Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Core / System / Windows / Media / textformatting / TextFormatter.cs / 1 / TextFormatter.cs
//------------------------------------------------------------------------ // // Microsoft Windows Client Platform // Copyright (C) Microsoft Corporation, 2001 // // File: TextFormatter.cs // // Contents: Text formatting API // // Spec: [....]/sites/Avalon/Specs/Text%20Formatting%20API.doc // // Created: 2-25-2003 [....] ([....]) // //----------------------------------------------------------------------- using System; using System.Windows.Threading; using System.Collections.Generic; using MS.Internal; using MS.Internal.TextFormatting; using MS.Internal.PresentationCore; namespace System.Windows.Media.TextFormatting { ////// The TextFormatter is the Windows Client Platform text engine that provides services /// for formatting text and breaking text lines. It handles different text character /// formats with different paragraph styles. It also implements all the writing system rules /// for international text layout. /// /// Unlike traditional text APIs, the TextFormatter interacts with a text layout client /// through a set of callback methods. It requires the client to provide these methods /// in an implementation of the TextSource class. /// public abstract class TextFormatter : IDisposable { private static object _staticLock = new object(); ////// Client to create a new instance of TextFormatter /// ///New instance of TextFormatter static public TextFormatter Create() { // create a new instance of TextFormatter which allows the use of multiple contexts. return new TextFormatterImp(); } ////// Client to create a new instance of TextFormatter from the specified TextFormatter context /// /// TextFormatter context ///New instance of TextFormatter #if OPTIMALBREAK_API static public TextFormatter CreateFromContext(TextFormatterContext soleContext) #else [FriendAccessAllowed] // used by Framework static internal TextFormatter CreateFromContext(TextFormatterContext soleContext) #endif { // create a new instance of TextFormatter for the specified context. // This creation prohibits the use of multiple contexts within the same TextFormatter via reentrance. return new TextFormatterImp(soleContext); } ////// Client to get TextFormatter associated with the current dispatcher /// ////// This method is available internally. It is exclusively used by Presentation Framework UIElement /// thru friend assembly mechanics to quickly reuse the default TextFormatter retained in the current /// dispatcher of the running thread. /// [FriendAccessAllowed] // used by Framework static internal TextFormatter FromCurrentDispatcher() { Dispatcher dispatcher = Dispatcher.CurrentDispatcher; if (dispatcher == null) throw new ArgumentException(SR.Get(SRID.CurrentDispatcherNotFound)); TextFormatter defaultTextFormatter = (TextFormatterImp)dispatcher.Reserved1; if (defaultTextFormatter == null) { lock (_staticLock) { if (defaultTextFormatter == null) { // Default formatter has not been created for this dispatcher, // create a new one and stick it to the dispatcher. defaultTextFormatter = Create(); dispatcher.Reserved1 = defaultTextFormatter; } } } Invariant.Assert(defaultTextFormatter != null); return defaultTextFormatter; } ////// Clean up text formatter internal resource /// public virtual void Dispose() {} ////// Client to format a text line that fills a paragraph in the document. /// /// an object representing text layout clients text source for TextFormatter. /// character index to specify where in the source text the line starts /// width of paragraph in which the line fills /// properties that can change from one paragraph to the next, such as text flow direction, text alignment, or indentation. /// text formatting state at the point where the previous line in the paragraph /// was broken by the text formatting process, as specified by the TextLine.LineBreak property for the previous /// line; this parameter can be null, and will always be null for the first line in a paragraph. ///object representing a line of text that client interacts with. public abstract TextLine FormatLine( TextSource textSource, int firstCharIndex, double paragraphWidth, TextParagraphProperties paragraphProperties, TextLineBreak previousLineBreak ); ////// Client to format a text line that fills a paragraph in the document. /// /// an object representing text layout clients text source for TextFormatter. /// character index to specify where in the source text the line starts /// width of paragraph in which the line fills /// properties that can change from one paragraph to the next, such as text flow direction, text alignment, or indentation. /// text formatting state at the point where the previous line in the paragraph /// was broken by the text formatting process, as specified by the TextLine.LineBreak property for the previous /// line; this parameter can be null, and will always be null for the first line in a paragraph. /// an object representing content cache of the client. ///object representing a line of text that client interacts with. public abstract TextLine FormatLine( TextSource textSource, int firstCharIndex, double paragraphWidth, TextParagraphProperties paragraphProperties, TextLineBreak previousLineBreak, TextRunCache textRunCache ); ////// Client to reconstruct a previously formatted text line /// /// an object representing text layout clients text source for TextFormatter. /// character index to specify where in the source text the line starts /// character length of the line /// width of paragraph in which the line fills /// properties that can change from one paragraph to the next, such as text flow direction, text alignment, or indentation. /// LineBreak property of the previous text line, or null if this is the first line in the paragraph /// an object representing content cache of the client. ///object representing a line of text that client interacts with. #if OPTIMALBREAK_API public abstract TextLine RecreateLine( #else [FriendAccessAllowed] // used by Framework internal abstract TextLine RecreateLine( #endif TextSource textSource, int firstCharIndex, int lineLength, double paragraphWidth, TextParagraphProperties paragraphProperties, TextLineBreak previousLineBreak, TextRunCache textRunCache ); ////// Client to cache information about a paragraph to be used during optimal paragraph line formatting /// /// an object representing text layout clients text source for TextFormatter. /// character index to specify where in the source text the line starts /// width of paragraph in which the line fills /// properties that can change from one paragraph to the next, such as text flow direction, text alignment, or indentation. /// text formatting state at the point where the previous line in the paragraph /// was broken by the text formatting process, as specified by the TextLine.LineBreak property for the previous /// line; this parameter can be null, and will always be null for the first line in a paragraph. /// an object representing content cache of the client. ///object representing a line of text that client interacts with. #if OPTIMALBREAK_API public abstract TextParagraphCache CreateParagraphCache( #else [FriendAccessAllowed] // used by Framework internal abstract TextParagraphCache CreateParagraphCache( #endif TextSource textSource, int firstCharIndex, double paragraphWidth, TextParagraphProperties paragraphProperties, TextLineBreak previousLineBreak, TextRunCache textRunCache ); ////// Client to ask for the possible smallest and largest paragraph width that can fully contain the passing text content /// /// an object representing text layout clients text source for TextFormatter. /// character index to specify where in the source text the line starts /// properties that can change from one paragraph to the next, such as text flow direction, text alignment, or indentation. ///min max paragraph width public abstract MinMaxParagraphWidth FormatMinMaxParagraphWidth( TextSource textSource, int firstCharIndex, TextParagraphProperties paragraphProperties ); ////// Client to ask for the possible smallest and largest paragraph width that can fully contain the passing text content /// /// an object representing text layout clients text source for TextFormatter. /// character index to specify where in the source text the line starts /// properties that can change from one paragraph to the next, such as text flow direction, text alignment, or indentation. /// an object representing content cache of the client. ///min max paragraph width public abstract MinMaxParagraphWidth FormatMinMaxParagraphWidth( TextSource textSource, int firstCharIndex, TextParagraphProperties paragraphProperties, TextRunCache textRunCache ); } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
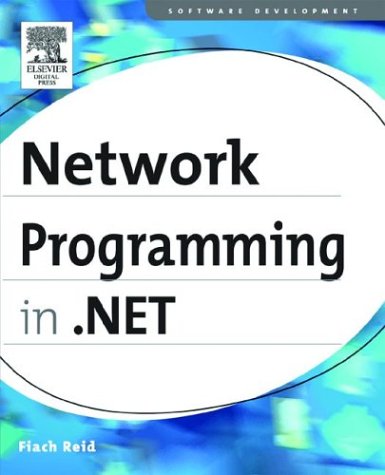
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ToolstripProfessionalRenderer.cs
- DataGridViewCellPaintingEventArgs.cs
- PointAnimationBase.cs
- HttpHandlerActionCollection.cs
- MemoryRecordBuffer.cs
- TaskFactory.cs
- PrtTicket_Base.cs
- MemberMaps.cs
- ViewgenContext.cs
- OleDbConnectionPoolGroupProviderInfo.cs
- DelegatedStream.cs
- WSFederationHttpBinding.cs
- AccessKeyManager.cs
- SQLString.cs
- StaticExtension.cs
- EventProviderWriter.cs
- CacheModeConverter.cs
- ColorTransform.cs
- TransformProviderWrapper.cs
- ConfigXmlReader.cs
- GeneralTransform3DGroup.cs
- Queue.cs
- IteratorDescriptor.cs
- DictationGrammar.cs
- AttachedPropertyInfo.cs
- AutomationIdentifier.cs
- DictionaryMarkupSerializer.cs
- PackUriHelper.cs
- OutOfMemoryException.cs
- CategoryAttribute.cs
- NamespaceList.cs
- PeerCollaboration.cs
- ColorConverter.cs
- UnknownWrapper.cs
- BoundConstants.cs
- SimpleWebHandlerParser.cs
- BuilderElements.cs
- PtsCache.cs
- PenContext.cs
- XamlFigureLengthSerializer.cs
- EmbossBitmapEffect.cs
- securitycriticaldataClass.cs
- FaultHandlingFilter.cs
- StringAnimationBase.cs
- Italic.cs
- RuntimeResourceSet.cs
- DataControlButton.cs
- CompositeCollectionView.cs
- DirectoryObjectSecurity.cs
- FontFamily.cs
- ZipIOZip64EndOfCentralDirectoryLocatorBlock.cs
- SoapAttributes.cs
- RegexStringValidator.cs
- Table.cs
- BuildResultCache.cs
- Int32KeyFrameCollection.cs
- HttpCacheParams.cs
- XmlHelper.cs
- GACMembershipCondition.cs
- HttpResponseHeader.cs
- BamlRecordHelper.cs
- DES.cs
- AsymmetricAlgorithm.cs
- WebPartActionVerb.cs
- ProjectedWrapper.cs
- SafeLibraryHandle.cs
- ModuleElement.cs
- CodeGeneratorAttribute.cs
- XmlBinaryReader.cs
- FontFamilyConverter.cs
- SamlAssertionKeyIdentifierClause.cs
- DBNull.cs
- ToolStripRenderer.cs
- DataGridCell.cs
- CodeDesigner.cs
- ImageAnimator.cs
- MessagePropertyDescriptionCollection.cs
- UrlPath.cs
- AspNetHostingPermission.cs
- XmlNodeChangedEventArgs.cs
- RegistrationServices.cs
- WCFBuildProvider.cs
- ObjectContextServiceProvider.cs
- sitestring.cs
- BooleanExpr.cs
- DataIdProcessor.cs
- FileNotFoundException.cs
- TextOutput.cs
- NamespaceCollection.cs
- GeometryGroup.cs
- InstanceStoreQueryResult.cs
- DataGridViewRowConverter.cs
- CqlWriter.cs
- SafeSecurityHelper.cs
- WebScriptMetadataMessage.cs
- PathTooLongException.cs
- VectorCollection.cs
- LockRenewalTask.cs
- ProfessionalColors.cs
- LabelLiteral.cs