Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Print / Reach / Serialization / XpsImageSerializationService.cs / 1 / XpsImageSerializationService.cs
/*++ Copyright (C) 2004 - 2005 Microsoft Corporation All rights reserved. Module Name: XpsImageSerializationService.cs Abstract: This file implements the XpsImageSerializationService used by the Xps Serialization APIs for serializing images to a Xps package. Author: [....] ([....]) 1-December-2004 Revision History: 07/12/2005: [....]: Reach -> Xps --*/ using System; using System.IO; using System.Windows.Media; using System.Windows.Media.Imaging; using System.Security; using System.Security.Permissions; using System.Windows.Xps.Packaging; using MS.Internal; namespace System.Windows.Xps.Serialization { ////// This class implements a support service for serialization /// of BitmapSource instances to a Xps package. /// internal class XpsImageSerializationService { #region Public methods ////// This method retrieves the BitmapEncoder to be used for /// serialization based on the specified BitmapSource. /// /// /// A reference to a BitmapSource that will be encoded. /// ////// Returns a reference to a new BitmapEncoder. /// ////// Critical - 1) Asserts to access the registry. May return path information which /// could disclose windows directory (ie. c:\windows\media\sound.wav) /// /// [SecurityCritical] public BitmapEncoder GetEncoder( BitmapSource bitmapSource ) { BitmapEncoder encoder = null; if (bitmapSource is BitmapFrame) { // // This code gets the encoder based on the decoder that was // used for this specific BitmapSource. // BitmapFrame bitmapImage = bitmapSource as BitmapFrame; BitmapCodecInfo codecInfo = null; if (bitmapImage != null && bitmapImage.Decoder != null) codecInfo = bitmapImage.Decoder.CodecInfo; if (codecInfo != null) { (new RegistryPermission(PermissionState.Unrestricted)).Assert(); try { encoder = BitmapEncoder.Create(codecInfo.ContainerFormat); } finally { RegistryPermission.RevertAssert(); } // Avoid GIF encoder which does not save transparency well if ( !( encoder is JpegBitmapEncoder || encoder is PngBitmapEncoder || encoder is TiffBitmapEncoder || encoder is WmpBitmapEncoder) ) { encoder = null; } } } // // The code above assumes that the BitmapSource is actually // a BitmapImage. If it is not then we assume Png and use // that encoder. // if (encoder == null) { if (Microsoft.Internal.AlphaFlattener.Utility.NeedPremultiplyAlpha(bitmapSource)) { encoder = new WmpBitmapEncoder(); } else { encoder = new PngBitmapEncoder(); } } return encoder; } ////// This method determines if a bitmap is of a supported /// Xps Mime type /// /// /// A reference to a BitmapSource to be tested. /// ////// Returns true if the bitmapSource is of supported mimetype /// ////// Critical - 1) Asserts to access the registry. May return path information which /// could disclose windows directory (ie. c:\windows\media\sound.wav) /// /// [SecurityCritical] public bool IsSupportedMimeType( BitmapSource bitmapSource ) { BitmapCodecInfo codecInfo = null; string imageMimeType = ""; if (bitmapSource is BitmapFrame) { // // This code gets the encoder based on the decoder that was // used for this specific BitmapSource. // BitmapFrame bitmapFrame = bitmapSource as BitmapFrame; if (bitmapFrame != null && bitmapFrame.Decoder != null) { codecInfo = bitmapFrame.Decoder.CodecInfo; } } if (codecInfo != null) { (new RegistryPermission(PermissionState.Unrestricted)).Assert(); try { imageMimeType = codecInfo.MimeTypes; } finally { RegistryPermission.RevertAssert(); } } int start = 0; int comma = imageMimeType.IndexOf(',', start); bool foundType = false; // // Test all strings before commas // if( comma != -1 ) { while (comma != -1 && !foundType) { string subString = imageMimeType.Substring(start, comma); foundType = XpsManager.SupportedImageType( new ContentType(subString) ); start = comma+1; comma = imageMimeType.IndexOf(',', start); } } // // If we still have not found a supported type // Test the remainder of the string // if( !foundType ) { foundType = XpsManager.SupportedImageType( new ContentType(imageMimeType.Substring(start)) ); } return foundType; } ////// This method verifies whether a given BitmapSource /// is serializable by this service. /// /// /// A reference to a BitmapSource to be checked. /// ////// A boolean value specifing serializability. /// public bool VerifyImageSourceSerializability( BitmapSource bitmapSource ) { throw new NotImplementedException(); } ////// This method serializes a given BitmapSource to a /// stream and returns a reference to the stream. /// /// /// A reference to a BitmapSource to be serialized. /// ////// A reference to a Stream where BitmapSource was serialized. /// public Stream SerializeToStream( BitmapSource bitmapSource ) { throw new NotImplementedException(); } #endregion Public methods } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
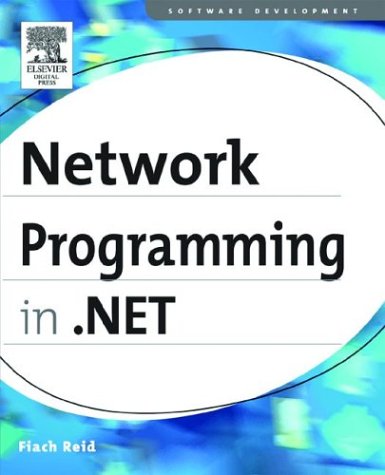
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- WinEventTracker.cs
- TreeSet.cs
- ExtendedProtectionPolicy.cs
- QueryExtender.cs
- DecimalMinMaxAggregationOperator.cs
- BufferModesCollection.cs
- ResourcesChangeInfo.cs
- MetafileHeaderEmf.cs
- XmlSchemaType.cs
- ToolboxItemAttribute.cs
- Viewport2DVisual3D.cs
- StoreItemCollection.cs
- ContainerTracking.cs
- DetailsView.cs
- PowerEase.cs
- ConfigErrorGlyph.cs
- TabletDeviceInfo.cs
- BuiltInPermissionSets.cs
- NoneExcludedImageIndexConverter.cs
- ConnectionPointCookie.cs
- TableLayoutColumnStyleCollection.cs
- HttpModulesSection.cs
- Shape.cs
- DocumentSignatureManager.cs
- BrowserCapabilitiesCodeGenerator.cs
- NavigateEvent.cs
- StrokeCollectionDefaultValueFactory.cs
- TokenBasedSet.cs
- TextTreePropertyUndoUnit.cs
- PtsCache.cs
- UniqueEventHelper.cs
- DbCommandDefinition.cs
- DataBindEngine.cs
- WindowsTokenRoleProvider.cs
- TransformerInfo.cs
- _FtpDataStream.cs
- LinkedResourceCollection.cs
- ActiveXHelper.cs
- DocumentCollection.cs
- StandardBindingCollectionElement.cs
- CancelEventArgs.cs
- ApplicationException.cs
- CloseSequenceResponse.cs
- IListConverters.cs
- IRCollection.cs
- _DisconnectOverlappedAsyncResult.cs
- RawStylusActions.cs
- IResourceProvider.cs
- KoreanLunisolarCalendar.cs
- ResourceExpressionBuilder.cs
- X509UI.cs
- DrawingDrawingContext.cs
- ResourceProviderFactory.cs
- SmtpSection.cs
- DataGridHelper.cs
- SafeLocalMemHandle.cs
- FontFamilyValueSerializer.cs
- HostingEnvironmentSection.cs
- CodeChecksumPragma.cs
- ReadOnlyObservableCollection.cs
- ThreadAbortException.cs
- Vector.cs
- ResourceWriter.cs
- EncoderParameter.cs
- PingOptions.cs
- Visual3D.cs
- TagPrefixCollection.cs
- FontCollection.cs
- Vector3DAnimationUsingKeyFrames.cs
- DBNull.cs
- XmlSchemaValidator.cs
- XmlText.cs
- ISAPIRuntime.cs
- XhtmlBasicSelectionListAdapter.cs
- ServerValidateEventArgs.cs
- StateMachineSubscriptionManager.cs
- ToolStripContentPanel.cs
- VirtualPathProvider.cs
- StrokeSerializer.cs
- MailWebEventProvider.cs
- TimelineCollection.cs
- XmlDataLoader.cs
- DisableDpiAwarenessAttribute.cs
- HtmlInputHidden.cs
- Dispatcher.cs
- wgx_exports.cs
- ConvertersCollection.cs
- XmlSignatureManifest.cs
- ThreadPoolTaskScheduler.cs
- TextRange.cs
- Exceptions.cs
- Camera.cs
- UdpDiscoveryMessageFilter.cs
- _TLSstream.cs
- UpdateProgress.cs
- CodeGeneratorAttribute.cs
- CollectionViewGroupInternal.cs
- FileChangesMonitor.cs
- SystemColors.cs
- ItemAutomationPeer.cs