Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / Designer / WinForms / System / WinForms / Design / FolderNameEditor.cs / 1 / FolderNameEditor.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms.Design { using System; using System.Design; using System.ComponentModel; using Microsoft.Win32; using System.Diagnostics; using System.Diagnostics.CodeAnalysis; using System.Drawing.Design; using System.IO; using System.Security; using System.Security.Permissions; using System.Runtime.InteropServices; ////// /// /// public class FolderNameEditor : UITypeEditor { private FolderBrowser folderBrowser; ///Provides an editor /// for choosing a folder from the filesystem. ////// /// /// [SuppressMessage("Microsoft.Security", "CA2123:OverrideLinkDemandsShouldBeIdenticalToBase")] // everything in this assembly is full trust. public override object EditValue(ITypeDescriptorContext context, IServiceProvider provider, object value) { if (folderBrowser == null) { folderBrowser = new FolderBrowser(); InitializeDialog(folderBrowser); } if (folderBrowser.ShowDialog() != System.Windows.Forms.DialogResult.OK) { return value; } return folderBrowser.DirectoryPath; } ///[To be supplied.] ////// /// /// Retrieves the editing style of the Edit method. If the method /// is not supported, this will return None. /// [SuppressMessage("Microsoft.Security", "CA2123:OverrideLinkDemandsShouldBeIdenticalToBase")] // everything in this assembly is full trust. public override UITypeEditorEditStyle GetEditStyle(ITypeDescriptorContext context) { return UITypeEditorEditStyle.Modal; } ////// /// /// Initializes the folder browser dialog when it is created. This gives you /// an opportunity to configure the dialog as you please. The default /// implementation provides a generic folder browser. /// protected virtual void InitializeDialog(FolderBrowser folderBrowser) { } ////// /// /// protected sealed class FolderBrowser : Component { private static readonly int MAX_PATH = 260; // Root node of the tree view. private FolderBrowserFolder startLocation = FolderBrowserFolder.Desktop; // Browse info options private FolderBrowserStyles publicOptions = FolderBrowserStyles.RestrictToFilesystem; private UnsafeNativeMethods.BrowseInfos privateOptions = UnsafeNativeMethods.BrowseInfos.NewDialogStyle; // Description text to show. private string descriptionText = String.Empty; // Folder picked by the user. private string directoryPath = String.Empty; ///[To be supplied.] ////// /// /// The styles the folder browser will use when browsing /// folders. This should be a combination of flags from /// the FolderBrowserStyles enum. /// public FolderBrowserStyles Style { get { return publicOptions; } set { publicOptions = value; } } ////// /// /// Gets the directory path of the folder the user picked. /// public string DirectoryPath { get { return directoryPath; } } ////// /// /// Gets/sets the start location of the root node. /// public FolderBrowserFolder StartLocation { get { return startLocation; } set { startLocation = value; } } ////// /// /// public string Description { get { return descriptionText; } set { descriptionText = (value == null) ? String.Empty: value; } } ////// Gets or sets a description to show above the folders. Here you can provide instructions for /// selecting a folder. /// ////// /// Helper function that returns the IMalloc interface used by the shell. /// private static UnsafeNativeMethods.IMalloc GetSHMalloc() { UnsafeNativeMethods.IMalloc[] malloc = new UnsafeNativeMethods.IMalloc[1]; UnsafeNativeMethods.Shell32.SHGetMalloc(malloc); return malloc[0]; } ////// /// /// Shows the folder browser dialog. /// public DialogResult ShowDialog() { return ShowDialog(null); } ////// /// /// Shows the folder browser dialog with the specified owner. /// public DialogResult ShowDialog(IWin32Window owner) { IntPtr pidlRoot = IntPtr.Zero; // Get/find an owner HWND for this dialog IntPtr hWndOwner; if (owner != null) { hWndOwner = owner.Handle; } else { hWndOwner = UnsafeNativeMethods.GetActiveWindow(); } // Get the IDL for the specific startLocation UnsafeNativeMethods.Shell32.SHGetSpecialFolderLocation(hWndOwner, (int) startLocation, ref pidlRoot); if (pidlRoot == IntPtr.Zero) { return DialogResult.Cancel; } int mergedOptions = (int)publicOptions | (int)privateOptions; if ((mergedOptions & (int)UnsafeNativeMethods.BrowseInfos.NewDialogStyle) != 0) { Application.OleRequired(); } IntPtr pidlRet = IntPtr.Zero; try { // Construct a BROWSEINFO UnsafeNativeMethods.BROWSEINFO bi = new UnsafeNativeMethods.BROWSEINFO(); IntPtr buffer = Marshal.AllocHGlobal(MAX_PATH); bi.pidlRoot = pidlRoot; bi.hwndOwner = hWndOwner; bi.pszDisplayName = buffer; bi.lpszTitle = descriptionText; bi.ulFlags = mergedOptions; bi.lpfn = IntPtr.Zero; bi.lParam = IntPtr.Zero; bi.iImage = 0; // And show the dialog pidlRet = UnsafeNativeMethods.Shell32.SHBrowseForFolder(bi); if (pidlRet == IntPtr.Zero) { // User pressed Cancel return DialogResult.Cancel; } // Then retrieve the path from the IDList UnsafeNativeMethods.Shell32.SHGetPathFromIDList(pidlRet, buffer); // Convert to a string directoryPath = Marshal.PtrToStringAuto(buffer); // Then free all the stuff we've allocated or the SH API gave us Marshal.FreeHGlobal(buffer); } finally { UnsafeNativeMethods.IMalloc malloc = GetSHMalloc(); malloc.Free(pidlRoot); if (pidlRet != IntPtr.Zero) { malloc.Free(pidlRet); } } return DialogResult.OK; } } ////// protected enum FolderBrowserFolder { /// Desktop = 0x0000, /// Favorites = 0x0006, /// MyComputer = 0x0011, /// MyDocuments = 0x0005, /// MyPictures = 0x0027, /// NetAndDialUpConnections = 0x0031, /// NetworkNeighborhood = 0x0012, /// Printers = 0x0004, /// Recent = 0x0008, /// SendTo = 0x0009, /// StartMenu = 0x000b, /// Templates = 0x0015, } /// /// [Flags] protected enum FolderBrowserStyles { /// BrowseForComputer = UnsafeNativeMethods.BrowseInfos.BrowseForComputer, /// BrowseForEverything = UnsafeNativeMethods.BrowseInfos.BrowseForEverything, /// BrowseForPrinter = UnsafeNativeMethods.BrowseInfos.BrowseForPrinter, /// RestrictToDomain = UnsafeNativeMethods.BrowseInfos.DontGoBelowDomain, /// RestrictToFilesystem = UnsafeNativeMethods.BrowseInfos.ReturnOnlyFSDirs, /// RestrictToSubfolders = UnsafeNativeMethods.BrowseInfos.ReturnFSAncestors, /// ShowTextBox = UnsafeNativeMethods.BrowseInfos.EditBox, } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
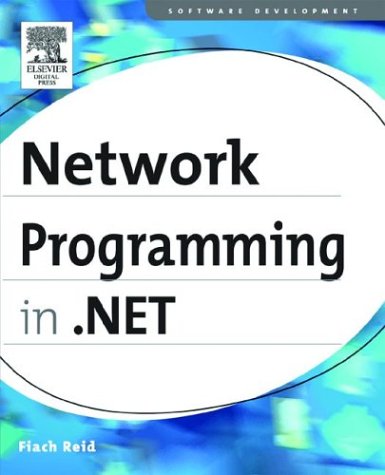
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- EntityCollection.cs
- ChildrenQuery.cs
- EntityDataSourceConfigureObjectContext.cs
- DataSourceView.cs
- SafeEventHandle.cs
- StsCommunicationException.cs
- WebCategoryAttribute.cs
- SQLGuidStorage.cs
- PathSegmentCollection.cs
- PageParserFilter.cs
- SecurityTokenTypes.cs
- ConnectionStringsSection.cs
- DropSource.cs
- MenuBase.cs
- JsonServiceDocumentSerializer.cs
- Image.cs
- OleDbConnectionPoolGroupProviderInfo.cs
- ImageListStreamer.cs
- RawTextInputReport.cs
- OdbcEnvironment.cs
- ObjectKeyFrameCollection.cs
- DoubleLinkList.cs
- DeflateInput.cs
- WindowsFormsHelpers.cs
- ArrayConverter.cs
- SeekStoryboard.cs
- BitmapData.cs
- MeasureData.cs
- ClassicBorderDecorator.cs
- FileDialog.cs
- UserControl.cs
- HtmlWindow.cs
- SocketElement.cs
- IndicShape.cs
- DesignerHierarchicalDataSourceView.cs
- DelimitedListTraceListener.cs
- DocumentPage.cs
- Size3DValueSerializer.cs
- SchemaElementLookUpTable.cs
- InvalidCastException.cs
- CharConverter.cs
- OdbcRowUpdatingEvent.cs
- CachingHintValidation.cs
- Form.cs
- SmiConnection.cs
- SerialErrors.cs
- DefinitionUpdate.cs
- TreeNodeBinding.cs
- DataViewManagerListItemTypeDescriptor.cs
- AssociatedControlConverter.cs
- TimelineClockCollection.cs
- ReliabilityContractAttribute.cs
- SingleStorage.cs
- TemplateBamlRecordReader.cs
- FunctionUpdateCommand.cs
- ObjectStateManager.cs
- ProfileSettings.cs
- ExpanderAutomationPeer.cs
- ADRoleFactory.cs
- MethodToken.cs
- precedingquery.cs
- SchemaImporter.cs
- basenumberconverter.cs
- DrawingVisual.cs
- FactoryGenerator.cs
- IconConverter.cs
- SessionStateContainer.cs
- MULTI_QI.cs
- KeyValueConfigurationElement.cs
- EmptyEnumerator.cs
- DesignerActionKeyboardBehavior.cs
- formatter.cs
- MDIWindowDialog.cs
- _emptywebproxy.cs
- OutputCacheSection.cs
- SessionPageStateSection.cs
- InputLangChangeRequestEvent.cs
- WindowsImpersonationContext.cs
- Calendar.cs
- Registry.cs
- ToolStripButton.cs
- ContentWrapperAttribute.cs
- PathGradientBrush.cs
- HttpClientCertificate.cs
- X509AudioLogo.cs
- MissingManifestResourceException.cs
- TextReader.cs
- DictionaryManager.cs
- GridErrorDlg.cs
- EntityException.cs
- DbParameterHelper.cs
- XmlNavigatorStack.cs
- NameTable.cs
- PasswordRecoveryDesigner.cs
- Property.cs
- IPEndPoint.cs
- TableItemPatternIdentifiers.cs
- EndpointAddressAugust2004.cs
- KeyEvent.cs
- DetailsView.cs