Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / Designer / WebForms / System / Web / UI / Design / TypeSchema.cs / 1 / TypeSchema.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.Design { using System; using System.Collections; using System.Collections.Generic; using System.Data; using System.Diagnostics; using System.Reflection; ////// Represents a schema based on an arbitrary object. /// /// DataSets and DataTables are special cased and processed for their strongly-typed properties. /// Types that implement the generic IEnumerable<T> will use the bound type T as their row type. /// Arbitrary enumerables are processed using their indexer's type. /// All other objects are directly reflected on and their public properties are exposed as fields. /// /// DataSet and DataTable schema is retrieved by creating instances of the /// objects, whereas all other schema is retrived using Reflection. /// [System.Security.Permissions.SecurityPermission(System.Security.Permissions.SecurityAction.Demand, Flags=System.Security.Permissions.SecurityPermissionFlag.UnmanagedCode)] public sealed class TypeSchema : IDataSourceSchema { private Type _type; private IDataSourceViewSchema[] _schema; public TypeSchema(Type type) { if (type == null) { throw new ArgumentNullException("type"); } _type = type; if (typeof(DataTable).IsAssignableFrom(_type)) { _schema = GetDataTableSchema(_type); } else { if (typeof(DataSet).IsAssignableFrom(_type)) { _schema = GetDataSetSchema(_type); } else { if (IsBoundGenericEnumerable(_type)) { _schema = GetGenericEnumerableSchema(_type); } else { if (typeof(IEnumerable).IsAssignableFrom(_type)) { _schema = GetEnumerableSchema(_type); } else { _schema = GetTypeSchema(_type); } } } } } public IDataSourceViewSchema[] GetViews() { return _schema; } ////// Gets schema for a strongly typed DataSet. /// private static IDataSourceViewSchema[] GetDataSetSchema(Type t) { try { DataSet dataSet = Activator.CreateInstance(t) as DataSet; System.Collections.Generic.Listviews = new System.Collections.Generic.List (); foreach (DataTable table in dataSet.Tables) { views.Add(new DataSetViewSchema(table)); } return views.ToArray(); } catch { return null; } } /// /// Gets schema for a strongly typed DataTable. /// private static IDataSourceViewSchema[] GetDataTableSchema(Type t) { try { DataTable table = Activator.CreateInstance(t) as DataTable; DataSetViewSchema tableSchema = new DataSetViewSchema(table); return new IDataSourceViewSchema[1] { tableSchema }; } catch { return null; } } ////// Gets schema for a strongly typed enumerable. /// private static IDataSourceViewSchema[] GetEnumerableSchema(Type t) { TypeEnumerableViewSchema enumerableSchema = new TypeEnumerableViewSchema(String.Empty, t); return new IDataSourceViewSchema[1] { enumerableSchema }; } ////// Gets schema for a generic IEnumerable. /// private static IDataSourceViewSchema[] GetGenericEnumerableSchema(Type t) { TypeGenericEnumerableViewSchema enumerableSchema = new TypeGenericEnumerableViewSchema(String.Empty, t); return new IDataSourceViewSchema[1] { enumerableSchema }; } ////// Gets schema for an arbitrary type. /// private static IDataSourceViewSchema[] GetTypeSchema(Type t) { TypeViewSchema typeSchema = new TypeViewSchema(String.Empty, t); return new IDataSourceViewSchema[1] { typeSchema }; } ////// Returns true if the type implements IEnumerable<T> with a bound /// value for T. In other words, this type: /// public class Foo<T> : IEnumerable<T> { ... } /// Does not bind the value of T. However this type: /// public class Foo2 : IEnumerable<string> { ... } /// Does have T bound to the type System.String. /// /// Although it would seem that this check: /// typeof(IEnumerable<>).IsAssignableFrom(t) /// Might be sufficient, it actually doesn't work, possibly due /// to covariance/contravariance limitations, etc. /// internal static bool IsBoundGenericEnumerable(Type t) { Type[] interfaces = null; if (t.IsInterface && t.IsGenericType && t.GetGenericTypeDefinition() == typeof(IEnumerable<>)) { // If the type already is IEnumerable, that's the interface we want to inspect interfaces = new Type[] { t }; } else { // Otherwise we get a list of all the interafaces the type implements interfaces = t.GetInterfaces(); } foreach (Type i in interfaces) { if (i.IsGenericType && i.GetGenericTypeDefinition() == typeof(IEnumerable<>)) { Type[] genericArguments = i.GetGenericArguments(); Debug.Assert(genericArguments != null && genericArguments.Length == 1, "Expected IEnumerable to have a generic argument"); // If a type has IsGenericParameter=true that means it is not bound. return !genericArguments[0].IsGenericParameter; } } return false; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
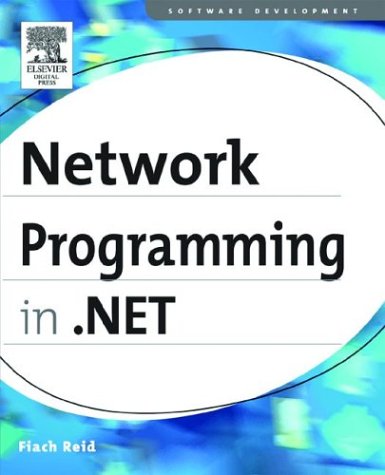
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- EntityClassGenerator.cs
- Button.cs
- RuleRefElement.cs
- METAHEADER.cs
- _IPv4Address.cs
- DispatcherExceptionEventArgs.cs
- DocumentSchemaValidator.cs
- ProxyHwnd.cs
- WebConfigurationHost.cs
- EncryptedPackage.cs
- MdiWindowListItemConverter.cs
- TracePayload.cs
- DependencyPropertyKey.cs
- EmbossBitmapEffect.cs
- BoundPropertyEntry.cs
- RadioButtonRenderer.cs
- XamlWriterExtensions.cs
- Parsers.cs
- RepeatBehaviorConverter.cs
- EmptyEnumerator.cs
- AVElementHelper.cs
- DataSourceXmlSerializer.cs
- DataServiceCollectionOfT.cs
- EdmItemError.cs
- SynchronizedDispatch.cs
- RtfToXamlReader.cs
- FilterEventArgs.cs
- SafeNativeMethods.cs
- MissingFieldException.cs
- WebHttpSecurityElement.cs
- InvalidPropValue.cs
- ResourceProviderFactory.cs
- RtType.cs
- ConstraintConverter.cs
- ChtmlImageAdapter.cs
- InternalSafeNativeMethods.cs
- ListViewTableCell.cs
- SplitterEvent.cs
- Repeater.cs
- LinkLabel.cs
- StaticExtensionConverter.cs
- XPathMessageFilterElementCollection.cs
- DelegateTypeInfo.cs
- ActiveXSite.cs
- SerializationAttributes.cs
- ToolStripSeparatorRenderEventArgs.cs
- Msmq3PoisonHandler.cs
- GridViewColumnCollectionChangedEventArgs.cs
- TagPrefixInfo.cs
- RepeatBehavior.cs
- ZipIOExtraField.cs
- MailWebEventProvider.cs
- DataGridViewCellParsingEventArgs.cs
- ListControl.cs
- UInt64Storage.cs
- XmlSchemaRedefine.cs
- SqlConnectionStringBuilder.cs
- IDReferencePropertyAttribute.cs
- ClientRuntimeConfig.cs
- Tool.cs
- UnsafeNativeMethods.cs
- ColumnBinding.cs
- GenerateTemporaryTargetAssembly.cs
- ProfileSettingsCollection.cs
- X509Utils.cs
- HttpModulesSection.cs
- ExtensionQuery.cs
- PopupRoot.cs
- TabItemWrapperAutomationPeer.cs
- TypographyProperties.cs
- TypefaceCollection.cs
- ECDiffieHellmanCngPublicKey.cs
- FlatButtonAppearance.cs
- SerializationTrace.cs
- BatchServiceHost.cs
- Renderer.cs
- XmlName.cs
- MonthCalendar.cs
- WindowsPrincipal.cs
- _OverlappedAsyncResult.cs
- IndexedGlyphRun.cs
- NameValueFileSectionHandler.cs
- DataGridViewRowHeaderCell.cs
- ObjectQueryState.cs
- PointAnimationUsingPath.cs
- PerfProviderCollection.cs
- TriggerBase.cs
- DragAssistanceManager.cs
- BufferedGraphicsContext.cs
- TextElement.cs
- WindowsFormsHost.cs
- DataBoundLiteralControl.cs
- ValueCollectionParameterReader.cs
- ValidationHelpers.cs
- KeyGestureConverter.cs
- QueryConverter.cs
- PageOrientation.cs
- QuaternionAnimation.cs
- LockedAssemblyCache.cs
- RegexStringValidator.cs