Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / Designer / System / data / design / TableAdapterManagerGenerator.cs / 3 / TableAdapterManagerGenerator.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All Rights Reserved. // Information Contained Herein is Proprietary and Confidential. // //----------------------------------------------------------------------------- namespace System.Data.Design { using System.Diagnostics; using System; using System.IO; using System.Data; using System.CodeDom; using System.Text; using System.Xml; using System.Collections; using System.Collections.Generic; using System.Reflection; using System.Globalization; using System.CodeDom.Compiler; using System.Runtime.InteropServices; ////// This class is used to generate the TableAdapterManager in the Hierarchical Update feature /// TypedDataSourceCodeGenerator will instanciate this class to generate TableAdapterManager related code. /// internal sealed class TableAdapterManagerGenerator { private TypedDataSourceCodeGenerator dataSourceGenerator = null; private const string adapterDesigner = "Microsoft.VSDesigner.DataSource.Design.TableAdapterManagerDesigner"; private const string helpKeyword = "vs.data.TableAdapterManager"; internal TableAdapterManagerGenerator(TypedDataSourceCodeGenerator codeGenerator) { this.dataSourceGenerator = codeGenerator; } internal CodeTypeDeclaration GenerateAdapterManager(DesignDataSource dataSource, CodeTypeDeclaration dataSourceClass) { // Create CodeTypeDeclaration // Type is internal if any TableAdapter is internal // TypeAttributes typeAttributes = TypeAttributes.Public; foreach (DesignTable table in dataSource.DesignTables) { if ((table.DataAccessorModifier & TypeAttributes.Public) != TypeAttributes.Public) { typeAttributes = table.DataAccessorModifier; } } CodeTypeDeclaration dataComponentClass = CodeGenHelper.Class(TableAdapterManagerNameHandler.TableAdapterManagerClassName, true, typeAttributes); dataComponentClass.Comments.Add(CodeGenHelper.Comment("TableAdapterManager is used to coordinate TableAdapters in the dataset to enable Hierarchical Update scenarios", true)); dataComponentClass.BaseTypes.Add(CodeGenHelper.GlobalType(typeof(ComponentModel.Component))); // Set Attributes dataComponentClass.CustomAttributes.Add(CodeGenHelper.AttributeDecl("System.ComponentModel.DesignerCategoryAttribute", CodeGenHelper.Str("code"))); dataComponentClass.CustomAttributes.Add(CodeGenHelper.AttributeDecl("System.ComponentModel.ToolboxItem", CodeGenHelper.Primitive(true))); dataComponentClass.CustomAttributes.Add(CodeGenHelper.AttributeDecl("System.ComponentModel.DesignerAttribute", CodeGenHelper.Str(adapterDesigner + ", " + AssemblyRef.MicrosoftVSDesigner))); dataComponentClass.CustomAttributes.Add(CodeGenHelper.AttributeDecl(typeof(System.ComponentModel.Design.HelpKeywordAttribute).FullName, CodeGenHelper.Str(helpKeyword))); // Create and Init the TableAdapterManager Method Generator TableAdapterManagerMethodGenerator dcMethodGenerator = new TableAdapterManagerMethodGenerator(this.dataSourceGenerator, dataSource, dataSourceClass); // Generate methods dcMethodGenerator.AddEverything(dataComponentClass); // Make sure that what we added so far doesn't contain any code injection (for the queries we're going to add right // after this all user input is validated in DataComponentNameHandler, so we're safe there). try { System.CodeDom.Compiler.CodeGenerator.ValidateIdentifiers(dataComponentClass); } catch (Exception er) { Debug.Fail(er.ToString()); } return dataComponentClass; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
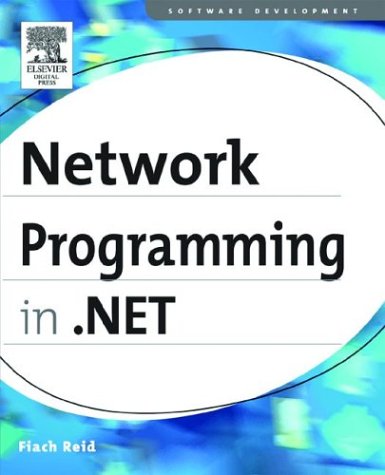
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TextOnlyOutput.cs
- PersistenceTypeAttribute.cs
- PageClientProxyGenerator.cs
- FileUtil.cs
- DataGridViewCellErrorTextNeededEventArgs.cs
- KeyValueSerializer.cs
- KoreanCalendar.cs
- OdbcParameter.cs
- FileDialogPermission.cs
- AccessibleObject.cs
- TemplateColumn.cs
- DynamicDocumentPaginator.cs
- HtmlTableRow.cs
- ResourceAttributes.cs
- DisplayMemberTemplateSelector.cs
- AppModelKnownContentFactory.cs
- SqlStatistics.cs
- SingleTagSectionHandler.cs
- GroupItemAutomationPeer.cs
- FontNameConverter.cs
- XslAstAnalyzer.cs
- M3DUtil.cs
- objectresult_tresulttype.cs
- AssemblyHash.cs
- DomNameTable.cs
- File.cs
- IconConverter.cs
- MemoryStream.cs
- ToolStripItemImageRenderEventArgs.cs
- Cursor.cs
- BitmapEffectOutputConnector.cs
- sqlstateclientmanager.cs
- PathSegmentCollection.cs
- HttpHeaderCollection.cs
- ClaimSet.cs
- ImportedNamespaceContextItem.cs
- XmlEntityReference.cs
- ProcessModule.cs
- FreezableDefaultValueFactory.cs
- Types.cs
- CalendarSelectionChangedEventArgs.cs
- TextFormatter.cs
- ContractTypeNameElement.cs
- DataGridViewComboBoxColumn.cs
- XmlSchemaException.cs
- NamedPipeConnectionPool.cs
- ListControl.cs
- XsltContext.cs
- EnumerableRowCollectionExtensions.cs
- SettingsProviderCollection.cs
- ControlCollection.cs
- NumericPagerField.cs
- ColumnClickEvent.cs
- MSAAEventDispatcher.cs
- RtfToXamlLexer.cs
- SQLCharsStorage.cs
- SmtpSpecifiedPickupDirectoryElement.cs
- TypeName.cs
- DataStreamFromComStream.cs
- MembershipValidatePasswordEventArgs.cs
- ConfigXmlSignificantWhitespace.cs
- HelpProvider.cs
- OdbcConnectionStringbuilder.cs
- MinimizableAttributeTypeConverter.cs
- HMACMD5.cs
- TracePayload.cs
- DomainLiteralReader.cs
- BaseTreeIterator.cs
- WebHttpDispatchOperationSelectorData.cs
- SmiMetaData.cs
- QuaternionAnimationBase.cs
- Condition.cs
- DataGridHeaderBorder.cs
- WorkflowInstanceExtensionCollection.cs
- PropertyEmitterBase.cs
- ExeContext.cs
- autovalidator.cs
- DetailsViewRow.cs
- HtmlContainerControl.cs
- WebPartConnectionsCloseVerb.cs
- GroupItemAutomationPeer.cs
- RegexRunner.cs
- ElementMarkupObject.cs
- SystemIPGlobalStatistics.cs
- DateTimeOffset.cs
- TextureBrush.cs
- MouseDevice.cs
- _ProxyRegBlob.cs
- SliderAutomationPeer.cs
- GreenMethods.cs
- EntitySqlQueryCacheKey.cs
- DataGridCommandEventArgs.cs
- TypedTableGenerator.cs
- SqlConnectionFactory.cs
- ObjectKeyFrameCollection.cs
- EnvironmentPermission.cs
- DesigntimeLicenseContextSerializer.cs
- ObjectComplexPropertyMapping.cs
- RSACryptoServiceProvider.cs
- VariantWrapper.cs