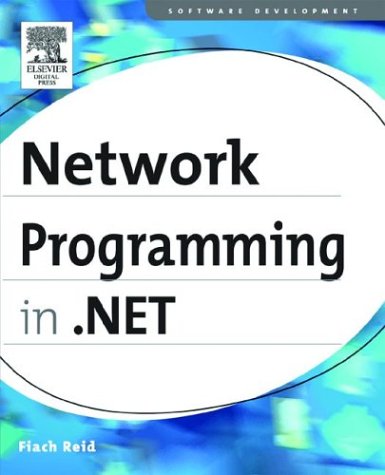
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CompensationHandlingFilter.cs
- IISMapPath.cs
- SqlServer2KCompatibilityCheck.cs
- SectionUpdates.cs
- ProcessStartInfo.cs
- BlobPersonalizationState.cs
- httpapplicationstate.cs
- TableAdapterManagerGenerator.cs
- TypeElement.cs
- ThrowHelper.cs
- CorePropertiesFilter.cs
- DescendantBaseQuery.cs
- ResourceType.cs
- DoubleConverter.cs
- RequestResizeEvent.cs
- SamlConstants.cs
- CellCreator.cs
- TextServicesContext.cs
- ReadOnlyMetadataCollection.cs
- DelegateInArgument.cs
- ToolboxComponentsCreatingEventArgs.cs
- WebPartConnection.cs
- ExpressionVisitor.cs
- FontResourceCache.cs
- processwaithandle.cs
- EntityClientCacheEntry.cs
- JsonDataContract.cs
- Figure.cs
- BinaryObjectReader.cs
- exports.cs
- CustomActivityDesigner.cs
- UIElement3D.cs
- URI.cs
- MDIWindowDialog.cs
- DeviceContexts.cs
- RowType.cs
- ObjectListDesigner.cs
- Parallel.cs
- CqlGenerator.cs
- EncryptedXml.cs
- DataStreamFromComStream.cs
- InvalidPropValue.cs
- ListViewItemCollectionEditor.cs
- RectValueSerializer.cs
- PopOutPanel.cs
- NamespaceDecl.cs
- CaretElement.cs
- RawAppCommandInputReport.cs
- CannotUnloadAppDomainException.cs
- CodeChecksumPragma.cs
- ToolStripSplitStackLayout.cs
- TimelineGroup.cs
- DataGridViewRowsAddedEventArgs.cs
- StringReader.cs
- PrintEvent.cs
- QilDataSource.cs
- Pair.cs
- Context.cs
- OLEDB_Enum.cs
- ClientWindowsAuthenticationMembershipProvider.cs
- MemoryRecordBuffer.cs
- PrinterResolution.cs
- Boolean.cs
- ExtendedProtectionPolicy.cs
- FontCacheUtil.cs
- TransportBindingElementImporter.cs
- FileDialogCustomPlace.cs
- MimeObjectFactory.cs
- Permission.cs
- ScriptManager.cs
- _HeaderInfo.cs
- SecurityContext.cs
- MulticastDelegate.cs
- ConnectionStringSettingsCollection.cs
- CommentAction.cs
- ProxyGenerator.cs
- InstanceLockException.cs
- SqlCommandSet.cs
- CodePrimitiveExpression.cs
- XmlSchemaFacet.cs
- DateTimePicker.cs
- RectangleConverter.cs
- ToolStripSeparator.cs
- Pair.cs
- DataGridViewColumnDesignTimeVisibleAttribute.cs
- PrinterUnitConvert.cs
- ValueHandle.cs
- WSSecurityPolicy12.cs
- TriState.cs
- StickyNoteAnnotations.cs
- InfoCardRSAOAEPKeyExchangeFormatter.cs
- StrokeNodeEnumerator.cs
- HandledMouseEvent.cs
- DesignTimeVisibleAttribute.cs
- WindowsAuthenticationModule.cs
- TaskHelper.cs
- Tracking.cs
- DataGridViewRowErrorTextNeededEventArgs.cs
- FrameDimension.cs
- XmlNode.cs